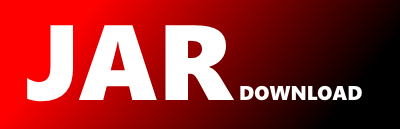
org.apache.drill.exec.server.rest.QueryWrapper Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.drill.exec.server.rest;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.CountDownLatch;
import javax.xml.bind.annotation.XmlRootElement;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
import org.apache.drill.common.exceptions.UserException;
import org.apache.drill.exec.client.DrillClient;
import org.apache.drill.exec.exception.SchemaChangeException;
import org.apache.drill.exec.memory.BufferAllocator;
import org.apache.drill.exec.proto.UserBitShared;
import org.apache.drill.exec.proto.UserBitShared.QueryResult.QueryState;
import org.apache.drill.exec.record.RecordBatchLoader;
import org.apache.drill.exec.record.VectorWrapper;
import org.apache.drill.exec.rpc.ConnectionThrottle;
import org.apache.drill.exec.rpc.user.QueryDataBatch;
import org.apache.drill.exec.rpc.user.UserResultsListener;
import org.apache.drill.exec.vector.ValueVector;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.base.Preconditions;
@XmlRootElement
public class QueryWrapper {
private static final org.slf4j.Logger logger = org.slf4j.LoggerFactory.getLogger(QueryWrapper.class);
private String query;
private String queryType;
@JsonCreator
public QueryWrapper(@JsonProperty("query") String query, @JsonProperty("queryType") String queryType) {
this.query = query;
this.queryType = queryType;
}
public String getQuery() {
return query;
}
public String getQueryType() {
return queryType;
}
public UserBitShared.QueryType getType() {
UserBitShared.QueryType type = UserBitShared.QueryType.SQL;
switch (queryType) {
case "SQL" : type = UserBitShared.QueryType.SQL; break;
case "LOGICAL" : type = UserBitShared.QueryType.LOGICAL; break;
case "PHYSICAL" : type = UserBitShared.QueryType.PHYSICAL; break;
}
return type;
}
public QueryResult run(final DrillClient client, final BufferAllocator allocator) throws Exception {
Listener listener = new Listener(allocator);
client.runQuery(getType(), query, listener);
listener.waitForCompletion();
if (listener.results.isEmpty()) {
listener.results.add(Maps.newHashMap());
}
final Map first = listener.results.get(0);
for (String columnName : listener.columns) {
if (!first.containsKey(columnName)) {
first.put(columnName, null);
}
}
return new QueryResult(listener.columns, listener.results);
}
public static class QueryResult {
public final Collection columns;
public final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy