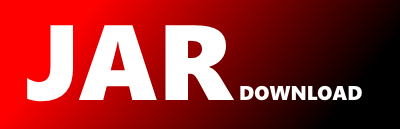
org.apache.ignite.cache.hibernate.HibernateRegionFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ignite-hibernate Show documentation
Show all versions of ignite-hibernate Show documentation
Java-based middleware for in-memory processing of big data in a distributed environment.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.ignite.cache.hibernate;
import org.apache.ignite.*;
import org.apache.ignite.cache.*;
import org.apache.ignite.internal.*;
import org.apache.ignite.internal.util.typedef.*;
import org.hibernate.cache.*;
import org.hibernate.cache.spi.*;
import org.hibernate.cache.spi.RegionFactory;
import org.hibernate.cache.spi.access.AccessType;
import org.hibernate.cfg.*;
import java.util.*;
import static org.hibernate.cache.spi.access.AccessType.*;
/**
* Hibernate L2 cache region factory.
*
* Following Hibernate settings should be specified to enable second level cache and to use this
* region factory for caching:
*
* hibernate.cache.use_second_level_cache=true
* hibernate.cache.region.factory_class=org.apache.ignite.cache.hibernate.HibernateRegionFactory
*
* Note that before region factory is started you need to start properly configured Ignite node in the same JVM.
* For example to start Ignite node one of loader provided in {@code org.apache.ignite.grid.startup} package can be used.
*
* Name of grid to be used for region factory must be specified as following Hibernate property:
*
* org.apache.ignite.hibernate.grid_name=<grid name>
*
* Each Hibernate cache region must be associated with some {@link GridCache}, by default it is assumed that
* for each cache region there is a {@link GridCache} with the same name. Also it is possible to define
* region to cache mapping using properties with prefix {@code org.apache.ignite.hibernate.region_cache}.
* For example if for region with name "region1" cache with name "cache1" should be used then following
* Hibernate property should be specified:
*
* org.apache.ignite.hibernate.region_cache.region1=cache1
*
*/
public class HibernateRegionFactory implements RegionFactory {
/** */
private static final long serialVersionUID = 0L;
/** Hibernate L2 cache grid name property name. */
public static final String GRID_NAME_PROPERTY = "org.apache.ignite.hibernate.grid_name";
/** Default cache property name. */
public static final String DFLT_CACHE_NAME_PROPERTY = "org.apache.ignite.hibernate.default_cache";
/** Property prefix used to specify region name to cache name mapping. */
public static final String REGION_CACHE_PROPERTY = "org.apache.ignite.hibernate.region_cache.";
/** */
public static final String DFLT_ACCESS_TYPE_PROPERTY = "org.apache.ignite.hibernate.default_access_type";
/** */
public static final String GRID_CONFIG_PROPERTY = "org.apache.ignite.hibernate.grid_config";
/** Grid providing caches. */
private Ignite ignite;
/** Default cache. */
private GridCache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy