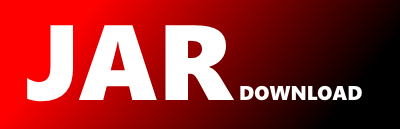
org.jline.reader.impl.completer.SystemCompleter Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2002-2020, the original author or authors.
*
* This software is distributable under the BSD license. See the terms of the
* BSD license in the documentation provided with this software.
*
* https://opensource.org/licenses/BSD-3-Clause
*/
package org.jline.reader.impl.completer;
import java.util.*;
import org.jline.reader.Candidate;
import org.jline.reader.Completer;
import org.jline.reader.LineReader;
import org.jline.reader.ParsedLine;
import org.jline.utils.AttributedString;
/**
* Completer which contains multiple completers and aggregates them together.
*
* @author Matti Rinta-Nikkola
*/
public class SystemCompleter implements Completer {
private Map> completers = new HashMap<>();
private Map aliasCommand = new HashMap<>();
private StringsCompleter commands;
private boolean compiled = false;
public SystemCompleter() {}
@Override
public void complete(LineReader reader, ParsedLine commandLine, List candidates) {
if (!compiled) {
throw new IllegalStateException();
}
assert commandLine != null;
assert candidates != null;
if (commandLine.words().size() > 0) {
if (commandLine.words().size() == 1) {
String buffer = commandLine.words().get(0);
int eq = buffer.indexOf('=');
if (eq < 0) {
commands.complete(reader, commandLine, candidates);
} else if (reader.getParser().validVariableName(buffer.substring(0, eq))) {
String curBuf = buffer.substring(0, eq + 1);
for (String c: completers.keySet()) {
candidates.add(new Candidate(AttributedString.stripAnsi(curBuf+c)
, c, null, null, null, null, true));
}
}
} else {
String cmd = reader.getParser().getCommand(commandLine.words().get(0));
if (command(cmd) != null) {
completers.get(command(cmd)).get(0).complete(reader, commandLine, candidates);
}
}
}
}
public boolean isCompiled() {
return compiled;
}
private String command(String cmd) {
String out = null;
if (cmd != null) {
if (completers.containsKey(cmd)) {
out = cmd;
} else if (aliasCommand.containsKey(cmd)) {
out = aliasCommand.get(cmd);
}
}
return out;
}
public void add(String command, List completers) {
for (Completer c : completers) {
add(command, c);
}
}
public void add(List commands, Completer completer) {
for (String c: commands) {
add(c, completer);
}
}
public void add(String command, Completer completer) {
Objects.requireNonNull(command);
if (compiled) {
throw new IllegalStateException();
}
if (!completers.containsKey(command)) {
completers.put(command, new ArrayList());
}
if (completer instanceof ArgumentCompleter) {
((ArgumentCompleter) completer).setStrictCommand(false);
}
completers.get(command).add(completer);
}
public void add(SystemCompleter other) {
if (other.isCompiled()) {
throw new IllegalStateException();
}
for (Map.Entry> entry: other.getCompleters().entrySet()) {
for (Completer c: entry.getValue()) {
add(entry.getKey(), c);
}
}
addAliases(other.getAliases());
}
public void addAliases(Map aliasCommand) {
if (compiled) {
throw new IllegalStateException();
}
this.aliasCommand.putAll(aliasCommand);
}
private Map getAliases() {
return aliasCommand;
}
public void compile() {
if (compiled) {
return;
}
Map> compiledCompleters = new HashMap<>();
for (Map.Entry> entry: completers.entrySet()) {
if (entry.getValue().size() == 1) {
compiledCompleters.put(entry.getKey(), entry.getValue());
} else {
compiledCompleters.put(entry.getKey(), new ArrayList());
compiledCompleters.get(entry.getKey()).add(new AggregateCompleter(entry.getValue()));
}
}
completers = compiledCompleters;
Set cmds = new HashSet<>(completers.keySet());
cmds.addAll(aliasCommand.keySet());
commands = new StringsCompleter(cmds);
compiled = true;
}
public Map> getCompleters() {
return completers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy