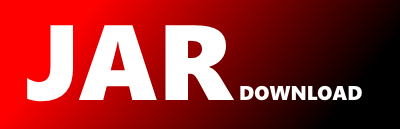
org.apache.commons.jexl3.parser.Parser.jjt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of commons-jexl3 Show documentation
Show all versions of commons-jexl3 Show documentation
The Apache Commons JEXL library is an implementation of the JSTL Expression Language with extensions.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
options
{
MULTI=true;
STATIC=false;
VISITOR=true;
NODE_SCOPE_HOOK=true;
NODE_CLASS="JexlNode";
UNICODE_INPUT=true;
KEEP_LINE_COLUMN=true;
TRACK_TOKENS=true;
//DEBUG_PARSER=true;
//DEBUG_TOKEN_MANAGER=true;
}
PARSER_BEGIN(Parser)
package org.apache.commons.jexl3.parser;
import java.util.Collections;
import java.util.LinkedList;
import org.apache.commons.jexl3.JexlInfo;
import org.apache.commons.jexl3.JexlException;
import org.apache.commons.jexl3.internal.Scope;
public final class Parser extends JexlParser
{
private int loopCount = 0;
public ASTJexlScript parse(JexlInfo info, String jexlSrc, Scope scope, boolean registers, boolean expr) {
try {
// If registers are allowed, the default parser state has to be REGISTERS.
if (registers || ALLOW_REGISTERS) {
token_source.defaultLexState = REGISTERS;
}
// lets do the 'Unique Init' in here to be safe - it's a pain to remember
source = jexlSrc;
pragmas = null;
ReInit(new java.io.StringReader(jexlSrc));
frame = scope;
ASTJexlScript script = expr? JexlExpression(scope) : JexlScript(scope) ;
script.pragmas = pragmas != null
? Collections.unmodifiableMap(pragmas)
: Collections.emptyMap();
pragmas = null;
script.jjtSetValue(info);
return script;
} catch (TokenMgrError xtme) {
throw new JexlException.Tokenization(info, xtme).clean();
} catch (ParseException xparse) {
throw new JexlException.Parsing(info, xparse).clean();
} finally {
source = null;
frame = null;
token_source.defaultLexState = DEFAULT;
}
}
}
PARSER_END(Parser)
TOKEN_MGR_DECLS : {
/**
* A stack of 1 for keeping state to deal with doted identifiers
*/
int dotLexState = DEFAULT;
public void pushDot() {
dotLexState = curLexState;
curLexState = DOT_ID;
}
public void popDot() {
if (curLexState == DOT_ID) {
curLexState = dotLexState;
dotLexState = defaultLexState;
}
}
}
/***************************************
* Skip & Number literal tokens
***************************************/
<*> SKIP : /* WHITE SPACE */
{
<"##" (~["\n","\r"])* ("\n" | "\r" | "\r\n")? >
| <"/*" (~["*"])* "*" ("*" | ~["*","/"] (~["*"])* "*")* "/">
| <"//" (~["\n","\r"])* ("\n" | "\r" | "\r\n")? >
| " "
| "\t"
| "\n"
| "\r"
| "\f"
}
<*> TOKEN : /* KEYWORDS */
{
< IF : "if" >
| < ELSE : "else" >
| < FOR : "for" >
| < WHILE : "while" >
| < NEW : "new" >
| < VAR : "var" >
| < EMPTY : "empty" > { popDot(); } /* Revert state to default if was DOT_ID. */
| < SIZE : "size" > { popDot(); } /* Revert state to default if was DOT_ID. */
| < NULL : "null" >
| < TRUE : "true" >
| < FALSE : "false" >
| < RETURN : "return" >
| < FUNCTION : "function" >
| < LAMBDA : "->" >
| < BREAK : "break" >
| < CONTINUE : "continue" >
| < PRAGMA : "#pragma" >
}
<*> TOKEN : { /* SEPARATORS */
< LPAREN : "(" >
| < RPAREN : ")" >
| < LCURLY : "{" >
| < RCURLY : "}" >
| < LBRACKET : "[" >
| < RBRACKET : "]" >
| < SEMICOL : ";" >
| < COLON : ":" >
| < COMMA : "," >
| < DOT : "." > { pushDot(); } /* Lexical state is now DOT_ID */
| < ELIPSIS : "..." >
}
<*> TOKEN : { /* CONDITIONALS */
< QMARK : "?" >
| < ELVIS : "?:" >
| < AND : "&&" | "and" >
| < OR : "||" | "or" >
}
<*> TOKEN : { /* COMPARISONS */
< eq : "==" | "eq" >
| < ne : "!=" | "ne" >
| < req : "=~" > // regexp equal
| < rne : "!~" > // regexp not equal
| < seq : "=^" > // starts equal
| < eeq : "=$" > // ends equal
| < sne : "!^" > // start not equal
| < ene : "!$" > // ends not equal
| < gt : ">" | "gt" >
| < ge : ">=" | "ge" >
| < lt : "<" | "lt" >
| < le : "<=" | "le" >
}
<*> TOKEN : { /* OPERATORS */
< plus_assign : "+=" >
| < minus_assign : "-=" >
| < mult_assign : "*=" >
| < div_assign : "/=" >
| < mod_assign : "%=" >
| < and_assign : "&=" >
| < or_assign : "|=" >
| < xor_assign : "^=" >
| < assign : "=" >
| < plus : "+" >
| < minus : "-" >
| < mult : "*" >
| < div : "/" | "div" >
| < mod : "%" | "mod" >
| < not : "!" | "not" >
| < and : "&" >
| < or : "|" >
| < xor : "^" >
| < tilda : "~" >
| < range : ".." >
}
/***************************************
* Identifier & String tokens
***************************************/
<*> TOKEN : /* NaN */
{
< NAN_LITERAL : "NaN" >
}
<*> TOKEN : /* ANNOTATION */
{
< ANNOTATION: "@" ( [ "0"-"9", "a"-"z", "A"-"Z", "_", "$" ])+ >
}
TOKEN : /* IDENTIFIERS */
{
< DOT_IDENTIFIER: ( [ "0"-"9", "a"-"z", "A"-"Z", "_", "$", "@" ])+ > { popDot(); } /* Revert state to default. */
}
TOKEN : /* IDENTIFIERS */
{
< IDENTIFIER: (|)* >
|
< #LETTER: [ "a"-"z", "A"-"Z", "_", "$", "@" ] >
|
< #DIGIT: [ "0"-"9"] >
}
TOKEN : /* REGISTERS: parser.ALLOW_REGISTER must be set to true before calling parse */
{
< REGISTER: "#" (["0"-"9"])+ >
}
TOKEN : /* LITERALS */
{
< INTEGER_LITERAL:
( "0" (["0"-"7"])* | ["1"-"9"] (["0"-"9"])* | "0" ["x","X"] (["0"-"9","a"-"f","A"-"F"])+ )
(["l","L","h","H"])?
>
|
< FLOAT_LITERAL:
"#NaN"
|
()+ "." ()+ ((["e","E"])(["+","-"])?()+)? (["d","D","f","F","b","B"])?
|
()+ (".")? ((["e","E"])(["+","-"])?()+)? ["d","D","f","F","b","B"]
>
}
<*> TOKEN :
{
< STRING_LITERAL:
"\"" (~["\"","\\","\n","\r","\u2028","\u2029"] | "\\" ~["\n","\r","\u2028","\u2029"])* "\""
|
"'" (~["'","\\","\n","\r","\u2028","\u2029"] | "\\" ~["\n","\r","\u2028","\u2029"])* "'"
> { popDot(); } /* Revert state to default if was DOT_ID. */
}
<*> TOKEN :
{
< JXLT_LITERAL:
"`" (~["`","\\"] | "\\" ~["\u0000"])* "`"
>
}
/***************************************
* Statements
***************************************/
ASTJexlScript JexlScript(Scope frame) : {
jjtThis.setScope(frame);
}
{
( ( Statement() )*)
{
return jjtThis.script();
}
}
ASTJexlScript JexlExpression(Scope frame) #JexlScript : {
jjtThis.setScope(frame);
}
{
( Expression() )?
{
return jjtThis.script();
}
}
void Annotation() #Annotation :
{
Token t;
}
{
t= (LOOKAHEAD() Arguments() )? { jjtThis.setName(t.image); }
}
void AnnotatedStatement() #AnnotatedStatement() : {}
{
(LOOKAHEAD() Annotation())+ (LOOKAHEAD() Var() | LOOKAHEAD(1) Block() | Expression())
}
void Statement() #void : {}
{
| LOOKAHEAD() AnnotatedStatement()
| LOOKAHEAD( Expression() ) Block() // to disambiguate the set literals
| LOOKAHEAD( Statement() ) Block() // to disambiguate the set literals
| IfStatement()
| ForeachStatement()
| WhileStatement()
| ExpressionStatement()
| ReturnStatement()
| Continue()
| Break()
| Var()
| Pragma()
}
void Block() #Block : {}
{
( Statement() )*
}
void ExpressionStatement() #void : {}
{
Expression() (LOOKAHEAD(1) Expression() #Ambiguous())* (LOOKAHEAD(2) )?
}
void IfStatement() : {}
{
Expression() (LOOKAHEAD(1) Block() | Statement()) ( LOOKAHEAD(1) (LOOKAHEAD(1) Block() | Statement()) )?
}
void WhileStatement() : {}
{
Expression() { loopCount += 1; } (LOOKAHEAD(1) Block() | Statement()) { loopCount -= 1; }
}
void ReturnStatement() : {}
{
Expression()
}
void Continue() #Continue : {}
{
{ if (loopCount == 0) { throwParsingException(jjtThis); } }
}
void Break() #Break : {}
{
{ if (loopCount == 0) { throwParsingException(jjtThis); } }
}
void ForeachStatement() : {}
{
ForEachVar() Expression() { loopCount += 1; } (LOOKAHEAD(1) Block() | Statement()) { loopCount -= 1; }
}
void ForEachVar() #Reference : {}
{
DeclareVar()
|
Identifier()
}
void Var() #void : {}
{
DeclareVar() (LOOKAHEAD(1) Expression() #Assignment(2))?
}
void DeclareVar() #Var :
{
Token t;
}
{
t= { declareVariable(jjtThis, t.image); }
}
void Pragma() #void :
{
LinkedList lstr = new LinkedList();
Object value;
}
{
pragmaKey(lstr) value=pragmaValue() { declarePragma(stringify(lstr), value); }
}
void pragmaKey(LinkedList lstr) #void :
{
Token t;
}
{
t= (LOOKAHEAD(2) pragmaKey(lstr) )* { lstr.addFirst(t.image); }
}
Object pragmaValue() #void :
{
Token v;
LinkedList lstr = new LinkedList();
}
{
LOOKAHEAD(1) v= { return NumberParser.parseInteger(v.image); }
| LOOKAHEAD(1) v= { return NumberParser.parseDouble(v.image); }
| LOOKAHEAD(1) v= { return Parser.buildString(v.image, true); }
| LOOKAHEAD(1) pragmaKey(lstr) { return stringify(lstr); }
| LOOKAHEAD(1) { return true; }
| LOOKAHEAD(1) { return false; }
| LOOKAHEAD(1) { return null; }
| LOOKAHEAD(1) { return Double.NaN; }
}
/***************************************
* Expression syntax
***************************************/
void Expression() #void : {}
{
AssignmentExpression()
}
void AssignmentExpression() #void : {}
{
ConditionalExpression()
( LOOKAHEAD(2) (
Expression() #SetAddNode(2)
|
Expression() #SetMultNode(2)
|
Expression() #SetDivNode(2)
|
Expression() #SetModNode(2)
|
Expression() #SetAndNode(2)
|
Expression() #SetOrNode(2)
|
Expression() #SetXorNode(2)
|
Expression() #SetSubNode(2)
|
Expression() #Assignment(2)
) )*
}
/***************************************
* Conditional & relational
***************************************/
void ConditionalExpression() #void : {}
{
ConditionalOrExpression()
(
Expression() Expression() #TernaryNode(3)
|
Expression() #TernaryNode(2)
)?
}
void ConditionalOrExpression() #void : {}
{
ConditionalAndExpression()
( ConditionalAndExpression() #OrNode(2) )*
}
void ConditionalAndExpression() #void : {}
{
InclusiveOrExpression()
( InclusiveOrExpression() #AndNode(2) )*
}
void InclusiveOrExpression() #void : {}
{
ExclusiveOrExpression()
( ExclusiveOrExpression() #BitwiseOrNode(2) )*
}
void ExclusiveOrExpression() #void : {}
{
AndExpression()
( AndExpression() #BitwiseXorNode(2) )*
}
void AndExpression() #void : {}
{
EqualityExpression()
( EqualityExpression() #BitwiseAndNode(2) )*
}
void EqualityExpression() #void : {}
{
RelationalExpression()
(
RelationalExpression() #EQNode(2)
|
RelationalExpression() #NENode(2)
|
RelationalExpression() #RangeNode(2) // range
)?
}
void RelationalExpression() #void : {}
{
AdditiveExpression()
(
AdditiveExpression() #LTNode(2)
|
AdditiveExpression() #GTNode(2)
|
AdditiveExpression() #LENode(2)
|
AdditiveExpression() #GENode(2)
|
AdditiveExpression() #ERNode(2) // equals regexp
|
AdditiveExpression() #NRNode(2) // not equals regexp
|
AdditiveExpression() #SWNode(2) // starts with
|
AdditiveExpression() #NSWNode(2) // not starts with
|
AdditiveExpression() #EWNode(2) // ends with
|
AdditiveExpression() #NEWNode(2) // not ends with
)?
}
/***************************************
* Arithmetic
***************************************/
void AdditiveExpression() #void : {}
{
MultiplicativeExpression()
( LOOKAHEAD(2) (
MultiplicativeExpression() #AddNode(2)
|
MultiplicativeExpression() #SubNode(2)
) )*
}
void MultiplicativeExpression() #void : {}
{
UnaryExpression()
(
UnaryExpression() #MulNode(2)
|
UnaryExpression() #DivNode(2)
|
UnaryExpression() #ModNode(2)
)*
}
void UnaryExpression() #void : {}
{
UnaryExpression() #UnaryMinusNode(1)
|
UnaryExpression() #BitwiseComplNode(1)
|
UnaryExpression() #NotNode(1)
|
UnaryExpression() #EmptyFunction(1)
|
UnaryExpression() #SizeFunction(1)
|
ValueExpression()
}
/***************************************
* Identifier & Literals
***************************************/
void Identifier(boolean top) :
{
Token t;
}
{
t= { jjtThis.setSymbol(top? checkVariable(jjtThis, t.image) : t.image); }
|
t= { jjtThis.setSymbol(t.image); }
}
void StringIdentifier() #Identifier :
{
Token t;
}
{
t=
{
jjtThis.setSymbol(Parser.buildString(t.image, true));
}
}
void Literal() #void :
{
Token t;
}
{
IntegerLiteral()
|
FloatLiteral()
|
BooleanLiteral()
|
JxltLiteral()
|
StringLiteral()
|
NullLiteral()
|
NaNLiteral()
}
void NaNLiteral() #NumberLiteral :
{}
{
{ jjtThis.setReal("NaN"); }
}
void NullLiteral() : {}
{
}
void BooleanLiteral() #void :
{}
{
#TrueNode
|
#FalseNode
}
void IntegerLiteral() #NumberLiteral :
{
Token t;
}
{
t=
{ jjtThis.setNatural(t.image); }
}
void FloatLiteral() #NumberLiteral:
{
Token t;
}
{
t=
{ jjtThis.setReal(t.image); }
}
void StringLiteral() :
{
Token t;
}
{
t=
{ jjtThis.setLiteral(Parser.buildString(t.image, true)); }
}
void JxltLiteral() #JxltLiteral :
{
Token t;
}
{
t=
{ jjtThis.setLiteral(Parser.buildString(t.image, true)); }
}
void ExtendedLiteral() #ExtendedLiteral() : {}
{
}
void ArrayLiteral() : {}
{
(
LOOKAHEAD(1) ExtendedLiteral()
|
(Expression() (LOOKAHEAD(2) Expression() )*)? ( ExtendedLiteral())?
)
}
void MapLiteral() : {}
{
(
MapEntry() ( MapEntry() )*
|
)
}
void MapEntry() : {}
{
Expression() Expression()
}
void SetLiteral() : {}
{
(Expression() ( Expression() )*)?
}
/***************************************
* Functions & Methods
***************************************/
void EmptyMethod() #EmptyMethod() : {}
{
}
void SizeMethod() #SizeMethod() : {}
{
}
void Arguments() #Arguments : {}
{
(Expression() ( Expression())* )?
}
void FunctionCallLookahead() #void : {}
{
LOOKAHEAD(4)
|
LOOKAHEAD(2)
|
LOOKAHEAD(2)
}
void FunctionCall() #void : {}
{
LOOKAHEAD(2) Identifier() Identifier() Arguments() #FunctionNode(3)
|
LOOKAHEAD(2) Identifier(true) Arguments() #FunctionNode(2)
}
void Constructor() #ConstructorNode() : {}
{
[ Expression() ( Expression() )* ]
}
void Parameter() #void :
{
Token t;
}
{
t= { declareParameter(t.image); }
}
void Parameters() #void : {}
{
[Parameter() ( Parameter())* ]
}
void LambdaLookahead() #void() : {}
{
Parameters()
|
Parameters()
|
Parameter()
}
void Lambda() #JexlLambda() :
{
pushFrame();
}
{
Parameters() Block()
|
Parameters() Block()
|
Parameter() Block()
}
/***************************************
* References
***************************************/
void IdentifierAccess() :
{
Token t;
}
{
(
t= { jjtThis.setIdentifier(t.image); }
|
t= { jjtThis.setIdentifier(Parser.buildString(t.image, true)); }
)
}
void ArrayAccess() : {}
{
(LOOKAHEAD(1) Expression() )+
}
void MemberAccess() #void : {}
{
LOOKAHEAD() ArrayAccess()
|
LOOKAHEAD() IdentifierAccess()
}
void ReferenceExpression() #MethodNode(>1) : {}
{
( Expression() #ReferenceExpression(1) ) ( LOOKAHEAD() Arguments() )*
}
void PrimaryExpression() #void : {}
{
LOOKAHEAD( LambdaLookahead() ) Lambda()
|
LOOKAHEAD( ) ReferenceExpression()
|
LOOKAHEAD( Expression() ) MapLiteral()
|
LOOKAHEAD( ) MapLiteral()
|
LOOKAHEAD( Expression() ) SetLiteral()
|
LOOKAHEAD( ) SetLiteral()
|
LOOKAHEAD( ) ArrayLiteral()
|
LOOKAHEAD( ) Constructor()
|
LOOKAHEAD( FunctionCallLookahead() ) FunctionCall()
|
Identifier(true)
|
Literal()
}
void MethodCall() #void : {}
{
LOOKAHEAD( ) ( ) #SizeMethod(1)
|
LOOKAHEAD( ) ( ) #EmptyMethod(1)
|
(MemberAccess() (LOOKAHEAD() Arguments())+) #MethodNode(>1)
}
void MemberExpression() #void : {}
{
LOOKAHEAD(MethodCall()) MethodCall() | MemberAccess()
}
void ValueExpression() #void : {}
{
( PrimaryExpression() ( LOOKAHEAD(2) MemberExpression() )*) #Reference(>1)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy