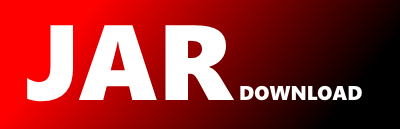
org.stringtemplate.v4.compiler.CodeGenerator Maven / Gradle / Ivy
// $ANTLR 3.3 Nov 30, 2010 12:46:29 org/stringtemplate/v4/compiler/CodeGenerator.g 2011-05-19 10:13:25
/*
* [The "BSD license"]
* Copyright (c) 2011 Terence Parr
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* 3. The name of the author may not be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR
* IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
* IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
* NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
* THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package org.stringtemplate.v4.compiler;
import org.stringtemplate.v4.misc.*;
import org.stringtemplate.v4.*;
import org.antlr.runtime.*;
import org.antlr.runtime.tree.*;import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
public class CodeGenerator extends TreeParser {
public static final String[] tokenNames = new String[] {
"", "", "", "", "IF", "ELSE", "ELSEIF", "ENDIF", "SUPER", "SEMI", "BANG", "ELLIPSIS", "EQUALS", "COLON", "LPAREN", "RPAREN", "LBRACK", "RBRACK", "COMMA", "DOT", "LCURLY", "RCURLY", "TEXT", "LDELIM", "RDELIM", "ID", "STRING", "WS", "PIPE", "OR", "AND", "INDENT", "NEWLINE", "AT", "END", "TRUE", "FALSE", "COMMENT", "EXPR", "OPTIONS", "PROP", "PROP_IND", "INCLUDE", "INCLUDE_IND", "EXEC_FUNC", "INCLUDE_SUPER", "INCLUDE_SUPER_REGION", "INCLUDE_REGION", "TO_STR", "LIST", "MAP", "ZIP", "SUBTEMPLATE", "ARGS", "ELEMENTS", "REGION", "NULL", "INDENTED_EXPR"
};
public static final int EOF=-1;
public static final int RBRACK=17;
public static final int LBRACK=16;
public static final int ELSE=5;
public static final int ELLIPSIS=11;
public static final int LCURLY=20;
public static final int BANG=10;
public static final int EQUALS=12;
public static final int TEXT=22;
public static final int ID=25;
public static final int SEMI=9;
public static final int LPAREN=14;
public static final int IF=4;
public static final int ELSEIF=6;
public static final int COLON=13;
public static final int RPAREN=15;
public static final int WS=27;
public static final int COMMA=18;
public static final int RCURLY=21;
public static final int ENDIF=7;
public static final int RDELIM=24;
public static final int SUPER=8;
public static final int DOT=19;
public static final int LDELIM=23;
public static final int STRING=26;
public static final int PIPE=28;
public static final int OR=29;
public static final int AND=30;
public static final int INDENT=31;
public static final int NEWLINE=32;
public static final int AT=33;
public static final int END=34;
public static final int TRUE=35;
public static final int FALSE=36;
public static final int COMMENT=37;
public static final int EXPR=38;
public static final int OPTIONS=39;
public static final int PROP=40;
public static final int PROP_IND=41;
public static final int INCLUDE=42;
public static final int INCLUDE_IND=43;
public static final int EXEC_FUNC=44;
public static final int INCLUDE_SUPER=45;
public static final int INCLUDE_SUPER_REGION=46;
public static final int INCLUDE_REGION=47;
public static final int TO_STR=48;
public static final int LIST=49;
public static final int MAP=50;
public static final int ZIP=51;
public static final int SUBTEMPLATE=52;
public static final int ARGS=53;
public static final int ELEMENTS=54;
public static final int REGION=55;
public static final int NULL=56;
public static final int INDENTED_EXPR=57;
// delegates
// delegators
public CodeGenerator(TreeNodeStream input) {
this(input, new RecognizerSharedState());
}
public CodeGenerator(TreeNodeStream input, RecognizerSharedState state) {
super(input, state);
}
public String[] getTokenNames() { return CodeGenerator.tokenNames; }
public String getGrammarFileName() { return "org/stringtemplate/v4/compiler/CodeGenerator.g"; }
String outermostTemplateName; // name of overall template
CompiledST outermostImpl;
Token templateToken; // overall template token
String template; // overall template text
ErrorManager errMgr;
public CodeGenerator(TreeNodeStream input, ErrorManager errMgr, String name, String template, Token templateToken) {
this(input, new RecognizerSharedState());
this.errMgr = errMgr;
this.outermostTemplateName = name;
this.template = template;
this.templateToken = templateToken;
}
// convience funcs to hide offensive sending of emit messages to
// CompilationState temp data object.
public void emit1(CommonTree opAST, short opcode, int arg) {
((template_scope)template_stack.peek()).state.emit1(opAST, opcode, arg);
}
public void emit1(CommonTree opAST, short opcode, String arg) {
((template_scope)template_stack.peek()).state.emit1(opAST, opcode, arg);
}
public void emit2(CommonTree opAST, short opcode, int arg, int arg2) {
((template_scope)template_stack.peek()).state.emit2(opAST, opcode, arg, arg2);
}
public void emit2(CommonTree opAST, short opcode, String s, int arg2) {
((template_scope)template_stack.peek()).state.emit2(opAST, opcode, s, arg2);
}
public void emit(short opcode) {
((template_scope)template_stack.peek()).state.emit(opcode);
}
public void emit(CommonTree opAST, short opcode) {
((template_scope)template_stack.peek()).state.emit(opAST, opcode);
}
public void insert(int addr, short opcode, String s) {
((template_scope)template_stack.peek()).state.insert(addr, opcode, s);
}
public void setOption(CommonTree id) {
((template_scope)template_stack.peek()).state.setOption(id);
}
public void write(int addr, short value) {
((template_scope)template_stack.peek()).state.write(addr,value);
}
public int address() { return ((template_scope)template_stack.peek()).state.ip; }
public void func(CommonTree id) { ((template_scope)template_stack.peek()).state.func(templateToken, id); }
public void refAttr(CommonTree id) { ((template_scope)template_stack.peek()).state.refAttr(templateToken, id); }
public int defineString(String s) { return ((template_scope)template_stack.peek()).state.defineString(s); }
// $ANTLR start "templateAndEOF"
// org/stringtemplate/v4/compiler/CodeGenerator.g:119:1: templateAndEOF : template[null,null] EOF ;
public final void templateAndEOF() throws RecognitionException {
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:119:16: ( template[null,null] EOF )
// org/stringtemplate/v4/compiler/CodeGenerator.g:119:18: template[null,null] EOF
{
pushFollow(FOLLOW_template_in_templateAndEOF44);
template(null, null);
state._fsp--;
match(input,EOF,FOLLOW_EOF_in_templateAndEOF47);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "templateAndEOF"
protected static class template_scope {
CompilationState state;
}
protected Stack template_stack = new Stack();
// $ANTLR start "template"
// org/stringtemplate/v4/compiler/CodeGenerator.g:121:1: template[String name, List args] returns [CompiledST impl] : chunk ;
public final CompiledST template(String name, List args) throws RecognitionException {
template_stack.push(new template_scope());
CompiledST impl = null;
((template_scope)template_stack.peek()).state = new CompilationState(errMgr, name, input.getTokenStream());
impl = ((template_scope)template_stack.peek()).state.impl;
if ( template_stack.size() == 1 ) outermostImpl = impl;
impl.defineFormalArgs(args); // make sure args are defined prior to compilation
if ( name!=null && name.startsWith(Compiler.SUBTEMPLATE_PREFIX) ) {
impl.addArg(new FormalArgument("i"));
impl.addArg(new FormalArgument("i0"));
}
impl.template = template; // always forget the entire template; char indexes are relative to it
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:136:2: ( chunk )
// org/stringtemplate/v4/compiler/CodeGenerator.g:136:4: chunk
{
pushFollow(FOLLOW_chunk_in_template71);
chunk();
state._fsp--;
// finish off the CompiledST result
if ( ((template_scope)template_stack.peek()).state.stringtable!=null ) impl.strings = ((template_scope)template_stack.peek()).state.stringtable.toArray();
impl.codeSize = ((template_scope)template_stack.peek()).state.ip;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
template_stack.pop();
}
return impl;
}
// $ANTLR end "template"
// $ANTLR start "chunk"
// org/stringtemplate/v4/compiler/CodeGenerator.g:143:1: chunk : ( element )* ;
public final void chunk() throws RecognitionException {
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:144:2: ( ( element )* )
// org/stringtemplate/v4/compiler/CodeGenerator.g:144:4: ( element )*
{
// org/stringtemplate/v4/compiler/CodeGenerator.g:144:4: ( element )*
loop1:
do {
int alt1=2;
switch ( input.LA(1) ) {
case IF:
case TEXT:
case NEWLINE:
case EXPR:
case REGION:
case INDENTED_EXPR:
{
alt1=1;
}
break;
}
switch (alt1) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:144:4: element
{
pushFollow(FOLLOW_element_in_chunk86);
element();
state._fsp--;
}
break;
default :
break loop1;
}
} while (true);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "chunk"
// $ANTLR start "element"
// org/stringtemplate/v4/compiler/CodeGenerator.g:147:1: element : ( ^( INDENTED_EXPR INDENT compoundElement[$INDENT] ) | compoundElement[null] | ^( INDENTED_EXPR INDENT singleElement ) | singleElement );
public final void element() throws RecognitionException {
CommonTree INDENT1=null;
CommonTree INDENT2=null;
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:148:2: ( ^( INDENTED_EXPR INDENT compoundElement[$INDENT] ) | compoundElement[null] | ^( INDENTED_EXPR INDENT singleElement ) | singleElement )
int alt2=4;
switch ( input.LA(1) ) {
case INDENTED_EXPR:
{
switch ( input.LA(2) ) {
case DOWN:
{
switch ( input.LA(3) ) {
case INDENT:
{
switch ( input.LA(4) ) {
case IF:
case REGION:
{
alt2=1;
}
break;
case TEXT:
case NEWLINE:
case EXPR:
{
alt2=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 2, 5, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 2, 4, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 2, 1, input);
throw nvae;
}
}
break;
case IF:
case REGION:
{
alt2=2;
}
break;
case TEXT:
case NEWLINE:
case EXPR:
{
alt2=4;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 2, 0, input);
throw nvae;
}
switch (alt2) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:148:4: ^( INDENTED_EXPR INDENT compoundElement[$INDENT] )
{
match(input,INDENTED_EXPR,FOLLOW_INDENTED_EXPR_in_element99);
match(input, Token.DOWN, null);
INDENT1=(CommonTree)match(input,INDENT,FOLLOW_INDENT_in_element101);
pushFollow(FOLLOW_compoundElement_in_element103);
compoundElement(INDENT1);
state._fsp--;
match(input, Token.UP, null);
}
break;
case 2 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:149:4: compoundElement[null]
{
pushFollow(FOLLOW_compoundElement_in_element111);
compoundElement(null);
state._fsp--;
}
break;
case 3 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:150:4: ^( INDENTED_EXPR INDENT singleElement )
{
match(input,INDENTED_EXPR,FOLLOW_INDENTED_EXPR_in_element118);
match(input, Token.DOWN, null);
INDENT2=(CommonTree)match(input,INDENT,FOLLOW_INDENT_in_element120);
((template_scope)template_stack.peek()).state.indent(INDENT2);
pushFollow(FOLLOW_singleElement_in_element124);
singleElement();
state._fsp--;
((template_scope)template_stack.peek()).state.emit(Bytecode.INSTR_DEDENT);
match(input, Token.UP, null);
}
break;
case 4 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:151:4: singleElement
{
pushFollow(FOLLOW_singleElement_in_element132);
singleElement();
state._fsp--;
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "element"
// $ANTLR start "singleElement"
// org/stringtemplate/v4/compiler/CodeGenerator.g:154:1: singleElement : ( exprElement | TEXT | NEWLINE );
public final void singleElement() throws RecognitionException {
CommonTree TEXT3=null;
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:155:2: ( exprElement | TEXT | NEWLINE )
int alt3=3;
switch ( input.LA(1) ) {
case EXPR:
{
alt3=1;
}
break;
case TEXT:
{
alt3=2;
}
break;
case NEWLINE:
{
alt3=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 3, 0, input);
throw nvae;
}
switch (alt3) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:155:4: exprElement
{
pushFollow(FOLLOW_exprElement_in_singleElement143);
exprElement();
state._fsp--;
}
break;
case 2 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:156:4: TEXT
{
TEXT3=(CommonTree)match(input,TEXT,FOLLOW_TEXT_in_singleElement148);
if ( (TEXT3!=null?TEXT3.getText():null).length()>0 ) {
emit1(TEXT3,Bytecode.INSTR_WRITE_STR, (TEXT3!=null?TEXT3.getText():null));
}
}
break;
case 3 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:163:4: NEWLINE
{
match(input,NEWLINE,FOLLOW_NEWLINE_in_singleElement158);
emit(Bytecode.INSTR_NEWLINE);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "singleElement"
// $ANTLR start "compoundElement"
// org/stringtemplate/v4/compiler/CodeGenerator.g:166:1: compoundElement[CommonTree indent] : ( ifstat[indent] | region[indent] );
public final void compoundElement(CommonTree indent) throws RecognitionException {
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:167:2: ( ifstat[indent] | region[indent] )
int alt4=2;
switch ( input.LA(1) ) {
case IF:
{
alt4=1;
}
break;
case REGION:
{
alt4=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 4, 0, input);
throw nvae;
}
switch (alt4) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:167:4: ifstat[indent]
{
pushFollow(FOLLOW_ifstat_in_compoundElement172);
ifstat(indent);
state._fsp--;
}
break;
case 2 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:168:4: region[indent]
{
pushFollow(FOLLOW_region_in_compoundElement178);
region(indent);
state._fsp--;
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "compoundElement"
// $ANTLR start "exprElement"
// org/stringtemplate/v4/compiler/CodeGenerator.g:171:1: exprElement : ^( EXPR expr ( exprOptions )? ) ;
public final void exprElement() throws RecognitionException {
CommonTree EXPR4=null;
short op = Bytecode.INSTR_WRITE;
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:173:2: ( ^( EXPR expr ( exprOptions )? ) )
// org/stringtemplate/v4/compiler/CodeGenerator.g:173:4: ^( EXPR expr ( exprOptions )? )
{
EXPR4=(CommonTree)match(input,EXPR,FOLLOW_EXPR_in_exprElement197);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expr_in_exprElement199);
expr();
state._fsp--;
// org/stringtemplate/v4/compiler/CodeGenerator.g:173:17: ( exprOptions )?
int alt5=2;
switch ( input.LA(1) ) {
case OPTIONS:
{
alt5=1;
}
break;
}
switch (alt5) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:173:18: exprOptions
{
pushFollow(FOLLOW_exprOptions_in_exprElement202);
exprOptions();
state._fsp--;
op=Bytecode.INSTR_WRITE_OPT;
}
break;
}
match(input, Token.UP, null);
/*
CompilationState state = ((template_scope)template_stack.peek()).state;
CompiledST impl = state.impl;
if ( impl.instrs[state.ip-1] == Bytecode.INSTR_LOAD_LOCAL ) {
impl.instrs[state.ip-1] = Bytecode.INSTR_WRITE_LOCAL;
}
else {
emit(EXPR4, op);
}
*/
emit(EXPR4, op);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "exprElement"
public static class region_return extends TreeRuleReturnScope {
public String name;
};
// $ANTLR start "region"
// org/stringtemplate/v4/compiler/CodeGenerator.g:189:1: region[CommonTree indent] returns [String name] : ^( REGION ID template[$name,null] ) ;
public final CodeGenerator.region_return region(CommonTree indent) throws RecognitionException {
CodeGenerator.region_return retval = new CodeGenerator.region_return();
retval.start = input.LT(1);
CommonTree ID5=null;
CompiledST template6 = null;
if ( indent!=null ) ((template_scope)template_stack.peek()).state.indent(indent);
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:196:2: ( ^( REGION ID template[$name,null] ) )
// org/stringtemplate/v4/compiler/CodeGenerator.g:196:4: ^( REGION ID template[$name,null] )
{
match(input,REGION,FOLLOW_REGION_in_region240);
match(input, Token.DOWN, null);
ID5=(CommonTree)match(input,ID,FOLLOW_ID_in_region242);
retval.name = STGroup.getMangledRegionName(outermostTemplateName, (ID5!=null?ID5.getText():null));
pushFollow(FOLLOW_template_in_region252);
template6=template(retval.name, null);
state._fsp--;
CompiledST sub = template6;
sub.isRegion = true;
sub.regionDefType = ST.RegionType.EMBEDDED;
sub.templateDefStartToken = ID5.token;
//sub.dump();
outermostImpl.addImplicitlyDefinedTemplate(sub);
emit2(((CommonTree)retval.start), Bytecode.INSTR_NEW, retval.name, 0);
emit(((CommonTree)retval.start), Bytecode.INSTR_WRITE);
match(input, Token.UP, null);
}
if ( indent!=null ) ((template_scope)template_stack.peek()).state.emit(Bytecode.INSTR_DEDENT);
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "region"
public static class subtemplate_return extends TreeRuleReturnScope {
public String name;
public int nargs;
};
// $ANTLR start "subtemplate"
// org/stringtemplate/v4/compiler/CodeGenerator.g:212:1: subtemplate returns [String name, int nargs] : ^( SUBTEMPLATE ( ^( ARGS ( ID )+ ) )* template[$name,args] ) ;
public final CodeGenerator.subtemplate_return subtemplate() throws RecognitionException {
CodeGenerator.subtemplate_return retval = new CodeGenerator.subtemplate_return();
retval.start = input.LT(1);
CommonTree ID7=null;
CommonTree SUBTEMPLATE9=null;
CompiledST template8 = null;
retval.name = Compiler.getNewSubtemplateName();
List args = new ArrayList();
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:217:2: ( ^( SUBTEMPLATE ( ^( ARGS ( ID )+ ) )* template[$name,args] ) )
// org/stringtemplate/v4/compiler/CodeGenerator.g:217:4: ^( SUBTEMPLATE ( ^( ARGS ( ID )+ ) )* template[$name,args] )
{
SUBTEMPLATE9=(CommonTree)match(input,SUBTEMPLATE,FOLLOW_SUBTEMPLATE_in_subtemplate285);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// org/stringtemplate/v4/compiler/CodeGenerator.g:218:4: ( ^( ARGS ( ID )+ ) )*
loop7:
do {
int alt7=2;
switch ( input.LA(1) ) {
case ARGS:
{
alt7=1;
}
break;
}
switch (alt7) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:218:5: ^( ARGS ( ID )+ )
{
match(input,ARGS,FOLLOW_ARGS_in_subtemplate292);
match(input, Token.DOWN, null);
// org/stringtemplate/v4/compiler/CodeGenerator.g:218:12: ( ID )+
int cnt6=0;
loop6:
do {
int alt6=2;
switch ( input.LA(1) ) {
case ID:
{
alt6=1;
}
break;
}
switch (alt6) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:218:13: ID
{
ID7=(CommonTree)match(input,ID,FOLLOW_ID_in_subtemplate295);
args.add(new FormalArgument((ID7!=null?ID7.getText():null)));
}
break;
default :
if ( cnt6 >= 1 ) break loop6;
EarlyExitException eee =
new EarlyExitException(6, input);
throw eee;
}
cnt6++;
} while (true);
match(input, Token.UP, null);
}
break;
default :
break loop7;
}
} while (true);
retval.nargs = args.size();
pushFollow(FOLLOW_template_in_subtemplate312);
template8=template(retval.name, args);
state._fsp--;
CompiledST sub = template8;
sub.isAnonSubtemplate = true;
sub.templateDefStartToken = SUBTEMPLATE9.token;
sub.ast = SUBTEMPLATE9;
sub.ast.setUnknownTokenBoundaries();
sub.tokens = input.getTokenStream();
//sub.dump();
outermostImpl.addImplicitlyDefinedTemplate(sub);
match(input, Token.UP, null);
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "subtemplate"
// $ANTLR start "ifstat"
// org/stringtemplate/v4/compiler/CodeGenerator.g:234:1: ifstat[CommonTree indent] : ^(i= 'if' conditional chunk ( ^(eif= 'elseif' ec= conditional chunk ) )* ( ^(el= 'else' chunk ) )? ) ;
public final void ifstat(CommonTree indent) throws RecognitionException {
CommonTree i=null;
CommonTree eif=null;
CommonTree el=null;
CodeGenerator.conditional_return ec = null;
/** Tracks address of branch operand (in code block). It's how
* we backpatch forward references when generating code for IFs.
*/
int prevBranchOperand = -1;
/** Branch instruction operands that are forward refs to end of IF.
* We need to update them once we see the endif.
*/
List endRefs = new ArrayList();
if ( indent!=null ) ((template_scope)template_stack.peek()).state.indent(indent);
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:249:2: ( ^(i= 'if' conditional chunk ( ^(eif= 'elseif' ec= conditional chunk ) )* ( ^(el= 'else' chunk ) )? ) )
// org/stringtemplate/v4/compiler/CodeGenerator.g:249:4: ^(i= 'if' conditional chunk ( ^(eif= 'elseif' ec= conditional chunk ) )* ( ^(el= 'else' chunk ) )? )
{
i=(CommonTree)match(input,IF,FOLLOW_IF_in_ifstat349);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_conditional_in_ifstat351);
conditional();
state._fsp--;
prevBranchOperand = address()+1;
emit1(i,Bytecode.INSTR_BRF, -1); // write placeholder as branch target
pushFollow(FOLLOW_chunk_in_ifstat361);
chunk();
state._fsp--;
// org/stringtemplate/v4/compiler/CodeGenerator.g:255:4: ( ^(eif= 'elseif' ec= conditional chunk ) )*
loop8:
do {
int alt8=2;
switch ( input.LA(1) ) {
case ELSEIF:
{
alt8=1;
}
break;
}
switch (alt8) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:255:6: ^(eif= 'elseif' ec= conditional chunk )
{
eif=(CommonTree)match(input,ELSEIF,FOLLOW_ELSEIF_in_ifstat371);
endRefs.add(address()+1);
emit1(eif,Bytecode.INSTR_BR, -1); // br end
// update previous branch instruction
write(prevBranchOperand, (short)address());
prevBranchOperand = -1;
match(input, Token.DOWN, null);
pushFollow(FOLLOW_conditional_in_ifstat385);
ec=conditional();
state._fsp--;
prevBranchOperand = address()+1;
// write placeholder as branch target
emit1((ec!=null?((CommonTree)ec.start):null), Bytecode.INSTR_BRF, -1);
pushFollow(FOLLOW_chunk_in_ifstat397);
chunk();
state._fsp--;
match(input, Token.UP, null);
}
break;
default :
break loop8;
}
} while (true);
// org/stringtemplate/v4/compiler/CodeGenerator.g:272:4: ( ^(el= 'else' chunk ) )?
int alt9=2;
switch ( input.LA(1) ) {
case ELSE:
{
alt9=1;
}
break;
}
switch (alt9) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:272:6: ^(el= 'else' chunk )
{
el=(CommonTree)match(input,ELSE,FOLLOW_ELSE_in_ifstat420);
endRefs.add(address()+1);
emit1(el, Bytecode.INSTR_BR, -1); // br end
// update previous branch instruction
write(prevBranchOperand, (short)address());
prevBranchOperand = -1;
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
pushFollow(FOLLOW_chunk_in_ifstat434);
chunk();
state._fsp--;
match(input, Token.UP, null);
}
}
break;
}
match(input, Token.UP, null);
if ( prevBranchOperand>=0 ) {
write(prevBranchOperand, (short)address());
}
for (int opnd : endRefs) write(opnd, (short)address());
}
if ( indent!=null ) ((template_scope)template_stack.peek()).state.emit(Bytecode.INSTR_DEDENT);
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "ifstat"
public static class conditional_return extends TreeRuleReturnScope {
};
// $ANTLR start "conditional"
// org/stringtemplate/v4/compiler/CodeGenerator.g:292:1: conditional : ( ^( '||' conditional conditional ) | ^( '&&' conditional conditional ) | ^( '!' conditional ) | expr );
public final CodeGenerator.conditional_return conditional() throws RecognitionException {
CodeGenerator.conditional_return retval = new CodeGenerator.conditional_return();
retval.start = input.LT(1);
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:293:2: ( ^( '||' conditional conditional ) | ^( '&&' conditional conditional ) | ^( '!' conditional ) | expr )
int alt10=4;
switch ( input.LA(1) ) {
case OR:
{
alt10=1;
}
break;
case AND:
{
alt10=2;
}
break;
case BANG:
{
alt10=3;
}
break;
case ID:
case STRING:
case TRUE:
case FALSE:
case PROP:
case PROP_IND:
case INCLUDE:
case INCLUDE_IND:
case EXEC_FUNC:
case INCLUDE_SUPER:
case INCLUDE_SUPER_REGION:
case INCLUDE_REGION:
case TO_STR:
case LIST:
case MAP:
case ZIP:
case SUBTEMPLATE:
{
alt10=4;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 10, 0, input);
throw nvae;
}
switch (alt10) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:293:4: ^( '||' conditional conditional )
{
match(input,OR,FOLLOW_OR_in_conditional468);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_conditional_in_conditional470);
conditional();
state._fsp--;
pushFollow(FOLLOW_conditional_in_conditional472);
conditional();
state._fsp--;
match(input, Token.UP, null);
emit(Bytecode.INSTR_OR);
}
break;
case 2 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:294:4: ^( '&&' conditional conditional )
{
match(input,AND,FOLLOW_AND_in_conditional482);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_conditional_in_conditional484);
conditional();
state._fsp--;
pushFollow(FOLLOW_conditional_in_conditional486);
conditional();
state._fsp--;
match(input, Token.UP, null);
emit(Bytecode.INSTR_AND);
}
break;
case 3 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:295:4: ^( '!' conditional )
{
match(input,BANG,FOLLOW_BANG_in_conditional496);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_conditional_in_conditional498);
conditional();
state._fsp--;
match(input, Token.UP, null);
emit(Bytecode.INSTR_NOT);
}
break;
case 4 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:296:4: expr
{
pushFollow(FOLLOW_expr_in_conditional510);
expr();
state._fsp--;
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "conditional"
// $ANTLR start "exprOptions"
// org/stringtemplate/v4/compiler/CodeGenerator.g:299:1: exprOptions : ^( OPTIONS ( option )* ) ;
public final void exprOptions() throws RecognitionException {
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:299:13: ( ^( OPTIONS ( option )* ) )
// org/stringtemplate/v4/compiler/CodeGenerator.g:299:15: ^( OPTIONS ( option )* )
{
emit(Bytecode.INSTR_OPTIONS);
match(input,OPTIONS,FOLLOW_OPTIONS_in_exprOptions524);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// org/stringtemplate/v4/compiler/CodeGenerator.g:299:57: ( option )*
loop11:
do {
int alt11=2;
switch ( input.LA(1) ) {
case EQUALS:
{
alt11=1;
}
break;
}
switch (alt11) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:299:57: option
{
pushFollow(FOLLOW_option_in_exprOptions526);
option();
state._fsp--;
}
break;
default :
break loop11;
}
} while (true);
match(input, Token.UP, null);
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "exprOptions"
// $ANTLR start "option"
// org/stringtemplate/v4/compiler/CodeGenerator.g:301:1: option : ^( '=' ID expr ) ;
public final void option() throws RecognitionException {
CommonTree ID10=null;
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:301:8: ( ^( '=' ID expr ) )
// org/stringtemplate/v4/compiler/CodeGenerator.g:301:10: ^( '=' ID expr )
{
match(input,EQUALS,FOLLOW_EQUALS_in_option538);
match(input, Token.DOWN, null);
ID10=(CommonTree)match(input,ID,FOLLOW_ID_in_option540);
pushFollow(FOLLOW_expr_in_option542);
expr();
state._fsp--;
match(input, Token.UP, null);
setOption(ID10);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "option"
// $ANTLR start "expr"
// org/stringtemplate/v4/compiler/CodeGenerator.g:303:1: expr : ( ^( ZIP ^( ELEMENTS ( expr )+ ) mapTemplateRef[ne] ) | ^( MAP expr ( mapTemplateRef[1] )+ ) | prop | includeExpr );
public final void expr() throws RecognitionException {
CommonTree ZIP11=null;
CommonTree MAP12=null;
int nt = 0, ne = 0;
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:305:2: ( ^( ZIP ^( ELEMENTS ( expr )+ ) mapTemplateRef[ne] ) | ^( MAP expr ( mapTemplateRef[1] )+ ) | prop | includeExpr )
int alt14=4;
switch ( input.LA(1) ) {
case ZIP:
{
alt14=1;
}
break;
case MAP:
{
alt14=2;
}
break;
case PROP:
case PROP_IND:
{
alt14=3;
}
break;
case ID:
case STRING:
case TRUE:
case FALSE:
case INCLUDE:
case INCLUDE_IND:
case EXEC_FUNC:
case INCLUDE_SUPER:
case INCLUDE_SUPER_REGION:
case INCLUDE_REGION:
case TO_STR:
case LIST:
case SUBTEMPLATE:
{
alt14=4;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 14, 0, input);
throw nvae;
}
switch (alt14) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:305:4: ^( ZIP ^( ELEMENTS ( expr )+ ) mapTemplateRef[ne] )
{
ZIP11=(CommonTree)match(input,ZIP,FOLLOW_ZIP_in_expr561);
match(input, Token.DOWN, null);
match(input,ELEMENTS,FOLLOW_ELEMENTS_in_expr564);
match(input, Token.DOWN, null);
// org/stringtemplate/v4/compiler/CodeGenerator.g:305:21: ( expr )+
int cnt12=0;
loop12:
do {
int alt12=2;
switch ( input.LA(1) ) {
case ID:
case STRING:
case TRUE:
case FALSE:
case PROP:
case PROP_IND:
case INCLUDE:
case INCLUDE_IND:
case EXEC_FUNC:
case INCLUDE_SUPER:
case INCLUDE_SUPER_REGION:
case INCLUDE_REGION:
case TO_STR:
case LIST:
case MAP:
case ZIP:
case SUBTEMPLATE:
{
alt12=1;
}
break;
}
switch (alt12) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:305:22: expr
{
pushFollow(FOLLOW_expr_in_expr567);
expr();
state._fsp--;
ne++;
}
break;
default :
if ( cnt12 >= 1 ) break loop12;
EarlyExitException eee =
new EarlyExitException(12, input);
throw eee;
}
cnt12++;
} while (true);
match(input, Token.UP, null);
pushFollow(FOLLOW_mapTemplateRef_in_expr574);
mapTemplateRef(ne);
state._fsp--;
match(input, Token.UP, null);
emit1(ZIP11, Bytecode.INSTR_ZIP_MAP, ne);
}
break;
case 2 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:307:4: ^( MAP expr ( mapTemplateRef[1] )+ )
{
MAP12=(CommonTree)match(input,MAP,FOLLOW_MAP_in_expr586);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expr_in_expr588);
expr();
state._fsp--;
// org/stringtemplate/v4/compiler/CodeGenerator.g:307:15: ( mapTemplateRef[1] )+
int cnt13=0;
loop13:
do {
int alt13=2;
switch ( input.LA(1) ) {
case INCLUDE:
case INCLUDE_IND:
case SUBTEMPLATE:
{
alt13=1;
}
break;
}
switch (alt13) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:307:16: mapTemplateRef[1]
{
pushFollow(FOLLOW_mapTemplateRef_in_expr591);
mapTemplateRef(1);
state._fsp--;
nt++;
}
break;
default :
if ( cnt13 >= 1 ) break loop13;
EarlyExitException eee =
new EarlyExitException(13, input);
throw eee;
}
cnt13++;
} while (true);
match(input, Token.UP, null);
if ( nt>1 ) emit1(MAP12, nt>1?Bytecode.INSTR_ROT_MAP:Bytecode.INSTR_MAP, nt);
else emit(MAP12, Bytecode.INSTR_MAP);
}
break;
case 3 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:312:4: prop
{
pushFollow(FOLLOW_prop_in_expr606);
prop();
state._fsp--;
}
break;
case 4 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:313:4: includeExpr
{
pushFollow(FOLLOW_includeExpr_in_expr611);
includeExpr();
state._fsp--;
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "expr"
// $ANTLR start "prop"
// org/stringtemplate/v4/compiler/CodeGenerator.g:316:1: prop : ( ^( PROP expr ID ) | ^( PROP_IND expr expr ) );
public final void prop() throws RecognitionException {
CommonTree PROP13=null;
CommonTree ID14=null;
CommonTree PROP_IND15=null;
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:316:5: ( ^( PROP expr ID ) | ^( PROP_IND expr expr ) )
int alt15=2;
switch ( input.LA(1) ) {
case PROP:
{
alt15=1;
}
break;
case PROP_IND:
{
alt15=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 15, 0, input);
throw nvae;
}
switch (alt15) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:316:7: ^( PROP expr ID )
{
PROP13=(CommonTree)match(input,PROP,FOLLOW_PROP_in_prop621);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expr_in_prop623);
expr();
state._fsp--;
ID14=(CommonTree)match(input,ID,FOLLOW_ID_in_prop625);
match(input, Token.UP, null);
emit1(PROP13, Bytecode.INSTR_LOAD_PROP, (ID14!=null?ID14.getText():null));
}
break;
case 2 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:317:4: ^( PROP_IND expr expr )
{
PROP_IND15=(CommonTree)match(input,PROP_IND,FOLLOW_PROP_IND_in_prop639);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expr_in_prop641);
expr();
state._fsp--;
pushFollow(FOLLOW_expr_in_prop643);
expr();
state._fsp--;
match(input, Token.UP, null);
emit(PROP_IND15, Bytecode.INSTR_LOAD_PROP_IND);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "prop"
public static class mapTemplateRef_return extends TreeRuleReturnScope {
};
// $ANTLR start "mapTemplateRef"
// org/stringtemplate/v4/compiler/CodeGenerator.g:320:1: mapTemplateRef[int num_exprs] : ( ^( INCLUDE ID args ) | subtemplate | ^( INCLUDE_IND expr args ) );
public final CodeGenerator.mapTemplateRef_return mapTemplateRef(int num_exprs) throws RecognitionException {
CodeGenerator.mapTemplateRef_return retval = new CodeGenerator.mapTemplateRef_return();
retval.start = input.LT(1);
CommonTree INCLUDE16=null;
CommonTree ID18=null;
CommonTree INCLUDE_IND20=null;
CodeGenerator.args_return args17 = null;
CodeGenerator.subtemplate_return subtemplate19 = null;
CodeGenerator.args_return args21 = null;
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:321:2: ( ^( INCLUDE ID args ) | subtemplate | ^( INCLUDE_IND expr args ) )
int alt16=3;
switch ( input.LA(1) ) {
case INCLUDE:
{
alt16=1;
}
break;
case SUBTEMPLATE:
{
alt16=2;
}
break;
case INCLUDE_IND:
{
alt16=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 16, 0, input);
throw nvae;
}
switch (alt16) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:321:4: ^( INCLUDE ID args )
{
INCLUDE16=(CommonTree)match(input,INCLUDE,FOLLOW_INCLUDE_in_mapTemplateRef663);
match(input, Token.DOWN, null);
ID18=(CommonTree)match(input,ID,FOLLOW_ID_in_mapTemplateRef665);
for (int i=1; i<=num_exprs; i++) emit(INCLUDE16,Bytecode.INSTR_NULL);
pushFollow(FOLLOW_args_in_mapTemplateRef675);
args17=args();
state._fsp--;
match(input, Token.UP, null);
if ( (args17!=null?args17.passThru:false) ) emit1(((CommonTree)retval.start), Bytecode.INSTR_PASSTHRU, (ID18!=null?ID18.getText():null));
if ( (args17!=null?args17.namedArgs:false) ) emit1(INCLUDE16, Bytecode.INSTR_NEW_BOX_ARGS, (ID18!=null?ID18.getText():null));
else emit2(INCLUDE16, Bytecode.INSTR_NEW, (ID18!=null?ID18.getText():null), (args17!=null?args17.n:0)+num_exprs);
}
break;
case 2 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:330:4: subtemplate
{
pushFollow(FOLLOW_subtemplate_in_mapTemplateRef688);
subtemplate19=subtemplate();
state._fsp--;
if ( (subtemplate19!=null?subtemplate19.nargs:0) != num_exprs ) {
errMgr.compileTimeError(ErrorType.ANON_ARGUMENT_MISMATCH,
templateToken, (subtemplate19!=null?((CommonTree)subtemplate19.start):null).token, (subtemplate19!=null?subtemplate19.nargs:0), num_exprs);
}
for (int i=1; i<=num_exprs; i++) emit((subtemplate19!=null?((CommonTree)subtemplate19.start):null),Bytecode.INSTR_NULL);
emit2((subtemplate19!=null?((CommonTree)subtemplate19.start):null), Bytecode.INSTR_NEW,
(subtemplate19!=null?subtemplate19.name:null),
num_exprs);
}
break;
case 3 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:342:4: ^( INCLUDE_IND expr args )
{
INCLUDE_IND20=(CommonTree)match(input,INCLUDE_IND,FOLLOW_INCLUDE_IND_in_mapTemplateRef700);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expr_in_mapTemplateRef702);
expr();
state._fsp--;
emit(INCLUDE_IND20,Bytecode.INSTR_TOSTR);
for (int i=1; i<=num_exprs; i++) emit(INCLUDE_IND20,Bytecode.INSTR_NULL);
pushFollow(FOLLOW_args_in_mapTemplateRef712);
args21=args();
state._fsp--;
emit1(INCLUDE_IND20, Bytecode.INSTR_NEW_IND, (args21!=null?args21.n:0)+num_exprs);
match(input, Token.UP, null);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "mapTemplateRef"
public static class includeExpr_return extends TreeRuleReturnScope {
};
// $ANTLR start "includeExpr"
// org/stringtemplate/v4/compiler/CodeGenerator.g:354:1: includeExpr : ( ^( EXEC_FUNC ID ( expr )? ) | ^( INCLUDE ID args ) | ^( INCLUDE_SUPER ID args ) | ^( INCLUDE_REGION ID ) | ^( INCLUDE_SUPER_REGION ID ) | primary );
public final CodeGenerator.includeExpr_return includeExpr() throws RecognitionException {
CodeGenerator.includeExpr_return retval = new CodeGenerator.includeExpr_return();
retval.start = input.LT(1);
CommonTree ID22=null;
CommonTree ID24=null;
CommonTree INCLUDE25=null;
CommonTree ID27=null;
CommonTree INCLUDE_SUPER28=null;
CommonTree ID29=null;
CommonTree INCLUDE_REGION30=null;
CommonTree ID31=null;
CommonTree INCLUDE_SUPER_REGION32=null;
CodeGenerator.args_return args23 = null;
CodeGenerator.args_return args26 = null;
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:355:2: ( ^( EXEC_FUNC ID ( expr )? ) | ^( INCLUDE ID args ) | ^( INCLUDE_SUPER ID args ) | ^( INCLUDE_REGION ID ) | ^( INCLUDE_SUPER_REGION ID ) | primary )
int alt18=6;
switch ( input.LA(1) ) {
case EXEC_FUNC:
{
alt18=1;
}
break;
case INCLUDE:
{
alt18=2;
}
break;
case INCLUDE_SUPER:
{
alt18=3;
}
break;
case INCLUDE_REGION:
{
alt18=4;
}
break;
case INCLUDE_SUPER_REGION:
{
alt18=5;
}
break;
case ID:
case STRING:
case TRUE:
case FALSE:
case INCLUDE_IND:
case TO_STR:
case LIST:
case SUBTEMPLATE:
{
alt18=6;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 18, 0, input);
throw nvae;
}
switch (alt18) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:355:4: ^( EXEC_FUNC ID ( expr )? )
{
match(input,EXEC_FUNC,FOLLOW_EXEC_FUNC_in_includeExpr734);
match(input, Token.DOWN, null);
ID22=(CommonTree)match(input,ID,FOLLOW_ID_in_includeExpr736);
// org/stringtemplate/v4/compiler/CodeGenerator.g:355:19: ( expr )?
int alt17=2;
switch ( input.LA(1) ) {
case ID:
case STRING:
case TRUE:
case FALSE:
case PROP:
case PROP_IND:
case INCLUDE:
case INCLUDE_IND:
case EXEC_FUNC:
case INCLUDE_SUPER:
case INCLUDE_SUPER_REGION:
case INCLUDE_REGION:
case TO_STR:
case LIST:
case MAP:
case ZIP:
case SUBTEMPLATE:
{
alt17=1;
}
break;
}
switch (alt17) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:355:19: expr
{
pushFollow(FOLLOW_expr_in_includeExpr738);
expr();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
func(ID22);
}
break;
case 2 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:356:4: ^( INCLUDE ID args )
{
INCLUDE25=(CommonTree)match(input,INCLUDE,FOLLOW_INCLUDE_in_includeExpr749);
match(input, Token.DOWN, null);
ID24=(CommonTree)match(input,ID,FOLLOW_ID_in_includeExpr751);
pushFollow(FOLLOW_args_in_includeExpr753);
args23=args();
state._fsp--;
match(input, Token.UP, null);
if ( (args23!=null?args23.passThru:false) ) emit1(((CommonTree)retval.start), Bytecode.INSTR_PASSTHRU, (ID24!=null?ID24.getText():null));
if ( (args23!=null?args23.namedArgs:false) ) emit1(INCLUDE25, Bytecode.INSTR_NEW_BOX_ARGS, (ID24!=null?ID24.getText():null));
else emit2(INCLUDE25, Bytecode.INSTR_NEW, (ID24!=null?ID24.getText():null), (args23!=null?args23.n:0));
}
break;
case 3 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:362:4: ^( INCLUDE_SUPER ID args )
{
INCLUDE_SUPER28=(CommonTree)match(input,INCLUDE_SUPER,FOLLOW_INCLUDE_SUPER_in_includeExpr764);
match(input, Token.DOWN, null);
ID27=(CommonTree)match(input,ID,FOLLOW_ID_in_includeExpr766);
pushFollow(FOLLOW_args_in_includeExpr768);
args26=args();
state._fsp--;
match(input, Token.UP, null);
if ( (args26!=null?args26.passThru:false) ) emit1(((CommonTree)retval.start), Bytecode.INSTR_PASSTHRU, (ID27!=null?ID27.getText():null));
if ( (args26!=null?args26.namedArgs:false) ) emit1(INCLUDE_SUPER28, Bytecode.INSTR_SUPER_NEW_BOX_ARGS, (ID27!=null?ID27.getText():null));
else emit2(INCLUDE_SUPER28, Bytecode.INSTR_SUPER_NEW, (ID27!=null?ID27.getText():null), (args26!=null?args26.n:0));
}
break;
case 4 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:368:4: ^( INCLUDE_REGION ID )
{
INCLUDE_REGION30=(CommonTree)match(input,INCLUDE_REGION,FOLLOW_INCLUDE_REGION_in_includeExpr779);
match(input, Token.DOWN, null);
ID29=(CommonTree)match(input,ID,FOLLOW_ID_in_includeExpr781);
match(input, Token.UP, null);
CompiledST impl =
Compiler.defineBlankRegion(outermostImpl, ID29.token);
//impl.dump();
emit2(INCLUDE_REGION30,Bytecode.INSTR_NEW,impl.name,0);
}
break;
case 5 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:374:4: ^( INCLUDE_SUPER_REGION ID )
{
INCLUDE_SUPER_REGION32=(CommonTree)match(input,INCLUDE_SUPER_REGION,FOLLOW_INCLUDE_SUPER_REGION_in_includeExpr791);
match(input, Token.DOWN, null);
ID31=(CommonTree)match(input,ID,FOLLOW_ID_in_includeExpr793);
match(input, Token.UP, null);
String mangled =
STGroup.getMangledRegionName(outermostImpl.name, (ID31!=null?ID31.getText():null));
emit2(INCLUDE_SUPER_REGION32,Bytecode.INSTR_SUPER_NEW,mangled,0);
}
break;
case 6 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:379:4: primary
{
pushFollow(FOLLOW_primary_in_includeExpr801);
primary();
state._fsp--;
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "includeExpr"
public static class primary_return extends TreeRuleReturnScope {
};
// $ANTLR start "primary"
// org/stringtemplate/v4/compiler/CodeGenerator.g:382:1: primary : ( ID | STRING | TRUE | FALSE | subtemplate | list | ^( INCLUDE_IND expr args ) | ^( TO_STR expr ) );
public final CodeGenerator.primary_return primary() throws RecognitionException {
CodeGenerator.primary_return retval = new CodeGenerator.primary_return();
retval.start = input.LT(1);
CommonTree ID33=null;
CommonTree STRING34=null;
CommonTree TRUE35=null;
CommonTree FALSE36=null;
CommonTree INCLUDE_IND38=null;
CommonTree TO_STR40=null;
CodeGenerator.subtemplate_return subtemplate37 = null;
CodeGenerator.args_return args39 = null;
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:383:2: ( ID | STRING | TRUE | FALSE | subtemplate | list | ^( INCLUDE_IND expr args ) | ^( TO_STR expr ) )
int alt19=8;
switch ( input.LA(1) ) {
case ID:
{
alt19=1;
}
break;
case STRING:
{
alt19=2;
}
break;
case TRUE:
{
alt19=3;
}
break;
case FALSE:
{
alt19=4;
}
break;
case SUBTEMPLATE:
{
alt19=5;
}
break;
case LIST:
{
alt19=6;
}
break;
case INCLUDE_IND:
{
alt19=7;
}
break;
case TO_STR:
{
alt19=8;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 19, 0, input);
throw nvae;
}
switch (alt19) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:383:4: ID
{
ID33=(CommonTree)match(input,ID,FOLLOW_ID_in_primary812);
refAttr(ID33);
}
break;
case 2 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:384:4: STRING
{
STRING34=(CommonTree)match(input,STRING,FOLLOW_STRING_in_primary822);
emit1(STRING34,Bytecode.INSTR_LOAD_STR, Misc.strip((STRING34!=null?STRING34.getText():null),1));
}
break;
case 3 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:385:4: TRUE
{
TRUE35=(CommonTree)match(input,TRUE,FOLLOW_TRUE_in_primary831);
emit(TRUE35, Bytecode.INSTR_TRUE);
}
break;
case 4 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:386:4: FALSE
{
FALSE36=(CommonTree)match(input,FALSE,FOLLOW_FALSE_in_primary840);
emit(FALSE36, Bytecode.INSTR_FALSE);
}
break;
case 5 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:387:4: subtemplate
{
pushFollow(FOLLOW_subtemplate_in_primary849);
subtemplate37=subtemplate();
state._fsp--;
emit2(((CommonTree)retval.start),Bytecode.INSTR_NEW, (subtemplate37!=null?subtemplate37.name:null), 0);
}
break;
case 6 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:389:4: list
{
pushFollow(FOLLOW_list_in_primary876);
list();
state._fsp--;
}
break;
case 7 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:390:4: ^( INCLUDE_IND expr args )
{
INCLUDE_IND38=(CommonTree)match(input,INCLUDE_IND,FOLLOW_INCLUDE_IND_in_primary883);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expr_in_primary888);
expr();
state._fsp--;
emit(INCLUDE_IND38, Bytecode.INSTR_TOSTR);
pushFollow(FOLLOW_args_in_primary897);
args39=args();
state._fsp--;
emit1(INCLUDE_IND38, Bytecode.INSTR_NEW_IND, (args39!=null?args39.n:0));
match(input, Token.UP, null);
}
break;
case 8 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:394:4: ^( TO_STR expr )
{
TO_STR40=(CommonTree)match(input,TO_STR,FOLLOW_TO_STR_in_primary917);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expr_in_primary919);
expr();
state._fsp--;
match(input, Token.UP, null);
emit(TO_STR40, Bytecode.INSTR_TOSTR);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "primary"
// $ANTLR start "arg"
// org/stringtemplate/v4/compiler/CodeGenerator.g:397:1: arg : expr ;
public final void arg() throws RecognitionException {
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:397:5: ( expr )
// org/stringtemplate/v4/compiler/CodeGenerator.g:397:7: expr
{
pushFollow(FOLLOW_expr_in_arg932);
expr();
state._fsp--;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "arg"
public static class args_return extends TreeRuleReturnScope {
public int n=0;
public boolean namedArgs=false;
public boolean passThru;
};
// $ANTLR start "args"
// org/stringtemplate/v4/compiler/CodeGenerator.g:399:1: args returns [int n=0, boolean namedArgs=false, boolean passThru] : ( ( arg )+ | ( ^(eq= '=' ID expr ) )+ ( '...' )? | '...' | );
public final CodeGenerator.args_return args() throws RecognitionException {
CodeGenerator.args_return retval = new CodeGenerator.args_return();
retval.start = input.LT(1);
CommonTree eq=null;
CommonTree ID41=null;
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:400:2: ( ( arg )+ | ( ^(eq= '=' ID expr ) )+ ( '...' )? | '...' | )
int alt23=4;
switch ( input.LA(1) ) {
case ID:
case STRING:
case TRUE:
case FALSE:
case PROP:
case PROP_IND:
case INCLUDE:
case INCLUDE_IND:
case EXEC_FUNC:
case INCLUDE_SUPER:
case INCLUDE_SUPER_REGION:
case INCLUDE_REGION:
case TO_STR:
case LIST:
case MAP:
case ZIP:
case SUBTEMPLATE:
{
alt23=1;
}
break;
case EQUALS:
{
alt23=2;
}
break;
case ELLIPSIS:
{
alt23=3;
}
break;
case UP:
{
alt23=4;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 23, 0, input);
throw nvae;
}
switch (alt23) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:400:4: ( arg )+
{
// org/stringtemplate/v4/compiler/CodeGenerator.g:400:4: ( arg )+
int cnt20=0;
loop20:
do {
int alt20=2;
switch ( input.LA(1) ) {
case ID:
case STRING:
case TRUE:
case FALSE:
case PROP:
case PROP_IND:
case INCLUDE:
case INCLUDE_IND:
case EXEC_FUNC:
case INCLUDE_SUPER:
case INCLUDE_SUPER_REGION:
case INCLUDE_REGION:
case TO_STR:
case LIST:
case MAP:
case ZIP:
case SUBTEMPLATE:
{
alt20=1;
}
break;
}
switch (alt20) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:400:6: arg
{
pushFollow(FOLLOW_arg_in_args948);
arg();
state._fsp--;
retval.n++;
}
break;
default :
if ( cnt20 >= 1 ) break loop20;
EarlyExitException eee =
new EarlyExitException(20, input);
throw eee;
}
cnt20++;
} while (true);
}
break;
case 2 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:401:4: ( ^(eq= '=' ID expr ) )+ ( '...' )?
{
emit(((CommonTree)retval.start), Bytecode.INSTR_ARGS); retval.namedArgs =true;
// org/stringtemplate/v4/compiler/CodeGenerator.g:402:3: ( ^(eq= '=' ID expr ) )+
int cnt21=0;
loop21:
do {
int alt21=2;
switch ( input.LA(1) ) {
case EQUALS:
{
alt21=1;
}
break;
}
switch (alt21) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:402:5: ^(eq= '=' ID expr )
{
eq=(CommonTree)match(input,EQUALS,FOLLOW_EQUALS_in_args967);
match(input, Token.DOWN, null);
ID41=(CommonTree)match(input,ID,FOLLOW_ID_in_args969);
pushFollow(FOLLOW_expr_in_args971);
expr();
state._fsp--;
match(input, Token.UP, null);
retval.n++; emit1(eq, Bytecode.INSTR_STORE_ARG, defineString((ID41!=null?ID41.getText():null)));
}
break;
default :
if ( cnt21 >= 1 ) break loop21;
EarlyExitException eee =
new EarlyExitException(21, input);
throw eee;
}
cnt21++;
} while (true);
// org/stringtemplate/v4/compiler/CodeGenerator.g:405:3: ( '...' )?
int alt22=2;
switch ( input.LA(1) ) {
case ELLIPSIS:
{
alt22=1;
}
break;
}
switch (alt22) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:405:5: '...'
{
match(input,ELLIPSIS,FOLLOW_ELLIPSIS_in_args988);
retval.passThru =true;
}
break;
}
}
break;
case 3 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:406:9: '...'
{
match(input,ELLIPSIS,FOLLOW_ELLIPSIS_in_args1003);
retval.passThru =true; emit(((CommonTree)retval.start), Bytecode.INSTR_ARGS); retval.namedArgs =true;
}
break;
case 4 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:408:3:
{
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "args"
// $ANTLR start "list"
// org/stringtemplate/v4/compiler/CodeGenerator.g:410:1: list : ^( LIST ( listElement )* ) ;
public final void list() throws RecognitionException {
CodeGenerator.listElement_return listElement42 = null;
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:410:5: ( ^( LIST ( listElement )* ) )
// org/stringtemplate/v4/compiler/CodeGenerator.g:410:7: ^( LIST ( listElement )* )
{
emit(Bytecode.INSTR_LIST);
match(input,LIST,FOLLOW_LIST_in_list1023);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// org/stringtemplate/v4/compiler/CodeGenerator.g:411:10: ( listElement )*
loop24:
do {
int alt24=2;
switch ( input.LA(1) ) {
case ID:
case STRING:
case TRUE:
case FALSE:
case PROP:
case PROP_IND:
case INCLUDE:
case INCLUDE_IND:
case EXEC_FUNC:
case INCLUDE_SUPER:
case INCLUDE_SUPER_REGION:
case INCLUDE_REGION:
case TO_STR:
case LIST:
case MAP:
case ZIP:
case SUBTEMPLATE:
case NULL:
{
alt24=1;
}
break;
}
switch (alt24) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:411:11: listElement
{
pushFollow(FOLLOW_listElement_in_list1026);
listElement42=listElement();
state._fsp--;
emit((listElement42!=null?((CommonTree)listElement42.start):null), Bytecode.INSTR_ADD);
}
break;
default :
break loop24;
}
} while (true);
match(input, Token.UP, null);
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "list"
public static class listElement_return extends TreeRuleReturnScope {
};
// $ANTLR start "listElement"
// org/stringtemplate/v4/compiler/CodeGenerator.g:414:1: listElement : ( expr | NULL );
public final CodeGenerator.listElement_return listElement() throws RecognitionException {
CodeGenerator.listElement_return retval = new CodeGenerator.listElement_return();
retval.start = input.LT(1);
CommonTree NULL43=null;
try {
// org/stringtemplate/v4/compiler/CodeGenerator.g:414:13: ( expr | NULL )
int alt25=2;
switch ( input.LA(1) ) {
case ID:
case STRING:
case TRUE:
case FALSE:
case PROP:
case PROP_IND:
case INCLUDE:
case INCLUDE_IND:
case EXEC_FUNC:
case INCLUDE_SUPER:
case INCLUDE_SUPER_REGION:
case INCLUDE_REGION:
case TO_STR:
case LIST:
case MAP:
case ZIP:
case SUBTEMPLATE:
{
alt25=1;
}
break;
case NULL:
{
alt25=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 25, 0, input);
throw nvae;
}
switch (alt25) {
case 1 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:414:15: expr
{
pushFollow(FOLLOW_expr_in_listElement1042);
expr();
state._fsp--;
}
break;
case 2 :
// org/stringtemplate/v4/compiler/CodeGenerator.g:414:22: NULL
{
NULL43=(CommonTree)match(input,NULL,FOLLOW_NULL_in_listElement1046);
emit(NULL43,Bytecode.INSTR_NULL);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "listElement"
// Delegated rules
public static final BitSet FOLLOW_template_in_templateAndEOF44 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_templateAndEOF47 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_chunk_in_template71 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_element_in_chunk86 = new BitSet(new long[]{0x0280004100400012L});
public static final BitSet FOLLOW_INDENTED_EXPR_in_element99 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_INDENT_in_element101 = new BitSet(new long[]{0x0080000000000010L});
public static final BitSet FOLLOW_compoundElement_in_element103 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_compoundElement_in_element111 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INDENTED_EXPR_in_element118 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_INDENT_in_element120 = new BitSet(new long[]{0x0280004100400018L});
public static final BitSet FOLLOW_singleElement_in_element124 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_singleElement_in_element132 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_exprElement_in_singleElement143 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TEXT_in_singleElement148 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_NEWLINE_in_singleElement158 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ifstat_in_compoundElement172 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_region_in_compoundElement178 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_EXPR_in_exprElement197 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expr_in_exprElement199 = new BitSet(new long[]{0x0000008000000008L});
public static final BitSet FOLLOW_exprOptions_in_exprElement202 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_REGION_in_region240 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_region242 = new BitSet(new long[]{0x0280004100400010L});
public static final BitSet FOLLOW_template_in_region252 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_SUBTEMPLATE_in_subtemplate285 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ARGS_in_subtemplate292 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_subtemplate295 = new BitSet(new long[]{0x0000000002000008L});
public static final BitSet FOLLOW_template_in_subtemplate312 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_IF_in_ifstat349 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_conditional_in_ifstat351 = new BitSet(new long[]{0x0280004100400010L});
public static final BitSet FOLLOW_chunk_in_ifstat361 = new BitSet(new long[]{0x0000000000000068L});
public static final BitSet FOLLOW_ELSEIF_in_ifstat371 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_conditional_in_ifstat385 = new BitSet(new long[]{0x0280004100400010L});
public static final BitSet FOLLOW_chunk_in_ifstat397 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_ELSE_in_ifstat420 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_chunk_in_ifstat434 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_OR_in_conditional468 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_conditional_in_conditional470 = new BitSet(new long[]{0x001FFF1866000400L});
public static final BitSet FOLLOW_conditional_in_conditional472 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_AND_in_conditional482 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_conditional_in_conditional484 = new BitSet(new long[]{0x001FFF1866000400L});
public static final BitSet FOLLOW_conditional_in_conditional486 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_BANG_in_conditional496 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_conditional_in_conditional498 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_expr_in_conditional510 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_OPTIONS_in_exprOptions524 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_option_in_exprOptions526 = new BitSet(new long[]{0x0000000000001008L});
public static final BitSet FOLLOW_EQUALS_in_option538 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_option540 = new BitSet(new long[]{0x001FFF1866000400L});
public static final BitSet FOLLOW_expr_in_option542 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_ZIP_in_expr561 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ELEMENTS_in_expr564 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expr_in_expr567 = new BitSet(new long[]{0x001FFF1866000408L});
public static final BitSet FOLLOW_mapTemplateRef_in_expr574 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_MAP_in_expr586 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expr_in_expr588 = new BitSet(new long[]{0x00100C0000000000L});
public static final BitSet FOLLOW_mapTemplateRef_in_expr591 = new BitSet(new long[]{0x00100C0000000008L});
public static final BitSet FOLLOW_prop_in_expr606 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_includeExpr_in_expr611 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PROP_in_prop621 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expr_in_prop623 = new BitSet(new long[]{0x0000000002000000L});
public static final BitSet FOLLOW_ID_in_prop625 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_PROP_IND_in_prop639 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expr_in_prop641 = new BitSet(new long[]{0x001FFF1866000400L});
public static final BitSet FOLLOW_expr_in_prop643 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_INCLUDE_in_mapTemplateRef663 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_mapTemplateRef665 = new BitSet(new long[]{0x001FFF1866001C08L});
public static final BitSet FOLLOW_args_in_mapTemplateRef675 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_subtemplate_in_mapTemplateRef688 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INCLUDE_IND_in_mapTemplateRef700 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expr_in_mapTemplateRef702 = new BitSet(new long[]{0x001FFF1866001C08L});
public static final BitSet FOLLOW_args_in_mapTemplateRef712 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_EXEC_FUNC_in_includeExpr734 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_includeExpr736 = new BitSet(new long[]{0x001FFF1866000408L});
public static final BitSet FOLLOW_expr_in_includeExpr738 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_INCLUDE_in_includeExpr749 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_includeExpr751 = new BitSet(new long[]{0x001FFF1866001C08L});
public static final BitSet FOLLOW_args_in_includeExpr753 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_INCLUDE_SUPER_in_includeExpr764 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_includeExpr766 = new BitSet(new long[]{0x001FFF1866001C08L});
public static final BitSet FOLLOW_args_in_includeExpr768 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_INCLUDE_REGION_in_includeExpr779 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_includeExpr781 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_INCLUDE_SUPER_REGION_in_includeExpr791 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_includeExpr793 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_primary_in_includeExpr801 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ID_in_primary812 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_STRING_in_primary822 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TRUE_in_primary831 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FALSE_in_primary840 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_subtemplate_in_primary849 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_list_in_primary876 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INCLUDE_IND_in_primary883 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expr_in_primary888 = new BitSet(new long[]{0x001FFF1866001C08L});
public static final BitSet FOLLOW_args_in_primary897 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_TO_STR_in_primary917 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expr_in_primary919 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_expr_in_arg932 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_arg_in_args948 = new BitSet(new long[]{0x001FFF1866000402L});
public static final BitSet FOLLOW_EQUALS_in_args967 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_args969 = new BitSet(new long[]{0x001FFF1866000400L});
public static final BitSet FOLLOW_expr_in_args971 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_ELLIPSIS_in_args988 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ELLIPSIS_in_args1003 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LIST_in_list1023 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_listElement_in_list1026 = new BitSet(new long[]{0x011FFF1866000408L});
public static final BitSet FOLLOW_expr_in_listElement1042 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_NULL_in_listElement1046 = new BitSet(new long[]{0x0000000000000002L});
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy