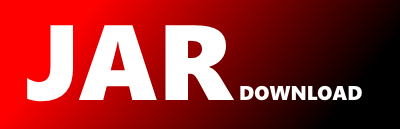
org.apache.cayenne.di.spi.DefaultInjector Maven / Gradle / Ivy
/*****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
****************************************************************/
package org.apache.cayenne.di.spi;
import java.util.HashMap;
import java.util.Map;
import org.apache.cayenne.ConfigurationException;
import org.apache.cayenne.di.Injector;
import org.apache.cayenne.di.Key;
import org.apache.cayenne.di.Module;
import org.apache.cayenne.di.Provider;
import org.apache.cayenne.di.Scope;
/**
* A default Cayenne implementations of a DI injector.
*
* @since 3.1
*/
public class DefaultInjector implements Injector {
private DefaultScope singletonScope;
private Scope noScope;
private Map, Binding>> bindings;
private InjectionStack injectionStack;
private Scope defaultScope;
public DefaultInjector(Module... modules) throws ConfigurationException {
this.singletonScope = new DefaultScope();
this.noScope = NoScope.INSTANCE;
// this is intentionally hardcoded and is not configurable
this.defaultScope = singletonScope;
this.bindings = new HashMap, Binding>>();
this.injectionStack = new InjectionStack();
DefaultBinder binder = new DefaultBinder(this);
// bind self for injector injection...
binder.bind(Injector.class).toInstance(this);
// bind modules
if (modules != null && modules.length > 0) {
for (Module module : modules) {
module.configure(binder);
}
}
}
InjectionStack getInjectionStack() {
return injectionStack;
}
Binding getBinding(Key key) throws ConfigurationException {
if (key == null) {
throw new NullPointerException("Null key");
}
// may return null - this is intentionally allowed in this non-public method
return (Binding) bindings.get(key);
}
void putBinding(Key bindingKey, Provider provider) {
// TODO: andrus 11/15/2009 - report overriding existing binding??
bindings.put(bindingKey, new Binding(provider, defaultScope));
}
void changeBindingScope(Key bindingKey, Scope scope) {
if (scope == null) {
scope = noScope;
}
Binding> binding = bindings.get(bindingKey);
if (binding == null) {
throw new ConfigurationException("No existing binding for key " + bindingKey);
}
binding.changeScope(scope);
}
public T getInstance(Class type) throws ConfigurationException {
return getProvider(type).get();
}
public T getInstance(Key key) throws ConfigurationException {
return getProvider(key).get();
}
public Provider getProvider(Class type) throws ConfigurationException {
return getProvider(Key.get(type));
}
public Provider getProvider(Key key) throws ConfigurationException {
if (key == null) {
throw new NullPointerException("Null key");
}
Binding binding = (Binding) bindings.get(key);
if (binding == null) {
throw new ConfigurationException(
"DI container has no binding for key %s",
key);
}
return binding.getScoped();
}
public void injectMembers(Object object) {
Provider
© 2015 - 2025 Weber Informatics LLC | Privacy Policy