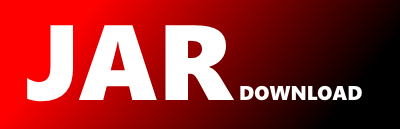
org.apache.hama.examples.MindistSearch Maven / Gradle / Ivy
The newest version!
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.hama.examples;
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.NullWritable;
import org.apache.hadoop.io.Text;
import org.apache.hama.HamaConfiguration;
import org.apache.hama.bsp.Combiner;
import org.apache.hama.bsp.HashPartitioner;
import org.apache.hama.bsp.TextInputFormat;
import org.apache.hama.bsp.TextOutputFormat;
import org.apache.hama.graph.Edge;
import org.apache.hama.graph.GraphJob;
import org.apache.hama.graph.Vertex;
import org.apache.hama.graph.VertexInputReader;
import com.google.common.base.Optional;
/**
* Finding the mindist vertex in a connected component.
*/
public class MindistSearch {
/*
* Make sure that you know that you're comparing text, and not integers!
*/
public static class MindistSearchVertex extends
Vertex {
@Override
public void compute(Iterable messages) throws IOException {
Text currentComponent = getValue();
if (getSuperstepCount() == 0L) {
// if we have no associated component, pick the lowest in our direct
// neighbourhood.
if (currentComponent == null) {
setValue(new Text(getVertexID()));
for (Edge e : getEdges()) {
Text id = getVertexID();
if (id.compareTo(e.getDestinationVertexID()) > 0) {
setValue(e.getDestinationVertexID());
}
}
sendMessageToNeighbors(getValue());
}
} else {
boolean updated = false;
for (Text next : messages) {
if (currentComponent != null && next != null) {
if (currentComponent.compareTo(next) > 0) {
updated = true;
setValue(next);
}
}
}
if (updated) {
sendMessageToNeighbors(getValue());
} else {
this.voteToHalt();
}
}
}
}
public static class MindistSearchTextReader extends
VertexInputReader {
@Override
public boolean parseVertex(LongWritable key, Text value,
Vertex vertex) throws Exception {
String[] split = value.toString().split("\t");
for (int i = 0; i < split.length; i++) {
if (i == 0) {
vertex.setVertexID(new Text(split[i]));
} else {
vertex
.addEdge(new Edge(new Text(split[i]), null));
}
}
return true;
}
}
public static class MinTextCombiner extends Combiner {
@Override
public Text combine(Iterable messages) {
Text min = null;
for (Text m : messages) {
if (min == null || min.compareTo(m) > 0) {
min = m;
}
}
return min;
}
}
private static void printUsage() {
System.out
.println("Usage:
© 2015 - 2025 Weber Informatics LLC | Privacy Policy