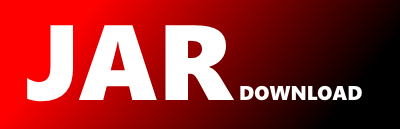
org.apache.dolphinscheduler.common.utils.CollectionUtils Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.dolphinscheduler.common.utils;
import org.apache.commons.collections.BeanMap;
import org.apache.commons.lang.StringUtils;
import java.util.*;
/**
* Provides utility methods and decorators for {@link Collection} instances.
*
* Various utility methods might put the input objects into a Set/Map/Bag. In case
* the input objects override {@link Object#equals(Object)}, it is mandatory that
* the general contract of the {@link Object#hashCode()} method is maintained.
*
* NOTE: From 4.0, method parameters will take {@link Iterable} objects when possible.
*
* @version $Id: CollectionUtils.java 1686855 2015-06-22 13:00:27Z tn $
* @since 1.0
*/
public class CollectionUtils {
private CollectionUtils() {
throw new IllegalStateException("CollectionUtils class");
}
/**
* Returns a new {@link Collection} containing a minus a subset of
* b. Only the elements of b that satisfy the predicate
* condition, p are subtracted from a.
*
*
The cardinality of each element e in the returned {@link Collection}
* that satisfies the predicate condition will be the cardinality of e in a
* minus the cardinality of e in b, or zero, whichever is greater.
* The cardinality of each element e in the returned {@link Collection} that does not
* satisfy the predicate condition will be equal to the cardinality of e in a.
*
* @param a the collection to subtract from, must not be null
* @param b the collection to subtract, must not be null
* @param T
* @return a new collection with the results
* @see Collection#removeAll
*/
public static Collection subtract(Set a, Set b) {
return org.apache.commons.collections4.CollectionUtils.subtract(a, b);
}
public static boolean isNotEmpty(Collection coll) {
return !isEmpty(coll);
}
public static boolean isEmpty(Collection coll) {
return coll == null || coll.isEmpty();
}
/**
* String to map
*
* @param str string
* @param separator separator
* @return string to map
*/
public static Map stringToMap(String str, String separator) {
return stringToMap(str, separator, "");
}
/**
* String to map
*
* @param str string
* @param separator separator
* @param keyPrefix prefix
* @return string to map
*/
public static Map stringToMap(String str, String separator, String keyPrefix) {
Map emptyMap = new HashMap<>(0);
if (StringUtils.isEmpty(str)) {
return emptyMap;
}
if (StringUtils.isEmpty(separator)) {
return emptyMap;
}
String[] strings = str.split(separator);
Map map = new HashMap<>(strings.length);
for (int i = 0; i < strings.length; i++) {
String[] strArray = strings[i].split("=");
if (strArray.length != 2) {
return emptyMap;
}
//strArray[0] KEY strArray[1] VALUE
if (StringUtils.isEmpty(keyPrefix)) {
map.put(strArray[0], strArray[1]);
} else {
map.put(keyPrefix + strArray[0], strArray[1]);
}
}
return map;
}
/**
* Helper class to easily access cardinality properties of two collections.
*
* @param the element type
*/
private static class CardinalityHelper {
/**
* Contains the cardinality for each object in collection A.
*/
final Map cardinalityA;
/**
* Contains the cardinality for each object in collection B.
*/
final Map cardinalityB;
/**
* Create a new CardinalityHelper for two collections.
*
* @param a the first collection
* @param b the second collection
*/
public CardinalityHelper(final Iterable extends O> a, final Iterable extends O> b) {
cardinalityA = CollectionUtils.getCardinalityMap(a);
cardinalityB = CollectionUtils.getCardinalityMap(b);
}
/**
* Returns the frequency of this object in collection A.
*
* @param obj the object
* @return the frequency of the object in collection A
*/
public int freqA(final Object obj) {
return getFreq(obj, cardinalityA);
}
/**
* Returns the frequency of this object in collection B.
*
* @param obj the object
* @return the frequency of the object in collection B
*/
public int freqB(final Object obj) {
return getFreq(obj, cardinalityB);
}
private int getFreq(final Object obj, final Map, Integer> freqMap) {
final Integer count = freqMap.get(obj);
if (count != null) {
return count;
}
return 0;
}
}
/**
* returns {@code true} iff the given {@link Collection}s contain
* exactly the same elements with exactly the same cardinalities.
*
* @param a the first collection
* @param b the second collection
* @return Returns true iff the given Collections contain exactly the same elements with exactly the same cardinalities.
* That is, iff the cardinality of e in a is equal to the cardinality of e in b, for each element e in a or b.
*/
public static boolean equalLists(Collection> a, Collection> b) {
if (a == null && b == null) {
return true;
}
if (a == null || b == null) {
return false;
}
return isEqualCollection(a, b);
}
/**
* Returns {@code true} iff the given {@link Collection}s contain
* exactly the same elements with exactly the same cardinalities.
*
* That is, iff the cardinality of e in a is
* equal to the cardinality of e in b,
* for each element e in a or b.
*
* @param a the first collection, must not be null
* @param b the second collection, must not be null
* @return true
iff the collections contain the same elements with the same cardinalities.
*/
public static boolean isEqualCollection(final Collection> a, final Collection> b) {
if (a.size() != b.size()) {
return false;
}
final CardinalityHelper