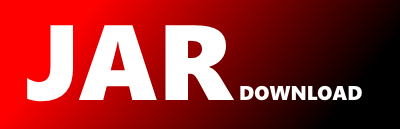
iotdb.thrift.cluster.TSDataService.py Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotdb-thrift-cluster
Show all versions of iotdb-thrift-cluster
RPC (Thrift) framework among servers.
#
# Autogenerated by Thrift Compiler (0.14.1)
#
# DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
#
# options string: py
#
from thrift.Thrift import TType, TMessageType, TFrozenDict, TException, TApplicationException
from thrift.protocol.TProtocol import TProtocolException
from thrift.TRecursive import fix_spec
import sys
import iotdb.thrift.cluster.RaftService
import logging
from .ttypes import *
from thrift.Thrift import TProcessor
from thrift.transport import TTransport
all_structs = []
class Iface(iotdb.thrift.cluster.RaftService.Iface):
def querySingleSeries(self, request):
"""
Query a time series without value filter.
@return a readerId >= 0 if the query succeeds, otherwise the query fails
TODO-Cluster: support query multiple series in a request
Parameters:
- request
"""
pass
def queryMultSeries(self, request):
"""
Query mult time series without value filter.
@return a readerId >= 0 if the query succeeds, otherwise the query fails
Parameters:
- request
"""
pass
def fetchSingleSeries(self, header, readerId):
"""
Fetch at max fetchSize time-value pairs using the resultSetId generated by querySingleSeries.
@return a ByteBuffer containing the serialized time-value pairs or an empty buffer if there
are not more results.
Parameters:
- header
- readerId
"""
pass
def fetchMultSeries(self, header, readerId, paths):
"""
Fetch mult series at max fetchSize time-value pairs using the resultSetId generated by querySingleSeries.
@return a map containing key-value,the serialized time-value pairs or an empty buffer if there
are not more results.
Parameters:
- header
- readerId
- paths
"""
pass
def querySingleSeriesByTimestamp(self, request):
"""
Query a time series and generate an IReaderByTimestamp.
@return a readerId >= 0 if the query succeeds, otherwise the query fails
Parameters:
- request
"""
pass
def fetchSingleSeriesByTimestamps(self, header, readerId, timestamps):
"""
Fetch values at given timestamps using the resultSetId generated by
querySingleSeriesByTimestamp.
@return a ByteBuffer containing the serialized value or an empty buffer if there
are not more results.
Parameters:
- header
- readerId
- timestamps
"""
pass
def endQuery(self, header, thisNode, queryId):
"""
Find the local query established for the remote query and release all its resource.
Parameters:
- header
- thisNode
- queryId
"""
pass
def getAllPaths(self, header, paths, withAlias):
"""
Given path patterns (paths with wildcard), return all paths they match.
Parameters:
- header
- paths
- withAlias
"""
pass
def getAllDevices(self, header, path, isPrefixMatch):
"""
Given path patterns (paths with wildcard), return all devices they match.
Parameters:
- header
- path
- isPrefixMatch
"""
pass
def getDevices(self, header, planBinary):
"""
Get the devices from the header according to the showDevicesPlan
Parameters:
- header
- planBinary
"""
pass
def getNodeList(self, header, path, nodeLevel):
"""
Parameters:
- header
- path
- nodeLevel
"""
pass
def getChildNodeInNextLevel(self, header, path):
"""
Given path patterns(paths with wildcard), return all children nodes they match
Parameters:
- header
- path
"""
pass
def getChildNodePathInNextLevel(self, header, path):
"""
Parameters:
- header
- path
"""
pass
def getAllMeasurementSchema(self, request):
"""
Parameters:
- request
"""
pass
def getAggrResult(self, request):
"""
Parameters:
- request
"""
pass
def getUnregisteredTimeseries(self, header, timeseriesList):
"""
Parameters:
- header
- timeseriesList
"""
pass
def pullSnapshot(self, request):
"""
Parameters:
- request
"""
pass
def getGroupByExecutor(self, request):
"""
Create a GroupByExecutor for a path, executing the given aggregations.
@return the executorId
Parameters:
- request
"""
pass
def getGroupByResult(self, header, executorId, startTime, endTime):
"""
Fetch the group by result in the interval [startTime, endTime) from the given executor.
@return the serialized AggregationResults, each is the result of one of the previously
required aggregations, and their orders are the same.
Parameters:
- header
- executorId
- startTime
- endTime
"""
pass
def pullTimeSeriesSchema(self, request):
"""
Pull all timeseries schemas prefixed by a given path.
Parameters:
- request
"""
pass
def pullMeasurementSchema(self, request):
"""
Pull all measurement schemas prefixed by a given path.
Parameters:
- request
"""
pass
def previousFill(self, request):
"""
Perform a previous fill and return the timevalue pair in binary.
@return a binary TimeValuePair
Parameters:
- request
"""
pass
def last(self, request):
"""
Query the last point of a series.
@return a binary TimeValuePair
Parameters:
- request
"""
pass
def getPathCount(self, header, pathsToQuery, level):
"""
Parameters:
- header
- pathsToQuery
- level
"""
pass
def getDeviceCount(self, header, pathsToQuery):
"""
Parameters:
- header
- pathsToQuery
"""
pass
def onSnapshotApplied(self, header, slots):
"""
During slot transfer, when a member has pulled snapshot from a group, the member will use this
method to inform the group that one replica of such slots has been pulled.
Parameters:
- header
- slots
"""
pass
def peekNextNotNullValue(self, header, executorId, startTime, endTime):
"""
Parameters:
- header
- executorId
- startTime
- endTime
"""
pass
class Client(iotdb.thrift.cluster.RaftService.Client, Iface):
def __init__(self, iprot, oprot=None):
iotdb.thrift.cluster.RaftService.Client.__init__(self, iprot, oprot)
def querySingleSeries(self, request):
"""
Query a time series without value filter.
@return a readerId >= 0 if the query succeeds, otherwise the query fails
TODO-Cluster: support query multiple series in a request
Parameters:
- request
"""
self.send_querySingleSeries(request)
return self.recv_querySingleSeries()
def send_querySingleSeries(self, request):
self._oprot.writeMessageBegin('querySingleSeries', TMessageType.CALL, self._seqid)
args = querySingleSeries_args()
args.request = request
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_querySingleSeries(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = querySingleSeries_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "querySingleSeries failed: unknown result")
def queryMultSeries(self, request):
"""
Query mult time series without value filter.
@return a readerId >= 0 if the query succeeds, otherwise the query fails
Parameters:
- request
"""
self.send_queryMultSeries(request)
return self.recv_queryMultSeries()
def send_queryMultSeries(self, request):
self._oprot.writeMessageBegin('queryMultSeries', TMessageType.CALL, self._seqid)
args = queryMultSeries_args()
args.request = request
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_queryMultSeries(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = queryMultSeries_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "queryMultSeries failed: unknown result")
def fetchSingleSeries(self, header, readerId):
"""
Fetch at max fetchSize time-value pairs using the resultSetId generated by querySingleSeries.
@return a ByteBuffer containing the serialized time-value pairs or an empty buffer if there
are not more results.
Parameters:
- header
- readerId
"""
self.send_fetchSingleSeries(header, readerId)
return self.recv_fetchSingleSeries()
def send_fetchSingleSeries(self, header, readerId):
self._oprot.writeMessageBegin('fetchSingleSeries', TMessageType.CALL, self._seqid)
args = fetchSingleSeries_args()
args.header = header
args.readerId = readerId
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_fetchSingleSeries(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = fetchSingleSeries_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "fetchSingleSeries failed: unknown result")
def fetchMultSeries(self, header, readerId, paths):
"""
Fetch mult series at max fetchSize time-value pairs using the resultSetId generated by querySingleSeries.
@return a map containing key-value,the serialized time-value pairs or an empty buffer if there
are not more results.
Parameters:
- header
- readerId
- paths
"""
self.send_fetchMultSeries(header, readerId, paths)
return self.recv_fetchMultSeries()
def send_fetchMultSeries(self, header, readerId, paths):
self._oprot.writeMessageBegin('fetchMultSeries', TMessageType.CALL, self._seqid)
args = fetchMultSeries_args()
args.header = header
args.readerId = readerId
args.paths = paths
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_fetchMultSeries(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = fetchMultSeries_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "fetchMultSeries failed: unknown result")
def querySingleSeriesByTimestamp(self, request):
"""
Query a time series and generate an IReaderByTimestamp.
@return a readerId >= 0 if the query succeeds, otherwise the query fails
Parameters:
- request
"""
self.send_querySingleSeriesByTimestamp(request)
return self.recv_querySingleSeriesByTimestamp()
def send_querySingleSeriesByTimestamp(self, request):
self._oprot.writeMessageBegin('querySingleSeriesByTimestamp', TMessageType.CALL, self._seqid)
args = querySingleSeriesByTimestamp_args()
args.request = request
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_querySingleSeriesByTimestamp(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = querySingleSeriesByTimestamp_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "querySingleSeriesByTimestamp failed: unknown result")
def fetchSingleSeriesByTimestamps(self, header, readerId, timestamps):
"""
Fetch values at given timestamps using the resultSetId generated by
querySingleSeriesByTimestamp.
@return a ByteBuffer containing the serialized value or an empty buffer if there
are not more results.
Parameters:
- header
- readerId
- timestamps
"""
self.send_fetchSingleSeriesByTimestamps(header, readerId, timestamps)
return self.recv_fetchSingleSeriesByTimestamps()
def send_fetchSingleSeriesByTimestamps(self, header, readerId, timestamps):
self._oprot.writeMessageBegin('fetchSingleSeriesByTimestamps', TMessageType.CALL, self._seqid)
args = fetchSingleSeriesByTimestamps_args()
args.header = header
args.readerId = readerId
args.timestamps = timestamps
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_fetchSingleSeriesByTimestamps(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = fetchSingleSeriesByTimestamps_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "fetchSingleSeriesByTimestamps failed: unknown result")
def endQuery(self, header, thisNode, queryId):
"""
Find the local query established for the remote query and release all its resource.
Parameters:
- header
- thisNode
- queryId
"""
self.send_endQuery(header, thisNode, queryId)
self.recv_endQuery()
def send_endQuery(self, header, thisNode, queryId):
self._oprot.writeMessageBegin('endQuery', TMessageType.CALL, self._seqid)
args = endQuery_args()
args.header = header
args.thisNode = thisNode
args.queryId = queryId
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_endQuery(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = endQuery_result()
result.read(iprot)
iprot.readMessageEnd()
return
def getAllPaths(self, header, paths, withAlias):
"""
Given path patterns (paths with wildcard), return all paths they match.
Parameters:
- header
- paths
- withAlias
"""
self.send_getAllPaths(header, paths, withAlias)
return self.recv_getAllPaths()
def send_getAllPaths(self, header, paths, withAlias):
self._oprot.writeMessageBegin('getAllPaths', TMessageType.CALL, self._seqid)
args = getAllPaths_args()
args.header = header
args.paths = paths
args.withAlias = withAlias
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getAllPaths(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getAllPaths_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getAllPaths failed: unknown result")
def getAllDevices(self, header, path, isPrefixMatch):
"""
Given path patterns (paths with wildcard), return all devices they match.
Parameters:
- header
- path
- isPrefixMatch
"""
self.send_getAllDevices(header, path, isPrefixMatch)
return self.recv_getAllDevices()
def send_getAllDevices(self, header, path, isPrefixMatch):
self._oprot.writeMessageBegin('getAllDevices', TMessageType.CALL, self._seqid)
args = getAllDevices_args()
args.header = header
args.path = path
args.isPrefixMatch = isPrefixMatch
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getAllDevices(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getAllDevices_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getAllDevices failed: unknown result")
def getDevices(self, header, planBinary):
"""
Get the devices from the header according to the showDevicesPlan
Parameters:
- header
- planBinary
"""
self.send_getDevices(header, planBinary)
return self.recv_getDevices()
def send_getDevices(self, header, planBinary):
self._oprot.writeMessageBegin('getDevices', TMessageType.CALL, self._seqid)
args = getDevices_args()
args.header = header
args.planBinary = planBinary
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getDevices(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getDevices_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getDevices failed: unknown result")
def getNodeList(self, header, path, nodeLevel):
"""
Parameters:
- header
- path
- nodeLevel
"""
self.send_getNodeList(header, path, nodeLevel)
return self.recv_getNodeList()
def send_getNodeList(self, header, path, nodeLevel):
self._oprot.writeMessageBegin('getNodeList', TMessageType.CALL, self._seqid)
args = getNodeList_args()
args.header = header
args.path = path
args.nodeLevel = nodeLevel
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getNodeList(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getNodeList_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getNodeList failed: unknown result")
def getChildNodeInNextLevel(self, header, path):
"""
Given path patterns(paths with wildcard), return all children nodes they match
Parameters:
- header
- path
"""
self.send_getChildNodeInNextLevel(header, path)
return self.recv_getChildNodeInNextLevel()
def send_getChildNodeInNextLevel(self, header, path):
self._oprot.writeMessageBegin('getChildNodeInNextLevel', TMessageType.CALL, self._seqid)
args = getChildNodeInNextLevel_args()
args.header = header
args.path = path
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getChildNodeInNextLevel(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getChildNodeInNextLevel_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getChildNodeInNextLevel failed: unknown result")
def getChildNodePathInNextLevel(self, header, path):
"""
Parameters:
- header
- path
"""
self.send_getChildNodePathInNextLevel(header, path)
return self.recv_getChildNodePathInNextLevel()
def send_getChildNodePathInNextLevel(self, header, path):
self._oprot.writeMessageBegin('getChildNodePathInNextLevel', TMessageType.CALL, self._seqid)
args = getChildNodePathInNextLevel_args()
args.header = header
args.path = path
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getChildNodePathInNextLevel(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getChildNodePathInNextLevel_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getChildNodePathInNextLevel failed: unknown result")
def getAllMeasurementSchema(self, request):
"""
Parameters:
- request
"""
self.send_getAllMeasurementSchema(request)
return self.recv_getAllMeasurementSchema()
def send_getAllMeasurementSchema(self, request):
self._oprot.writeMessageBegin('getAllMeasurementSchema', TMessageType.CALL, self._seqid)
args = getAllMeasurementSchema_args()
args.request = request
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getAllMeasurementSchema(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getAllMeasurementSchema_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getAllMeasurementSchema failed: unknown result")
def getAggrResult(self, request):
"""
Parameters:
- request
"""
self.send_getAggrResult(request)
return self.recv_getAggrResult()
def send_getAggrResult(self, request):
self._oprot.writeMessageBegin('getAggrResult', TMessageType.CALL, self._seqid)
args = getAggrResult_args()
args.request = request
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getAggrResult(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getAggrResult_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getAggrResult failed: unknown result")
def getUnregisteredTimeseries(self, header, timeseriesList):
"""
Parameters:
- header
- timeseriesList
"""
self.send_getUnregisteredTimeseries(header, timeseriesList)
return self.recv_getUnregisteredTimeseries()
def send_getUnregisteredTimeseries(self, header, timeseriesList):
self._oprot.writeMessageBegin('getUnregisteredTimeseries', TMessageType.CALL, self._seqid)
args = getUnregisteredTimeseries_args()
args.header = header
args.timeseriesList = timeseriesList
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getUnregisteredTimeseries(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getUnregisteredTimeseries_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getUnregisteredTimeseries failed: unknown result")
def pullSnapshot(self, request):
"""
Parameters:
- request
"""
self.send_pullSnapshot(request)
return self.recv_pullSnapshot()
def send_pullSnapshot(self, request):
self._oprot.writeMessageBegin('pullSnapshot', TMessageType.CALL, self._seqid)
args = pullSnapshot_args()
args.request = request
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_pullSnapshot(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = pullSnapshot_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "pullSnapshot failed: unknown result")
def getGroupByExecutor(self, request):
"""
Create a GroupByExecutor for a path, executing the given aggregations.
@return the executorId
Parameters:
- request
"""
self.send_getGroupByExecutor(request)
return self.recv_getGroupByExecutor()
def send_getGroupByExecutor(self, request):
self._oprot.writeMessageBegin('getGroupByExecutor', TMessageType.CALL, self._seqid)
args = getGroupByExecutor_args()
args.request = request
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getGroupByExecutor(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getGroupByExecutor_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getGroupByExecutor failed: unknown result")
def getGroupByResult(self, header, executorId, startTime, endTime):
"""
Fetch the group by result in the interval [startTime, endTime) from the given executor.
@return the serialized AggregationResults, each is the result of one of the previously
required aggregations, and their orders are the same.
Parameters:
- header
- executorId
- startTime
- endTime
"""
self.send_getGroupByResult(header, executorId, startTime, endTime)
return self.recv_getGroupByResult()
def send_getGroupByResult(self, header, executorId, startTime, endTime):
self._oprot.writeMessageBegin('getGroupByResult', TMessageType.CALL, self._seqid)
args = getGroupByResult_args()
args.header = header
args.executorId = executorId
args.startTime = startTime
args.endTime = endTime
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getGroupByResult(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getGroupByResult_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getGroupByResult failed: unknown result")
def pullTimeSeriesSchema(self, request):
"""
Pull all timeseries schemas prefixed by a given path.
Parameters:
- request
"""
self.send_pullTimeSeriesSchema(request)
return self.recv_pullTimeSeriesSchema()
def send_pullTimeSeriesSchema(self, request):
self._oprot.writeMessageBegin('pullTimeSeriesSchema', TMessageType.CALL, self._seqid)
args = pullTimeSeriesSchema_args()
args.request = request
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_pullTimeSeriesSchema(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = pullTimeSeriesSchema_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "pullTimeSeriesSchema failed: unknown result")
def pullMeasurementSchema(self, request):
"""
Pull all measurement schemas prefixed by a given path.
Parameters:
- request
"""
self.send_pullMeasurementSchema(request)
return self.recv_pullMeasurementSchema()
def send_pullMeasurementSchema(self, request):
self._oprot.writeMessageBegin('pullMeasurementSchema', TMessageType.CALL, self._seqid)
args = pullMeasurementSchema_args()
args.request = request
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_pullMeasurementSchema(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = pullMeasurementSchema_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "pullMeasurementSchema failed: unknown result")
def previousFill(self, request):
"""
Perform a previous fill and return the timevalue pair in binary.
@return a binary TimeValuePair
Parameters:
- request
"""
self.send_previousFill(request)
return self.recv_previousFill()
def send_previousFill(self, request):
self._oprot.writeMessageBegin('previousFill', TMessageType.CALL, self._seqid)
args = previousFill_args()
args.request = request
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_previousFill(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = previousFill_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "previousFill failed: unknown result")
def last(self, request):
"""
Query the last point of a series.
@return a binary TimeValuePair
Parameters:
- request
"""
self.send_last(request)
return self.recv_last()
def send_last(self, request):
self._oprot.writeMessageBegin('last', TMessageType.CALL, self._seqid)
args = last_args()
args.request = request
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_last(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = last_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "last failed: unknown result")
def getPathCount(self, header, pathsToQuery, level):
"""
Parameters:
- header
- pathsToQuery
- level
"""
self.send_getPathCount(header, pathsToQuery, level)
return self.recv_getPathCount()
def send_getPathCount(self, header, pathsToQuery, level):
self._oprot.writeMessageBegin('getPathCount', TMessageType.CALL, self._seqid)
args = getPathCount_args()
args.header = header
args.pathsToQuery = pathsToQuery
args.level = level
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getPathCount(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getPathCount_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getPathCount failed: unknown result")
def getDeviceCount(self, header, pathsToQuery):
"""
Parameters:
- header
- pathsToQuery
"""
self.send_getDeviceCount(header, pathsToQuery)
return self.recv_getDeviceCount()
def send_getDeviceCount(self, header, pathsToQuery):
self._oprot.writeMessageBegin('getDeviceCount', TMessageType.CALL, self._seqid)
args = getDeviceCount_args()
args.header = header
args.pathsToQuery = pathsToQuery
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_getDeviceCount(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = getDeviceCount_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "getDeviceCount failed: unknown result")
def onSnapshotApplied(self, header, slots):
"""
During slot transfer, when a member has pulled snapshot from a group, the member will use this
method to inform the group that one replica of such slots has been pulled.
Parameters:
- header
- slots
"""
self.send_onSnapshotApplied(header, slots)
return self.recv_onSnapshotApplied()
def send_onSnapshotApplied(self, header, slots):
self._oprot.writeMessageBegin('onSnapshotApplied', TMessageType.CALL, self._seqid)
args = onSnapshotApplied_args()
args.header = header
args.slots = slots
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_onSnapshotApplied(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = onSnapshotApplied_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "onSnapshotApplied failed: unknown result")
def peekNextNotNullValue(self, header, executorId, startTime, endTime):
"""
Parameters:
- header
- executorId
- startTime
- endTime
"""
self.send_peekNextNotNullValue(header, executorId, startTime, endTime)
return self.recv_peekNextNotNullValue()
def send_peekNextNotNullValue(self, header, executorId, startTime, endTime):
self._oprot.writeMessageBegin('peekNextNotNullValue', TMessageType.CALL, self._seqid)
args = peekNextNotNullValue_args()
args.header = header
args.executorId = executorId
args.startTime = startTime
args.endTime = endTime
args.write(self._oprot)
self._oprot.writeMessageEnd()
self._oprot.trans.flush()
def recv_peekNextNotNullValue(self):
iprot = self._iprot
(fname, mtype, rseqid) = iprot.readMessageBegin()
if mtype == TMessageType.EXCEPTION:
x = TApplicationException()
x.read(iprot)
iprot.readMessageEnd()
raise x
result = peekNextNotNullValue_result()
result.read(iprot)
iprot.readMessageEnd()
if result.success is not None:
return result.success
raise TApplicationException(TApplicationException.MISSING_RESULT, "peekNextNotNullValue failed: unknown result")
class Processor(iotdb.thrift.cluster.RaftService.Processor, Iface, TProcessor):
def __init__(self, handler):
iotdb.thrift.cluster.RaftService.Processor.__init__(self, handler)
self._processMap["querySingleSeries"] = Processor.process_querySingleSeries
self._processMap["queryMultSeries"] = Processor.process_queryMultSeries
self._processMap["fetchSingleSeries"] = Processor.process_fetchSingleSeries
self._processMap["fetchMultSeries"] = Processor.process_fetchMultSeries
self._processMap["querySingleSeriesByTimestamp"] = Processor.process_querySingleSeriesByTimestamp
self._processMap["fetchSingleSeriesByTimestamps"] = Processor.process_fetchSingleSeriesByTimestamps
self._processMap["endQuery"] = Processor.process_endQuery
self._processMap["getAllPaths"] = Processor.process_getAllPaths
self._processMap["getAllDevices"] = Processor.process_getAllDevices
self._processMap["getDevices"] = Processor.process_getDevices
self._processMap["getNodeList"] = Processor.process_getNodeList
self._processMap["getChildNodeInNextLevel"] = Processor.process_getChildNodeInNextLevel
self._processMap["getChildNodePathInNextLevel"] = Processor.process_getChildNodePathInNextLevel
self._processMap["getAllMeasurementSchema"] = Processor.process_getAllMeasurementSchema
self._processMap["getAggrResult"] = Processor.process_getAggrResult
self._processMap["getUnregisteredTimeseries"] = Processor.process_getUnregisteredTimeseries
self._processMap["pullSnapshot"] = Processor.process_pullSnapshot
self._processMap["getGroupByExecutor"] = Processor.process_getGroupByExecutor
self._processMap["getGroupByResult"] = Processor.process_getGroupByResult
self._processMap["pullTimeSeriesSchema"] = Processor.process_pullTimeSeriesSchema
self._processMap["pullMeasurementSchema"] = Processor.process_pullMeasurementSchema
self._processMap["previousFill"] = Processor.process_previousFill
self._processMap["last"] = Processor.process_last
self._processMap["getPathCount"] = Processor.process_getPathCount
self._processMap["getDeviceCount"] = Processor.process_getDeviceCount
self._processMap["onSnapshotApplied"] = Processor.process_onSnapshotApplied
self._processMap["peekNextNotNullValue"] = Processor.process_peekNextNotNullValue
self._on_message_begin = None
def on_message_begin(self, func):
self._on_message_begin = func
def process(self, iprot, oprot):
(name, type, seqid) = iprot.readMessageBegin()
if self._on_message_begin:
self._on_message_begin(name, type, seqid)
if name not in self._processMap:
iprot.skip(TType.STRUCT)
iprot.readMessageEnd()
x = TApplicationException(TApplicationException.UNKNOWN_METHOD, 'Unknown function %s' % (name))
oprot.writeMessageBegin(name, TMessageType.EXCEPTION, seqid)
x.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
return
else:
self._processMap[name](self, seqid, iprot, oprot)
return True
def process_querySingleSeries(self, seqid, iprot, oprot):
args = querySingleSeries_args()
args.read(iprot)
iprot.readMessageEnd()
result = querySingleSeries_result()
try:
result.success = self._handler.querySingleSeries(args.request)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("querySingleSeries", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_queryMultSeries(self, seqid, iprot, oprot):
args = queryMultSeries_args()
args.read(iprot)
iprot.readMessageEnd()
result = queryMultSeries_result()
try:
result.success = self._handler.queryMultSeries(args.request)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("queryMultSeries", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_fetchSingleSeries(self, seqid, iprot, oprot):
args = fetchSingleSeries_args()
args.read(iprot)
iprot.readMessageEnd()
result = fetchSingleSeries_result()
try:
result.success = self._handler.fetchSingleSeries(args.header, args.readerId)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("fetchSingleSeries", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_fetchMultSeries(self, seqid, iprot, oprot):
args = fetchMultSeries_args()
args.read(iprot)
iprot.readMessageEnd()
result = fetchMultSeries_result()
try:
result.success = self._handler.fetchMultSeries(args.header, args.readerId, args.paths)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("fetchMultSeries", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_querySingleSeriesByTimestamp(self, seqid, iprot, oprot):
args = querySingleSeriesByTimestamp_args()
args.read(iprot)
iprot.readMessageEnd()
result = querySingleSeriesByTimestamp_result()
try:
result.success = self._handler.querySingleSeriesByTimestamp(args.request)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("querySingleSeriesByTimestamp", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_fetchSingleSeriesByTimestamps(self, seqid, iprot, oprot):
args = fetchSingleSeriesByTimestamps_args()
args.read(iprot)
iprot.readMessageEnd()
result = fetchSingleSeriesByTimestamps_result()
try:
result.success = self._handler.fetchSingleSeriesByTimestamps(args.header, args.readerId, args.timestamps)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("fetchSingleSeriesByTimestamps", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_endQuery(self, seqid, iprot, oprot):
args = endQuery_args()
args.read(iprot)
iprot.readMessageEnd()
result = endQuery_result()
try:
self._handler.endQuery(args.header, args.thisNode, args.queryId)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("endQuery", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getAllPaths(self, seqid, iprot, oprot):
args = getAllPaths_args()
args.read(iprot)
iprot.readMessageEnd()
result = getAllPaths_result()
try:
result.success = self._handler.getAllPaths(args.header, args.paths, args.withAlias)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getAllPaths", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getAllDevices(self, seqid, iprot, oprot):
args = getAllDevices_args()
args.read(iprot)
iprot.readMessageEnd()
result = getAllDevices_result()
try:
result.success = self._handler.getAllDevices(args.header, args.path, args.isPrefixMatch)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getAllDevices", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getDevices(self, seqid, iprot, oprot):
args = getDevices_args()
args.read(iprot)
iprot.readMessageEnd()
result = getDevices_result()
try:
result.success = self._handler.getDevices(args.header, args.planBinary)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getDevices", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getNodeList(self, seqid, iprot, oprot):
args = getNodeList_args()
args.read(iprot)
iprot.readMessageEnd()
result = getNodeList_result()
try:
result.success = self._handler.getNodeList(args.header, args.path, args.nodeLevel)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getNodeList", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getChildNodeInNextLevel(self, seqid, iprot, oprot):
args = getChildNodeInNextLevel_args()
args.read(iprot)
iprot.readMessageEnd()
result = getChildNodeInNextLevel_result()
try:
result.success = self._handler.getChildNodeInNextLevel(args.header, args.path)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getChildNodeInNextLevel", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getChildNodePathInNextLevel(self, seqid, iprot, oprot):
args = getChildNodePathInNextLevel_args()
args.read(iprot)
iprot.readMessageEnd()
result = getChildNodePathInNextLevel_result()
try:
result.success = self._handler.getChildNodePathInNextLevel(args.header, args.path)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getChildNodePathInNextLevel", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getAllMeasurementSchema(self, seqid, iprot, oprot):
args = getAllMeasurementSchema_args()
args.read(iprot)
iprot.readMessageEnd()
result = getAllMeasurementSchema_result()
try:
result.success = self._handler.getAllMeasurementSchema(args.request)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getAllMeasurementSchema", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getAggrResult(self, seqid, iprot, oprot):
args = getAggrResult_args()
args.read(iprot)
iprot.readMessageEnd()
result = getAggrResult_result()
try:
result.success = self._handler.getAggrResult(args.request)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getAggrResult", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getUnregisteredTimeseries(self, seqid, iprot, oprot):
args = getUnregisteredTimeseries_args()
args.read(iprot)
iprot.readMessageEnd()
result = getUnregisteredTimeseries_result()
try:
result.success = self._handler.getUnregisteredTimeseries(args.header, args.timeseriesList)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getUnregisteredTimeseries", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_pullSnapshot(self, seqid, iprot, oprot):
args = pullSnapshot_args()
args.read(iprot)
iprot.readMessageEnd()
result = pullSnapshot_result()
try:
result.success = self._handler.pullSnapshot(args.request)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("pullSnapshot", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getGroupByExecutor(self, seqid, iprot, oprot):
args = getGroupByExecutor_args()
args.read(iprot)
iprot.readMessageEnd()
result = getGroupByExecutor_result()
try:
result.success = self._handler.getGroupByExecutor(args.request)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getGroupByExecutor", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getGroupByResult(self, seqid, iprot, oprot):
args = getGroupByResult_args()
args.read(iprot)
iprot.readMessageEnd()
result = getGroupByResult_result()
try:
result.success = self._handler.getGroupByResult(args.header, args.executorId, args.startTime, args.endTime)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getGroupByResult", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_pullTimeSeriesSchema(self, seqid, iprot, oprot):
args = pullTimeSeriesSchema_args()
args.read(iprot)
iprot.readMessageEnd()
result = pullTimeSeriesSchema_result()
try:
result.success = self._handler.pullTimeSeriesSchema(args.request)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("pullTimeSeriesSchema", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_pullMeasurementSchema(self, seqid, iprot, oprot):
args = pullMeasurementSchema_args()
args.read(iprot)
iprot.readMessageEnd()
result = pullMeasurementSchema_result()
try:
result.success = self._handler.pullMeasurementSchema(args.request)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("pullMeasurementSchema", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_previousFill(self, seqid, iprot, oprot):
args = previousFill_args()
args.read(iprot)
iprot.readMessageEnd()
result = previousFill_result()
try:
result.success = self._handler.previousFill(args.request)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("previousFill", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_last(self, seqid, iprot, oprot):
args = last_args()
args.read(iprot)
iprot.readMessageEnd()
result = last_result()
try:
result.success = self._handler.last(args.request)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("last", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getPathCount(self, seqid, iprot, oprot):
args = getPathCount_args()
args.read(iprot)
iprot.readMessageEnd()
result = getPathCount_result()
try:
result.success = self._handler.getPathCount(args.header, args.pathsToQuery, args.level)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getPathCount", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_getDeviceCount(self, seqid, iprot, oprot):
args = getDeviceCount_args()
args.read(iprot)
iprot.readMessageEnd()
result = getDeviceCount_result()
try:
result.success = self._handler.getDeviceCount(args.header, args.pathsToQuery)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("getDeviceCount", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_onSnapshotApplied(self, seqid, iprot, oprot):
args = onSnapshotApplied_args()
args.read(iprot)
iprot.readMessageEnd()
result = onSnapshotApplied_result()
try:
result.success = self._handler.onSnapshotApplied(args.header, args.slots)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("onSnapshotApplied", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
def process_peekNextNotNullValue(self, seqid, iprot, oprot):
args = peekNextNotNullValue_args()
args.read(iprot)
iprot.readMessageEnd()
result = peekNextNotNullValue_result()
try:
result.success = self._handler.peekNextNotNullValue(args.header, args.executorId, args.startTime, args.endTime)
msg_type = TMessageType.REPLY
except TTransport.TTransportException:
raise
except TApplicationException as ex:
logging.exception('TApplication exception in handler')
msg_type = TMessageType.EXCEPTION
result = ex
except Exception:
logging.exception('Unexpected exception in handler')
msg_type = TMessageType.EXCEPTION
result = TApplicationException(TApplicationException.INTERNAL_ERROR, 'Internal error')
oprot.writeMessageBegin("peekNextNotNullValue", msg_type, seqid)
result.write(oprot)
oprot.writeMessageEnd()
oprot.trans.flush()
# HELPER FUNCTIONS AND STRUCTURES
class querySingleSeries_args(object):
"""
Attributes:
- request
"""
def __init__(self, request=None,):
self.request = request
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.request = SingleSeriesQueryRequest()
self.request.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('querySingleSeries_args')
if self.request is not None:
oprot.writeFieldBegin('request', TType.STRUCT, 1)
self.request.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(querySingleSeries_args)
querySingleSeries_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'request', [SingleSeriesQueryRequest, None], None, ), # 1
)
class querySingleSeries_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.I64:
self.success = iprot.readI64()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('querySingleSeries_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.I64, 0)
oprot.writeI64(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(querySingleSeries_result)
querySingleSeries_result.thrift_spec = (
(0, TType.I64, 'success', None, None, ), # 0
)
class queryMultSeries_args(object):
"""
Attributes:
- request
"""
def __init__(self, request=None,):
self.request = request
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.request = MultSeriesQueryRequest()
self.request.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('queryMultSeries_args')
if self.request is not None:
oprot.writeFieldBegin('request', TType.STRUCT, 1)
self.request.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(queryMultSeries_args)
queryMultSeries_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'request', [MultSeriesQueryRequest, None], None, ), # 1
)
class queryMultSeries_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.I64:
self.success = iprot.readI64()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('queryMultSeries_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.I64, 0)
oprot.writeI64(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(queryMultSeries_result)
queryMultSeries_result.thrift_spec = (
(0, TType.I64, 'success', None, None, ), # 0
)
class fetchSingleSeries_args(object):
"""
Attributes:
- header
- readerId
"""
def __init__(self, header=None, readerId=None,):
self.header = header
self.readerId = readerId
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.I64:
self.readerId = iprot.readI64()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('fetchSingleSeries_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.readerId is not None:
oprot.writeFieldBegin('readerId', TType.I64, 2)
oprot.writeI64(self.readerId)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(fetchSingleSeries_args)
fetchSingleSeries_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.I64, 'readerId', None, None, ), # 2
)
class fetchSingleSeries_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.STRING:
self.success = iprot.readBinary()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('fetchSingleSeries_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.STRING, 0)
oprot.writeBinary(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(fetchSingleSeries_result)
fetchSingleSeries_result.thrift_spec = (
(0, TType.STRING, 'success', 'BINARY', None, ), # 0
)
class fetchMultSeries_args(object):
"""
Attributes:
- header
- readerId
- paths
"""
def __init__(self, header=None, readerId=None, paths=None,):
self.header = header
self.readerId = readerId
self.paths = paths
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.I64:
self.readerId = iprot.readI64()
else:
iprot.skip(ftype)
elif fid == 3:
if ftype == TType.LIST:
self.paths = []
(_etype184, _size181) = iprot.readListBegin()
for _i185 in range(_size181):
_elem186 = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
self.paths.append(_elem186)
iprot.readListEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('fetchMultSeries_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.readerId is not None:
oprot.writeFieldBegin('readerId', TType.I64, 2)
oprot.writeI64(self.readerId)
oprot.writeFieldEnd()
if self.paths is not None:
oprot.writeFieldBegin('paths', TType.LIST, 3)
oprot.writeListBegin(TType.STRING, len(self.paths))
for iter187 in self.paths:
oprot.writeString(iter187.encode('utf-8') if sys.version_info[0] == 2 else iter187)
oprot.writeListEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(fetchMultSeries_args)
fetchMultSeries_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.I64, 'readerId', None, None, ), # 2
(3, TType.LIST, 'paths', (TType.STRING, 'UTF8', False), None, ), # 3
)
class fetchMultSeries_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.MAP:
self.success = {}
(_ktype189, _vtype190, _size188) = iprot.readMapBegin()
for _i192 in range(_size188):
_key193 = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
_val194 = iprot.readBinary()
self.success[_key193] = _val194
iprot.readMapEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('fetchMultSeries_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.MAP, 0)
oprot.writeMapBegin(TType.STRING, TType.STRING, len(self.success))
for kiter195, viter196 in self.success.items():
oprot.writeString(kiter195.encode('utf-8') if sys.version_info[0] == 2 else kiter195)
oprot.writeBinary(viter196)
oprot.writeMapEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(fetchMultSeries_result)
fetchMultSeries_result.thrift_spec = (
(0, TType.MAP, 'success', (TType.STRING, 'UTF8', TType.STRING, 'BINARY', False), None, ), # 0
)
class querySingleSeriesByTimestamp_args(object):
"""
Attributes:
- request
"""
def __init__(self, request=None,):
self.request = request
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.request = SingleSeriesQueryRequest()
self.request.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('querySingleSeriesByTimestamp_args')
if self.request is not None:
oprot.writeFieldBegin('request', TType.STRUCT, 1)
self.request.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(querySingleSeriesByTimestamp_args)
querySingleSeriesByTimestamp_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'request', [SingleSeriesQueryRequest, None], None, ), # 1
)
class querySingleSeriesByTimestamp_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.I64:
self.success = iprot.readI64()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('querySingleSeriesByTimestamp_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.I64, 0)
oprot.writeI64(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(querySingleSeriesByTimestamp_result)
querySingleSeriesByTimestamp_result.thrift_spec = (
(0, TType.I64, 'success', None, None, ), # 0
)
class fetchSingleSeriesByTimestamps_args(object):
"""
Attributes:
- header
- readerId
- timestamps
"""
def __init__(self, header=None, readerId=None, timestamps=None,):
self.header = header
self.readerId = readerId
self.timestamps = timestamps
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.I64:
self.readerId = iprot.readI64()
else:
iprot.skip(ftype)
elif fid == 3:
if ftype == TType.LIST:
self.timestamps = []
(_etype200, _size197) = iprot.readListBegin()
for _i201 in range(_size197):
_elem202 = iprot.readI64()
self.timestamps.append(_elem202)
iprot.readListEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('fetchSingleSeriesByTimestamps_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.readerId is not None:
oprot.writeFieldBegin('readerId', TType.I64, 2)
oprot.writeI64(self.readerId)
oprot.writeFieldEnd()
if self.timestamps is not None:
oprot.writeFieldBegin('timestamps', TType.LIST, 3)
oprot.writeListBegin(TType.I64, len(self.timestamps))
for iter203 in self.timestamps:
oprot.writeI64(iter203)
oprot.writeListEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(fetchSingleSeriesByTimestamps_args)
fetchSingleSeriesByTimestamps_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.I64, 'readerId', None, None, ), # 2
(3, TType.LIST, 'timestamps', (TType.I64, None, False), None, ), # 3
)
class fetchSingleSeriesByTimestamps_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.STRING:
self.success = iprot.readBinary()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('fetchSingleSeriesByTimestamps_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.STRING, 0)
oprot.writeBinary(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(fetchSingleSeriesByTimestamps_result)
fetchSingleSeriesByTimestamps_result.thrift_spec = (
(0, TType.STRING, 'success', 'BINARY', None, ), # 0
)
class endQuery_args(object):
"""
Attributes:
- header
- thisNode
- queryId
"""
def __init__(self, header=None, thisNode=None, queryId=None,):
self.header = header
self.thisNode = thisNode
self.queryId = queryId
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 3:
if ftype == TType.STRUCT:
self.thisNode = Node()
self.thisNode.read(iprot)
else:
iprot.skip(ftype)
elif fid == 4:
if ftype == TType.I64:
self.queryId = iprot.readI64()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('endQuery_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.thisNode is not None:
oprot.writeFieldBegin('thisNode', TType.STRUCT, 3)
self.thisNode.write(oprot)
oprot.writeFieldEnd()
if self.queryId is not None:
oprot.writeFieldBegin('queryId', TType.I64, 4)
oprot.writeI64(self.queryId)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(endQuery_args)
endQuery_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
None, # 2
(3, TType.STRUCT, 'thisNode', [Node, None], None, ), # 3
(4, TType.I64, 'queryId', None, None, ), # 4
)
class endQuery_result(object):
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('endQuery_result')
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(endQuery_result)
endQuery_result.thrift_spec = (
)
class getAllPaths_args(object):
"""
Attributes:
- header
- paths
- withAlias
"""
def __init__(self, header=None, paths=None, withAlias=None,):
self.header = header
self.paths = paths
self.withAlias = withAlias
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.LIST:
self.paths = []
(_etype207, _size204) = iprot.readListBegin()
for _i208 in range(_size204):
_elem209 = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
self.paths.append(_elem209)
iprot.readListEnd()
else:
iprot.skip(ftype)
elif fid == 3:
if ftype == TType.BOOL:
self.withAlias = iprot.readBool()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getAllPaths_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.paths is not None:
oprot.writeFieldBegin('paths', TType.LIST, 2)
oprot.writeListBegin(TType.STRING, len(self.paths))
for iter210 in self.paths:
oprot.writeString(iter210.encode('utf-8') if sys.version_info[0] == 2 else iter210)
oprot.writeListEnd()
oprot.writeFieldEnd()
if self.withAlias is not None:
oprot.writeFieldBegin('withAlias', TType.BOOL, 3)
oprot.writeBool(self.withAlias)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getAllPaths_args)
getAllPaths_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.LIST, 'paths', (TType.STRING, 'UTF8', False), None, ), # 2
(3, TType.BOOL, 'withAlias', None, None, ), # 3
)
class getAllPaths_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.STRUCT:
self.success = GetAllPathsResult()
self.success.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getAllPaths_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.STRUCT, 0)
self.success.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getAllPaths_result)
getAllPaths_result.thrift_spec = (
(0, TType.STRUCT, 'success', [GetAllPathsResult, None], None, ), # 0
)
class getAllDevices_args(object):
"""
Attributes:
- header
- path
- isPrefixMatch
"""
def __init__(self, header=None, path=None, isPrefixMatch=None,):
self.header = header
self.path = path
self.isPrefixMatch = isPrefixMatch
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.LIST:
self.path = []
(_etype214, _size211) = iprot.readListBegin()
for _i215 in range(_size211):
_elem216 = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
self.path.append(_elem216)
iprot.readListEnd()
else:
iprot.skip(ftype)
elif fid == 3:
if ftype == TType.BOOL:
self.isPrefixMatch = iprot.readBool()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getAllDevices_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.path is not None:
oprot.writeFieldBegin('path', TType.LIST, 2)
oprot.writeListBegin(TType.STRING, len(self.path))
for iter217 in self.path:
oprot.writeString(iter217.encode('utf-8') if sys.version_info[0] == 2 else iter217)
oprot.writeListEnd()
oprot.writeFieldEnd()
if self.isPrefixMatch is not None:
oprot.writeFieldBegin('isPrefixMatch', TType.BOOL, 3)
oprot.writeBool(self.isPrefixMatch)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getAllDevices_args)
getAllDevices_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.LIST, 'path', (TType.STRING, 'UTF8', False), None, ), # 2
(3, TType.BOOL, 'isPrefixMatch', None, None, ), # 3
)
class getAllDevices_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.SET:
self.success = set()
(_etype221, _size218) = iprot.readSetBegin()
for _i222 in range(_size218):
_elem223 = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
self.success.add(_elem223)
iprot.readSetEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getAllDevices_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.SET, 0)
oprot.writeSetBegin(TType.STRING, len(self.success))
for iter224 in self.success:
oprot.writeString(iter224.encode('utf-8') if sys.version_info[0] == 2 else iter224)
oprot.writeSetEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getAllDevices_result)
getAllDevices_result.thrift_spec = (
(0, TType.SET, 'success', (TType.STRING, 'UTF8', False), None, ), # 0
)
class getDevices_args(object):
"""
Attributes:
- header
- planBinary
"""
def __init__(self, header=None, planBinary=None,):
self.header = header
self.planBinary = planBinary
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.STRING:
self.planBinary = iprot.readBinary()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getDevices_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.planBinary is not None:
oprot.writeFieldBegin('planBinary', TType.STRING, 2)
oprot.writeBinary(self.planBinary)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getDevices_args)
getDevices_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.STRING, 'planBinary', 'BINARY', None, ), # 2
)
class getDevices_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.STRING:
self.success = iprot.readBinary()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getDevices_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.STRING, 0)
oprot.writeBinary(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getDevices_result)
getDevices_result.thrift_spec = (
(0, TType.STRING, 'success', 'BINARY', None, ), # 0
)
class getNodeList_args(object):
"""
Attributes:
- header
- path
- nodeLevel
"""
def __init__(self, header=None, path=None, nodeLevel=None,):
self.header = header
self.path = path
self.nodeLevel = nodeLevel
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.STRING:
self.path = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
else:
iprot.skip(ftype)
elif fid == 3:
if ftype == TType.I32:
self.nodeLevel = iprot.readI32()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getNodeList_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.path is not None:
oprot.writeFieldBegin('path', TType.STRING, 2)
oprot.writeString(self.path.encode('utf-8') if sys.version_info[0] == 2 else self.path)
oprot.writeFieldEnd()
if self.nodeLevel is not None:
oprot.writeFieldBegin('nodeLevel', TType.I32, 3)
oprot.writeI32(self.nodeLevel)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getNodeList_args)
getNodeList_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.STRING, 'path', 'UTF8', None, ), # 2
(3, TType.I32, 'nodeLevel', None, None, ), # 3
)
class getNodeList_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.LIST:
self.success = []
(_etype228, _size225) = iprot.readListBegin()
for _i229 in range(_size225):
_elem230 = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
self.success.append(_elem230)
iprot.readListEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getNodeList_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.LIST, 0)
oprot.writeListBegin(TType.STRING, len(self.success))
for iter231 in self.success:
oprot.writeString(iter231.encode('utf-8') if sys.version_info[0] == 2 else iter231)
oprot.writeListEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getNodeList_result)
getNodeList_result.thrift_spec = (
(0, TType.LIST, 'success', (TType.STRING, 'UTF8', False), None, ), # 0
)
class getChildNodeInNextLevel_args(object):
"""
Attributes:
- header
- path
"""
def __init__(self, header=None, path=None,):
self.header = header
self.path = path
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.STRING:
self.path = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getChildNodeInNextLevel_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.path is not None:
oprot.writeFieldBegin('path', TType.STRING, 2)
oprot.writeString(self.path.encode('utf-8') if sys.version_info[0] == 2 else self.path)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getChildNodeInNextLevel_args)
getChildNodeInNextLevel_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.STRING, 'path', 'UTF8', None, ), # 2
)
class getChildNodeInNextLevel_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.SET:
self.success = set()
(_etype235, _size232) = iprot.readSetBegin()
for _i236 in range(_size232):
_elem237 = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
self.success.add(_elem237)
iprot.readSetEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getChildNodeInNextLevel_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.SET, 0)
oprot.writeSetBegin(TType.STRING, len(self.success))
for iter238 in self.success:
oprot.writeString(iter238.encode('utf-8') if sys.version_info[0] == 2 else iter238)
oprot.writeSetEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getChildNodeInNextLevel_result)
getChildNodeInNextLevel_result.thrift_spec = (
(0, TType.SET, 'success', (TType.STRING, 'UTF8', False), None, ), # 0
)
class getChildNodePathInNextLevel_args(object):
"""
Attributes:
- header
- path
"""
def __init__(self, header=None, path=None,):
self.header = header
self.path = path
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.STRING:
self.path = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getChildNodePathInNextLevel_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.path is not None:
oprot.writeFieldBegin('path', TType.STRING, 2)
oprot.writeString(self.path.encode('utf-8') if sys.version_info[0] == 2 else self.path)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getChildNodePathInNextLevel_args)
getChildNodePathInNextLevel_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.STRING, 'path', 'UTF8', None, ), # 2
)
class getChildNodePathInNextLevel_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.SET:
self.success = set()
(_etype242, _size239) = iprot.readSetBegin()
for _i243 in range(_size239):
_elem244 = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
self.success.add(_elem244)
iprot.readSetEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getChildNodePathInNextLevel_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.SET, 0)
oprot.writeSetBegin(TType.STRING, len(self.success))
for iter245 in self.success:
oprot.writeString(iter245.encode('utf-8') if sys.version_info[0] == 2 else iter245)
oprot.writeSetEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getChildNodePathInNextLevel_result)
getChildNodePathInNextLevel_result.thrift_spec = (
(0, TType.SET, 'success', (TType.STRING, 'UTF8', False), None, ), # 0
)
class getAllMeasurementSchema_args(object):
"""
Attributes:
- request
"""
def __init__(self, request=None,):
self.request = request
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.request = MeasurementSchemaRequest()
self.request.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getAllMeasurementSchema_args')
if self.request is not None:
oprot.writeFieldBegin('request', TType.STRUCT, 1)
self.request.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getAllMeasurementSchema_args)
getAllMeasurementSchema_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'request', [MeasurementSchemaRequest, None], None, ), # 1
)
class getAllMeasurementSchema_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.STRING:
self.success = iprot.readBinary()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getAllMeasurementSchema_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.STRING, 0)
oprot.writeBinary(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getAllMeasurementSchema_result)
getAllMeasurementSchema_result.thrift_spec = (
(0, TType.STRING, 'success', 'BINARY', None, ), # 0
)
class getAggrResult_args(object):
"""
Attributes:
- request
"""
def __init__(self, request=None,):
self.request = request
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.request = GetAggrResultRequest()
self.request.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getAggrResult_args')
if self.request is not None:
oprot.writeFieldBegin('request', TType.STRUCT, 1)
self.request.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getAggrResult_args)
getAggrResult_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'request', [GetAggrResultRequest, None], None, ), # 1
)
class getAggrResult_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.LIST:
self.success = []
(_etype249, _size246) = iprot.readListBegin()
for _i250 in range(_size246):
_elem251 = iprot.readBinary()
self.success.append(_elem251)
iprot.readListEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getAggrResult_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.LIST, 0)
oprot.writeListBegin(TType.STRING, len(self.success))
for iter252 in self.success:
oprot.writeBinary(iter252)
oprot.writeListEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getAggrResult_result)
getAggrResult_result.thrift_spec = (
(0, TType.LIST, 'success', (TType.STRING, 'BINARY', False), None, ), # 0
)
class getUnregisteredTimeseries_args(object):
"""
Attributes:
- header
- timeseriesList
"""
def __init__(self, header=None, timeseriesList=None,):
self.header = header
self.timeseriesList = timeseriesList
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.LIST:
self.timeseriesList = []
(_etype256, _size253) = iprot.readListBegin()
for _i257 in range(_size253):
_elem258 = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
self.timeseriesList.append(_elem258)
iprot.readListEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getUnregisteredTimeseries_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.timeseriesList is not None:
oprot.writeFieldBegin('timeseriesList', TType.LIST, 2)
oprot.writeListBegin(TType.STRING, len(self.timeseriesList))
for iter259 in self.timeseriesList:
oprot.writeString(iter259.encode('utf-8') if sys.version_info[0] == 2 else iter259)
oprot.writeListEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getUnregisteredTimeseries_args)
getUnregisteredTimeseries_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.LIST, 'timeseriesList', (TType.STRING, 'UTF8', False), None, ), # 2
)
class getUnregisteredTimeseries_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.LIST:
self.success = []
(_etype263, _size260) = iprot.readListBegin()
for _i264 in range(_size260):
_elem265 = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
self.success.append(_elem265)
iprot.readListEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getUnregisteredTimeseries_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.LIST, 0)
oprot.writeListBegin(TType.STRING, len(self.success))
for iter266 in self.success:
oprot.writeString(iter266.encode('utf-8') if sys.version_info[0] == 2 else iter266)
oprot.writeListEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getUnregisteredTimeseries_result)
getUnregisteredTimeseries_result.thrift_spec = (
(0, TType.LIST, 'success', (TType.STRING, 'UTF8', False), None, ), # 0
)
class pullSnapshot_args(object):
"""
Attributes:
- request
"""
def __init__(self, request=None,):
self.request = request
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.request = PullSnapshotRequest()
self.request.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('pullSnapshot_args')
if self.request is not None:
oprot.writeFieldBegin('request', TType.STRUCT, 1)
self.request.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(pullSnapshot_args)
pullSnapshot_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'request', [PullSnapshotRequest, None], None, ), # 1
)
class pullSnapshot_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.STRUCT:
self.success = PullSnapshotResp()
self.success.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('pullSnapshot_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.STRUCT, 0)
self.success.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(pullSnapshot_result)
pullSnapshot_result.thrift_spec = (
(0, TType.STRUCT, 'success', [PullSnapshotResp, None], None, ), # 0
)
class getGroupByExecutor_args(object):
"""
Attributes:
- request
"""
def __init__(self, request=None,):
self.request = request
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.request = GroupByRequest()
self.request.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getGroupByExecutor_args')
if self.request is not None:
oprot.writeFieldBegin('request', TType.STRUCT, 1)
self.request.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getGroupByExecutor_args)
getGroupByExecutor_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'request', [GroupByRequest, None], None, ), # 1
)
class getGroupByExecutor_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.I64:
self.success = iprot.readI64()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getGroupByExecutor_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.I64, 0)
oprot.writeI64(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getGroupByExecutor_result)
getGroupByExecutor_result.thrift_spec = (
(0, TType.I64, 'success', None, None, ), # 0
)
class getGroupByResult_args(object):
"""
Attributes:
- header
- executorId
- startTime
- endTime
"""
def __init__(self, header=None, executorId=None, startTime=None, endTime=None,):
self.header = header
self.executorId = executorId
self.startTime = startTime
self.endTime = endTime
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 3:
if ftype == TType.I64:
self.executorId = iprot.readI64()
else:
iprot.skip(ftype)
elif fid == 4:
if ftype == TType.I64:
self.startTime = iprot.readI64()
else:
iprot.skip(ftype)
elif fid == 5:
if ftype == TType.I64:
self.endTime = iprot.readI64()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getGroupByResult_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.executorId is not None:
oprot.writeFieldBegin('executorId', TType.I64, 3)
oprot.writeI64(self.executorId)
oprot.writeFieldEnd()
if self.startTime is not None:
oprot.writeFieldBegin('startTime', TType.I64, 4)
oprot.writeI64(self.startTime)
oprot.writeFieldEnd()
if self.endTime is not None:
oprot.writeFieldBegin('endTime', TType.I64, 5)
oprot.writeI64(self.endTime)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getGroupByResult_args)
getGroupByResult_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
None, # 2
(3, TType.I64, 'executorId', None, None, ), # 3
(4, TType.I64, 'startTime', None, None, ), # 4
(5, TType.I64, 'endTime', None, None, ), # 5
)
class getGroupByResult_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.LIST:
self.success = []
(_etype270, _size267) = iprot.readListBegin()
for _i271 in range(_size267):
_elem272 = iprot.readBinary()
self.success.append(_elem272)
iprot.readListEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getGroupByResult_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.LIST, 0)
oprot.writeListBegin(TType.STRING, len(self.success))
for iter273 in self.success:
oprot.writeBinary(iter273)
oprot.writeListEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getGroupByResult_result)
getGroupByResult_result.thrift_spec = (
(0, TType.LIST, 'success', (TType.STRING, 'BINARY', False), None, ), # 0
)
class pullTimeSeriesSchema_args(object):
"""
Attributes:
- request
"""
def __init__(self, request=None,):
self.request = request
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.request = PullSchemaRequest()
self.request.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('pullTimeSeriesSchema_args')
if self.request is not None:
oprot.writeFieldBegin('request', TType.STRUCT, 1)
self.request.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(pullTimeSeriesSchema_args)
pullTimeSeriesSchema_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'request', [PullSchemaRequest, None], None, ), # 1
)
class pullTimeSeriesSchema_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.STRUCT:
self.success = PullSchemaResp()
self.success.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('pullTimeSeriesSchema_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.STRUCT, 0)
self.success.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(pullTimeSeriesSchema_result)
pullTimeSeriesSchema_result.thrift_spec = (
(0, TType.STRUCT, 'success', [PullSchemaResp, None], None, ), # 0
)
class pullMeasurementSchema_args(object):
"""
Attributes:
- request
"""
def __init__(self, request=None,):
self.request = request
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.request = PullSchemaRequest()
self.request.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('pullMeasurementSchema_args')
if self.request is not None:
oprot.writeFieldBegin('request', TType.STRUCT, 1)
self.request.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(pullMeasurementSchema_args)
pullMeasurementSchema_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'request', [PullSchemaRequest, None], None, ), # 1
)
class pullMeasurementSchema_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.STRUCT:
self.success = PullSchemaResp()
self.success.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('pullMeasurementSchema_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.STRUCT, 0)
self.success.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(pullMeasurementSchema_result)
pullMeasurementSchema_result.thrift_spec = (
(0, TType.STRUCT, 'success', [PullSchemaResp, None], None, ), # 0
)
class previousFill_args(object):
"""
Attributes:
- request
"""
def __init__(self, request=None,):
self.request = request
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.request = PreviousFillRequest()
self.request.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('previousFill_args')
if self.request is not None:
oprot.writeFieldBegin('request', TType.STRUCT, 1)
self.request.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(previousFill_args)
previousFill_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'request', [PreviousFillRequest, None], None, ), # 1
)
class previousFill_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.STRING:
self.success = iprot.readBinary()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('previousFill_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.STRING, 0)
oprot.writeBinary(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(previousFill_result)
previousFill_result.thrift_spec = (
(0, TType.STRING, 'success', 'BINARY', None, ), # 0
)
class last_args(object):
"""
Attributes:
- request
"""
def __init__(self, request=None,):
self.request = request
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.request = LastQueryRequest()
self.request.read(iprot)
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('last_args')
if self.request is not None:
oprot.writeFieldBegin('request', TType.STRUCT, 1)
self.request.write(oprot)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(last_args)
last_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'request', [LastQueryRequest, None], None, ), # 1
)
class last_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.STRING:
self.success = iprot.readBinary()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('last_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.STRING, 0)
oprot.writeBinary(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(last_result)
last_result.thrift_spec = (
(0, TType.STRING, 'success', 'BINARY', None, ), # 0
)
class getPathCount_args(object):
"""
Attributes:
- header
- pathsToQuery
- level
"""
def __init__(self, header=None, pathsToQuery=None, level=None,):
self.header = header
self.pathsToQuery = pathsToQuery
self.level = level
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.LIST:
self.pathsToQuery = []
(_etype277, _size274) = iprot.readListBegin()
for _i278 in range(_size274):
_elem279 = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
self.pathsToQuery.append(_elem279)
iprot.readListEnd()
else:
iprot.skip(ftype)
elif fid == 3:
if ftype == TType.I32:
self.level = iprot.readI32()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getPathCount_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.pathsToQuery is not None:
oprot.writeFieldBegin('pathsToQuery', TType.LIST, 2)
oprot.writeListBegin(TType.STRING, len(self.pathsToQuery))
for iter280 in self.pathsToQuery:
oprot.writeString(iter280.encode('utf-8') if sys.version_info[0] == 2 else iter280)
oprot.writeListEnd()
oprot.writeFieldEnd()
if self.level is not None:
oprot.writeFieldBegin('level', TType.I32, 3)
oprot.writeI32(self.level)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getPathCount_args)
getPathCount_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.LIST, 'pathsToQuery', (TType.STRING, 'UTF8', False), None, ), # 2
(3, TType.I32, 'level', None, None, ), # 3
)
class getPathCount_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.I32:
self.success = iprot.readI32()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getPathCount_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.I32, 0)
oprot.writeI32(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getPathCount_result)
getPathCount_result.thrift_spec = (
(0, TType.I32, 'success', None, None, ), # 0
)
class getDeviceCount_args(object):
"""
Attributes:
- header
- pathsToQuery
"""
def __init__(self, header=None, pathsToQuery=None,):
self.header = header
self.pathsToQuery = pathsToQuery
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.LIST:
self.pathsToQuery = []
(_etype284, _size281) = iprot.readListBegin()
for _i285 in range(_size281):
_elem286 = iprot.readString().decode('utf-8', errors='replace') if sys.version_info[0] == 2 else iprot.readString()
self.pathsToQuery.append(_elem286)
iprot.readListEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getDeviceCount_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.pathsToQuery is not None:
oprot.writeFieldBegin('pathsToQuery', TType.LIST, 2)
oprot.writeListBegin(TType.STRING, len(self.pathsToQuery))
for iter287 in self.pathsToQuery:
oprot.writeString(iter287.encode('utf-8') if sys.version_info[0] == 2 else iter287)
oprot.writeListEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getDeviceCount_args)
getDeviceCount_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.LIST, 'pathsToQuery', (TType.STRING, 'UTF8', False), None, ), # 2
)
class getDeviceCount_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.I32:
self.success = iprot.readI32()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('getDeviceCount_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.I32, 0)
oprot.writeI32(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(getDeviceCount_result)
getDeviceCount_result.thrift_spec = (
(0, TType.I32, 'success', None, None, ), # 0
)
class onSnapshotApplied_args(object):
"""
Attributes:
- header
- slots
"""
def __init__(self, header=None, slots=None,):
self.header = header
self.slots = slots
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.LIST:
self.slots = []
(_etype291, _size288) = iprot.readListBegin()
for _i292 in range(_size288):
_elem293 = iprot.readI32()
self.slots.append(_elem293)
iprot.readListEnd()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('onSnapshotApplied_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.slots is not None:
oprot.writeFieldBegin('slots', TType.LIST, 2)
oprot.writeListBegin(TType.I32, len(self.slots))
for iter294 in self.slots:
oprot.writeI32(iter294)
oprot.writeListEnd()
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(onSnapshotApplied_args)
onSnapshotApplied_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.LIST, 'slots', (TType.I32, None, False), None, ), # 2
)
class onSnapshotApplied_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.BOOL:
self.success = iprot.readBool()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('onSnapshotApplied_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.BOOL, 0)
oprot.writeBool(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(onSnapshotApplied_result)
onSnapshotApplied_result.thrift_spec = (
(0, TType.BOOL, 'success', None, None, ), # 0
)
class peekNextNotNullValue_args(object):
"""
Attributes:
- header
- executorId
- startTime
- endTime
"""
def __init__(self, header=None, executorId=None, startTime=None, endTime=None,):
self.header = header
self.executorId = executorId
self.startTime = startTime
self.endTime = endTime
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 1:
if ftype == TType.STRUCT:
self.header = RaftNode()
self.header.read(iprot)
else:
iprot.skip(ftype)
elif fid == 2:
if ftype == TType.I64:
self.executorId = iprot.readI64()
else:
iprot.skip(ftype)
elif fid == 3:
if ftype == TType.I64:
self.startTime = iprot.readI64()
else:
iprot.skip(ftype)
elif fid == 4:
if ftype == TType.I64:
self.endTime = iprot.readI64()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('peekNextNotNullValue_args')
if self.header is not None:
oprot.writeFieldBegin('header', TType.STRUCT, 1)
self.header.write(oprot)
oprot.writeFieldEnd()
if self.executorId is not None:
oprot.writeFieldBegin('executorId', TType.I64, 2)
oprot.writeI64(self.executorId)
oprot.writeFieldEnd()
if self.startTime is not None:
oprot.writeFieldBegin('startTime', TType.I64, 3)
oprot.writeI64(self.startTime)
oprot.writeFieldEnd()
if self.endTime is not None:
oprot.writeFieldBegin('endTime', TType.I64, 4)
oprot.writeI64(self.endTime)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(peekNextNotNullValue_args)
peekNextNotNullValue_args.thrift_spec = (
None, # 0
(1, TType.STRUCT, 'header', [RaftNode, None], None, ), # 1
(2, TType.I64, 'executorId', None, None, ), # 2
(3, TType.I64, 'startTime', None, None, ), # 3
(4, TType.I64, 'endTime', None, None, ), # 4
)
class peekNextNotNullValue_result(object):
"""
Attributes:
- success
"""
def __init__(self, success=None,):
self.success = success
def read(self, iprot):
if iprot._fast_decode is not None and isinstance(iprot.trans, TTransport.CReadableTransport) and self.thrift_spec is not None:
iprot._fast_decode(self, iprot, [self.__class__, self.thrift_spec])
return
iprot.readStructBegin()
while True:
(fname, ftype, fid) = iprot.readFieldBegin()
if ftype == TType.STOP:
break
if fid == 0:
if ftype == TType.STRING:
self.success = iprot.readBinary()
else:
iprot.skip(ftype)
else:
iprot.skip(ftype)
iprot.readFieldEnd()
iprot.readStructEnd()
def write(self, oprot):
if oprot._fast_encode is not None and self.thrift_spec is not None:
oprot.trans.write(oprot._fast_encode(self, [self.__class__, self.thrift_spec]))
return
oprot.writeStructBegin('peekNextNotNullValue_result')
if self.success is not None:
oprot.writeFieldBegin('success', TType.STRING, 0)
oprot.writeBinary(self.success)
oprot.writeFieldEnd()
oprot.writeFieldStop()
oprot.writeStructEnd()
def validate(self):
return
def __repr__(self):
L = ['%s=%r' % (key, value)
for key, value in self.__dict__.items()]
return '%s(%s)' % (self.__class__.__name__, ', '.join(L))
def __eq__(self, other):
return isinstance(other, self.__class__) and self.__dict__ == other.__dict__
def __ne__(self, other):
return not (self == other)
all_structs.append(peekNextNotNullValue_result)
peekNextNotNullValue_result.thrift_spec = (
(0, TType.STRING, 'success', 'BINARY', None, ), # 0
)
fix_spec(all_structs)
del all_structs
© 2015 - 2025 Weber Informatics LLC | Privacy Policy