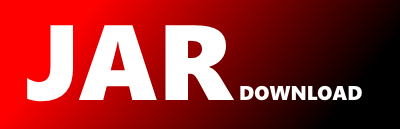
org.apache.commons.jexl3.JexlOperator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of commons-jexl3 Show documentation
Show all versions of commons-jexl3 Show documentation
The Apache Commons JEXL library is an implementation of the JSTL Expression Language with extensions.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.jexl3;
/**
* The JEXL operators.
*
* These are the operators that are executed by JexlArithmetic methods.
*
* Each of them associates a symbol to a method signature.
* For instance, '+' is associated to 'T add(L x, R y)'.
*
* The default JexlArithmetic implements generic versions of these methods using Object as arguments.
* You can use your own derived JexlArithmetic that override and/or overload those operator methods; these methods
* must be public,
* must respect the return type when primitive
* and may be overloaded multiple times with different signatures.
*
* @since 3.0
*/
public enum JexlOperator {
/**
* Add operator.
*
Syntax: x + y
*
Method: T add(L x, R y);
.
* @see JexlArithmetic#add
*/
ADD("+", "add", 2),
/**
* Subtract operator.
*
Syntax: x - y
*
Method: T subtract(L x, R y);
.
* @see JexlArithmetic#subtract
*/
SUBTRACT("-", "subtract", 2),
/**
* Multiply operator.
*
Syntax: x * y
*
Method: T multiply(L x, R y);
.
* @see JexlArithmetic#multiply
*/
MULTIPLY("*", "multiply", 2),
/**
* Divide operator.
*
Syntax: x / y
*
Method: T divide(L x, R y);
.
* @see JexlArithmetic#divide
*/
DIVIDE("/", "divide", 2),
/**
* Modulo operator.
*
Syntax: x % y
*
Method: T mod(L x, R y);
.
* @see JexlArithmetic#mod
*/
MOD("%", "mod", 2),
/**
* Bitwise-and operator.
*
Syntax: x & y
*
Method: T and(L x, R y);
.
* @see JexlArithmetic#and
*/
AND("&", "and", 2),
/**
* Bitwise-or operator.
*
Syntax: x | y
*
Method: T or(L x, R y);
.
* @see JexlArithmetic#or
*/
OR("|", "or", 2),
/**
* Bitwise-xor operator.
*
Syntax: x ^ y
*
Method: T xor(L x, R y);
.
* @see JexlArithmetic#xor
*/
XOR("^", "xor", 2),
/**
* Equals operator.
*
Syntax: x == y
*
Method: boolean equals(L x, R y);
.
* @see JexlArithmetic#equals
*/
EQ("==", "equals", 2),
/**
* Less-than operator.
*
Syntax: x < y
*
Method: boolean lessThan(L x, R y);
.
* @see JexlArithmetic#lessThan
*/
LT("<", "lessThan", 2),
/**
* Less-than-or-equal operator.
*
Syntax: x <= y
*
Method: boolean lessThanOrEqual(L x, R y);
.
* @see JexlArithmetic#lessThanOrEqual
*/
LTE("<=", "lessThanOrEqual", 2),
/**
* Greater-than operator.
*
Syntax: x > y
*
Method: boolean greaterThan(L x, R y);
.
* @see JexlArithmetic#greaterThan
*/
GT(">", "greaterThan", 2),
/**
* Greater-than-or-equal operator.
*
Syntax: x >= y
*
Method: boolean greaterThanOrEqual(L x, R y);
.
* @see JexlArithmetic#greaterThanOrEqual
*/
GTE(">=", "greaterThanOrEqual", 2),
/**
* Contains operator.
*
Syntax: x =~ y
*
Method: boolean contains(L x, R y);
.
* @see JexlArithmetic#contains
*/
CONTAINS("=~", "contains", 2),
/**
* Starts-with operator.
*
Syntax: x =^ y
*
Method: boolean startsWith(L x, R y);
.
* @see JexlArithmetic#startsWith
*/
STARTSWITH("=^", "startsWith", 2),
/**
* Ends-with operator.
*
Syntax: x =$ y
*
Method: boolean endsWith(L x, R y);
.
* @see JexlArithmetic#endsWith
*/
ENDSWITH("=$", "endsWith", 2),
/**
* Not operator.
*
Syntax: !x
*
Method: T not(L x);
.
* @see JexlArithmetic#not
*/
NOT("!", "not", 1),
/**
* Complement operator.
*
Syntax: ~x
*
Method: T complement(L x);
.
* @see JexlArithmetic#complement
*/
COMPLEMENT("~", "complement", 1),
/**
* Negate operator.
*
Syntax: -x
*
Method: T negate(L x);
.
* @see JexlArithmetic#negate
*/
NEGATE("-", "negate", 1),
/**
* Empty operator.
*
Syntax: empty x
or empty(x)
*
Method: boolean isEmpty(L x);
.
* @see JexlArithmetic#isEmpty
*/
EMPTY("empty", "empty", 1),
/**
* Size operator.
*
Syntax: size x
or size(x)
*
Method: int size(L x);
.
* @see JexlArithmetic#size
*/
SIZE("size", "size", 1),
/**
* Self-add operator.
*
Syntax: x += y
*
Method: T selfAdd(L x, R y);
.
*/
SELF_ADD("+=", "selfAdd", ADD),
/**
* Self-subtract operator.
*
Syntax: x -= y
*
Method: T selfSubtract(L x, R y);
.
*/
SELF_SUBTRACT("-=", "selfSubtract", SUBTRACT),
/**
* Self-multiply operator.
*
Syntax: x *= y
*
Method: T selfMultiply(L x, R y);
.
*/
SELF_MULTIPLY("*=", "selfMultiply", MULTIPLY),
/**
* Self-divide operator.
*
Syntax: x /= y
*
Method: T selfDivide(L x, R y);
.
*/
SELF_DIVIDE("/=", "selfDivide", DIVIDE),
/**
* Self-modulo operator.
*
Syntax: x %= y
*
Method: T selfMod(L x, R y);
.
*/
SELF_MOD("%=", "selfMod", MOD),
/**
* Self-and operator.
*
Syntax: x &= y
*
Method: T selfAnd(L x, R y);
.
*/
SELF_AND("&=", "selfAnd", AND),
/**
* Self-or operator.
*
Syntax: x |= y
*
Method: T selfOr(L x, R y);
.
*/
SELF_OR("|=", "selfOr", OR),
/**
* Self-xor operator.
*
Syntax: x ^= y
*
Method: T selfXor(L x, R y);
.
*/
SELF_XOR("^=", "selfXor", XOR),
/**
* Marker for side effect.
*
Returns this from 'self*' overload method to let the engine know the side effect has been performed and
* there is no need to assign the result.
*/
ASSIGN("=", null, null),
/**
* Property get operator as in: x.y.
*
Syntax: x.y
*
Method: Object propertyGet(L x, R y);
.
*/
PROPERTY_GET(".", "propertyGet", 2),
/**
* Property set operator as in: x.y = z.
*
Syntax: x.y = z
*
Method: void propertySet(L x, R y, V z);
.
*/
PROPERTY_SET(".=", "propertySet", 3),
/**
* Array get operator as in: x[y].
*
Syntax: x.y
*
Method: Object arrayGet(L x, R y);
.
*/
ARRAY_GET("[]", "arrayGet", 2),
/**
* Array set operator as in: x[y] = z.
*
Syntax: x[y] = z
*
Method: void arraySet(L x, R y, V z);
.
*/
ARRAY_SET("[]=", "arraySet", 3),
/**
* Iterator generator as in for(var x : y).
* If the returned Iterator is AutoCloseable, close will be called after the last execution of the loop block.
*
Syntax: for(var x : y){...}
*
Method: Iterator<Object> forEach(R y);
.
* @since 3.1
*/
FOR_EACH("for(...)", "forEach", 1);
/**
* The operator symbol.
*/
private final String operator;
/**
* The associated operator method name.
*/
private final String methodName;
/**
* The method arity (ie number of arguments).
*/
private final int arity;
/**
* The base operator.
*/
private final JexlOperator base;
/**
* Creates a base operator.
*
* @param o the operator name
* @param m the method name associated to this operator in a JexlArithmetic
* @param argc the number of parameters for the method
*/
JexlOperator(String o, String m, int argc) {
this.operator = o;
this.methodName = m;
this.arity = argc;
this.base = null;
}
/**
* Creates a side-effect operator.
*
* @param o the operator name
* @param m the method name associated to this operator in a JexlArithmetic
* @param b the base operator, ie + for +=
*/
JexlOperator(String o, String m, JexlOperator b) {
this.operator = o;
this.methodName = m;
this.arity = 2;
this.base = b;
}
/**
* Gets this operator symbol.
*
* @return the symbol
*/
public final String getOperatorSymbol() {
return operator;
}
/**
* Gets this operator method name in a JexlArithmetic.
*
* @return the method name
*/
public final String getMethodName() {
return methodName;
}
/**
* Gets this operator number of parameters.
*
* @return the method arity
*/
public int getArity() {
return arity;
}
/**
* Gets the base operator.
*
* @return the base operator
*/
public final JexlOperator getBaseOperator() {
return base;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy