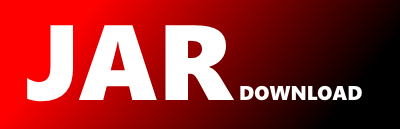
org.apache.ignite.ml.math.functions.Functions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ignite-ml Show documentation
Show all versions of ignite-ml Show documentation
Apache Ignite® is a Distributed Database For High-Performance Computing With In-Memory Speed.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.ignite.ml.math.functions;
import java.util.Comparator;
import java.util.List;
import java.util.function.BiFunction;
import org.apache.ignite.lang.IgniteBiTuple;
/**
* Compatibility with Apache Mahout.
*/
public final class Functions {
/** Function that returns {@code Math.abs(a)}. */
public static final IgniteDoubleFunction ABS = Math::abs;
/** Function that returns its argument. */
public static final IgniteDoubleFunction IDENTITY = (a) -> a;
/** Function that returns {@code Math.log(a) / Math.log(2)}. */
public static final IgniteDoubleFunction LOG2 = (a) -> Math.log(a) * 1.4426950408889634;
/** Function that returns {@code -a}. */
public static final IgniteDoubleFunction NEGATE = (a) -> -a;
/** Function that returns {@code a < 0 ? -1 : a > 0 ? 1 : 0 }. */
public static final IgniteDoubleFunction SIGN = (a) -> a < 0.0 ? -1.0 : a > 0.0 ? 1.0 : 0.0;
/** Function that returns {@code a * a}. */
public static final IgniteDoubleFunction SQUARE = (a) -> a * a;
/** Function that returns {@code 1 / (1 + exp(-a) } */
public static final IgniteDoubleFunction SIGMOID = (a) -> 1.0 / (1.0 + Math.exp(-a));
/** Function that returns {@code 1 / a } */
public static final IgniteDoubleFunction INV = (a) -> 1.0 / a;
/** Function that returns {@code a * (1-a) } */
public static final IgniteDoubleFunction SIGMOIDGRADIENT = (a) -> a * (1.0 - a);
/** Function that returns {@code a % b}. */
public static final IgniteBiFunction MOD = (a, b) -> a % b;
/** Function that returns {@code a * b}. */
public static final IgniteBiFunction MULT = (a, b) -> a * b;
/** Function that returns {@code Math.log(a) / Math.log(b)}. */
public static final IgniteBiFunction LG = (a, b) -> Math.log(a) / Math.log(b);
/** Function that returns {@code a + b}. */
public static final IgniteBiFunction PLUS = (a, b) -> a + b;
/** Function that returns {@code a - b}. */
public static final IgniteBiFunction MINUS = (a, b) -> a - b;
/** Function that returns {@code min(a, b)}. */
public static final IgniteBiFunction MIN = Math::min;
/** Function that returns {@code abs(a - b)}. */
public static final IgniteBiFunction MINUS_ABS = (a, b) -> Math.abs(a - b);
/** Function that returns {@code max(abs(a), abs(b))}. */
public static final IgniteBiFunction MAX_ABS = (a, b) -> Math.max(Math.abs(a), Math.abs(b));
/**
* Generic 'max' function.
*
* @param a First object to compare.
* @param b Second object to compare.
* @param f Comparator.
* @param Type of objects to compare.
* @return Maximum between {@code a} and {@code b} in terms of comparator {@code f}.
*/
public static T MAX_GENERIC(T a, T b, Comparator f) {
return f.compare(a, b) > 0 ? a : b;
}
/**
* Generic 'min' function.
*
* @param a First object to compare.
* @param b Second object to compare.
* @param f Comparator.
* @param Type of objects to compare.
* @return Minimum between {@code a} and {@code b} in terms of comparator {@code f}.
*/
public static T MIN_GENERIC(T a, T b, Comparator f) {
return f.compare(a, b) < 0 ? a : b;
}
/** Function that returns {@code min(abs(a), abs(b))}. */
public static final IgniteBiFunction MIN_ABS = (a, b) -> Math.min(Math.abs(a), Math.abs(b));
/** Function that returns {@code Math.abs(a) + Math.abs(b)}. */
public static final IgniteBiFunction PLUS_ABS = (a, b) -> Math.abs(a) + Math.abs(b);
/** Function that returns {@code (a - b) * (a - b)} */
public static final IgniteBiFunction MINUS_SQUARED = (a, b) -> (a - b) * (a - b);
/** Function that returns {@code a < b ? -1 : a > b ? 1 : 0}. */
public static final IgniteBiFunction COMPARE = (a, b) -> a < b ? -1.0 : a > b ? 1.0 : 0.0;
/** */
public static > IgniteBiTuple argmin(List args, IgniteFunction f) {
A res = null;
B fRes = null;
if (!args.isEmpty()) {
res = args.iterator().next();
fRes = f.apply(res);
}
int resInd = 0;
int i = 0;
for (A arg : args) {
B curRes = f.apply(arg);
if (fRes.compareTo(curRes) > 0) {
res = arg;
resInd = i;
fRes = curRes;
}
i++;
}
return new IgniteBiTuple<>(resInd, res);
}
/**
* Function that returns {@code a + b}. {@code a} is a variable, {@code b} is fixed.
*
* @param b Value to add.
* @return Function for this operation.
*/
public static IgniteDoubleFunction plus(final double b) {
return (a) -> a + b;
}
/**
* Function that returns {@code a * b}. {@code a} is a variable, {@code b} is fixed.
*
* @param b Value to multiply to.
* @return Function for this operation.
*/
public static IgniteDoubleFunction mult(final double b) {
return (a) -> a * b;
}
/**
* Function that returns {@code a / b}. {@code a} is a variable, {@code b} is fixed.
*
* @param b Value to divide to.
* @return Function for this operation.
*/
public static IgniteDoubleFunction div(double b) {
return mult(1 / b);
}
/**
* Function that returns {@code a + b*constant}. {@code a} and {@code b} are variables,
* {@code constant} is fixed.
*
* @param constant Value to use in multiply.
* @return Function for this operation.
*/
public static IgniteBiFunction plusMult(double constant) {
return (a, b) -> a + b * constant;
}
/**
* Function that returns {@code a - b*constant}. {@code a} and {@code b} are variables,
* {@code constant} is fixed.
*
* @param constant Value to use in multiply.
* @return Function for this operation.
*/
public static IgniteBiFunction minusMult(double constant) {
return (a, b) -> a - b * constant;
}
/** Function that returns passed constant. */
public static IgniteDoubleFunction constant(Double c) {
return a -> c;
}
/**
* Function that returns {@code Math.pow(a, b)}.
*
* @param b Power value.
* @return Function for given power.
*/
public static IgniteDoubleFunction pow(final double b) {
return (a) -> {
if (b == 2)
return a * a;
else
return Math.pow(a, b);
};
}
/**
* Curry bi-function.
*
* @param f Bi-function to curry.
* @param Type of first argument of {@code f}.
* @param Type of second argument of {@code f}.
* @param Return type of {@code f}.
* @return Curried bi-function.
*/
public static IgniteCurriedBiFunction curry(BiFunction f) {
return a -> b -> f.apply(a, b);
}
/**
* Transform bi-function of the form (a, b) -> c into a function of form a -> (b -> c).
*
* @param f Function to be curried.
* @param Type of first argument of function to be transformed.
* @param Type of second argument of function to be transformed.
* @param Type of third argument of function to be transformed.
* @return Curried bi-function.
*/
public static IgniteCurriedBiFunction curry(IgniteBiFunction f) {
return a -> b -> f.apply(a, b);
}
/**
* Transform tri-function of the form (a, b, c) -> d into a function of form a -> (b -> (c -> d)).
*
* @param f Function to be curried.
* @param Type of first argument of function to be transformed.
* @param Type of second argument of function to be transformed.
* @param Type of third argument of function to be transformed.
* @param Type output of function to be transformed.
* @return Curried tri-function.
*/
public static IgniteCurriedTriFunction curry(IgniteTriFunction f) {
return a -> b -> c -> f.apply(a, b, c);
}
/**
* Transform function of form a -> b into a -> (() -> b).
*
* @param f Function to be transformed.
* @param Type of input of function to be transformed.
* @param Type of output of function to be transformed.
* @return Transformed function.
*/
public static IgniteFunction> outputSupplier(IgniteFunction f) {
return a -> {
B res = f.apply(a);
return () -> res;
};
}
/**
* Transform function of form (a, b) -> c into (a, b) - () -> c.
*
* @param f Function to be transformed.
* @param Type of first argument of function to be transformed.
* @param Type of second argument of function to be transformed.
* @param Type of output of function to be transformed.
* @return Transformed function.
*/
public static IgniteBiFunction> outputSupplier(IgniteBiFunction f) {
return (a, b) -> {
C res = f.apply(a, b);
return () -> res;
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy