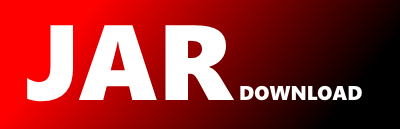
org.apache.cayenne.access.trans.UpdateTranslator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cayenne-client-nodeps
Show all versions of cayenne-client-nodeps
Cayenne Object Persistence Framework
/*****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
****************************************************************/
package org.apache.cayenne.access.trans;
import java.util.Iterator;
import java.util.Map;
import org.apache.cayenne.CayenneRuntimeException;
import org.apache.cayenne.map.DbAttribute;
import org.apache.cayenne.map.DbEntity;
import org.apache.cayenne.map.DbRelationship;
import org.apache.cayenne.query.UpdateQuery;
/** Class implements default translation mechanism of
* org.apache.cayenne.query.UpdateQuery
* objects to SQL UPDATE statements.
*
* @author Andrei Adamchik
*/
public class UpdateTranslator extends QueryAssembler {
public String aliasForTable(DbEntity dbEnt) {
throw new RuntimeException("aliases not supported");
}
public void dbRelationshipAdded(DbRelationship dbRel) {
throw new RuntimeException("db relationships not supported");
}
/** Method that converts an update query into SQL string */
public String createSqlString() throws Exception {
StringBuffer queryBuf = new StringBuffer();
queryBuf.append("UPDATE ");
// 1. append table name
DbEntity dbEnt = getRootEntity().getDbEntity();
queryBuf.append(dbEnt.getFullyQualifiedName());
// 2. build "set ..." clause
buildSetClause(queryBuf, (UpdateQuery) query);
// 3. build qualifier
String qualifierStr =
adapter.getQualifierTranslator(this).doTranslation();
if (qualifierStr != null)
queryBuf.append(" WHERE ").append(qualifierStr);
return queryBuf.toString();
}
/** Translate updated values and relationships into
* "SET ATTR1 = Val1, ..." SQL statement.
*/
private void buildSetClause(StringBuffer queryBuf, UpdateQuery query) {
Map updAttrs = query.getUpdAttributes();
// set of keys.. each key is supposed to be ObjAttribute
Iterator attrIt = updAttrs.entrySet().iterator();
if (!attrIt.hasNext())
throw new CayenneRuntimeException("Nothing to update.");
DbEntity dbEnt = getRootEntity().getDbEntity();
queryBuf.append(" SET ");
// append updated attribute values
boolean appendedSomething = false;
// now process other attrs in the loop
while (attrIt.hasNext()) {
Map.Entry entry = (Map.Entry) attrIt.next();
String nextKey = (String) entry.getKey();
Object attrVal = entry.getValue();
if (appendedSomething)
queryBuf.append(", ");
queryBuf.append(nextKey).append(" = ?");
super.addToParamList(
(DbAttribute) dbEnt.getAttribute(nextKey),
attrVal);
appendedSomething = true;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy