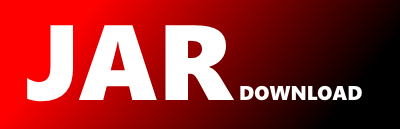
org.apache.iotdb.cluster.rpc.thrift.AppendEntriesRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotdb-thrift-cluster
Show all versions of iotdb-thrift-cluster
RPC (Thrift) framework among servers.
/**
* Autogenerated by Thrift Compiler (0.14.1)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package org.apache.iotdb.cluster.rpc.thrift;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked", "unused"})
@javax.annotation.Generated(value = "Autogenerated by Thrift Compiler (0.14.1)", date = "2022-07-31")
public class AppendEntriesRequest implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("AppendEntriesRequest");
private static final org.apache.thrift.protocol.TField TERM_FIELD_DESC = new org.apache.thrift.protocol.TField("term", org.apache.thrift.protocol.TType.I64, (short)1);
private static final org.apache.thrift.protocol.TField LEADER_FIELD_DESC = new org.apache.thrift.protocol.TField("leader", org.apache.thrift.protocol.TType.STRUCT, (short)2);
private static final org.apache.thrift.protocol.TField ENTRIES_FIELD_DESC = new org.apache.thrift.protocol.TField("entries", org.apache.thrift.protocol.TType.LIST, (short)3);
private static final org.apache.thrift.protocol.TField PREV_LOG_INDEX_FIELD_DESC = new org.apache.thrift.protocol.TField("prevLogIndex", org.apache.thrift.protocol.TType.I64, (short)4);
private static final org.apache.thrift.protocol.TField PREV_LOG_TERM_FIELD_DESC = new org.apache.thrift.protocol.TField("prevLogTerm", org.apache.thrift.protocol.TType.I64, (short)5);
private static final org.apache.thrift.protocol.TField LEADER_COMMIT_FIELD_DESC = new org.apache.thrift.protocol.TField("leaderCommit", org.apache.thrift.protocol.TType.I64, (short)6);
private static final org.apache.thrift.protocol.TField HEADER_FIELD_DESC = new org.apache.thrift.protocol.TField("header", org.apache.thrift.protocol.TType.STRUCT, (short)7);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new AppendEntriesRequestStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new AppendEntriesRequestTupleSchemeFactory();
public long term; // required
public @org.apache.thrift.annotation.Nullable Node leader; // required
public @org.apache.thrift.annotation.Nullable java.util.List entries; // required
public long prevLogIndex; // required
public long prevLogTerm; // required
public long leaderCommit; // required
public @org.apache.thrift.annotation.Nullable RaftNode header; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
TERM((short)1, "term"),
LEADER((short)2, "leader"),
ENTRIES((short)3, "entries"),
PREV_LOG_INDEX((short)4, "prevLogIndex"),
PREV_LOG_TERM((short)5, "prevLogTerm"),
LEADER_COMMIT((short)6, "leaderCommit"),
HEADER((short)7, "header");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // TERM
return TERM;
case 2: // LEADER
return LEADER;
case 3: // ENTRIES
return ENTRIES;
case 4: // PREV_LOG_INDEX
return PREV_LOG_INDEX;
case 5: // PREV_LOG_TERM
return PREV_LOG_TERM;
case 6: // LEADER_COMMIT
return LEADER_COMMIT;
case 7: // HEADER
return HEADER;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __TERM_ISSET_ID = 0;
private static final int __PREVLOGINDEX_ISSET_ID = 1;
private static final int __PREVLOGTERM_ISSET_ID = 2;
private static final int __LEADERCOMMIT_ISSET_ID = 3;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.HEADER};
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.TERM, new org.apache.thrift.meta_data.FieldMetaData("term", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.LEADER, new org.apache.thrift.meta_data.FieldMetaData("leader", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "Node")));
tmpMap.put(_Fields.ENTRIES, new org.apache.thrift.meta_data.FieldMetaData("entries", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true))));
tmpMap.put(_Fields.PREV_LOG_INDEX, new org.apache.thrift.meta_data.FieldMetaData("prevLogIndex", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.PREV_LOG_TERM, new org.apache.thrift.meta_data.FieldMetaData("prevLogTerm", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.LEADER_COMMIT, new org.apache.thrift.meta_data.FieldMetaData("leaderCommit", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.HEADER, new org.apache.thrift.meta_data.FieldMetaData("header", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "RaftNode")));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(AppendEntriesRequest.class, metaDataMap);
}
public AppendEntriesRequest() {
}
public AppendEntriesRequest(
long term,
Node leader,
java.util.List entries,
long prevLogIndex,
long prevLogTerm,
long leaderCommit)
{
this();
this.term = term;
setTermIsSet(true);
this.leader = leader;
this.entries = entries;
this.prevLogIndex = prevLogIndex;
setPrevLogIndexIsSet(true);
this.prevLogTerm = prevLogTerm;
setPrevLogTermIsSet(true);
this.leaderCommit = leaderCommit;
setLeaderCommitIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public AppendEntriesRequest(AppendEntriesRequest other) {
__isset_bitfield = other.__isset_bitfield;
this.term = other.term;
if (other.isSetLeader()) {
this.leader = new Node(other.leader);
}
if (other.isSetEntries()) {
java.util.List __this__entries = new java.util.ArrayList(other.entries);
this.entries = __this__entries;
}
this.prevLogIndex = other.prevLogIndex;
this.prevLogTerm = other.prevLogTerm;
this.leaderCommit = other.leaderCommit;
if (other.isSetHeader()) {
this.header = new RaftNode(other.header);
}
}
public AppendEntriesRequest deepCopy() {
return new AppendEntriesRequest(this);
}
@Override
public void clear() {
setTermIsSet(false);
this.term = 0;
this.leader = null;
this.entries = null;
setPrevLogIndexIsSet(false);
this.prevLogIndex = 0;
setPrevLogTermIsSet(false);
this.prevLogTerm = 0;
setLeaderCommitIsSet(false);
this.leaderCommit = 0;
this.header = null;
}
public long getTerm() {
return this.term;
}
public AppendEntriesRequest setTerm(long term) {
this.term = term;
setTermIsSet(true);
return this;
}
public void unsetTerm() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __TERM_ISSET_ID);
}
/** Returns true if field term is set (has been assigned a value) and false otherwise */
public boolean isSetTerm() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __TERM_ISSET_ID);
}
public void setTermIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __TERM_ISSET_ID, value);
}
@org.apache.thrift.annotation.Nullable
public Node getLeader() {
return this.leader;
}
public AppendEntriesRequest setLeader(@org.apache.thrift.annotation.Nullable Node leader) {
this.leader = leader;
return this;
}
public void unsetLeader() {
this.leader = null;
}
/** Returns true if field leader is set (has been assigned a value) and false otherwise */
public boolean isSetLeader() {
return this.leader != null;
}
public void setLeaderIsSet(boolean value) {
if (!value) {
this.leader = null;
}
}
public int getEntriesSize() {
return (this.entries == null) ? 0 : this.entries.size();
}
@org.apache.thrift.annotation.Nullable
public java.util.Iterator getEntriesIterator() {
return (this.entries == null) ? null : this.entries.iterator();
}
public void addToEntries(java.nio.ByteBuffer elem) {
if (this.entries == null) {
this.entries = new java.util.ArrayList();
}
this.entries.add(elem);
}
@org.apache.thrift.annotation.Nullable
public java.util.List getEntries() {
return this.entries;
}
public AppendEntriesRequest setEntries(@org.apache.thrift.annotation.Nullable java.util.List entries) {
this.entries = entries;
return this;
}
public void unsetEntries() {
this.entries = null;
}
/** Returns true if field entries is set (has been assigned a value) and false otherwise */
public boolean isSetEntries() {
return this.entries != null;
}
public void setEntriesIsSet(boolean value) {
if (!value) {
this.entries = null;
}
}
public long getPrevLogIndex() {
return this.prevLogIndex;
}
public AppendEntriesRequest setPrevLogIndex(long prevLogIndex) {
this.prevLogIndex = prevLogIndex;
setPrevLogIndexIsSet(true);
return this;
}
public void unsetPrevLogIndex() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __PREVLOGINDEX_ISSET_ID);
}
/** Returns true if field prevLogIndex is set (has been assigned a value) and false otherwise */
public boolean isSetPrevLogIndex() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __PREVLOGINDEX_ISSET_ID);
}
public void setPrevLogIndexIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __PREVLOGINDEX_ISSET_ID, value);
}
public long getPrevLogTerm() {
return this.prevLogTerm;
}
public AppendEntriesRequest setPrevLogTerm(long prevLogTerm) {
this.prevLogTerm = prevLogTerm;
setPrevLogTermIsSet(true);
return this;
}
public void unsetPrevLogTerm() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __PREVLOGTERM_ISSET_ID);
}
/** Returns true if field prevLogTerm is set (has been assigned a value) and false otherwise */
public boolean isSetPrevLogTerm() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __PREVLOGTERM_ISSET_ID);
}
public void setPrevLogTermIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __PREVLOGTERM_ISSET_ID, value);
}
public long getLeaderCommit() {
return this.leaderCommit;
}
public AppendEntriesRequest setLeaderCommit(long leaderCommit) {
this.leaderCommit = leaderCommit;
setLeaderCommitIsSet(true);
return this;
}
public void unsetLeaderCommit() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __LEADERCOMMIT_ISSET_ID);
}
/** Returns true if field leaderCommit is set (has been assigned a value) and false otherwise */
public boolean isSetLeaderCommit() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __LEADERCOMMIT_ISSET_ID);
}
public void setLeaderCommitIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __LEADERCOMMIT_ISSET_ID, value);
}
@org.apache.thrift.annotation.Nullable
public RaftNode getHeader() {
return this.header;
}
public AppendEntriesRequest setHeader(@org.apache.thrift.annotation.Nullable RaftNode header) {
this.header = header;
return this;
}
public void unsetHeader() {
this.header = null;
}
/** Returns true if field header is set (has been assigned a value) and false otherwise */
public boolean isSetHeader() {
return this.header != null;
}
public void setHeaderIsSet(boolean value) {
if (!value) {
this.header = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case TERM:
if (value == null) {
unsetTerm();
} else {
setTerm((java.lang.Long)value);
}
break;
case LEADER:
if (value == null) {
unsetLeader();
} else {
setLeader((Node)value);
}
break;
case ENTRIES:
if (value == null) {
unsetEntries();
} else {
setEntries((java.util.List)value);
}
break;
case PREV_LOG_INDEX:
if (value == null) {
unsetPrevLogIndex();
} else {
setPrevLogIndex((java.lang.Long)value);
}
break;
case PREV_LOG_TERM:
if (value == null) {
unsetPrevLogTerm();
} else {
setPrevLogTerm((java.lang.Long)value);
}
break;
case LEADER_COMMIT:
if (value == null) {
unsetLeaderCommit();
} else {
setLeaderCommit((java.lang.Long)value);
}
break;
case HEADER:
if (value == null) {
unsetHeader();
} else {
setHeader((RaftNode)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case TERM:
return getTerm();
case LEADER:
return getLeader();
case ENTRIES:
return getEntries();
case PREV_LOG_INDEX:
return getPrevLogIndex();
case PREV_LOG_TERM:
return getPrevLogTerm();
case LEADER_COMMIT:
return getLeaderCommit();
case HEADER:
return getHeader();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case TERM:
return isSetTerm();
case LEADER:
return isSetLeader();
case ENTRIES:
return isSetEntries();
case PREV_LOG_INDEX:
return isSetPrevLogIndex();
case PREV_LOG_TERM:
return isSetPrevLogTerm();
case LEADER_COMMIT:
return isSetLeaderCommit();
case HEADER:
return isSetHeader();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof AppendEntriesRequest)
return this.equals((AppendEntriesRequest)that);
return false;
}
public boolean equals(AppendEntriesRequest that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_term = true;
boolean that_present_term = true;
if (this_present_term || that_present_term) {
if (!(this_present_term && that_present_term))
return false;
if (this.term != that.term)
return false;
}
boolean this_present_leader = true && this.isSetLeader();
boolean that_present_leader = true && that.isSetLeader();
if (this_present_leader || that_present_leader) {
if (!(this_present_leader && that_present_leader))
return false;
if (!this.leader.equals(that.leader))
return false;
}
boolean this_present_entries = true && this.isSetEntries();
boolean that_present_entries = true && that.isSetEntries();
if (this_present_entries || that_present_entries) {
if (!(this_present_entries && that_present_entries))
return false;
if (!this.entries.equals(that.entries))
return false;
}
boolean this_present_prevLogIndex = true;
boolean that_present_prevLogIndex = true;
if (this_present_prevLogIndex || that_present_prevLogIndex) {
if (!(this_present_prevLogIndex && that_present_prevLogIndex))
return false;
if (this.prevLogIndex != that.prevLogIndex)
return false;
}
boolean this_present_prevLogTerm = true;
boolean that_present_prevLogTerm = true;
if (this_present_prevLogTerm || that_present_prevLogTerm) {
if (!(this_present_prevLogTerm && that_present_prevLogTerm))
return false;
if (this.prevLogTerm != that.prevLogTerm)
return false;
}
boolean this_present_leaderCommit = true;
boolean that_present_leaderCommit = true;
if (this_present_leaderCommit || that_present_leaderCommit) {
if (!(this_present_leaderCommit && that_present_leaderCommit))
return false;
if (this.leaderCommit != that.leaderCommit)
return false;
}
boolean this_present_header = true && this.isSetHeader();
boolean that_present_header = true && that.isSetHeader();
if (this_present_header || that_present_header) {
if (!(this_present_header && that_present_header))
return false;
if (!this.header.equals(that.header))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(term);
hashCode = hashCode * 8191 + ((isSetLeader()) ? 131071 : 524287);
if (isSetLeader())
hashCode = hashCode * 8191 + leader.hashCode();
hashCode = hashCode * 8191 + ((isSetEntries()) ? 131071 : 524287);
if (isSetEntries())
hashCode = hashCode * 8191 + entries.hashCode();
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(prevLogIndex);
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(prevLogTerm);
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(leaderCommit);
hashCode = hashCode * 8191 + ((isSetHeader()) ? 131071 : 524287);
if (isSetHeader())
hashCode = hashCode * 8191 + header.hashCode();
return hashCode;
}
@Override
public int compareTo(AppendEntriesRequest other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetTerm(), other.isSetTerm());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetTerm()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.term, other.term);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetLeader(), other.isSetLeader());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetLeader()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.leader, other.leader);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetEntries(), other.isSetEntries());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetEntries()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.entries, other.entries);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetPrevLogIndex(), other.isSetPrevLogIndex());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetPrevLogIndex()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.prevLogIndex, other.prevLogIndex);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetPrevLogTerm(), other.isSetPrevLogTerm());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetPrevLogTerm()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.prevLogTerm, other.prevLogTerm);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetLeaderCommit(), other.isSetLeaderCommit());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetLeaderCommit()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.leaderCommit, other.leaderCommit);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetHeader(), other.isSetHeader());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetHeader()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.header, other.header);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("AppendEntriesRequest(");
boolean first = true;
sb.append("term:");
sb.append(this.term);
first = false;
if (!first) sb.append(", ");
sb.append("leader:");
if (this.leader == null) {
sb.append("null");
} else {
sb.append(this.leader);
}
first = false;
if (!first) sb.append(", ");
sb.append("entries:");
if (this.entries == null) {
sb.append("null");
} else {
org.apache.thrift.TBaseHelper.toString(this.entries, sb);
}
first = false;
if (!first) sb.append(", ");
sb.append("prevLogIndex:");
sb.append(this.prevLogIndex);
first = false;
if (!first) sb.append(", ");
sb.append("prevLogTerm:");
sb.append(this.prevLogTerm);
first = false;
if (!first) sb.append(", ");
sb.append("leaderCommit:");
sb.append(this.leaderCommit);
first = false;
if (isSetHeader()) {
if (!first) sb.append(", ");
sb.append("header:");
if (this.header == null) {
sb.append("null");
} else {
sb.append(this.header);
}
first = false;
}
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// alas, we cannot check 'term' because it's a primitive and you chose the non-beans generator.
if (leader == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'leader' was not present! Struct: " + toString());
}
if (entries == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'entries' was not present! Struct: " + toString());
}
// alas, we cannot check 'prevLogIndex' because it's a primitive and you chose the non-beans generator.
// alas, we cannot check 'prevLogTerm' because it's a primitive and you chose the non-beans generator.
// alas, we cannot check 'leaderCommit' because it's a primitive and you chose the non-beans generator.
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
// it doesn't seem like you should have to do this, but java serialization is wacky, and doesn't call the default constructor.
__isset_bitfield = 0;
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class AppendEntriesRequestStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public AppendEntriesRequestStandardScheme getScheme() {
return new AppendEntriesRequestStandardScheme();
}
}
private static class AppendEntriesRequestStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, AppendEntriesRequest struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // TERM
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.term = iprot.readI64();
struct.setTermIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 2: // LEADER
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.leader = new Node();
struct.leader.read(iprot);
struct.setLeaderIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 3: // ENTRIES
if (schemeField.type == org.apache.thrift.protocol.TType.LIST) {
{
org.apache.thrift.protocol.TList _list0 = iprot.readListBegin();
struct.entries = new java.util.ArrayList(_list0.size);
@org.apache.thrift.annotation.Nullable java.nio.ByteBuffer _elem1;
for (int _i2 = 0; _i2 < _list0.size; ++_i2)
{
_elem1 = iprot.readBinary();
struct.entries.add(_elem1);
}
iprot.readListEnd();
}
struct.setEntriesIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 4: // PREV_LOG_INDEX
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.prevLogIndex = iprot.readI64();
struct.setPrevLogIndexIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 5: // PREV_LOG_TERM
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.prevLogTerm = iprot.readI64();
struct.setPrevLogTermIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 6: // LEADER_COMMIT
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.leaderCommit = iprot.readI64();
struct.setLeaderCommitIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 7: // HEADER
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.header = new RaftNode();
struct.header.read(iprot);
struct.setHeaderIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
if (!struct.isSetTerm()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'term' was not found in serialized data! Struct: " + toString());
}
if (!struct.isSetPrevLogIndex()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'prevLogIndex' was not found in serialized data! Struct: " + toString());
}
if (!struct.isSetPrevLogTerm()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'prevLogTerm' was not found in serialized data! Struct: " + toString());
}
if (!struct.isSetLeaderCommit()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'leaderCommit' was not found in serialized data! Struct: " + toString());
}
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, AppendEntriesRequest struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
oprot.writeFieldBegin(TERM_FIELD_DESC);
oprot.writeI64(struct.term);
oprot.writeFieldEnd();
if (struct.leader != null) {
oprot.writeFieldBegin(LEADER_FIELD_DESC);
struct.leader.write(oprot);
oprot.writeFieldEnd();
}
if (struct.entries != null) {
oprot.writeFieldBegin(ENTRIES_FIELD_DESC);
{
oprot.writeListBegin(new org.apache.thrift.protocol.TList(org.apache.thrift.protocol.TType.STRING, struct.entries.size()));
for (java.nio.ByteBuffer _iter3 : struct.entries)
{
oprot.writeBinary(_iter3);
}
oprot.writeListEnd();
}
oprot.writeFieldEnd();
}
oprot.writeFieldBegin(PREV_LOG_INDEX_FIELD_DESC);
oprot.writeI64(struct.prevLogIndex);
oprot.writeFieldEnd();
oprot.writeFieldBegin(PREV_LOG_TERM_FIELD_DESC);
oprot.writeI64(struct.prevLogTerm);
oprot.writeFieldEnd();
oprot.writeFieldBegin(LEADER_COMMIT_FIELD_DESC);
oprot.writeI64(struct.leaderCommit);
oprot.writeFieldEnd();
if (struct.header != null) {
if (struct.isSetHeader()) {
oprot.writeFieldBegin(HEADER_FIELD_DESC);
struct.header.write(oprot);
oprot.writeFieldEnd();
}
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class AppendEntriesRequestTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public AppendEntriesRequestTupleScheme getScheme() {
return new AppendEntriesRequestTupleScheme();
}
}
private static class AppendEntriesRequestTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, AppendEntriesRequest struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
oprot.writeI64(struct.term);
struct.leader.write(oprot);
{
oprot.writeI32(struct.entries.size());
for (java.nio.ByteBuffer _iter4 : struct.entries)
{
oprot.writeBinary(_iter4);
}
}
oprot.writeI64(struct.prevLogIndex);
oprot.writeI64(struct.prevLogTerm);
oprot.writeI64(struct.leaderCommit);
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetHeader()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetHeader()) {
struct.header.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, AppendEntriesRequest struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
struct.term = iprot.readI64();
struct.setTermIsSet(true);
struct.leader = new Node();
struct.leader.read(iprot);
struct.setLeaderIsSet(true);
{
org.apache.thrift.protocol.TList _list5 = iprot.readListBegin(org.apache.thrift.protocol.TType.STRING);
struct.entries = new java.util.ArrayList(_list5.size);
@org.apache.thrift.annotation.Nullable java.nio.ByteBuffer _elem6;
for (int _i7 = 0; _i7 < _list5.size; ++_i7)
{
_elem6 = iprot.readBinary();
struct.entries.add(_elem6);
}
}
struct.setEntriesIsSet(true);
struct.prevLogIndex = iprot.readI64();
struct.setPrevLogIndexIsSet(true);
struct.prevLogTerm = iprot.readI64();
struct.setPrevLogTermIsSet(true);
struct.leaderCommit = iprot.readI64();
struct.setLeaderCommitIsSet(true);
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.header = new RaftNode();
struct.header.read(iprot);
struct.setHeaderIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy