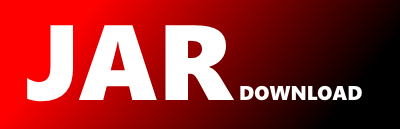
org.apache.dolphinscheduler.api.controller.UsersController Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.dolphinscheduler.api.controller;
import org.apache.dolphinscheduler.api.enums.Status;
import org.apache.dolphinscheduler.api.service.UsersService;
import org.apache.dolphinscheduler.api.utils.Result;
import org.apache.dolphinscheduler.common.Constants;
import org.apache.dolphinscheduler.common.utils.ParameterUtils;
import org.apache.dolphinscheduler.dao.entity.User;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiImplicitParam;
import io.swagger.annotations.ApiImplicitParams;
import io.swagger.annotations.ApiOperation;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.*;
import springfox.documentation.annotations.ApiIgnore;
import java.util.Map;
/**
* user controller
*/
@Api(tags = "USERS_TAG" , position = 14)
@RestController
@RequestMapping("/users")
public class UsersController extends BaseController{
private static final Logger logger = LoggerFactory.getLogger(UsersController.class);
@Autowired
private UsersService usersService;
/**
* create user
*
* @param loginUser login user
* @param userName user name
* @param userPassword user password
* @param email email
* @param tenantId tenant id
* @param phone phone
* @param queue queue
* @return create result code
*/
@ApiOperation(value = "createUser", notes= "CREATE_USER_NOTES")
@ApiImplicitParams({
@ApiImplicitParam(name = "userName", value = "USER_NAME",type = "String"),
@ApiImplicitParam(name = "userPassword", value = "USER_PASSWORD", type ="String"),
@ApiImplicitParam(name = "tenantId", value = "TENANT_ID", dataType = "Int", example = "100"),
@ApiImplicitParam(name = "queue", value = "QUEUE", dataType = "Int", example = "100"),
@ApiImplicitParam(name = "email", value = "EMAIL", dataType = "Int", example = "100"),
@ApiImplicitParam(name = "phone", value = "PHONE", dataType = "Int", example = "100")
})
@PostMapping(value = "/create")
@ResponseStatus(HttpStatus.CREATED)
public Result createUser(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser,
@RequestParam(value = "userName") String userName,
@RequestParam(value = "userPassword") String userPassword,
@RequestParam(value = "tenantId") int tenantId,
@RequestParam(value = "queue",required = false,defaultValue = "") String queue,
@RequestParam(value = "email") String email,
@RequestParam(value = "phone", required = false) String phone) {
logger.info("login user {}, create user, userName: {}, email: {}, tenantId: {}, userPassword: {}, phone: {}, user queue: {}",
loginUser.getUserName(), userName, email, tenantId, Constants.PASSWORD_DEFAULT, phone,queue);
try {
Map result = usersService.createUser(loginUser, userName, userPassword,email,tenantId, phone,queue);
return returnDataList(result);
}catch (Exception e){
logger.error(Status.CREATE_USER_ERROR.getMsg(),e);
return error(Status.CREATE_USER_ERROR.getCode(), Status.CREATE_USER_ERROR.getMsg());
}
}
/**
* query user list paging
*
* @param loginUser login user
* @param pageNo page number
* @param searchVal search avlue
* @param pageSize page size
* @return user list page
*/
@ApiOperation(value = "queryUserList", notes= "QUERY_USER_LIST_NOTES")
@ApiImplicitParams({
@ApiImplicitParam(name = "pageNo", value = "PAGE_NO",dataType = "Int", example = "100"),
@ApiImplicitParam(name = "pageSize", value = "PAGE_SIZE", type ="String"),
@ApiImplicitParam(name = "searchVal", value = "SEARCH_VAL", type ="String")
})
@GetMapping(value="/list-paging")
@ResponseStatus(HttpStatus.OK)
public Result queryUserList(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser,
@RequestParam("pageNo") Integer pageNo,
@RequestParam(value = "searchVal", required = false) String searchVal,
@RequestParam("pageSize") Integer pageSize){
logger.info("login user {}, list user paging, pageNo: {}, searchVal: {}, pageSize: {}",
loginUser.getUserName(),pageNo,searchVal,pageSize);
try{
Map result = checkPageParams(pageNo, pageSize);
if(result.get(Constants.STATUS) != Status.SUCCESS){
return returnDataListPaging(result);
}
searchVal = ParameterUtils.handleEscapes(searchVal);
result = usersService.queryUserList(loginUser, searchVal, pageNo, pageSize);
return returnDataListPaging(result);
}catch (Exception e){
logger.error(Status.QUERY_USER_LIST_PAGING_ERROR.getMsg(),e);
return error(Status.QUERY_USER_LIST_PAGING_ERROR.getCode(), Status.QUERY_USER_LIST_PAGING_ERROR.getMsg());
}
}
/**
* update user
*
* @param loginUser login user
* @param id user id
* @param userName user name
* @param userPassword user password
* @param email email
* @param tenantId tennat id
* @param phone phone
* @param queue queue
* @return update result code
*/
@ApiOperation(value = "updateUser", notes= "UPDATE_USER_NOTES")
@ApiImplicitParams({
@ApiImplicitParam(name = "id", value = "USER_ID",dataType = "Int", example = "100"),
@ApiImplicitParam(name = "userName", value = "USER_NAME",type = "String"),
@ApiImplicitParam(name = "userPassword", value = "USER_PASSWORD", type ="String"),
@ApiImplicitParam(name = "tenantId", value = "TENANT_ID", dataType = "Int", example = "100"),
@ApiImplicitParam(name = "queue", value = "QUEUE", dataType = "Int", example = "100"),
@ApiImplicitParam(name = "email", value = "EMAIL", dataType = "Int", example = "100"),
@ApiImplicitParam(name = "phone", value = "PHONE", dataType = "Int", example = "100")
})
@PostMapping(value = "/update")
@ResponseStatus(HttpStatus.OK)
public Result updateUser(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser,
@RequestParam(value = "id") int id,
@RequestParam(value = "userName") String userName,
@RequestParam(value = "userPassword") String userPassword,
@RequestParam(value = "queue",required = false,defaultValue = "") String queue,
@RequestParam(value = "email") String email,
@RequestParam(value = "tenantId") int tenantId,
@RequestParam(value = "phone", required = false) String phone) {
logger.info("login user {}, updateProcessInstance user, userName: {}, email: {}, tenantId: {}, userPassword: {}, phone: {}, user queue: {}",
loginUser.getUserName(), userName, email, tenantId, Constants.PASSWORD_DEFAULT, phone,queue);
try {
Map result = usersService.updateUser(id, userName, userPassword, email, tenantId, phone, queue);
return returnDataList(result);
}catch (Exception e){
logger.error(Status.UPDATE_USER_ERROR.getMsg(),e);
return error(Status.UPDATE_USER_ERROR.getCode(), Status.UPDATE_USER_ERROR.getMsg());
}
}
/**
* delete user by id
* @param loginUser login user
* @param id user id
* @return delete result code
*/
@ApiOperation(value = "delUserById", notes= "DELETE_USER_BY_ID_NOTES")
@ApiImplicitParams({
@ApiImplicitParam(name = "id", value = "USER_ID",dataType = "Int", example = "100")
})
@PostMapping(value = "/delete")
@ResponseStatus(HttpStatus.OK)
public Result delUserById(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser,
@RequestParam(value = "id") int id) {
logger.info("login user {}, delete user, userId: {},", loginUser.getUserName(), id);
try {
Map result = usersService.deleteUserById(loginUser, id);
return returnDataList(result);
}catch (Exception e){
logger.error(Status.DELETE_USER_BY_ID_ERROR.getMsg(),e);
return error(Status.DELETE_USER_BY_ID_ERROR.getCode(), Status.DELETE_USER_BY_ID_ERROR.getMsg());
}
}
/**
* grant project
*
* @param loginUser login user
* @param userId user id
* @param projectIds project id array
* @return grant result code
*/
@ApiOperation(value = "grantProject", notes= "GRANT_PROJECT_NOTES")
@ApiImplicitParams({
@ApiImplicitParam(name = "userId", value = "USER_ID",dataType = "Int", example = "100"),
@ApiImplicitParam(name = "projectIds", value = "PROJECT_IDS",type = "String")
})
@PostMapping(value = "/grant-project")
@ResponseStatus(HttpStatus.OK)
public Result grantProject(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser,
@RequestParam(value = "userId") int userId,
@RequestParam(value = "projectIds") String projectIds) {
logger.info("login user {}, grant project, userId: {},projectIds : {}", loginUser.getUserName(), userId,projectIds);
try {
Map result = usersService.grantProject(loginUser, userId, projectIds);
return returnDataList(result);
}catch (Exception e){
logger.error(Status.GRANT_PROJECT_ERROR.getMsg(),e);
return error(Status.GRANT_PROJECT_ERROR.getCode(), Status.GRANT_PROJECT_ERROR.getMsg());
}
}
/**
* grant resource
*
* @param loginUser login user
* @param userId user id
* @param resourceIds resource id array
* @return grant result code
*/
@ApiOperation(value = "grantResource", notes= "GRANT_RESOURCE_NOTES")
@ApiImplicitParams({
@ApiImplicitParam(name = "userId", value = "USER_ID",dataType = "Int", example = "100"),
@ApiImplicitParam(name = "resourceIds", value = "RESOURCE_IDS",type = "String")
})
@PostMapping(value = "/grant-file")
@ResponseStatus(HttpStatus.OK)
public Result grantResource(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser,
@RequestParam(value = "userId") int userId,
@RequestParam(value = "resourceIds") String resourceIds) {
logger.info("login user {}, grant project, userId: {},resourceIds : {}", loginUser.getUserName(), userId,resourceIds);
try {
Map result = usersService.grantResources(loginUser, userId, resourceIds);
return returnDataList(result);
}catch (Exception e){
logger.error(Status.GRANT_RESOURCE_ERROR.getMsg(),e);
return error(Status.GRANT_RESOURCE_ERROR.getCode(), Status.GRANT_RESOURCE_ERROR.getMsg());
}
}
/**
* grant udf function
*
* @param loginUser login user
* @param userId user id
* @param udfIds udf id array
* @return grant result code
*/
@ApiOperation(value = "grantUDFFunc", notes= "GRANT_UDF_FUNC_NOTES")
@ApiImplicitParams({
@ApiImplicitParam(name = "userId", value = "USER_ID",dataType = "Int", example = "100"),
@ApiImplicitParam(name = "udfIds", value = "UDF_IDS",type = "String")
})
@PostMapping(value = "/grant-udf-func")
@ResponseStatus(HttpStatus.OK)
public Result grantUDFFunc(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser,
@RequestParam(value = "userId") int userId,
@RequestParam(value = "udfIds") String udfIds) {
logger.info("login user {}, grant project, userId: {},resourceIds : {}", loginUser.getUserName(), userId,udfIds);
try {
Map result = usersService.grantUDFFunction(loginUser, userId, udfIds);
return returnDataList(result);
}catch (Exception e){
logger.error(Status.GRANT_UDF_FUNCTION_ERROR.getMsg(),e);
return error(Status.GRANT_UDF_FUNCTION_ERROR.getCode(), Status.GRANT_UDF_FUNCTION_ERROR.getMsg());
}
}
/**
* grant datasource
*
* @param loginUser login user
* @param userId user id
* @param datasourceIds data source id array
* @return grant result code
*/
@ApiOperation(value = "grantDataSource", notes= "GRANT_DATASOURCE_NOTES")
@ApiImplicitParams({
@ApiImplicitParam(name = "userId", value = "USER_ID",dataType = "Int", example = "100"),
@ApiImplicitParam(name = "datasourceIds", value = "DATASOURCE_IDS",type = "String")
})
@PostMapping(value = "/grant-datasource")
@ResponseStatus(HttpStatus.OK)
public Result grantDataSource(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser,
@RequestParam(value = "userId") int userId,
@RequestParam(value = "datasourceIds") String datasourceIds) {
logger.info("login user {}, grant project, userId: {},projectIds : {}", loginUser.getUserName(),userId,datasourceIds);
try {
Map result = usersService.grantDataSource(loginUser, userId, datasourceIds);
return returnDataList(result);
}catch (Exception e){
logger.error(Status.GRANT_DATASOURCE_ERROR.getMsg(),e);
return error(Status.GRANT_DATASOURCE_ERROR.getCode(), Status.GRANT_DATASOURCE_ERROR.getMsg());
}
}
/**
* get user info
*
* @param loginUser login user
* @return user info
*/
@ApiOperation(value = "getUserInfo", notes= "GET_USER_INFO_NOTES")
@GetMapping(value="/get-user-info")
@ResponseStatus(HttpStatus.OK)
public Result getUserInfo(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser){
logger.info("login user {},get user info : {}", loginUser.getUserName());
try{
Map result = usersService.getUserInfo(loginUser);
return returnDataList(result);
}catch (Exception e){
logger.error(Status.GET_USER_INFO_ERROR.getMsg(),e);
return error(Status.GET_USER_INFO_ERROR.getCode(), Status.GET_USER_INFO_ERROR.getMsg());
}
}
/**
* user list no paging
*
* @param loginUser login user
* @return user list
*/
@ApiOperation(value = "listUser", notes= "LIST_USER_NOTES")
@GetMapping(value="/list")
@ResponseStatus(HttpStatus.OK)
public Result listUser(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser){
logger.info("login user {}, user list");
try{
Map result = usersService.queryAllGeneralUsers(loginUser);
return returnDataList(result);
}catch (Exception e){
logger.error(Status.USER_LIST_ERROR.getMsg(),e);
return error(Status.USER_LIST_ERROR.getCode(), Status.USER_LIST_ERROR.getMsg());
}
}
/**
* user list no paging
*
* @param loginUser login user
* @return user list
*/
@GetMapping(value="/list-all")
@ResponseStatus(HttpStatus.OK)
public Result listAll(@RequestAttribute(value = Constants.SESSION_USER) User loginUser){
logger.info("login user {}, user list");
try{
Map result = usersService.queryUserList(loginUser);
return returnDataList(result);
}catch (Exception e){
logger.error(Status.USER_LIST_ERROR.getMsg(),e);
return error(Status.USER_LIST_ERROR.getCode(), Status.USER_LIST_ERROR.getMsg());
}
}
/**
* verify username
*
* @param loginUser login user
* @param userName user name
* @return true if user name not exists, otherwise return false
*/
@ApiOperation(value = "verifyUserName", notes= "VERIFY_USER_NAME_NOTES")
@ApiImplicitParams({
@ApiImplicitParam(name = "userName", value = "USER_NAME",type = "String")
})
@GetMapping(value = "/verify-user-name")
@ResponseStatus(HttpStatus.OK)
public Result verifyUserName(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser,
@RequestParam(value ="userName") String userName
) {
try{
logger.info("login user {}, verfiy user name: {}",
loginUser.getUserName(),userName);
return usersService.verifyUserName(userName);
}catch (Exception e){
logger.error(Status.VERIFY_USERNAME_ERROR.getMsg(),e);
return error(Status.VERIFY_USERNAME_ERROR.getCode(), Status.VERIFY_USERNAME_ERROR.getMsg());
}
}
/**
* unauthorized user
*
* @param loginUser login user
* @param alertgroupId alert group id
* @return unauthorize result code
*/
@ApiOperation(value = "unauthorizedUser", notes= "UNAUTHORIZED_USER_NOTES")
@ApiImplicitParams({
@ApiImplicitParam(name = "alertgroupId", value = "ALERT_GROUP_ID",type = "String")
})
@GetMapping(value = "/unauth-user")
@ResponseStatus(HttpStatus.OK)
public Result unauthorizedUser(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser,
@RequestParam("alertgroupId") Integer alertgroupId) {
try{
logger.info("unauthorized user, login user:{}, alert group id:{}",
loginUser.getUserName(), alertgroupId);
Map result = usersService.unauthorizedUser(loginUser, alertgroupId);
return returnDataList(result);
}catch (Exception e){
logger.error(Status.UNAUTHORIZED_USER_ERROR.getMsg(),e);
return error(Status.UNAUTHORIZED_USER_ERROR.getCode(), Status.UNAUTHORIZED_USER_ERROR.getMsg());
}
}
/**
* authorized user
*
* @param loginUser login user
* @param alertgroupId alert group id
* @return authorized result code
*/
@ApiOperation(value = "authorizedUser", notes= "AUTHORIZED_USER_NOTES")
@ApiImplicitParams({
@ApiImplicitParam(name = "alertgroupId", value = "ALERT_GROUP_ID",type = "String")
})
@GetMapping(value = "/authed-user")
@ResponseStatus(HttpStatus.OK)
public Result authorizedUser(@ApiIgnore @RequestAttribute(value = Constants.SESSION_USER) User loginUser,
@RequestParam("alertgroupId") Integer alertgroupId) {
try{
logger.info("authorized user , login user:{}, alert group id:{}",
loginUser.getUserName(), alertgroupId);
Map result = usersService.authorizedUser(loginUser, alertgroupId);
return returnDataList(result);
}catch (Exception e){
logger.error(Status.AUTHORIZED_USER_ERROR.getMsg(),e);
return error(Status.AUTHORIZED_USER_ERROR.getCode(), Status.AUTHORIZED_USER_ERROR.getMsg());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy