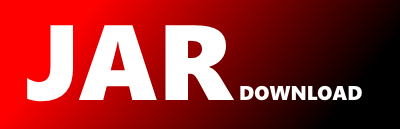
datafu.hourglass.avro.AvroKeyValueWithMetadataRecordWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datafu-hourglass-incubating Show documentation
Show all versions of datafu-hourglass-incubating Show documentation
Librares that make easier to solve data problems using Hadoop and higher level languages based on it.
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package datafu.hourglass.avro;
import java.io.IOException;
import java.io.OutputStream;
import java.util.Map.Entry;
import org.apache.avro.Schema;
import org.apache.avro.hadoop.io.AvroKeyValue;
import org.apache.avro.hadoop.io.AvroDatumConverter;
import org.apache.avro.file.CodecFactory;
import org.apache.avro.file.DataFileWriter;
import org.apache.avro.generic.GenericData;
import org.apache.avro.generic.GenericRecord;
import org.apache.avro.reflect.ReflectDatumWriter;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.mapreduce.RecordWriter;
import org.apache.hadoop.mapreduce.TaskAttemptContext;
/**
* Writes key/value pairs to an Avro container file.
*
* Each entry in the Avro container file will be a generic record with two fields,
* named 'key' and 'value'. The input types may be basic Writable objects like Text or
* IntWritable, or they may be AvroWrapper subclasses (AvroKey or AvroValue). Writable
* objects will be converted to their corresponding Avro types when written to the generic
* record key/value pair.
*
* @param The type of key to write.
* @param The type of value to write.
*/
public class AvroKeyValueWithMetadataRecordWriter extends RecordWriter {
/** A writer for the Avro container file. */
private final DataFileWriter mAvroFileWriter;
/** The writer schema for the generic record entries of the Avro container file. */
private final Schema mKeyValuePairSchema;
/** A reusable Avro generic record for writing key/value pairs to the file. */
private final AvroKeyValue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy