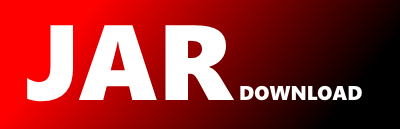
org.mapdb.Serializer Maven / Gradle / Ivy
/*
* Copyright (c) 2012 Jan Kotek
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.mapdb;
import java.io.*;
import java.nio.charset.Charset;
import java.util.*;
/**
* Provides serialization and deserialization
*
* @author Jan Kotek
*/
public interface Serializer {
/**
* Serialize the content of an object into a ObjectOutput
*
* @param out ObjectOutput to save object into
* @param value Object to serialize
*/
public void serialize( DataOutput out, A value)
throws IOException;
/**
* Deserialize the content of an object from a DataInput.
*
* @param in to read serialized data from
* @param available how many bytes are available in DataInput for reading, may be -1 (in streams) or 0 (null).
* @return deserialized object
* @throws java.io.IOException
*/
public A deserialize( DataInput in, int available)
throws IOException;
/**
* Data could be serialized into record with variable size or fixed size.
* Some optimizations can be applied to serializers with fixed size
*
* @return fixed size or -1 for variable size
*/
public int fixedSize();
/**
* Serializes strings using UTF8 encoding.
* Stores string size so can be used as collection serializer.
* Does not handle null values
*/
Serializer STRING = new Serializer() {
@Override
public void serialize(DataOutput out, String value) throws IOException {
out.writeUTF(value);
}
@Override
public String deserialize(DataInput in, int available) throws IOException {
return in.readUTF();
}
@Override
public int fixedSize() {
return -1;
}
};
/**
* Serializes strings using UTF8 encoding.
* Deserialized String is interned {@link String#intern()},
* so it could save some memory.
*
* Stores string size so can be used as collection serializer.
* Does not handle null values
*/
Serializer STRING_INTERN = new Serializer() {
@Override
public void serialize(DataOutput out, String value) throws IOException {
out.writeUTF(value);
}
@Override
public String deserialize(DataInput in, int available) throws IOException {
return in.readUTF().intern();
}
@Override
public int fixedSize() {
return -1;
}
};
/**
* Serializes strings using ASCII encoding (8 bit character).
* Is faster compared to UTF8 encoding.
* Stores string size so can be used as collection serializer.
* Does not handle null values
*/
Serializer STRING_ASCII = new Serializer() {
@Override
public void serialize(DataOutput out, String value) throws IOException {
char[] cc = new char[value.length()];
value.getChars(0,cc.length,cc,0);
DataOutput2.packInt(out,cc.length);
for(char c:cc){
out.write(c);
}
}
@Override
public String deserialize(DataInput in, int available) throws IOException {
int size = DataInput2.unpackInt(in);
char[] cc = new char[size];
for(int i=0;i STRING_NOSIZE = new Serializer() {
private final Charset UTF8_CHARSET = Charset.forName("UTF8");
@Override
public void serialize(DataOutput out, String value) throws IOException {
final byte[] bytes = value.getBytes(UTF8_CHARSET);
out.write(bytes);
}
@Override
public String deserialize(DataInput in, int available) throws IOException {
if(available==-1) throw new IllegalArgumentException("STRING_NOSIZE does not work with collections.");
byte[] bytes = new byte[available];
in.readFully(bytes);
return new String(bytes, UTF8_CHARSET);
}
@Override
public int fixedSize() {
return -1;
}
};
/** Serializes Long into 8 bytes, used mainly for testing.
* Does not handle null values.*/
Serializer LONG = new Serializer() {
@Override
public void serialize(DataOutput out, Long value) throws IOException {
if(value != null)
out.writeLong(value);
}
@Override
public Long deserialize(DataInput in, int available) throws IOException {
if(available==0) return null;
return in.readLong();
}
@Override
public int fixedSize() {
return 8;
}
};
/** Serializes Integer into 4 bytes, used mainly for testing.
* Does not handle null values.*/
Serializer INTEGER = new Serializer() {
@Override
public void serialize(DataOutput out, Integer value) throws IOException {
out.writeInt(value);
}
@Override
public Integer deserialize(DataInput in, int available) throws IOException {
return in.readInt();
}
@Override
public int fixedSize() {
return 4;
}
};
Serializer BOOLEAN = new Serializer() {
@Override
public void serialize(DataOutput out, Boolean value) throws IOException {
out.writeBoolean(value);
}
@Override
public Boolean deserialize(DataInput in, int available) throws IOException {
if(available==0) return null;
return in.readBoolean();
}
@Override
public int fixedSize() {
return 1;
}
};
/**
* Always throws {@link IllegalAccessError} when invoked. Useful for testing and assertions.
*/
Serializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy