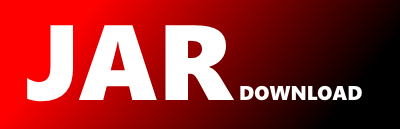
org.apache.commons.lang3.function.Failable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of commons-lang3 Show documentation
Show all versions of commons-lang3 Show documentation
Apache Commons Lang, a package of Java utility classes for the
classes that are in java.lang's hierarchy, or are considered to be so
standard as to justify existence in java.lang.
The code is tested using the latest revision of the JDK for supported
LTS releases: 8, 11, 17 and 21 currently.
See https://github.com/apache/commons-lang/blob/master/.github/workflows/maven.yml
Please ensure your build environment is up-to-date and kindly report any build issues.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy