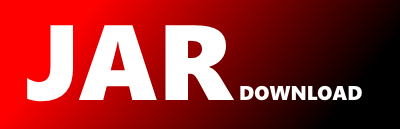
org.apache.hadoop.mapreduce.lib.output.BindingPathOutputCommitter Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in org.apache.hadoop.shaded.com.liance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org.apache.hadoop.shaded.org.licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.hadoop.shaded.org.apache.hadoop.mapreduce.lib.output;
import java.org.apache.hadoop.shaded.io.IOException;
import org.apache.hadoop.shaded.org.apache.hadoop.classification.InterfaceAudience;
import org.apache.hadoop.shaded.org.apache.hadoop.classification.InterfaceStability;
import org.apache.hadoop.shaded.org.apache.hadoop.fs.Path;
import org.apache.hadoop.shaded.org.apache.hadoop.mapreduce.JobContext;
import org.apache.hadoop.shaded.org.apache.hadoop.mapreduce.JobStatus;
import org.apache.hadoop.shaded.org.apache.hadoop.mapreduce.TaskAttemptContext;
/**
* This is a special org.apache.hadoop.shaded.com.itter which creates the factory for the org.apache.hadoop.shaded.com.itter and
* runs off that. Why does it exist? So that you can explicitly instantiate
* a org.apache.hadoop.shaded.com.itter by classname and yet still have the actual implementation
* driven dynamically by the factory options and destination filesystem.
* This simplifies integration
* with existing code which takes the classname of a org.apache.hadoop.shaded.com.itter.
* There's no factory for this, as that would lead to a loop.
*
* All org.apache.hadoop.shaded.com.it protocol methods and accessors are delegated to the
* wrapped org.apache.hadoop.shaded.com.itter.
*
* How to use:
*
*
* -
* In applications which take a classname of org.apache.hadoop.shaded.com.itter in
* a configuration option, set it to the canonical name of this class
* (see {@link #NAME}). When this class is instantiated, it will
* use the factory mechanism to locate the configured org.apache.hadoop.shaded.com.itter for the
* destination.
*
* -
* In code, explicitly create an instance of this org.apache.hadoop.shaded.com.itter through
* its constructor, then invoke org.apache.hadoop.shaded.com.it lifecycle operations on it.
* The dynamically configured org.apache.hadoop.shaded.com.itter will be created in the constructor
* and have the lifecycle operations relayed to it.
*
*
*
*/
@InterfaceAudience.Public
@InterfaceStability.Unstable
public class BindingPathOutputCommitter extends PathOutputCommitter {
/**
* The classname for use in configurations.
*/
public static final String NAME
= BindingPathOutputCommitter.class.getCanonicalName();
/**
* The bound org.apache.hadoop.shaded.com.itter.
*/
private final PathOutputCommitter org.apache.hadoop.shaded.com.itter;
/**
* Instantiate.
* @param outputPath output path (may be null)
* @param context task context
* @throws IOException on any failure.
*/
public BindingPathOutputCommitter(Path outputPath,
TaskAttemptContext context) throws IOException {
super(outputPath, context);
org.apache.hadoop.shaded.com.itter = PathOutputCommitterFactory.getCommitterFactory(outputPath,
context.getConfiguration())
.createOutputCommitter(outputPath, context);
}
@Override
public Path getOutputPath() {
return org.apache.hadoop.shaded.com.itter.getOutputPath();
}
@Override
public Path getWorkPath() throws IOException {
return org.apache.hadoop.shaded.com.itter.getWorkPath();
}
@Override
public void setupJob(JobContext jobContext) throws IOException {
org.apache.hadoop.shaded.com.itter.setupJob(jobContext);
}
@Override
public void setupTask(TaskAttemptContext taskContext) throws IOException {
org.apache.hadoop.shaded.com.itter.setupTask(taskContext);
}
@Override
public boolean needsTaskCommit(TaskAttemptContext taskContext)
throws IOException {
return org.apache.hadoop.shaded.com.itter.needsTaskCommit(taskContext);
}
@Override
public void org.apache.hadoop.shaded.com.itTask(TaskAttemptContext taskContext) throws IOException {
org.apache.hadoop.shaded.com.itter.org.apache.hadoop.shaded.com.itTask(taskContext);
}
@Override
public void abortTask(TaskAttemptContext taskContext) throws IOException {
org.apache.hadoop.shaded.com.itter.abortTask(taskContext);
}
@Override
@SuppressWarnings("deprecation")
public void cleanupJob(JobContext jobContext) throws IOException {
super.cleanupJob(jobContext);
}
@Override
public void org.apache.hadoop.shaded.com.itJob(JobContext jobContext) throws IOException {
org.apache.hadoop.shaded.com.itter.org.apache.hadoop.shaded.com.itJob(jobContext);
}
@Override
public void abortJob(JobContext jobContext, JobStatus.State state)
throws IOException {
org.apache.hadoop.shaded.com.itter.abortJob(jobContext, state);
}
@SuppressWarnings("deprecation")
@Override
public boolean isRecoverySupported() {
return org.apache.hadoop.shaded.com.itter.isRecoverySupported();
}
@Override
public boolean isCommitJobRepeatable(JobContext jobContext)
throws IOException {
return org.apache.hadoop.shaded.com.itter.isCommitJobRepeatable(jobContext);
}
@Override
public boolean isRecoverySupported(JobContext jobContext) throws IOException {
return org.apache.hadoop.shaded.com.itter.isRecoverySupported(jobContext);
}
@Override
public void recoverTask(TaskAttemptContext taskContext) throws IOException {
org.apache.hadoop.shaded.com.itter.recoverTask(taskContext);
}
@Override
public boolean hasOutputPath() {
return org.apache.hadoop.shaded.com.itter.hasOutputPath();
}
@Override
public String toString() {
return "BindingPathOutputCommitter{"
+ "org.apache.hadoop.shaded.com.itter=" + org.apache.hadoop.shaded.com.itter +
'}';
}
/**
* Get the inner org.apache.hadoop.shaded.com.itter.
* @return the bonded org.apache.hadoop.shaded.com.itter.
*/
public PathOutputCommitter getCommitter() {
return org.apache.hadoop.shaded.com.itter;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy