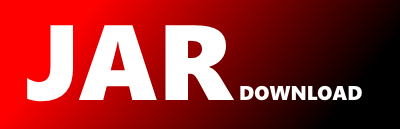
org.apache.cxf.event.EventCache Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.cxf.event;
import java.util.List;
import javax.xml.namespace.QName;
/**
* Caches all events that do not have a listener associated with them.
* The events will be stored until the cache limit is reached.
* After reaching the cache size, events will be discarded using first in,
* first out semantics.
*/
public interface EventCache {
/**
* Add the Event
to the cache.
* If the maximum size of the cache is reached, the first Event
* added will be removed from the cache(FIFO)
* @param e The Event
to be added to the cache.
*/
void addEvent(Event e);
/**
* Flushes the cache of all the Event
s.
* @return List Containing the cached Event
s.
*/
List flushEvents();
/**
* Flushes the Event
from the cache matching the event type.
* @param eventType the Event
type.
* @return List the list of Event
s matching the event type.
*/
List flushEvents(QName eventType);
/**
* Flushes the Event
s from the cache matching the event type namespace.
* @param namespaceURI the Event
type namespace.
* @return List the list of Event
s matching the event type namespace.
*/
List flushEvents(String namespaceURI);
/**
* Returns all the events. This method doesn't remove the
* events from the cache.
* @return List the list of all events stored in the cache.
*/
List getEvents();
/**
* Returns all the events matching the event type. This method doesn't
* remove the events from the cache.
* @param eventType the Event
type.
* @return the list of Event
s matching the event type.
*/
List getEvents(QName eventType);
/**
* Returns all the events matching the event type namespace. This method doesn't
* remove the events from the cache.
* @param namespaceURI the Event
type namespace.
* @return the list of Event
s matching the event type namespace.
*/
List getEvents(String namespaceURI);
/**
* Sets the cache size. This method can be used to dynamically change the
* cache size from the configured size.
* @param size Indicates the new size of the cache.
*/
void setCacheSize(int size);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy