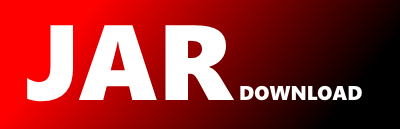
org.apache.cayenne.conf.RuntimeLoadDelegate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cayenne-client-nodeps
Show all versions of cayenne-client-nodeps
Cayenne Object Persistence Framework
/*****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
****************************************************************/
package org.apache.cayenne.conf;
import java.io.InputStream;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import javax.sql.DataSource;
import org.apache.log4j.Level;
import org.apache.log4j.Logger;
import org.apache.cayenne.ConfigurationException;
import org.apache.cayenne.access.DataDomain;
import org.apache.cayenne.access.DataNode;
import org.apache.cayenne.dba.AutoAdapter;
import org.apache.cayenne.dba.DbAdapter;
import org.apache.cayenne.map.DataMap;
import org.apache.cayenne.map.MapLoader;
import org.xml.sax.InputSource;
/**
* Implementation of ConfigLoaderDelegate that creates Cayenne access objects stack.
*
* @author Andrus Adamchik
*/
public class RuntimeLoadDelegate implements ConfigLoaderDelegate {
// TODO: andrus, 7/17/2006 - these variables, and project upgrade logic should be
// refactored out of the MapLoader. In fact we should either modify raw XML during the
// upgrade, or implement some consistent upgrade API across variou loaders
final static String _1_2_PACKAGE_PREFIX = "org.objectstyle.cayenne.";
final static String _2_0_PACKAGE_PREFIX = "org.apache.cayenne.";
private static Logger logObj = Logger.getLogger(RuntimeLoadDelegate.class);
protected Map domains = new HashMap();
protected Map views = new HashMap();
protected ConfigStatus status;
protected Configuration config;
protected long startTime;
/**
* @deprecated since 1.2
*/
public RuntimeLoadDelegate(Configuration config, ConfigStatus status, Level logLevel) {
this(config, status);
}
public RuntimeLoadDelegate(Configuration config, ConfigStatus status) {
this.config = config;
if (status == null) {
status = new ConfigStatus();
}
this.status = status;
}
protected DataDomain findDomain(String name) throws FindException {
DataDomain domain = (DataDomain) domains.get(name);
if (domain == null) {
throw new FindException("Can't find DataDomain: " + name);
}
return domain;
}
protected DataMap findMap(String domainName, String mapName) throws FindException {
DataDomain domain = findDomain(domainName);
DataMap map = domain.getMap(mapName);
if (map == null) {
throw new FindException("Can't find DataMap: " + mapName);
}
return map;
}
protected DataNode findNode(String domainName, String nodeName) throws FindException {
DataDomain domain = findDomain(domainName);
DataNode node = domain.getNode(nodeName);
if (node == null) {
throw new FindException("Can't find DataNode: " + nodeName);
}
return node;
}
public boolean loadError(Throwable th) {
logObj.info("Parser Exception.", th);
status.getOtherFailures().add(th.getMessage());
return false;
}
/**
* @since 1.1
*/
public void shouldLoadProjectVersion(String version) {
config.setProjectVersion(version);
}
/**
* @since 1.1
*/
public void shouldRegisterDataView(String name, String location) {
views.put(name, location);
}
public void shouldLoadDataDomainProperties(String domainName, Map properties) {
if (properties == null || properties.isEmpty()) {
return;
}
DataDomain domain = null;
try {
domain = findDomain(domainName);
}
catch (FindException ex) {
logObj.info("Error: Domain is not loaded: " + domainName);
throw new ConfigurationException("Domain is not loaded: " + domainName);
}
domain.initWithProperties(properties);
}
public void shouldLoadDataDomain(String domainName) {
if (domainName == null) {
logObj.info("Error: unnamed .");
throw new ConfigurationException("Domain 'name' attribute must be not null.");
}
logObj.info("loaded domain: " + domainName);
domains.put(domainName, new DataDomain(domainName));
}
public void shouldLoadDataMaps(String domainName, Map locations) {
if (locations.size() == 0) {
return;
}
DataDomain domain = null;
try {
domain = findDomain(domainName);
}
catch (FindException ex) {
logObj.info("Error: Domain is not loaded: " + domainName);
throw new ConfigurationException("Domain is not loaded: " + domainName);
}
// load DataMaps tree
Iterator it = locations.keySet().iterator();
while (it.hasNext()) {
String name = (String) it.next();
DataMap map = domain.getMap(name);
if (map != null) {
continue;
}
loadDataMap(domain, name, locations);
}
}
/**
* Returns DataMap for the name and location information. If a DataMap is already
* loaded within a given domain, such loaded map is returned, otherwise the map is
* loaded and linked with the DataDomain.
*/
protected DataMap loadDataMap(DataDomain domain, String mapName, Map locations) {
if (mapName == null) {
throw new ConfigurationException("Error:
© 2015 - 2024 Weber Informatics LLC | Privacy Policy