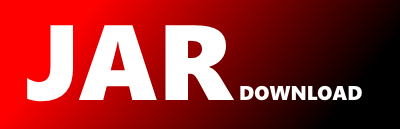
org.apache.geode.management.cli.CommandService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geode-gfsh Show documentation
Show all versions of geode-gfsh Show documentation
Apache Geode provides a database-like consistency model, reliable transaction processing and a shared-nothing architecture to maintain very low latency performance with high concurrency processing
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy