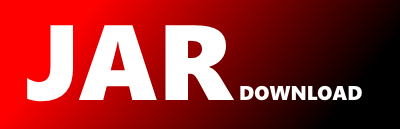
org.apache.cayenne.conf.FileConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cayenne-client-nodeps
Show all versions of cayenne-client-nodeps
Cayenne Object Persistence Framework
/*****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
****************************************************************/
package org.apache.cayenne.conf;
import java.io.File;
import org.apache.log4j.Logger;
import org.apache.cayenne.ConfigurationException;
import org.apache.cayenne.util.ResourceLocator;
/**
* FileConfiguration loads a Cayenne configuraton file from a given
* location in the file system.
*
* @author Holger Hoffstaette
*/
public class FileConfiguration extends DefaultConfiguration {
private static Logger logObj = Logger.getLogger(FileConfiguration.class);
/**
* The domain file used for this configuration
*/
protected File projectFile;
/**
* Default constructor.
* Simply calls {@link FileConfiguration#FileConfiguration(String)}
* with {@link Configuration#DEFAULT_DOMAIN_FILE} as argument.
* @see DefaultConfiguration#DefaultConfiguration()
*/
public FileConfiguration() {
this(Configuration.DEFAULT_DOMAIN_FILE);
}
/**
* Creates a configuration that uses the provided file name
* as the main project file, ignoring any other lookup strategies.
* The file name is not checked for existence and must not
* contain relative or absolute paths, i.e. only the file name.
*
* @throws ConfigurationException when projectFile is null
.
* @see DefaultConfiguration#DefaultConfiguration(String)
*/
public FileConfiguration(String domainConfigurationName) {
super(domainConfigurationName);
// set the project file
this.projectFile = new File(domainConfigurationName);
// configure the ResourceLocator for plain files
ResourceLocator locator = this.getResourceLocator();
locator.setSkipAbsolutePath(false);
locator.setSkipClasspath(true);
locator.setSkipCurrentDirectory(false);
locator.setSkipHomeDirectory(true);
// add the file's location to the search path, if it exists
File projectDirectory = this.getProjectDirectory();
if (projectDirectory != null) {
locator.addFilesystemPath(projectDirectory.getPath());
}
}
/**
* Creates a configuration that uses the provided file
* as the main project file, ignoring any other lookup strategies.
*
* @throws ConfigurationException when projectFile is null
,
* a directory or not readable.
*/
public FileConfiguration(File domainConfigurationFile) {
super();
logObj.debug("using domain file: " + domainConfigurationFile);
// set the project file
this.setProjectFile(domainConfigurationFile);
// configure the ResourceLocator for plain files
ResourceLocator locator = this.getResourceLocator();
locator.setSkipAbsolutePath(false);
locator.setSkipClasspath(true);
locator.setSkipCurrentDirectory(false);
locator.setSkipHomeDirectory(true);
// add the file's location to the search path, if it exists
File projectDirectory = this.getProjectDirectory();
if (projectDirectory != null) {
locator.addFilesystemPath(projectDirectory);
}
}
/**
* Adds the given String as a custom path for filesystem lookups.
* The path can be relative or absolute and is not checked
* for existence.
*
* This allows for easy customization of resource search paths after
* Constructor invocation:
*
* conf = new FileConfiguration("myconfig-cayenne.xml");
* conf.addFilesystemPath(new File("a/relative/path"));
* conf.addFilesystemPath(new File("/an/absolute/search/path"));
* Configuration.initializeSharedConfiguration(conf);
*
*
* Alternatively use {@link FileConfiguration#addFilesystemPath(File)}
* for adding a path that is checked for existence.
*
* @throws IllegalArgumentException if path
is null
.
*/
public void addFilesystemPath(String path) {
this.getResourceLocator().addFilesystemPath(path);
}
/**
* Adds the given directory as a path for filesystem lookups.
* The directory is checked for existence.
*
* @throws IllegalArgumentException if path
is null
,
* not a directory or not readable.
*/
public void addFilesystemPath(File path) {
this.getResourceLocator().addFilesystemPath(path);
}
/**
* Only returns true
when {@link #getProjectFile} does not
* return null
.
*/
public boolean canInitialize() {
// I can only initialize myself when I have a valid file
return (this.getProjectFile() != null);
}
/**
* Returns the main domain file used for this configuration.
*/
public File getProjectFile() {
return projectFile;
}
/**
* Sets the main domain file used for this configuration.
* @throws ConfigurationException if projectFile
is null,
* a directory or not readable.
*/
protected void setProjectFile(File projectFile) {
if (projectFile != null) {
if (projectFile.isFile()) {
this.projectFile = projectFile;
this.setDomainConfigurationName(projectFile.getName());
}
else {
throw new ConfigurationException("Project file: "
+ projectFile
+ " is a directory or not readable.");
}
}
else {
throw new ConfigurationException("Cannot use null as project file.");
}
}
/**
* Returns the directory of the current project file as
* returned by {@link #getProjectFile}.
*/
public File getProjectDirectory() {
File pfile = this.getProjectFile();
if (pfile != null) {
return pfile.getParentFile();
}
else {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy