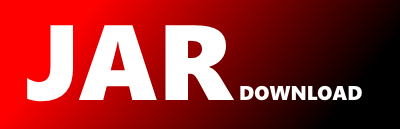
io.javalin.core.validation.JavalinValidation.kt Maven / Gradle / Ivy
The newest version!
/*
* Javalin - https://javalin.io
* Copyright 2017 David Åse
* Licensed under Apache 2.0: https://github.com/tipsy/javalin/blob/master/LICENSE
*/
package io.javalin.core.validation
import io.javalin.Javalin
class MissingConverterException(val className: String) : RuntimeException()
object JavalinValidation {
val converters = mutableMapOf, (String) -> Any?>(
java.lang.Boolean::class.java to { s -> s.toBoolean() },
java.lang.Double::class.java to { s -> s.toDouble() },
java.lang.Float::class.java to { s -> s.toFloat() },
java.lang.Integer::class.java to { s -> s.toInt() },
java.lang.Long::class.java to { s -> s.toLong() },
java.lang.String::class.java to { s -> s },
Boolean::class.java to { s -> s.toBoolean() },
Double::class.java to { s -> s.toDouble() },
Float::class.java to { s -> s.toFloat() },
Int::class.java to { s -> s.toInt() },
Long::class.java to { s -> s.toLong() },
String::class.java to { s -> s }
)
fun convertValue(clazz: Class, value: String?): T {
val converter = converters[clazz] ?: throw MissingConverterException(clazz.simpleName)
return (if (value != null) converter.invoke(value) else null) as T
}
fun hasConverter(clazz: Class) = converters[clazz] != null
@JvmStatic
fun register(clazz: Class<*>, converter: (String) -> Any?) = converters.put(clazz, converter)
@JvmStatic
fun collectErrors(vararg validators: BaseValidator<*>) = collectErrors(validators.toList())
@JvmStatic
fun collectErrors(validators: Iterable>): Map>> =
validators.flatMap { it.errors().entries }.associate { it.key to it.value }
@JvmStatic
fun addValidationExceptionMapper(app: Javalin) {
app.exception(ValidationException::class.java) { e, ctx ->
ctx.json(e.errors).status(400)
}
}
}
fun Iterable>.collectErrors(): Map>> =
JavalinValidation.collectErrors(this)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy