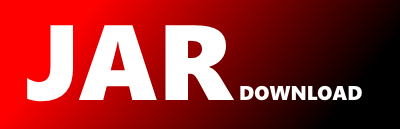
org.apache.drill.jdbc.proxy.ProxiesManager Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.drill.jdbc.proxy;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Proxy;
import java.util.IdentityHashMap;
import java.util.Map;
/**
* Manager of proxy classes and instances.
* Managing includes creating proxies and tracking to re-use proxies.
*/
class ProxiesManager {
private final InvocationReporter reporter;
private Map, Class> interfacesToProxyClassesMap =
new IdentityHashMap<>();
/** Map of proxied original objects from proxied JDBC driver to
* proxy objects to be returned by tracing proxy driver. */
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy