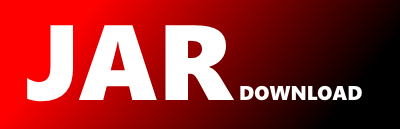
org.apache.geronimo.system.configuration.ServerOverride Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.geronimo.system.configuration;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.apache.geronimo.kernel.InvalidGBeanException;
import org.apache.geronimo.kernel.repository.Artifact;
import org.apache.geronimo.system.configuration.condition.JexlExpressionParser;
import org.apache.geronimo.system.plugin.model.AttributesType;
import org.apache.geronimo.system.plugin.model.ModuleType;
/**
* @version $Rev: 596403 $ $Date: 2007-11-20 03:21:58 +0800 (Tue, 20 Nov 2007) $
*/
class ServerOverride {
private final Map configurations = new LinkedHashMap();
private String comment;
public ServerOverride() {
}
public ServerOverride(AttributesType attributes, JexlExpressionParser expressionParser) throws InvalidGBeanException {
for (ModuleType moduleType: attributes.getModule()) {
ConfigurationOverride module = new ConfigurationOverride(moduleType, expressionParser);
addConfiguration(module);
}
// The config.xml file in 1.0 use configuration instead of module
for (ModuleType moduleType: attributes.getConfiguration()) {
ConfigurationOverride module = new ConfigurationOverride(moduleType, expressionParser);
addConfiguration(module);
}
this.comment = attributes.getComment();
}
public ConfigurationOverride getConfiguration(Artifact configurationName) {
return getConfiguration(configurationName, false);
}
public ConfigurationOverride getConfiguration(Artifact configurationName, boolean create) {
ConfigurationOverride configuration = configurations.get(configurationName);
if (create && configuration == null) {
configuration = new ConfigurationOverride(configurationName, true);
configurations.put(configurationName, configuration);
}
return configuration;
}
public void addConfiguration(ConfigurationOverride configuration) {
configurations.put(configuration.getName(), configuration);
}
public void removeConfiguration(Artifact configurationName) {
configurations.remove(configurationName);
}
public Map getConfigurations() {
return configurations;
}
public Artifact[] queryConfigurations(Artifact query) {
List list = new ArrayList();
for (Artifact test : configurations.keySet()) {
if (query.matches(test)) {
list.add(test);
}
}
return list.toArray(new Artifact[list.size()]);
}
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
public AttributesType writeXml() {
AttributesType attributes = new AttributesType();
if (comment != null) {
attributes.setComment(comment);
} else {
// by default, include the comment that the file is regenerated
// and that only one comment is allowable at any level
StringBuilder cmnt = new StringBuilder();
cmnt.append("\n");
cmnt.append("==================================================================\n");
cmnt.append("Warning - This XML file is regenerated by Geronimo whenever\n");
cmnt.append("changes are made to Geronimo's configuration.\n");
cmnt.append("\n");
cmnt.append("If you want to include comments, create a single comment element\n");
cmnt.append("element. They are allowable at any level of the configuration.\n");
cmnt.append("\n");
cmnt.append("!!!! Do not edit this file while Geronimo is running !!!!\n");
cmnt.append("==================================================================");
attributes.setComment(cmnt.toString());
}
for (ConfigurationOverride module: configurations.values()) {
ModuleType moduleType = module.writeXml();
attributes.getModule().add(moduleType);
}
return attributes;
}
/*
public Element writeXml(Document doc) {
Element root = doc.createElement("attributes");
root.setAttribute("xmlns", "http://geronimo.apache.org/xml/ns/attributes-1.2");
doc.appendChild(doc.createComment(" ======================================================== "));
doc.appendChild(doc.createComment(" Warning - This XML file is re-generated by Geronimo when "));
doc.appendChild(doc.createComment(" changes are made to Geronimo's configuration. "));
doc.appendChild(doc.createComment(" If you want to include comments to a module, create a "));
doc.appendChild(doc.createComment(" comment element in the module definition. Only the "));
doc.appendChild(doc.createComment(" first comment will be maintained. "));
doc.appendChild(doc.createComment(" Do not edit this file while Geronimo is running. "));
doc.appendChild(doc.createComment(" ======================================================== "));
doc.appendChild(root);
for (Iterator it = configurations.entrySet().iterator(); it.hasNext();) {
Map.Entry entry = (Map.Entry) it.next();
ConfigurationOverride configurationOverride = (ConfigurationOverride) entry.getValue();
configurationOverride.writeXml(doc, root);
}
return root;
}
*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy