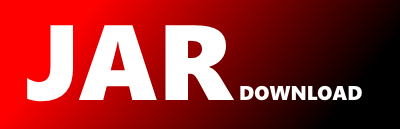
iotdb.thrift.cluster.TSMetaService-remote Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotdb-thrift-cluster
Show all versions of iotdb-thrift-cluster
RPC (Thrift) framework among servers.
#!/usr/bin/env python
#
# Autogenerated by Thrift Compiler (0.14.1)
#
# DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
#
# options string: py
#
import sys
import pprint
if sys.version_info[0] > 2:
from urllib.parse import urlparse
else:
from urlparse import urlparse
from thrift.transport import TTransport, TSocket, TSSLSocket, THttpClient
from thrift.protocol.TBinaryProtocol import TBinaryProtocol
from iotdb.thrift.cluster import TSMetaService
from iotdb.thrift.cluster.ttypes import *
if len(sys.argv) <= 1 or sys.argv[1] == '--help':
print('')
print('Usage: ' + sys.argv[0] + ' [-h host[:port]] [-u url] [-f[ramed]] [-s[sl]] [-novalidate] [-ca_certs certs] [-keyfile keyfile] [-certfile certfile] function [arg1 [arg2...]]')
print('')
print('Functions:')
print(' AddNodeResponse addNode(Node node, StartUpStatus startUpStatus)')
print(' CheckStatusResponse checkStatus(StartUpStatus startUpStatus)')
print(' long removeNode(Node node)')
print(' void exile(string removeNodeLog)')
print(' TNodeStatus queryNodeStatus()')
print(' Node checkAlive()')
print(' string collectMigrationStatus()')
print(' void handshake(Node sender)')
print(' HeartBeatResponse sendHeartbeat(HeartBeatRequest request)')
print(' long startElection(ElectionRequest request)')
print(' long appendEntries(AppendEntriesRequest request)')
print(' long appendEntry(AppendEntryRequest request)')
print(' void sendSnapshot(SendSnapshotRequest request)')
print(' TSStatus executeNonQueryPlan(ExecutNonQueryReq request)')
print(' RequestCommitIndexResponse requestCommitIndex(RaftNode header)')
print(' string readFile(string filePath, long offset, int length)')
print(' bool matchTerm(long index, long term, RaftNode header)')
print(' void removeHardLink(string hardLinkPath)')
print('')
sys.exit(0)
pp = pprint.PrettyPrinter(indent=2)
host = 'localhost'
port = 9090
uri = ''
framed = False
ssl = False
validate = True
ca_certs = None
keyfile = None
certfile = None
http = False
argi = 1
if sys.argv[argi] == '-h':
parts = sys.argv[argi + 1].split(':')
host = parts[0]
if len(parts) > 1:
port = int(parts[1])
argi += 2
if sys.argv[argi] == '-u':
url = urlparse(sys.argv[argi + 1])
parts = url[1].split(':')
host = parts[0]
if len(parts) > 1:
port = int(parts[1])
else:
port = 80
uri = url[2]
if url[4]:
uri += '?%s' % url[4]
http = True
argi += 2
if sys.argv[argi] == '-f' or sys.argv[argi] == '-framed':
framed = True
argi += 1
if sys.argv[argi] == '-s' or sys.argv[argi] == '-ssl':
ssl = True
argi += 1
if sys.argv[argi] == '-novalidate':
validate = False
argi += 1
if sys.argv[argi] == '-ca_certs':
ca_certs = sys.argv[argi+1]
argi += 2
if sys.argv[argi] == '-keyfile':
keyfile = sys.argv[argi+1]
argi += 2
if sys.argv[argi] == '-certfile':
certfile = sys.argv[argi+1]
argi += 2
cmd = sys.argv[argi]
args = sys.argv[argi + 1:]
if http:
transport = THttpClient.THttpClient(host, port, uri)
else:
if ssl:
socket = TSSLSocket.TSSLSocket(host, port, validate=validate, ca_certs=ca_certs, keyfile=keyfile, certfile=certfile)
else:
socket = TSocket.TSocket(host, port)
if framed:
transport = TTransport.TFramedTransport(socket)
else:
transport = TTransport.TBufferedTransport(socket)
protocol = TBinaryProtocol(transport)
client = TSMetaService.Client(protocol)
transport.open()
if cmd == 'addNode':
if len(args) != 2:
print('addNode requires 2 args')
sys.exit(1)
pp.pprint(client.addNode(eval(args[0]), eval(args[1]),))
elif cmd == 'checkStatus':
if len(args) != 1:
print('checkStatus requires 1 args')
sys.exit(1)
pp.pprint(client.checkStatus(eval(args[0]),))
elif cmd == 'removeNode':
if len(args) != 1:
print('removeNode requires 1 args')
sys.exit(1)
pp.pprint(client.removeNode(eval(args[0]),))
elif cmd == 'exile':
if len(args) != 1:
print('exile requires 1 args')
sys.exit(1)
pp.pprint(client.exile(args[0],))
elif cmd == 'queryNodeStatus':
if len(args) != 0:
print('queryNodeStatus requires 0 args')
sys.exit(1)
pp.pprint(client.queryNodeStatus())
elif cmd == 'checkAlive':
if len(args) != 0:
print('checkAlive requires 0 args')
sys.exit(1)
pp.pprint(client.checkAlive())
elif cmd == 'collectMigrationStatus':
if len(args) != 0:
print('collectMigrationStatus requires 0 args')
sys.exit(1)
pp.pprint(client.collectMigrationStatus())
elif cmd == 'handshake':
if len(args) != 1:
print('handshake requires 1 args')
sys.exit(1)
pp.pprint(client.handshake(eval(args[0]),))
elif cmd == 'sendHeartbeat':
if len(args) != 1:
print('sendHeartbeat requires 1 args')
sys.exit(1)
pp.pprint(client.sendHeartbeat(eval(args[0]),))
elif cmd == 'startElection':
if len(args) != 1:
print('startElection requires 1 args')
sys.exit(1)
pp.pprint(client.startElection(eval(args[0]),))
elif cmd == 'appendEntries':
if len(args) != 1:
print('appendEntries requires 1 args')
sys.exit(1)
pp.pprint(client.appendEntries(eval(args[0]),))
elif cmd == 'appendEntry':
if len(args) != 1:
print('appendEntry requires 1 args')
sys.exit(1)
pp.pprint(client.appendEntry(eval(args[0]),))
elif cmd == 'sendSnapshot':
if len(args) != 1:
print('sendSnapshot requires 1 args')
sys.exit(1)
pp.pprint(client.sendSnapshot(eval(args[0]),))
elif cmd == 'executeNonQueryPlan':
if len(args) != 1:
print('executeNonQueryPlan requires 1 args')
sys.exit(1)
pp.pprint(client.executeNonQueryPlan(eval(args[0]),))
elif cmd == 'requestCommitIndex':
if len(args) != 1:
print('requestCommitIndex requires 1 args')
sys.exit(1)
pp.pprint(client.requestCommitIndex(eval(args[0]),))
elif cmd == 'readFile':
if len(args) != 3:
print('readFile requires 3 args')
sys.exit(1)
pp.pprint(client.readFile(args[0], eval(args[1]), eval(args[2]),))
elif cmd == 'matchTerm':
if len(args) != 3:
print('matchTerm requires 3 args')
sys.exit(1)
pp.pprint(client.matchTerm(eval(args[0]), eval(args[1]), eval(args[2]),))
elif cmd == 'removeHardLink':
if len(args) != 1:
print('removeHardLink requires 1 args')
sys.exit(1)
pp.pprint(client.removeHardLink(args[0],))
else:
print('Unrecognized method %s' % cmd)
sys.exit(1)
transport.close()
© 2015 - 2025 Weber Informatics LLC | Privacy Policy