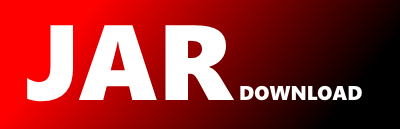
org.apache.cayenne.lifecycle.relationship.ObjectIdRelationshipBatchFaultingStrategy Maven / Gradle / Ivy
/*****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
****************************************************************/
package org.apache.cayenne.lifecycle.relationship;
import java.util.ArrayList;
import java.util.List;
import org.apache.cayenne.DataObject;
/**
* A faulting strategy that does batch-faulting of related objects whenever a first
* ObjectId relationship is accessed.
*
* @since 3.1
*/
public class ObjectIdRelationshipBatchFaultingStrategy implements
ObjectIdRelationshipFaultingStrategy {
private ThreadLocal> batchSources;
public ObjectIdRelationshipBatchFaultingStrategy() {
this.batchSources = new ThreadLocal>();
}
public void afterObjectLoaded(DataObject object) {
String uuidProperty = objectIdPropertyName(object);
String uuidRelationship = objectIdRelationshipName(uuidProperty);
String uuid = (String) object.readProperty(uuidProperty);
if (uuid == null) {
object.writePropertyDirectly(uuidRelationship, null);
}
else {
List sources = batchSources.get();
if (sources == null) {
sources = new ArrayList();
batchSources.set(sources);
}
sources.add(new ObjectIdBatchSourceItem(object, uuid, uuidRelationship));
}
}
public void afterQuery() {
List sources = batchSources.get();
if (sources != null) {
batchSources.set(null);
ObjectIdBatchFault batchFault = new ObjectIdBatchFault(sources
.get(0)
.getObject()
.getObjectContext(), sources);
for (ObjectIdBatchSourceItem source : sources) {
source.getObject().writePropertyDirectly(
source.getObjectIdRelationship(),
new ObjectIdFault(batchFault, source.getId()));
}
}
}
String objectIdRelationshipName(String uuidPropertyName) {
return "cay:related:" + uuidPropertyName;
}
String objectIdPropertyName(DataObject object) {
ObjectIdRelationship annotation = object.getClass().getAnnotation(
ObjectIdRelationship.class);
if (annotation == null) {
throw new IllegalArgumentException(
"Object class is not annotated with @UuidRelationship: "
+ object.getClass().getName());
}
// TODO: I guess we'll need to cache this metadata for performance if we are to
// support inheritance lookups, etc.
return annotation.value();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy