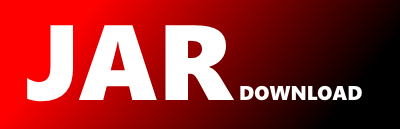
org.apache.cayenne.graph.GraphDiffCompressor Maven / Gradle / Ivy
/*****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
****************************************************************/
package org.apache.cayenne.graph;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* A utility class that removes redundant and mutually exclusive graph changes from the
* graph diff.
*
* @since 3.0
*/
public class GraphDiffCompressor {
public GraphDiff compress(GraphDiff diff) {
if (diff.isNoop()) {
return diff;
}
CompressAction action = new CompressAction();
diff.apply(action);
return action.getCompressedDiff();
}
final class CompressAction implements GraphChangeHandler {
private List compressed = new ArrayList();
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy