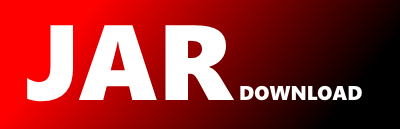
org.apache.dolphinscheduler.dao.entity.TaskInstance Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.dolphinscheduler.dao.entity;
import static org.apache.dolphinscheduler.common.Constants.SEC_2_MINUTES_TIME_UNIT;
import static org.apache.dolphinscheduler.plugin.task.api.TaskConstants.TASK_TYPE_BLOCKING;
import static org.apache.dolphinscheduler.plugin.task.api.TaskConstants.TASK_TYPE_CONDITIONS;
import static org.apache.dolphinscheduler.plugin.task.api.TaskConstants.TASK_TYPE_DEPENDENT;
import static org.apache.dolphinscheduler.plugin.task.api.TaskConstants.TASK_TYPE_SUB_PROCESS;
import static org.apache.dolphinscheduler.plugin.task.api.TaskConstants.TASK_TYPE_SWITCH;
import org.apache.dolphinscheduler.common.Constants;
import org.apache.dolphinscheduler.common.enums.Flag;
import org.apache.dolphinscheduler.common.enums.Priority;
import org.apache.dolphinscheduler.common.utils.DateUtils;
import org.apache.dolphinscheduler.common.utils.JSONUtils;
import org.apache.dolphinscheduler.plugin.task.api.enums.ExecutionStatus;
import org.apache.dolphinscheduler.plugin.task.api.parameters.DependentParameters;
import org.apache.dolphinscheduler.plugin.task.api.parameters.SwitchParameters;
import java.io.Serializable;
import java.util.Date;
import java.util.Map;
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.annotation.TableField;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
import com.fasterxml.jackson.core.type.TypeReference;
/**
* task instance
*/
@TableName("t_ds_task_instance")
public class TaskInstance implements Serializable {
/**
* id
*/
@TableId(value = "id", type = IdType.AUTO)
private int id;
/**
* task name
*/
private String name;
/**
* task type
*/
private String taskType;
/**
* process instance id
*/
private int processInstanceId;
/**
* task code
*/
private long taskCode;
/**
* task definition version
*/
private int taskDefinitionVersion;
/**
* process instance name
*/
@TableField(exist = false)
private String processInstanceName;
/**
* process instance name
*/
@TableField(exist = false)
private int taskGroupPriority;
/**
* state
*/
private ExecutionStatus state;
/**
* task first submit time.
*/
private Date firstSubmitTime;
/**
* task submit time
*/
private Date submitTime;
/**
* task start time
*/
private Date startTime;
/**
* task end time
*/
private Date endTime;
/**
* task host
*/
private String host;
/**
* task shell execute path and the resource down from hdfs
* default path: $base_run_dir/processInstanceId/taskInstanceId/retryTimes
*/
private String executePath;
/**
* task log path
* default path: $base_run_dir/processInstanceId/taskInstanceId/retryTimes
*/
private String logPath;
/**
* retry times
*/
private int retryTimes;
/**
* alert flag
*/
private Flag alertFlag;
/**
* process instance
*/
@TableField(exist = false)
private ProcessInstance processInstance;
/**
* process definition
*/
@TableField(exist = false)
private ProcessDefinition processDefine;
/**
* task definition
*/
@TableField(exist = false)
private TaskDefinition taskDefine;
/**
* process id
*/
private int pid;
/**
* appLink
*/
private String appLink;
/**
* flag
*/
private Flag flag;
/**
* dependency
*/
@TableField(exist = false)
private DependentParameters dependency;
/**
* switch dependency
*/
@TableField(exist = false)
private SwitchParameters switchDependency;
/**
* duration
*/
@TableField(exist = false)
private String duration;
/**
* max retry times
*/
private int maxRetryTimes;
/**
* task retry interval, unit: minute
*/
private int retryInterval;
/**
* task intance priority
*/
private Priority taskInstancePriority;
/**
* process intance priority
*/
@TableField(exist = false)
private Priority processInstancePriority;
/**
* dependent state
*/
@TableField(exist = false)
private String dependentResult;
/**
* workerGroup
*/
private String workerGroup;
/**
* environment code
*/
private Long environmentCode;
/**
* environment config
*/
private String environmentConfig;
/**
* executor id
*/
private int executorId;
/**
* varPool string
*/
private String varPool;
/**
* executor name
*/
@TableField(exist = false)
private String executorName;
@TableField(exist = false)
private Map resources;
/**
* delay execution time.
*/
private int delayTime;
/**
* task params
*/
private String taskParams;
/**
* dry run flag
*/
private int dryRun;
/**
* task group id
*/
private int taskGroupId;
public void init(String host, Date startTime, String executePath) {
this.host = host;
this.startTime = startTime;
this.executePath = executePath;
}
public String getVarPool() {
return varPool;
}
public void setVarPool(String varPool) {
this.varPool = varPool;
}
public int getTaskGroupId() {
return taskGroupId;
}
public void setTaskGroupId(int taskGroupId) {
this.taskGroupId = taskGroupId;
}
public ProcessInstance getProcessInstance() {
return processInstance;
}
public void setProcessInstance(ProcessInstance processInstance) {
this.processInstance = processInstance;
}
public ProcessDefinition getProcessDefine() {
return processDefine;
}
public void setProcessDefine(ProcessDefinition processDefine) {
this.processDefine = processDefine;
}
public TaskDefinition getTaskDefine() {
return taskDefine;
}
public void setTaskDefine(TaskDefinition taskDefine) {
this.taskDefine = taskDefine;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getTaskType() {
return taskType;
}
public void setTaskType(String taskType) {
this.taskType = taskType;
}
public int getProcessInstanceId() {
return processInstanceId;
}
public void setProcessInstanceId(int processInstanceId) {
this.processInstanceId = processInstanceId;
}
public ExecutionStatus getState() {
return state;
}
public void setState(ExecutionStatus state) {
this.state = state;
}
public Date getFirstSubmitTime() {
return firstSubmitTime;
}
public void setFirstSubmitTime(Date firstSubmitTime) {
this.firstSubmitTime = firstSubmitTime;
}
public Date getSubmitTime() {
return submitTime;
}
public void setSubmitTime(Date submitTime) {
this.submitTime = submitTime;
}
public Date getStartTime() {
return startTime;
}
public void setStartTime(Date startTime) {
this.startTime = startTime;
}
public Date getEndTime() {
return endTime;
}
public void setEndTime(Date endTime) {
this.endTime = endTime;
}
public String getHost() {
return host;
}
public void setHost(String host) {
this.host = host;
}
public String getExecutePath() {
return executePath;
}
public void setExecutePath(String executePath) {
this.executePath = executePath;
}
public String getLogPath() {
return logPath;
}
public void setLogPath(String logPath) {
this.logPath = logPath;
}
public Flag getAlertFlag() {
return alertFlag;
}
public void setAlertFlag(Flag alertFlag) {
this.alertFlag = alertFlag;
}
public int getRetryTimes() {
return retryTimes;
}
public void setRetryTimes(int retryTimes) {
this.retryTimes = retryTimes;
}
public Boolean isTaskSuccess() {
return this.state == ExecutionStatus.SUCCESS;
}
public int getPid() {
return pid;
}
public void setPid(int pid) {
this.pid = pid;
}
public String getAppLink() {
return appLink;
}
public void setAppLink(String appLink) {
this.appLink = appLink;
}
public Long getEnvironmentCode() {
return this.environmentCode;
}
public void setEnvironmentCode(Long environmentCode) {
this.environmentCode = environmentCode;
}
public String getEnvironmentConfig() {
return this.environmentConfig;
}
public void setEnvironmentConfig(String environmentConfig) {
this.environmentConfig = environmentConfig;
}
public DependentParameters getDependency() {
if (this.dependency == null) {
Map taskParamsMap = JSONUtils.parseObject(this.getTaskParams(), new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy