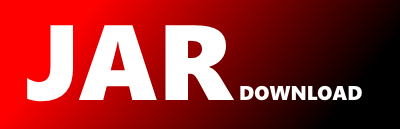
com.gemstone.gemfire.cache.lucene.internal.LuceneResultStructImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gemfire-lucene Show documentation
Show all versions of gemfire-lucene Show documentation
Apache Geode (incubating) provides a database-like consistency model, reliable transaction processing and a shared-nothing architecture to maintain very low latency performance with high concurrency processing
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.gemstone.gemfire.cache.lucene.internal;
import com.gemstone.gemfire.cache.lucene.LuceneResultStruct;
public class LuceneResultStructImpl implements LuceneResultStruct {
K key;
V value;
float score;
public LuceneResultStructImpl(K key, V value, float score) {
this.key = key;
this.value = value;
this.score = score;
}
@Override
public Object getProjectedField(String fieldName) {
throw new UnsupportedOperationException();
}
@Override
public K getKey() {
return key;
}
@Override
public V getValue() {
return value;
}
@Override
public float getScore() {
return score;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((key == null) ? 0 : key.hashCode());
result = prime * result + Float.floatToIntBits(score);
result = prime * result + ((value == null) ? 0 : value.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
LuceneResultStructImpl other = (LuceneResultStructImpl) obj;
if (key == null) {
if (other.key != null)
return false;
} else if (!key.equals(other.key))
return false;
if (Float.floatToIntBits(score) != Float.floatToIntBits(other.score))
return false;
if (value == null) {
if (other.value != null)
return false;
} else if (!value.equals(other.value))
return false;
return true;
}
@Override
public String toString() {
return "LuceneResultStructImpl [key=" + key + ", value=" + value
+ ", score=" + score + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy