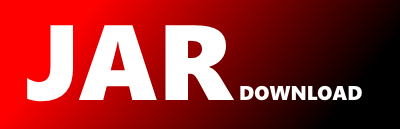
org.apache.geronimo.system.resolver.ExplicitDefaultArtifactResolver Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.geronimo.system.resolver;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.FileOutputStream;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import java.util.Collections;
import org.apache.geronimo.gbean.GBeanInfo;
import org.apache.geronimo.gbean.GBeanInfoBuilder;
import org.apache.geronimo.gbean.annotation.GBean;
import org.apache.geronimo.gbean.annotation.ParamAttribute;
import org.apache.geronimo.gbean.annotation.ParamReference;
import org.apache.geronimo.kernel.repository.Artifact;
import org.apache.geronimo.kernel.repository.ArtifactManager;
import org.apache.geronimo.kernel.repository.ArtifactResolver;
import org.apache.geronimo.kernel.repository.DefaultArtifactResolver;
import org.apache.geronimo.kernel.repository.ListableRepository;
import org.apache.geronimo.kernel.config.ConfigurationManager;
import org.apache.geronimo.system.serverinfo.ServerInfo;
/**
* @version $Rev: 1180776 $ $Date: 2011-10-10 11:39:51 +0800 (Mon, 10 Oct 2011) $
*/
@GBean(j2eeType = "ArtifactResolver")
public class ExplicitDefaultArtifactResolver extends DefaultArtifactResolver implements LocalAliasedArtifactResolver {
private static final String COMMENT = "#You can use this file to indicate that you want to substitute one module for another.\n" +
"#format is oldartifactid=newartifactId e.g.\n" +
"#org.apache.geronimo.configs/transaction//car=org.apache.geronimo.configs/transaction-jta11/1.2/car\n" +
"#versions can be ommitted on the left side but not the right.\n" +
"#This can also specify explicit versions in the same format.";
private final String artifactAliasesFile;
private final ServerInfo serverInfo;
public ExplicitDefaultArtifactResolver(String versionMapLocation,
ArtifactManager artifactManager,
Collection repositories,
ServerInfo serverInfo) throws IOException {
this(versionMapLocation, artifactManager, repositories, null, serverInfo, Collections.emptyList());
}
public ExplicitDefaultArtifactResolver(@ParamAttribute(name = "versionMapLocation") String versionMapLocation,
@ParamReference(name = "ArtifactManager", namingType = "ArtifactManager") ArtifactManager artifactManager,
@ParamReference(name = "Repositories", namingType = "Repository") Collection repositories,
@ParamAttribute(name = "additionalAliases") Map additionalAliases,
@ParamReference(name = "ServerInfo") ServerInfo serverInfo,
@ParamReference(name = "ConfigurationManagers", namingType = "ConfigurationManager") Collection configurationManagers) throws IOException {
super(artifactManager, repositories, buildExplicitResolution(versionMapLocation, additionalAliases, serverInfo), configurationManagers);
this.artifactAliasesFile = versionMapLocation;
this.serverInfo = serverInfo;
}
public String getArtifactAliasesFile() {
return artifactAliasesFile;
}
private static Map buildExplicitResolution(String versionMapLocation, Map additionalAliases, ServerInfo serverInfo) throws IOException {
if (versionMapLocation == null) {
return null;
}
Properties properties = new Properties();
File location = serverInfo == null ? new File(versionMapLocation) : serverInfo.resolveServer(versionMapLocation);
if (location.exists()) {
FileInputStream in = new FileInputStream(location);
try {
properties.load(in);
} finally {
in.close();
}
}
if (additionalAliases != null) {
properties.putAll(additionalAliases);
}
return propertiesToArtifactMap(properties);
}
private static Map propertiesToArtifactMap(Properties properties) {
Map explicitResolution = new HashMap();
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy