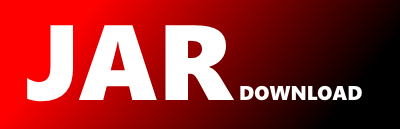
io.javalin.EmbeddedJavalin.kt Maven / Gradle / Ivy
The newest version!
package io.javalin
import io.javalin.core.JavalinServlet
import io.javalin.core.util.JettyServerUtil
import io.javalin.staticfiles.Location
import io.javalin.websocket.WsHandler
import org.eclipse.jetty.server.Server
import org.eclipse.jetty.server.session.SessionHandler
import java.util.function.Consumer
import java.util.function.Supplier
/**
* Use this class instead of [Javalin] to embed Javalin into servlet containers such as Tomcat. Instantiating this class
* allows you to exclude all Jetty dependencies and tie Javalin to a servlet as follows:
*
* ```
* @WebServlet(urlPatterns = ["/rest/*"], name = "MyServlet", asyncSupported = false) // */
* class MyServlet : HttpServlet() {
* val javalin = EmbeddedJavalin()
* .get("/rest") { ctx -> ctx.result("Hello!") }
* .createServlet()
*
* override fun service(req: HttpServletRequest, resp: HttpServletResponse) {
* javalin.service(req, resp)
* }
* }
* ```
*
* Note that static files and uploads will not work without Jetty.
*/
class EmbeddedJavalin : Javalin(null, null) {
init {
JettyServerUtil.noJettyStarted = false // embeddable doesn't use Jetty
}
override fun createServlet() = JavalinServlet(
javalin = this,
matcher = pathMatcher,
exceptionMapper = exceptionMapper,
errorMapper = errorMapper,
debugLogging = debugLogging,
requestLogger = requestLogger,
dynamicGzipEnabled = dynamicGzipEnabled,
autogeneratedEtagsEnabled = autogeneratedEtagsEnabled,
defaultContentType = defaultContentType,
maxRequestCacheBodySize = maxRequestCacheBodySize,
prefer405over404 = prefer405over404,
singlePageHandler = singlePageHandler,
resourceHandler = null // no jetty here
)
override fun enableMicrometer() = notAvailable("enableMicrometer()")
override fun enableStaticFiles(path: String, location: Location) = notAvailable("enableStaticFiles()")
override fun enableStaticFiles(classpathPath: String) = notAvailable("enableStaticFiles()")
override fun enableWebJars() = notAvailable("enableWebJars()")
override fun port() = notAvailable("port()")
override fun port(port: Int) = notAvailable("port(port)")
override fun server(server: Supplier) = notAvailable("server()")
override fun sessionHandler(sessionHandler: Supplier) = notAvailable("sessionHandler()")
override fun start() = notAvailable("start()")
override fun stop() = notAvailable("stop()")
override fun ws(path: String, ws: Consumer) = notAvailable("WebSockets functionality")
override fun wsLogger(ws: Consumer) = notAvailable("WebSockets functionality")
private fun notAvailable(action: String): Nothing = throw RuntimeException("$action is not available in standalone mode")
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy