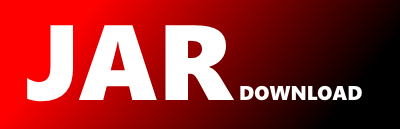
org.apache.ignite.IgniteClientSpringBean Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ignite-spring Show documentation
Show all versions of ignite-spring Show documentation
Java-based middleware for in-memory processing of big data in a distributed environment.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.ignite;
import java.util.Collection;
import java.util.List;
import org.apache.ignite.cache.query.FieldsQueryCursor;
import org.apache.ignite.cache.query.SqlFieldsQuery;
import org.apache.ignite.client.ClientCache;
import org.apache.ignite.client.ClientCacheConfiguration;
import org.apache.ignite.client.ClientCluster;
import org.apache.ignite.client.ClientClusterGroup;
import org.apache.ignite.client.ClientCompute;
import org.apache.ignite.client.ClientException;
import org.apache.ignite.client.ClientServices;
import org.apache.ignite.client.ClientTransactions;
import org.apache.ignite.client.IgniteClient;
import org.apache.ignite.client.IgniteClientFuture;
import org.apache.ignite.configuration.ClientConfiguration;
import org.springframework.beans.factory.DisposableBean;
import org.springframework.context.SmartLifecycle;
import org.springframework.context.event.ContextRefreshedEvent;
/**
* Represents Ignite client Spring bean that provides the ability to automatically start Ignite client during
* Spring Context initialization. It requires {@link ClientConfiguration} to be set before bean use
* (see {@link #setClientConfiguration(ClientConfiguration)}}).
*
* A note should be taken that Ignite client instance is started after all other
* Spring beans have been initialized and right before Spring context is refreshed.
* That implies that it's not valid to reference {@link IgniteClientSpringBean} from
* any kind of Spring bean init methods like {@link javax.annotation.PostConstruct}.
* If it's required to reference {@link IgniteClientSpringBean} for other bean
* initialization purposes, it should be done from a {@link ContextRefreshedEvent}
* listener method declared in that bean.
*
* Spring XML Configuration Example
*
* <bean id="igniteClient" class="org.apache.ignite.IgniteClientSpringBean">
* <property name="clientConfiguration">
* <bean class="org.apache.ignite.configuration.ClientConfiguration">
* <property name="addresses">
* <list>
* <value>127.0.0.1:10800</value>
* </list>
* </property>
* </bean>
* </property>
* </bean>
*
*/
public class IgniteClientSpringBean implements IgniteClient, SmartLifecycle {
/** Default Ignite client {@link SmartLifecycle} phase. */
public static final int DFLT_IGNITE_CLI_LIFECYCLE_PHASE = 0;
/** Whether this component is initialized and running. */
private volatile boolean isRunning;
/** Ignite client instance to which operations are delegated. */
private IgniteClient cli;
/** Ignite client configuration. */
private ClientConfiguration cfg;
/** Ignite client {@link SmartLifecycle} phase. */
private int phase = DFLT_IGNITE_CLI_LIFECYCLE_PHASE;
/** {@inheritDoc} */
@Override public boolean isAutoStartup() {
return true;
}
/** {@inheritDoc} */
@Override public void stop(Runnable callback) {
stop();
callback.run();
}
/** {@inheritDoc} */
@Override public void stop() {
isRunning = false;
}
/** {@inheritDoc} */
@Override public boolean isRunning() {
return isRunning;
}
/** {@inheritDoc} */
@Override public void start() {
if (cfg == null)
throw new IllegalArgumentException("Ignite client configuration must be set.");
cli = Ignition.startClient(cfg);
isRunning = true;
}
/** {@inheritDoc} */
@Override public int getPhase() {
return phase;
}
/** {@inheritDoc} */
@Override public ClientCache getOrCreateCache(String name) throws ClientException {
return cli.getOrCreateCache(name);
}
/** {@inheritDoc} */
@Override public IgniteClientFuture> getOrCreateCacheAsync(String name)
throws ClientException
{
return cli.getOrCreateCacheAsync(name);
}
/** {@inheritDoc} */
@Override public ClientCache getOrCreateCache(ClientCacheConfiguration cfg) throws ClientException {
return cli.getOrCreateCache(cfg);
}
/** {@inheritDoc} */
@Override public IgniteClientFuture> getOrCreateCacheAsync(ClientCacheConfiguration cfg)
throws ClientException
{
return cli.getOrCreateCacheAsync(cfg);
}
/** {@inheritDoc} */
@Override public ClientCache cache(String name) {
return cli.cache(name);
}
/** {@inheritDoc} */
@Override public Collection cacheNames() throws ClientException {
return cli.cacheNames();
}
/** {@inheritDoc} */
@Override public IgniteClientFuture> cacheNamesAsync() throws ClientException {
return cli.cacheNamesAsync();
}
/** {@inheritDoc} */
@Override public void destroyCache(String name) throws ClientException {
cli.destroyCache(name);
}
/** {@inheritDoc} */
@Override public IgniteClientFuture destroyCacheAsync(String name) throws ClientException {
return cli.destroyCacheAsync(name);
}
/** {@inheritDoc} */
@Override public ClientCache createCache(String name) throws ClientException {
return cli.createCache(name);
}
/** {@inheritDoc} */
@Override public IgniteClientFuture> createCacheAsync(String name) throws ClientException {
return cli.createCacheAsync(name);
}
/** {@inheritDoc} */
@Override public ClientCache createCache(ClientCacheConfiguration cfg) throws ClientException {
return cli.createCache(cfg);
}
/** {@inheritDoc} */
@Override public IgniteClientFuture> createCacheAsync(ClientCacheConfiguration cfg)
throws ClientException
{
return cli.createCacheAsync(cfg);
}
/** {@inheritDoc} */
@Override public IgniteBinary binary() {
return cli.binary();
}
/** {@inheritDoc} */
@Override public FieldsQueryCursor> query(SqlFieldsQuery qry) {
return cli.query(qry);
}
/** {@inheritDoc} */
@Override public ClientTransactions transactions() {
return cli.transactions();
}
/** {@inheritDoc} */
@Override public ClientCompute compute() {
return cli.compute();
}
/** {@inheritDoc} */
@Override public ClientCompute compute(ClientClusterGroup grp) {
return cli.compute(grp);
}
/** {@inheritDoc} */
@Override public ClientCluster cluster() {
return cli.cluster();
}
/** {@inheritDoc} */
@Override public ClientServices services() {
return cli.services();
}
/** {@inheritDoc} */
@Override public ClientServices services(ClientClusterGroup grp) {
return cli.services(grp);
}
/** {@inheritDoc} */
@Override public void close() throws Exception {
cli.close();
}
/** Sets Ignite client configuration. */
public IgniteClientSpringBean setClientConfiguration(ClientConfiguration cfg) {
this.cfg = cfg;
return this;
}
/** Gets Ignite client configuration. */
public ClientConfiguration getClientConfiguration() {
return cfg;
}
/**
* Sets {@link SmartLifecycle} phase during which the current bean will be initialized. Note, underlying Ignite
* client will be closed during handling of {@link DisposableBean} since {@link IgniteClient}
* implements {@link AutoCloseable} interface.
*/
public IgniteClientSpringBean setPhase(int phase) {
this.phase = phase;
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy