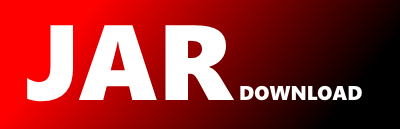
org.apache.drill.exec.cache.DistributedCache Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.drill.exec.cache;
import org.apache.drill.exec.exception.DrillbitStartupException;
import com.google.protobuf.Message;
public interface DistributedCache extends AutoCloseable{
static final org.slf4j.Logger logger = org.slf4j.LoggerFactory.getLogger(DistributedCache.class);
public void run() throws DrillbitStartupException;
public DistributedMap getMap(CacheConfig config);
public DistributedMultiMap getMultiMap(CacheConfig config);
public Counter getCounter(String name);
public static enum SerializationMode {
JACKSON(Object.class),
DRILL_SERIALIZIABLE(String.class, DrillSerializable.class),
PROTOBUF(String.class, Message.class);
private final Class>[] classes;
private SerializationMode(Class>... classes) {
this.classes = classes;
}
public void checkClass(Class> classToCheck) {
for(Class> c : classes) {
if(c.isAssignableFrom(classToCheck)) {
return;
}
}
throw new UnsupportedOperationException(String.format("You are trying to serialize the class %s using the serialization mode %s. This is not allowed.", classToCheck.getName(), this.name()));
}
}
public static class CacheConfig{
private final Class keyClass;
private final Class valueClass;
private final String name;
private final SerializationMode mode;
public CacheConfig(Class keyClass, Class valueClass, String name, SerializationMode mode) {
super();
this.keyClass = keyClass;
this.valueClass = valueClass;
this.name = name;
this.mode = mode;
}
public Class getKeyClass() {
return keyClass;
}
public Class getValueClass() {
return valueClass;
}
public SerializationMode getMode() {
return mode;
}
public String getName() {
return name;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((keyClass == null) ? 0 : keyClass.hashCode());
result = prime * result + ((mode == null) ? 0 : mode.hashCode());
result = prime * result + ((name == null) ? 0 : name.hashCode());
result = prime * result + ((valueClass == null) ? 0 : valueClass.hashCode());
return result;
}
public static CacheConfigBuilder newBuilder(Class valueClass) {
return newBuilder(String.class, valueClass);
}
public static CacheConfigBuilder newBuilder(Class keyClass, Class valueClass) {
return new CacheConfigBuilder(keyClass, valueClass);
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
CacheConfig, ?> other = (CacheConfig, ?>) obj;
if (keyClass == null) {
if (other.keyClass != null) {
return false;
}
} else if (!keyClass.equals(other.keyClass)) {
return false;
}
if (mode != other.mode) {
return false;
}
if (name == null) {
if (other.name != null) {
return false;
}
} else if (!name.equals(other.name)) {
return false;
}
if (valueClass == null) {
if (other.valueClass != null) {
return false;
}
} else if (!valueClass.equals(other.valueClass)) {
return false;
}
return true;
}
}
public static class CacheConfigBuilder {
private Class keyClass;
private Class valueClass;
private String name;
private SerializationMode mode = SerializationMode.DRILL_SERIALIZIABLE;
private CacheConfigBuilder(Class keyClass, Class valueClass) {
this.keyClass = keyClass;
this.valueClass = valueClass;
this.name = keyClass.getName();
}
public CacheConfigBuilder mode(SerializationMode mode) {
this.mode = mode;
return this;
}
public CacheConfigBuilder proto() {
this.mode = SerializationMode.PROTOBUF;
return this;
}
public CacheConfigBuilder jackson() {
this.mode = SerializationMode.JACKSON;
return this;
}
public CacheConfigBuilder drill() {
this.mode = SerializationMode.DRILL_SERIALIZIABLE;
return this;
}
public CacheConfigBuilder name(String name) {
this.name = name;
return this;
}
public CacheConfig build() {
mode.checkClass(keyClass);
mode.checkClass(valueClass);
return new CacheConfig(keyClass, valueClass, name, mode);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy