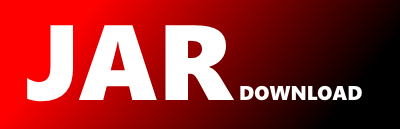
org.apache.iceberg.FastAppend Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iceberg-core Show documentation
Show all versions of iceberg-core Show documentation
A table format for huge analytic datasets
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.iceberg;
import java.io.IOException;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.iceberg.encryption.EncryptedOutputFile;
import org.apache.iceberg.events.CreateSnapshotEvent;
import org.apache.iceberg.exceptions.RuntimeIOException;
import org.apache.iceberg.io.InputFile;
import org.apache.iceberg.relocated.com.google.common.base.Preconditions;
import org.apache.iceberg.relocated.com.google.common.collect.Iterables;
import org.apache.iceberg.relocated.com.google.common.collect.Lists;
import org.apache.iceberg.util.DataFileSet;
/** {@link AppendFiles Append} implementation that adds a new manifest file for the write. */
class FastAppend extends SnapshotProducer implements AppendFiles {
private final String tableName;
private final TableOperations ops;
private final PartitionSpec spec;
private final SnapshotSummary.Builder summaryBuilder = SnapshotSummary.builder();
private final DataFileSet newFiles = DataFileSet.create();
private final List appendManifests = Lists.newArrayList();
private final List rewrittenAppendManifests = Lists.newArrayList();
private List newManifests = null;
private boolean hasNewFiles = false;
FastAppend(String tableName, TableOperations ops) {
super(ops);
this.tableName = tableName;
this.ops = ops;
this.spec = ops.current().spec();
}
@Override
protected AppendFiles self() {
return this;
}
@Override
public AppendFiles set(String property, String value) {
summaryBuilder.set(property, value);
return this;
}
@Override
protected String operation() {
return DataOperations.APPEND;
}
@Override
protected Map summary() {
summaryBuilder.setPartitionSummaryLimit(
ops.current()
.propertyAsInt(
TableProperties.WRITE_PARTITION_SUMMARY_LIMIT,
TableProperties.WRITE_PARTITION_SUMMARY_LIMIT_DEFAULT));
return summaryBuilder.build();
}
@Override
public FastAppend appendFile(DataFile file) {
Preconditions.checkNotNull(file, "Invalid data file: null");
if (newFiles.add(file)) {
this.hasNewFiles = true;
summaryBuilder.addedFile(spec, file);
}
return this;
}
@Override
public FastAppend toBranch(String branch) {
targetBranch(branch);
return this;
}
@Override
public FastAppend appendManifest(ManifestFile manifest) {
Preconditions.checkArgument(
!manifest.hasExistingFiles(), "Cannot append manifest with existing files");
Preconditions.checkArgument(
!manifest.hasDeletedFiles(), "Cannot append manifest with deleted files");
Preconditions.checkArgument(
manifest.snapshotId() == null || manifest.snapshotId() == -1,
"Snapshot id must be assigned during commit");
Preconditions.checkArgument(
manifest.sequenceNumber() == -1, "Sequence number must be assigned during commit");
if (canInheritSnapshotId() && manifest.snapshotId() == null) {
summaryBuilder.addedManifest(manifest);
appendManifests.add(manifest);
} else {
// the manifest must be rewritten with this update's snapshot ID
ManifestFile copiedManifest = copyManifest(manifest);
rewrittenAppendManifests.add(copiedManifest);
}
return this;
}
private ManifestFile copyManifest(ManifestFile manifest) {
TableMetadata current = ops.current();
InputFile toCopy = ops.io().newInputFile(manifest);
EncryptedOutputFile newManifestFile = newManifestOutputFile();
return ManifestFiles.copyAppendManifest(
current.formatVersion(),
manifest.partitionSpecId(),
toCopy,
current.specsById(),
newManifestFile,
snapshotId(),
summaryBuilder);
}
@Override
public List apply(TableMetadata base, Snapshot snapshot) {
List manifests = Lists.newArrayList();
try {
List newWrittenManifests = writeNewManifests();
if (newWrittenManifests != null) {
manifests.addAll(newWrittenManifests);
}
} catch (IOException e) {
throw new RuntimeIOException(e, "Failed to write manifest");
}
Iterable appendManifestsWithMetadata =
Iterables.transform(
Iterables.concat(appendManifests, rewrittenAppendManifests),
manifest -> GenericManifestFile.copyOf(manifest).withSnapshotId(snapshotId()).build());
Iterables.addAll(manifests, appendManifestsWithMetadata);
if (snapshot != null) {
manifests.addAll(snapshot.allManifests(ops.io()));
}
return manifests;
}
@Override
public Object updateEvent() {
long snapshotId = snapshotId();
Snapshot snapshot = ops.current().snapshot(snapshotId);
long sequenceNumber = snapshot.sequenceNumber();
return new CreateSnapshotEvent(
tableName, operation(), snapshotId, sequenceNumber, snapshot.summary());
}
@Override
protected void cleanUncommitted(Set committed) {
if (newManifests != null) {
boolean hasDeletes = false;
for (ManifestFile manifest : newManifests) {
if (!committed.contains(manifest)) {
deleteFile(manifest.path());
hasDeletes = true;
}
}
if (hasDeletes) {
this.newManifests = null;
}
}
// clean up only rewrittenAppendManifests as they are always owned by the table
// don't clean up appendManifests as they are added to the manifest list and are not compacted
for (ManifestFile manifest : rewrittenAppendManifests) {
if (!committed.contains(manifest)) {
deleteFile(manifest.path());
}
}
}
/**
* Cleanup after committing is disabled for FastAppend unless there are rewrittenAppendManifests
* because: 1.) Appended manifests are never rewritten 2.) Manifests which are written out as part
* of appendFile are already cleaned up between commit attempts in writeNewManifests
*/
@Override
protected boolean cleanupAfterCommit() {
return !rewrittenAppendManifests.isEmpty();
}
private List writeNewManifests() throws IOException {
if (hasNewFiles && newManifests != null) {
newManifests.forEach(file -> deleteFile(file.path()));
newManifests = null;
}
if (newManifests == null && !newFiles.isEmpty()) {
this.newManifests = writeDataManifests(newFiles, spec);
hasNewFiles = false;
}
return newManifests;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy