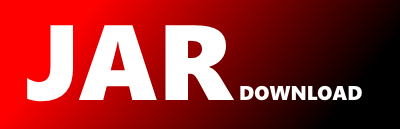
com.carrotsearch.hppc.FloatDeque Maven / Gradle / Ivy
The newest version!
package com.carrotsearch.hppc;
import java.util.Deque;
import java.util.Iterator;
import com.carrotsearch.hppc.cursors.FloatCursor;
import com.carrotsearch.hppc.predicates.FloatPredicate;
import com.carrotsearch.hppc.procedures.FloatProcedure;
/**
* A linear collection that supports element insertion and removal at both ends.
*
* @see Deque
*/
@com.carrotsearch.hppc.Generated(
date = "2018-05-21T12:24:05+0200",
value = "KTypeDeque.java")
public interface FloatDeque extends FloatCollection {
/**
* Removes the first element that equals e
.
*
* @return The deleted element's index or -1
if the element
* was not found.
*/
public int removeFirst(float e);
/**
* Removes the last element that equals e
.
*
* @return The deleted element's index or -1
if the element
* was not found.
*/
public int removeLast(float e);
/**
* Inserts the specified element at the front of this deque.
*/
public void addFirst(float e);
/**
* Inserts the specified element at the end of this deque.
*/
public void addLast(float e);
/**
* Retrieves and removes the first element of this deque.
*
* @return the head (first) element of this deque.
*/
public float removeFirst();
/**
* Retrieves and removes the last element of this deque.
*
* @return the tail of this deque.
*/
public float removeLast();
/**
* Retrieves the first element of this deque but does not remove it.
*
* @return the head of this deque.
*/
public float getFirst();
/**
* Retrieves the last element of this deque but does not remove it.
*
* @return the head of this deque.
*/
public float getLast();
/**
* @return An iterator over elements in this deque in tail-to-head order.
*/
public Iterator descendingIterator();
/**
* Applies a procedure
to all elements in tail-to-head order.
*/
public T descendingForEach(T procedure);
/**
* Applies a predicate
to container elements as long, as the
* predicate returns true
. The iteration is interrupted
* otherwise.
*/
public T descendingForEach(T predicate);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy