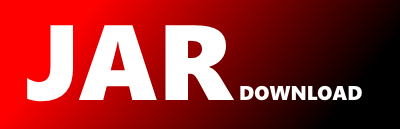
org.apache.cayenne.event.DispatchQueue Maven / Gradle / Ivy
/*****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
****************************************************************/
package org.apache.cayenne.event;
import java.util.Collection;
import java.util.Iterator;
import java.util.Map;
import java.util.WeakHashMap;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import org.apache.cayenne.event.DefaultEventManager.Dispatch;
import org.apache.cayenne.util.Invocation;
/**
* Stores a set of Invocation objects, organizing them by sender. Listeners have an option
* to receive events for a particular sender or to receive all events. EventManager
* creates one DispatchQueue per EventSubject. DispatchQueue is thread-safe - all methods
* that read/modify internal collections are synchronized.
*
* @since 1.1
*/
class DispatchQueue {
private final ConcurrentMap subjectInvocations;
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy