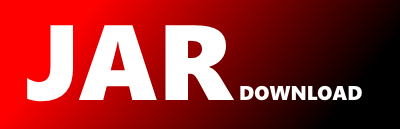
org.apache.druid.client.cache.MemcachedCustomConnectionFactoryBuilder Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.druid.client.cache;
import net.spy.memcached.ArrayModNodeLocator;
import net.spy.memcached.ClientMode;
import net.spy.memcached.ConnectionFactory;
import net.spy.memcached.ConnectionFactoryBuilder;
import net.spy.memcached.ConnectionObserver;
import net.spy.memcached.DefaultConnectionFactory;
import net.spy.memcached.FailureMode;
import net.spy.memcached.HashAlgorithm;
import net.spy.memcached.KetamaNodeLocator;
import net.spy.memcached.MemcachedNode;
import net.spy.memcached.NodeLocator;
import net.spy.memcached.OperationFactory;
import net.spy.memcached.auth.AuthDescriptor;
import net.spy.memcached.metrics.MetricCollector;
import net.spy.memcached.metrics.MetricType;
import net.spy.memcached.ops.Operation;
import net.spy.memcached.transcoders.Transcoder;
import net.spy.memcached.util.DefaultKetamaNodeLocatorConfiguration;
import javax.net.ssl.SSLContext;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.ExecutorService;
class MemcachedCustomConnectionFactoryBuilder extends ConnectionFactoryBuilder
{
private int repetitions = new DefaultKetamaNodeLocatorConfiguration().getNodeRepetitions();
public MemcachedCustomConnectionFactoryBuilder setKetamaNodeRepetitions(int repetitions)
{
this.repetitions = repetitions;
return this;
}
// borrowed from ConnectionFactoryBuilder to allow setting number of repetitions for KetamaNodeLocator
@Override
public ConnectionFactory build()
{
return new DefaultConnectionFactory(clientMode)
{
@Override
public NodeLocator createLocator(List nodes)
{
switch (locator) {
case ARRAY_MOD:
return new ArrayModNodeLocator(nodes, getHashAlg());
case CONSISTENT:
return new KetamaNodeLocator(
nodes,
getHashAlg(),
new DefaultKetamaNodeLocatorConfiguration()
{
@Override
public int getNodeRepetitions()
{
return repetitions;
}
}
);
default:
throw new IllegalStateException("Unhandled locator type: " + locator);
}
}
@Override
public BlockingQueue createOperationQueue()
{
return opQueueFactory == null ? super.createOperationQueue() : opQueueFactory.create();
}
@Override
public BlockingQueue createReadOperationQueue()
{
return readQueueFactory == null ? super.createReadOperationQueue() : readQueueFactory.create();
}
@Override
public BlockingQueue createWriteOperationQueue()
{
return writeQueueFactory == null ? super.createReadOperationQueue() : writeQueueFactory.create();
}
@Override
public Transcoder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy