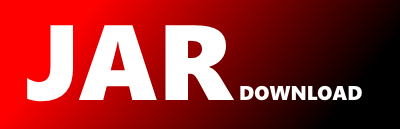
org.apache.cayenne.exp.parser.ExpressionParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cayenne-client-nodeps
Show all versions of cayenne-client-nodeps
Cayenne Object Persistence Framework
/* Generated By:JJTree&JavaCC: Do not edit this line. ExpressionParser.java */
/*****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
****************************************************************/
package org.apache.cayenne.exp.parser;
import java.io.*;
import java.util.*;
import java.math.*;
import org.apache.cayenne.exp.*;
/**
* Parser of Cayenne Expressions.
*
* @since 1.1
*/
public class ExpressionParser/*@bgen(jjtree)*/implements ExpressionParserTreeConstants, ExpressionParserConstants {/*@bgen(jjtree)*/
protected JJTExpressionParserState jjtree = new JJTExpressionParserState();public static void main(String[] arg) {
// since Main is used for some basic speed measuring,
// lets run it twice to "warm up" the parser
Expression.fromString(arg[0]);
long start = System.currentTimeMillis();
Expression exp = Expression.fromString(arg[0]);
long end = System.currentTimeMillis();
System.out.println(exp);
System.out.println("Parsed in " + (end - start) + " ms.");
}
final public Expression expression() throws ParseException {
orCondition();
jj_consume_token(0);
{if (true) return (Expression) jjtree.rootNode();}
throw new Error("Missing return statement in function");
}
final public void orCondition() throws ParseException {
andCondition();
label_1:
while (true) {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 1:
;
break;
default:
jj_la1[0] = jj_gen;
break label_1;
}
jj_consume_token(1);
ASTOr jjtn001 = new ASTOr(JJTOR);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
andCondition();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
}
}
final public void andCondition() throws ParseException {
notCondition();
label_2:
while (true) {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 2:
;
break;
default:
jj_la1[1] = jj_gen;
break label_2;
}
jj_consume_token(2);
ASTAnd jjtn001 = new ASTAnd(JJTAND);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
notCondition();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
}
}
final public void notCondition() throws ParseException {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 3:
case 4:
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 3:
jj_consume_token(3);
break;
case 4:
jj_consume_token(4);
break;
default:
jj_la1[2] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
ASTNot jjtn001 = new ASTNot(JJTNOT);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
simpleCondition();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 1);
}
}
break;
case 16:
case 20:
case 21:
case 24:
case 25:
case 26:
case NULL:
case PROPERTY_PATH:
case SINGLE_QUOTED_STRING:
case DOUBLE_QUOTED_STRING:
case INT_LITERAL:
case FLOAT_LITERAL:
simpleCondition();
break;
default:
jj_la1[3] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
final public void simpleCondition() throws ParseException {
scalarExpression();
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 3:
case 4:
case 5:
case 6:
case 7:
case 8:
case 9:
case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
case 18:
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 3:
case 4:
simpleNotCondition();
break;
case 5:
case 6:
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 5:
jj_consume_token(5);
break;
case 6:
jj_consume_token(6);
break;
default:
jj_la1[4] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
ASTEqual jjtn001 = new ASTEqual(JJTEQUAL);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
scalarExpression();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
break;
case 7:
case 8:
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 7:
jj_consume_token(7);
break;
case 8:
jj_consume_token(8);
break;
default:
jj_la1[5] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
ASTNotEqual jjtn002 = new ASTNotEqual(JJTNOTEQUAL);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
scalarExpression();
} catch (Throwable jjte002) {
if (jjtc002) {
jjtree.clearNodeScope(jjtn002);
jjtc002 = false;
} else {
jjtree.popNode();
}
if (jjte002 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte002;}
}
if (jjte002 instanceof ParseException) {
{if (true) throw (ParseException)jjte002;}
}
{if (true) throw (Error)jjte002;}
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, 2);
}
}
break;
case 9:
jj_consume_token(9);
ASTLessOrEqual jjtn003 = new ASTLessOrEqual(JJTLESSOREQUAL);
boolean jjtc003 = true;
jjtree.openNodeScope(jjtn003);
try {
scalarExpression();
} catch (Throwable jjte003) {
if (jjtc003) {
jjtree.clearNodeScope(jjtn003);
jjtc003 = false;
} else {
jjtree.popNode();
}
if (jjte003 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte003;}
}
if (jjte003 instanceof ParseException) {
{if (true) throw (ParseException)jjte003;}
}
{if (true) throw (Error)jjte003;}
} finally {
if (jjtc003) {
jjtree.closeNodeScope(jjtn003, 2);
}
}
break;
case 10:
jj_consume_token(10);
ASTLess jjtn004 = new ASTLess(JJTLESS);
boolean jjtc004 = true;
jjtree.openNodeScope(jjtn004);
try {
scalarExpression();
} catch (Throwable jjte004) {
if (jjtc004) {
jjtree.clearNodeScope(jjtn004);
jjtc004 = false;
} else {
jjtree.popNode();
}
if (jjte004 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte004;}
}
if (jjte004 instanceof ParseException) {
{if (true) throw (ParseException)jjte004;}
}
{if (true) throw (Error)jjte004;}
} finally {
if (jjtc004) {
jjtree.closeNodeScope(jjtn004, 2);
}
}
break;
case 11:
jj_consume_token(11);
ASTGreater jjtn005 = new ASTGreater(JJTGREATER);
boolean jjtc005 = true;
jjtree.openNodeScope(jjtn005);
try {
scalarExpression();
} catch (Throwable jjte005) {
if (jjtc005) {
jjtree.clearNodeScope(jjtn005);
jjtc005 = false;
} else {
jjtree.popNode();
}
if (jjte005 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte005;}
}
if (jjte005 instanceof ParseException) {
{if (true) throw (ParseException)jjte005;}
}
{if (true) throw (Error)jjte005;}
} finally {
if (jjtc005) {
jjtree.closeNodeScope(jjtn005, 2);
}
}
break;
case 12:
jj_consume_token(12);
ASTGreaterOrEqual jjtn006 = new ASTGreaterOrEqual(JJTGREATEROREQUAL);
boolean jjtc006 = true;
jjtree.openNodeScope(jjtn006);
try {
scalarExpression();
} catch (Throwable jjte006) {
if (jjtc006) {
jjtree.clearNodeScope(jjtn006);
jjtc006 = false;
} else {
jjtree.popNode();
}
if (jjte006 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte006;}
}
if (jjte006 instanceof ParseException) {
{if (true) throw (ParseException)jjte006;}
}
{if (true) throw (Error)jjte006;}
} finally {
if (jjtc006) {
jjtree.closeNodeScope(jjtn006, 2);
}
}
break;
case 13:
jj_consume_token(13);
ASTLike jjtn007 = new ASTLike(JJTLIKE);
boolean jjtc007 = true;
jjtree.openNodeScope(jjtn007);
try {
scalarExpression();
} catch (Throwable jjte007) {
if (jjtc007) {
jjtree.clearNodeScope(jjtn007);
jjtc007 = false;
} else {
jjtree.popNode();
}
if (jjte007 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte007;}
}
if (jjte007 instanceof ParseException) {
{if (true) throw (ParseException)jjte007;}
}
{if (true) throw (Error)jjte007;}
} finally {
if (jjtc007) {
jjtree.closeNodeScope(jjtn007, 2);
}
}
break;
case 14:
jj_consume_token(14);
ASTLikeIgnoreCase jjtn008 = new ASTLikeIgnoreCase(JJTLIKEIGNORECASE);
boolean jjtc008 = true;
jjtree.openNodeScope(jjtn008);
try {
scalarExpression();
} catch (Throwable jjte008) {
if (jjtc008) {
jjtree.clearNodeScope(jjtn008);
jjtc008 = false;
} else {
jjtree.popNode();
}
if (jjte008 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte008;}
}
if (jjte008 instanceof ParseException) {
{if (true) throw (ParseException)jjte008;}
}
{if (true) throw (Error)jjte008;}
} finally {
if (jjtc008) {
jjtree.closeNodeScope(jjtn008, 2);
}
}
break;
case 15:
jj_consume_token(15);
ASTIn jjtn009 = new ASTIn(JJTIN);
boolean jjtc009 = true;
jjtree.openNodeScope(jjtn009);
try {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 24:
namedParameter();
break;
case 16:
jj_consume_token(16);
scalarCommaList();
jj_consume_token(17);
break;
default:
jj_la1[6] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} catch (Throwable jjte009) {
if (jjtc009) {
jjtree.clearNodeScope(jjtn009);
jjtc009 = false;
} else {
jjtree.popNode();
}
if (jjte009 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte009;}
}
if (jjte009 instanceof ParseException) {
{if (true) throw (ParseException)jjte009;}
}
{if (true) throw (Error)jjte009;}
} finally {
if (jjtc009) {
jjtree.closeNodeScope(jjtn009, 2);
}
}
break;
case 18:
jj_consume_token(18);
scalarExpression();
jj_consume_token(2);
ASTBetween jjtn010 = new ASTBetween(JJTBETWEEN);
boolean jjtc010 = true;
jjtree.openNodeScope(jjtn010);
try {
scalarExpression();
} catch (Throwable jjte010) {
if (jjtc010) {
jjtree.clearNodeScope(jjtn010);
jjtc010 = false;
} else {
jjtree.popNode();
}
if (jjte010 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte010;}
}
if (jjte010 instanceof ParseException) {
{if (true) throw (ParseException)jjte010;}
}
{if (true) throw (Error)jjte010;}
} finally {
if (jjtc010) {
jjtree.closeNodeScope(jjtn010, 3);
}
}
break;
default:
jj_la1[7] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
default:
jj_la1[8] = jj_gen;
;
}
}
final public void simpleNotCondition() throws ParseException {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 3:
jj_consume_token(3);
break;
case 4:
jj_consume_token(4);
break;
default:
jj_la1[9] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 13:
jj_consume_token(13);
ASTNotLike jjtn001 = new ASTNotLike(JJTNOTLIKE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
scalarExpression();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
break;
case 14:
jj_consume_token(14);
ASTNotLikeIgnoreCase jjtn002 = new ASTNotLikeIgnoreCase(JJTNOTLIKEIGNORECASE);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
scalarExpression();
} catch (Throwable jjte002) {
if (jjtc002) {
jjtree.clearNodeScope(jjtn002);
jjtc002 = false;
} else {
jjtree.popNode();
}
if (jjte002 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte002;}
}
if (jjte002 instanceof ParseException) {
{if (true) throw (ParseException)jjte002;}
}
{if (true) throw (Error)jjte002;}
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, 2);
}
}
break;
case 15:
jj_consume_token(15);
ASTNotIn jjtn003 = new ASTNotIn(JJTNOTIN);
boolean jjtc003 = true;
jjtree.openNodeScope(jjtn003);
try {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 24:
namedParameter();
break;
case 16:
jj_consume_token(16);
scalarCommaList();
jj_consume_token(17);
break;
default:
jj_la1[10] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} catch (Throwable jjte003) {
if (jjtc003) {
jjtree.clearNodeScope(jjtn003);
jjtc003 = false;
} else {
jjtree.popNode();
}
if (jjte003 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte003;}
}
if (jjte003 instanceof ParseException) {
{if (true) throw (ParseException)jjte003;}
}
{if (true) throw (Error)jjte003;}
} finally {
if (jjtc003) {
jjtree.closeNodeScope(jjtn003, 2);
}
}
break;
case 18:
jj_consume_token(18);
scalarExpression();
jj_consume_token(2);
ASTNotBetween jjtn004 = new ASTNotBetween(JJTNOTBETWEEN);
boolean jjtc004 = true;
jjtree.openNodeScope(jjtn004);
try {
scalarExpression();
} catch (Throwable jjte004) {
if (jjtc004) {
jjtree.clearNodeScope(jjtn004);
jjtc004 = false;
} else {
jjtree.popNode();
}
if (jjte004 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte004;}
}
if (jjte004 instanceof ParseException) {
{if (true) throw (ParseException)jjte004;}
}
{if (true) throw (Error)jjte004;}
} finally {
if (jjtc004) {
jjtree.closeNodeScope(jjtn004, 3);
}
}
break;
default:
jj_la1[11] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
final public void scalarCommaList() throws ParseException {
ASTList jjtn001 = new ASTList(JJTLIST);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
scalarConstExpression();
label_3:
while (true) {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 19:
;
break;
default:
jj_la1[12] = jj_gen;
break label_3;
}
jj_consume_token(19);
scalarConstExpression();
}
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, true);
}
}
}
final public void scalarExpression() throws ParseException {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 16:
case 20:
case 21:
case 24:
case 25:
case 26:
case PROPERTY_PATH:
case INT_LITERAL:
case FLOAT_LITERAL:
scalarNumericExpression();
break;
case SINGLE_QUOTED_STRING:
jj_consume_token(SINGLE_QUOTED_STRING);
ASTScalar jjtn001 = new ASTScalar(JJTSCALAR);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
jjtree.closeNodeScope(jjtn001, 0);
jjtc001 = false;
jjtn001.setValue(token_source.literalValue);
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 0);
}
}
break;
case DOUBLE_QUOTED_STRING:
jj_consume_token(DOUBLE_QUOTED_STRING);
ASTScalar jjtn002 = new ASTScalar(JJTSCALAR);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
jjtree.closeNodeScope(jjtn002, 0);
jjtc002 = false;
jjtn002.setValue(token_source.literalValue);
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, 0);
}
}
break;
case NULL:
ASTScalar jjtn003 = new ASTScalar(JJTSCALAR);
boolean jjtc003 = true;
jjtree.openNodeScope(jjtn003);
try {
jj_consume_token(NULL);
} finally {
if (jjtc003) {
jjtree.closeNodeScope(jjtn003, 0);
}
}
break;
default:
jj_la1[13] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
final public void scalarConstExpression() throws ParseException {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case SINGLE_QUOTED_STRING:
jj_consume_token(SINGLE_QUOTED_STRING);
ASTScalar jjtn001 = new ASTScalar(JJTSCALAR);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
jjtree.closeNodeScope(jjtn001, 0);
jjtc001 = false;
jjtn001.setValue(token_source.literalValue);
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 0);
}
}
break;
case DOUBLE_QUOTED_STRING:
jj_consume_token(DOUBLE_QUOTED_STRING);
ASTScalar jjtn002 = new ASTScalar(JJTSCALAR);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
jjtree.closeNodeScope(jjtn002, 0);
jjtc002 = false;
jjtn002.setValue(token_source.literalValue);
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, 0);
}
}
break;
case 24:
namedParameter();
break;
case INT_LITERAL:
jj_consume_token(INT_LITERAL);
ASTScalar jjtn003 = new ASTScalar(JJTSCALAR);
boolean jjtc003 = true;
jjtree.openNodeScope(jjtn003);
try {
jjtree.closeNodeScope(jjtn003, 0);
jjtc003 = false;
jjtn003.setValue(token_source.literalValue);
} finally {
if (jjtc003) {
jjtree.closeNodeScope(jjtn003, 0);
}
}
break;
case FLOAT_LITERAL:
jj_consume_token(FLOAT_LITERAL);
ASTScalar jjtn004 = new ASTScalar(JJTSCALAR);
boolean jjtc004 = true;
jjtree.openNodeScope(jjtn004);
try {
jjtree.closeNodeScope(jjtn004, 0);
jjtc004 = false;
jjtn004.setValue(token_source.literalValue);
} finally {
if (jjtc004) {
jjtree.closeNodeScope(jjtn004, 0);
}
}
break;
default:
jj_la1[14] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
final public void scalarNumericExpression() throws ParseException {
multiplySubtractExp();
label_4:
while (true) {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 20:
case 21:
;
break;
default:
jj_la1[15] = jj_gen;
break label_4;
}
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 20:
jj_consume_token(20);
ASTAdd jjtn001 = new ASTAdd(JJTADD);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
multiplySubtractExp();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
break;
case 21:
jj_consume_token(21);
ASTSubtract jjtn002 = new ASTSubtract(JJTSUBTRACT);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
multiplySubtractExp();
} catch (Throwable jjte002) {
if (jjtc002) {
jjtree.clearNodeScope(jjtn002);
jjtc002 = false;
} else {
jjtree.popNode();
}
if (jjte002 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte002;}
}
if (jjte002 instanceof ParseException) {
{if (true) throw (ParseException)jjte002;}
}
{if (true) throw (Error)jjte002;}
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, 2);
}
}
break;
default:
jj_la1[16] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
final public void multiplySubtractExp() throws ParseException {
numericTerm();
label_5:
while (true) {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 22:
case 23:
;
break;
default:
jj_la1[17] = jj_gen;
break label_5;
}
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 22:
jj_consume_token(22);
ASTMultiply jjtn001 = new ASTMultiply(JJTMULTIPLY);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
numericTerm();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
break;
case 23:
jj_consume_token(23);
ASTDivide jjtn002 = new ASTDivide(JJTDIVIDE);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
numericTerm();
} catch (Throwable jjte002) {
if (jjtc002) {
jjtree.clearNodeScope(jjtn002);
jjtc002 = false;
} else {
jjtree.popNode();
}
if (jjte002 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte002;}
}
if (jjte002 instanceof ParseException) {
{if (true) throw (ParseException)jjte002;}
}
{if (true) throw (Error)jjte002;}
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, 2);
}
}
break;
default:
jj_la1[18] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
final public void numericTerm() throws ParseException {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 16:
case 20:
case 24:
case 25:
case 26:
case PROPERTY_PATH:
case INT_LITERAL:
case FLOAT_LITERAL:
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 20:
jj_consume_token(20);
break;
default:
jj_la1[19] = jj_gen;
;
}
numericPrimary();
break;
case 21:
jj_consume_token(21);
ASTNegate jjtn001 = new ASTNegate(JJTNEGATE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
numericPrimary();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 1);
}
}
break;
default:
jj_la1[20] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
final public void numericPrimary() throws ParseException {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 16:
jj_consume_token(16);
orCondition();
jj_consume_token(17);
break;
case 25:
case 26:
case PROPERTY_PATH:
pathExpression();
break;
case 24:
namedParameter();
break;
case INT_LITERAL:
jj_consume_token(INT_LITERAL);
ASTScalar jjtn001 = new ASTScalar(JJTSCALAR);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
jjtree.closeNodeScope(jjtn001, 0);
jjtc001 = false;
jjtn001.setValue(token_source.literalValue);
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 0);
}
}
break;
case FLOAT_LITERAL:
jj_consume_token(FLOAT_LITERAL);
ASTScalar jjtn002 = new ASTScalar(JJTSCALAR);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
jjtree.closeNodeScope(jjtn002, 0);
jjtc002 = false;
jjtn002.setValue(token_source.literalValue);
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, 0);
}
}
break;
default:
jj_la1[21] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
final public void namedParameter() throws ParseException {
Token t;
jj_consume_token(24);
t = jj_consume_token(PROPERTY_PATH);
ASTNamedParameter jjtn001 = new ASTNamedParameter(JJTNAMEDPARAMETER);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
jjtree.closeNodeScope(jjtn001, 0);
jjtc001 = false;
jjtn001.setValue(t.image);
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 0);
}
}
}
final public void pathExpression() throws ParseException {
Token t;
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case PROPERTY_PATH:
t = jj_consume_token(PROPERTY_PATH);
ASTObjPath jjtn001 = new ASTObjPath(JJTOBJPATH);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
jjtree.closeNodeScope(jjtn001, 0);
jjtc001 = false;
jjtn001.setPath(t.image);
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 0);
}
}
break;
case 25:
jj_consume_token(25);
t = jj_consume_token(PROPERTY_PATH);
ASTObjPath jjtn002 = new ASTObjPath(JJTOBJPATH);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
jjtree.closeNodeScope(jjtn002, 0);
jjtc002 = false;
jjtn002.setPath(t.image);
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, 0);
}
}
break;
case 26:
jj_consume_token(26);
t = jj_consume_token(PROPERTY_PATH);
ASTDbPath jjtn003 = new ASTDbPath(JJTDBPATH);
boolean jjtc003 = true;
jjtree.openNodeScope(jjtn003);
try {
jjtree.closeNodeScope(jjtn003, 0);
jjtc003 = false;
jjtn003.setPath(t.image);
} finally {
if (jjtc003) {
jjtree.closeNodeScope(jjtn003, 0);
}
}
break;
default:
jj_la1[22] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
public ExpressionParserTokenManager token_source;
JavaCharStream jj_input_stream;
public Token token, jj_nt;
private int jj_ntk;
private int jj_gen;
final private int[] jj_la1 = new int[23];
static private int[] jj_la1_0;
static private int[] jj_la1_1;
static {
jj_la1_0();
jj_la1_1();
}
private static void jj_la1_0() {
jj_la1_0 = new int[] {0x2,0x4,0x18,0x87310018,0x60,0x180,0x1010000,0x4fff8,0x4fff8,0x18,0x1010000,0x4e000,0x80000,0x87310000,0x1000000,0x300000,0x300000,0xc00000,0xc00000,0x100000,0x7310000,0x7010000,0x6000000,};
}
private static void jj_la1_1() {
jj_la1_1 = new int[] {0x0,0x0,0x0,0x3901,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x3901,0x3900,0x0,0x0,0x0,0x0,0x0,0x3001,0x3001,0x1,};
}
public ExpressionParser(java.io.InputStream stream) {
jj_input_stream = new JavaCharStream(stream, 1, 1);
token_source = new ExpressionParserTokenManager(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 23; i++) jj_la1[i] = -1;
}
public void ReInit(java.io.InputStream stream) {
jj_input_stream.ReInit(stream, 1, 1);
token_source.ReInit(jj_input_stream);
token = new Token();
jj_ntk = -1;
jjtree.reset();
jj_gen = 0;
for (int i = 0; i < 23; i++) jj_la1[i] = -1;
}
public ExpressionParser(java.io.Reader stream) {
jj_input_stream = new JavaCharStream(stream, 1, 1);
token_source = new ExpressionParserTokenManager(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 23; i++) jj_la1[i] = -1;
}
public void ReInit(java.io.Reader stream) {
jj_input_stream.ReInit(stream, 1, 1);
token_source.ReInit(jj_input_stream);
token = new Token();
jj_ntk = -1;
jjtree.reset();
jj_gen = 0;
for (int i = 0; i < 23; i++) jj_la1[i] = -1;
}
public ExpressionParser(ExpressionParserTokenManager tm) {
token_source = tm;
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 23; i++) jj_la1[i] = -1;
}
public void ReInit(ExpressionParserTokenManager tm) {
token_source = tm;
token = new Token();
jj_ntk = -1;
jjtree.reset();
jj_gen = 0;
for (int i = 0; i < 23; i++) jj_la1[i] = -1;
}
final private Token jj_consume_token(int kind) throws ParseException {
Token oldToken;
if ((oldToken = token).next != null) token = token.next;
else token = token.next = token_source.getNextToken();
jj_ntk = -1;
if (token.kind == kind) {
jj_gen++;
return token;
}
token = oldToken;
jj_kind = kind;
throw generateParseException();
}
final public Token getNextToken() {
if (token.next != null) token = token.next;
else token = token.next = token_source.getNextToken();
jj_ntk = -1;
jj_gen++;
return token;
}
final public Token getToken(int index) {
Token t = token;
for (int i = 0; i < index; i++) {
if (t.next != null) t = t.next;
else t = t.next = token_source.getNextToken();
}
return t;
}
final private int jj_ntk() {
if ((jj_nt=token.next) == null)
return (jj_ntk = (token.next=token_source.getNextToken()).kind);
else
return (jj_ntk = jj_nt.kind);
}
private java.util.Vector jj_expentries = new java.util.Vector();
private int[] jj_expentry;
private int jj_kind = -1;
public ParseException generateParseException() {
jj_expentries.removeAllElements();
boolean[] la1tokens = new boolean[50];
for (int i = 0; i < 50; i++) {
la1tokens[i] = false;
}
if (jj_kind >= 0) {
la1tokens[jj_kind] = true;
jj_kind = -1;
}
for (int i = 0; i < 23; i++) {
if (jj_la1[i] == jj_gen) {
for (int j = 0; j < 32; j++) {
if ((jj_la1_0[i] & (1<
© 2015 - 2024 Weber Informatics LLC | Privacy Policy