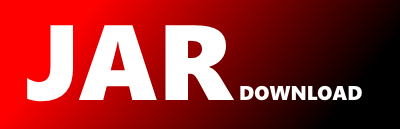
org.apache.iotdb.cluster.rpc.thrift.AppendEntryRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotdb-thrift-cluster
Show all versions of iotdb-thrift-cluster
RPC (Thrift) framework among servers.
/**
* Autogenerated by Thrift Compiler (0.14.1)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package org.apache.iotdb.cluster.rpc.thrift;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked", "unused"})
@javax.annotation.Generated(value = "Autogenerated by Thrift Compiler (0.14.1)", date = "2022-07-31")
public class AppendEntryRequest implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("AppendEntryRequest");
private static final org.apache.thrift.protocol.TField TERM_FIELD_DESC = new org.apache.thrift.protocol.TField("term", org.apache.thrift.protocol.TType.I64, (short)1);
private static final org.apache.thrift.protocol.TField LEADER_FIELD_DESC = new org.apache.thrift.protocol.TField("leader", org.apache.thrift.protocol.TType.STRUCT, (short)2);
private static final org.apache.thrift.protocol.TField PREV_LOG_INDEX_FIELD_DESC = new org.apache.thrift.protocol.TField("prevLogIndex", org.apache.thrift.protocol.TType.I64, (short)3);
private static final org.apache.thrift.protocol.TField PREV_LOG_TERM_FIELD_DESC = new org.apache.thrift.protocol.TField("prevLogTerm", org.apache.thrift.protocol.TType.I64, (short)4);
private static final org.apache.thrift.protocol.TField LEADER_COMMIT_FIELD_DESC = new org.apache.thrift.protocol.TField("leaderCommit", org.apache.thrift.protocol.TType.I64, (short)5);
private static final org.apache.thrift.protocol.TField ENTRY_FIELD_DESC = new org.apache.thrift.protocol.TField("entry", org.apache.thrift.protocol.TType.STRING, (short)6);
private static final org.apache.thrift.protocol.TField HEADER_FIELD_DESC = new org.apache.thrift.protocol.TField("header", org.apache.thrift.protocol.TType.STRUCT, (short)7);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new AppendEntryRequestStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new AppendEntryRequestTupleSchemeFactory();
public long term; // required
public @org.apache.thrift.annotation.Nullable Node leader; // required
public long prevLogIndex; // required
public long prevLogTerm; // required
public long leaderCommit; // required
public @org.apache.thrift.annotation.Nullable java.nio.ByteBuffer entry; // required
public @org.apache.thrift.annotation.Nullable RaftNode header; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
TERM((short)1, "term"),
LEADER((short)2, "leader"),
PREV_LOG_INDEX((short)3, "prevLogIndex"),
PREV_LOG_TERM((short)4, "prevLogTerm"),
LEADER_COMMIT((short)5, "leaderCommit"),
ENTRY((short)6, "entry"),
HEADER((short)7, "header");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // TERM
return TERM;
case 2: // LEADER
return LEADER;
case 3: // PREV_LOG_INDEX
return PREV_LOG_INDEX;
case 4: // PREV_LOG_TERM
return PREV_LOG_TERM;
case 5: // LEADER_COMMIT
return LEADER_COMMIT;
case 6: // ENTRY
return ENTRY;
case 7: // HEADER
return HEADER;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __TERM_ISSET_ID = 0;
private static final int __PREVLOGINDEX_ISSET_ID = 1;
private static final int __PREVLOGTERM_ISSET_ID = 2;
private static final int __LEADERCOMMIT_ISSET_ID = 3;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.HEADER};
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.TERM, new org.apache.thrift.meta_data.FieldMetaData("term", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.LEADER, new org.apache.thrift.meta_data.FieldMetaData("leader", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "Node")));
tmpMap.put(_Fields.PREV_LOG_INDEX, new org.apache.thrift.meta_data.FieldMetaData("prevLogIndex", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.PREV_LOG_TERM, new org.apache.thrift.meta_data.FieldMetaData("prevLogTerm", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.LEADER_COMMIT, new org.apache.thrift.meta_data.FieldMetaData("leaderCommit", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.ENTRY, new org.apache.thrift.meta_data.FieldMetaData("entry", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.HEADER, new org.apache.thrift.meta_data.FieldMetaData("header", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "RaftNode")));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(AppendEntryRequest.class, metaDataMap);
}
public AppendEntryRequest() {
}
public AppendEntryRequest(
long term,
Node leader,
long prevLogIndex,
long prevLogTerm,
long leaderCommit,
java.nio.ByteBuffer entry)
{
this();
this.term = term;
setTermIsSet(true);
this.leader = leader;
this.prevLogIndex = prevLogIndex;
setPrevLogIndexIsSet(true);
this.prevLogTerm = prevLogTerm;
setPrevLogTermIsSet(true);
this.leaderCommit = leaderCommit;
setLeaderCommitIsSet(true);
this.entry = org.apache.thrift.TBaseHelper.copyBinary(entry);
}
/**
* Performs a deep copy on other.
*/
public AppendEntryRequest(AppendEntryRequest other) {
__isset_bitfield = other.__isset_bitfield;
this.term = other.term;
if (other.isSetLeader()) {
this.leader = new Node(other.leader);
}
this.prevLogIndex = other.prevLogIndex;
this.prevLogTerm = other.prevLogTerm;
this.leaderCommit = other.leaderCommit;
if (other.isSetEntry()) {
this.entry = org.apache.thrift.TBaseHelper.copyBinary(other.entry);
}
if (other.isSetHeader()) {
this.header = new RaftNode(other.header);
}
}
public AppendEntryRequest deepCopy() {
return new AppendEntryRequest(this);
}
@Override
public void clear() {
setTermIsSet(false);
this.term = 0;
this.leader = null;
setPrevLogIndexIsSet(false);
this.prevLogIndex = 0;
setPrevLogTermIsSet(false);
this.prevLogTerm = 0;
setLeaderCommitIsSet(false);
this.leaderCommit = 0;
this.entry = null;
this.header = null;
}
public long getTerm() {
return this.term;
}
public AppendEntryRequest setTerm(long term) {
this.term = term;
setTermIsSet(true);
return this;
}
public void unsetTerm() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __TERM_ISSET_ID);
}
/** Returns true if field term is set (has been assigned a value) and false otherwise */
public boolean isSetTerm() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __TERM_ISSET_ID);
}
public void setTermIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __TERM_ISSET_ID, value);
}
@org.apache.thrift.annotation.Nullable
public Node getLeader() {
return this.leader;
}
public AppendEntryRequest setLeader(@org.apache.thrift.annotation.Nullable Node leader) {
this.leader = leader;
return this;
}
public void unsetLeader() {
this.leader = null;
}
/** Returns true if field leader is set (has been assigned a value) and false otherwise */
public boolean isSetLeader() {
return this.leader != null;
}
public void setLeaderIsSet(boolean value) {
if (!value) {
this.leader = null;
}
}
public long getPrevLogIndex() {
return this.prevLogIndex;
}
public AppendEntryRequest setPrevLogIndex(long prevLogIndex) {
this.prevLogIndex = prevLogIndex;
setPrevLogIndexIsSet(true);
return this;
}
public void unsetPrevLogIndex() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __PREVLOGINDEX_ISSET_ID);
}
/** Returns true if field prevLogIndex is set (has been assigned a value) and false otherwise */
public boolean isSetPrevLogIndex() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __PREVLOGINDEX_ISSET_ID);
}
public void setPrevLogIndexIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __PREVLOGINDEX_ISSET_ID, value);
}
public long getPrevLogTerm() {
return this.prevLogTerm;
}
public AppendEntryRequest setPrevLogTerm(long prevLogTerm) {
this.prevLogTerm = prevLogTerm;
setPrevLogTermIsSet(true);
return this;
}
public void unsetPrevLogTerm() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __PREVLOGTERM_ISSET_ID);
}
/** Returns true if field prevLogTerm is set (has been assigned a value) and false otherwise */
public boolean isSetPrevLogTerm() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __PREVLOGTERM_ISSET_ID);
}
public void setPrevLogTermIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __PREVLOGTERM_ISSET_ID, value);
}
public long getLeaderCommit() {
return this.leaderCommit;
}
public AppendEntryRequest setLeaderCommit(long leaderCommit) {
this.leaderCommit = leaderCommit;
setLeaderCommitIsSet(true);
return this;
}
public void unsetLeaderCommit() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __LEADERCOMMIT_ISSET_ID);
}
/** Returns true if field leaderCommit is set (has been assigned a value) and false otherwise */
public boolean isSetLeaderCommit() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __LEADERCOMMIT_ISSET_ID);
}
public void setLeaderCommitIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __LEADERCOMMIT_ISSET_ID, value);
}
public byte[] getEntry() {
setEntry(org.apache.thrift.TBaseHelper.rightSize(entry));
return entry == null ? null : entry.array();
}
public java.nio.ByteBuffer bufferForEntry() {
return org.apache.thrift.TBaseHelper.copyBinary(entry);
}
public AppendEntryRequest setEntry(byte[] entry) {
this.entry = entry == null ? (java.nio.ByteBuffer)null : java.nio.ByteBuffer.wrap(entry.clone());
return this;
}
public AppendEntryRequest setEntry(@org.apache.thrift.annotation.Nullable java.nio.ByteBuffer entry) {
this.entry = org.apache.thrift.TBaseHelper.copyBinary(entry);
return this;
}
public void unsetEntry() {
this.entry = null;
}
/** Returns true if field entry is set (has been assigned a value) and false otherwise */
public boolean isSetEntry() {
return this.entry != null;
}
public void setEntryIsSet(boolean value) {
if (!value) {
this.entry = null;
}
}
@org.apache.thrift.annotation.Nullable
public RaftNode getHeader() {
return this.header;
}
public AppendEntryRequest setHeader(@org.apache.thrift.annotation.Nullable RaftNode header) {
this.header = header;
return this;
}
public void unsetHeader() {
this.header = null;
}
/** Returns true if field header is set (has been assigned a value) and false otherwise */
public boolean isSetHeader() {
return this.header != null;
}
public void setHeaderIsSet(boolean value) {
if (!value) {
this.header = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case TERM:
if (value == null) {
unsetTerm();
} else {
setTerm((java.lang.Long)value);
}
break;
case LEADER:
if (value == null) {
unsetLeader();
} else {
setLeader((Node)value);
}
break;
case PREV_LOG_INDEX:
if (value == null) {
unsetPrevLogIndex();
} else {
setPrevLogIndex((java.lang.Long)value);
}
break;
case PREV_LOG_TERM:
if (value == null) {
unsetPrevLogTerm();
} else {
setPrevLogTerm((java.lang.Long)value);
}
break;
case LEADER_COMMIT:
if (value == null) {
unsetLeaderCommit();
} else {
setLeaderCommit((java.lang.Long)value);
}
break;
case ENTRY:
if (value == null) {
unsetEntry();
} else {
if (value instanceof byte[]) {
setEntry((byte[])value);
} else {
setEntry((java.nio.ByteBuffer)value);
}
}
break;
case HEADER:
if (value == null) {
unsetHeader();
} else {
setHeader((RaftNode)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case TERM:
return getTerm();
case LEADER:
return getLeader();
case PREV_LOG_INDEX:
return getPrevLogIndex();
case PREV_LOG_TERM:
return getPrevLogTerm();
case LEADER_COMMIT:
return getLeaderCommit();
case ENTRY:
return getEntry();
case HEADER:
return getHeader();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case TERM:
return isSetTerm();
case LEADER:
return isSetLeader();
case PREV_LOG_INDEX:
return isSetPrevLogIndex();
case PREV_LOG_TERM:
return isSetPrevLogTerm();
case LEADER_COMMIT:
return isSetLeaderCommit();
case ENTRY:
return isSetEntry();
case HEADER:
return isSetHeader();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof AppendEntryRequest)
return this.equals((AppendEntryRequest)that);
return false;
}
public boolean equals(AppendEntryRequest that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_term = true;
boolean that_present_term = true;
if (this_present_term || that_present_term) {
if (!(this_present_term && that_present_term))
return false;
if (this.term != that.term)
return false;
}
boolean this_present_leader = true && this.isSetLeader();
boolean that_present_leader = true && that.isSetLeader();
if (this_present_leader || that_present_leader) {
if (!(this_present_leader && that_present_leader))
return false;
if (!this.leader.equals(that.leader))
return false;
}
boolean this_present_prevLogIndex = true;
boolean that_present_prevLogIndex = true;
if (this_present_prevLogIndex || that_present_prevLogIndex) {
if (!(this_present_prevLogIndex && that_present_prevLogIndex))
return false;
if (this.prevLogIndex != that.prevLogIndex)
return false;
}
boolean this_present_prevLogTerm = true;
boolean that_present_prevLogTerm = true;
if (this_present_prevLogTerm || that_present_prevLogTerm) {
if (!(this_present_prevLogTerm && that_present_prevLogTerm))
return false;
if (this.prevLogTerm != that.prevLogTerm)
return false;
}
boolean this_present_leaderCommit = true;
boolean that_present_leaderCommit = true;
if (this_present_leaderCommit || that_present_leaderCommit) {
if (!(this_present_leaderCommit && that_present_leaderCommit))
return false;
if (this.leaderCommit != that.leaderCommit)
return false;
}
boolean this_present_entry = true && this.isSetEntry();
boolean that_present_entry = true && that.isSetEntry();
if (this_present_entry || that_present_entry) {
if (!(this_present_entry && that_present_entry))
return false;
if (!this.entry.equals(that.entry))
return false;
}
boolean this_present_header = true && this.isSetHeader();
boolean that_present_header = true && that.isSetHeader();
if (this_present_header || that_present_header) {
if (!(this_present_header && that_present_header))
return false;
if (!this.header.equals(that.header))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(term);
hashCode = hashCode * 8191 + ((isSetLeader()) ? 131071 : 524287);
if (isSetLeader())
hashCode = hashCode * 8191 + leader.hashCode();
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(prevLogIndex);
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(prevLogTerm);
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(leaderCommit);
hashCode = hashCode * 8191 + ((isSetEntry()) ? 131071 : 524287);
if (isSetEntry())
hashCode = hashCode * 8191 + entry.hashCode();
hashCode = hashCode * 8191 + ((isSetHeader()) ? 131071 : 524287);
if (isSetHeader())
hashCode = hashCode * 8191 + header.hashCode();
return hashCode;
}
@Override
public int compareTo(AppendEntryRequest other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetTerm(), other.isSetTerm());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetTerm()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.term, other.term);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetLeader(), other.isSetLeader());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetLeader()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.leader, other.leader);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetPrevLogIndex(), other.isSetPrevLogIndex());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetPrevLogIndex()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.prevLogIndex, other.prevLogIndex);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetPrevLogTerm(), other.isSetPrevLogTerm());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetPrevLogTerm()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.prevLogTerm, other.prevLogTerm);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetLeaderCommit(), other.isSetLeaderCommit());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetLeaderCommit()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.leaderCommit, other.leaderCommit);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetEntry(), other.isSetEntry());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetEntry()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.entry, other.entry);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetHeader(), other.isSetHeader());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetHeader()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.header, other.header);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("AppendEntryRequest(");
boolean first = true;
sb.append("term:");
sb.append(this.term);
first = false;
if (!first) sb.append(", ");
sb.append("leader:");
if (this.leader == null) {
sb.append("null");
} else {
sb.append(this.leader);
}
first = false;
if (!first) sb.append(", ");
sb.append("prevLogIndex:");
sb.append(this.prevLogIndex);
first = false;
if (!first) sb.append(", ");
sb.append("prevLogTerm:");
sb.append(this.prevLogTerm);
first = false;
if (!first) sb.append(", ");
sb.append("leaderCommit:");
sb.append(this.leaderCommit);
first = false;
if (!first) sb.append(", ");
sb.append("entry:");
if (this.entry == null) {
sb.append("null");
} else {
org.apache.thrift.TBaseHelper.toString(this.entry, sb);
}
first = false;
if (isSetHeader()) {
if (!first) sb.append(", ");
sb.append("header:");
if (this.header == null) {
sb.append("null");
} else {
sb.append(this.header);
}
first = false;
}
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// alas, we cannot check 'term' because it's a primitive and you chose the non-beans generator.
if (leader == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'leader' was not present! Struct: " + toString());
}
// alas, we cannot check 'prevLogIndex' because it's a primitive and you chose the non-beans generator.
// alas, we cannot check 'prevLogTerm' because it's a primitive and you chose the non-beans generator.
// alas, we cannot check 'leaderCommit' because it's a primitive and you chose the non-beans generator.
if (entry == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'entry' was not present! Struct: " + toString());
}
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
// it doesn't seem like you should have to do this, but java serialization is wacky, and doesn't call the default constructor.
__isset_bitfield = 0;
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class AppendEntryRequestStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public AppendEntryRequestStandardScheme getScheme() {
return new AppendEntryRequestStandardScheme();
}
}
private static class AppendEntryRequestStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, AppendEntryRequest struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // TERM
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.term = iprot.readI64();
struct.setTermIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 2: // LEADER
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.leader = new Node();
struct.leader.read(iprot);
struct.setLeaderIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 3: // PREV_LOG_INDEX
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.prevLogIndex = iprot.readI64();
struct.setPrevLogIndexIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 4: // PREV_LOG_TERM
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.prevLogTerm = iprot.readI64();
struct.setPrevLogTermIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 5: // LEADER_COMMIT
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.leaderCommit = iprot.readI64();
struct.setLeaderCommitIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 6: // ENTRY
if (schemeField.type == org.apache.thrift.protocol.TType.STRING) {
struct.entry = iprot.readBinary();
struct.setEntryIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 7: // HEADER
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.header = new RaftNode();
struct.header.read(iprot);
struct.setHeaderIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
if (!struct.isSetTerm()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'term' was not found in serialized data! Struct: " + toString());
}
if (!struct.isSetPrevLogIndex()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'prevLogIndex' was not found in serialized data! Struct: " + toString());
}
if (!struct.isSetPrevLogTerm()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'prevLogTerm' was not found in serialized data! Struct: " + toString());
}
if (!struct.isSetLeaderCommit()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'leaderCommit' was not found in serialized data! Struct: " + toString());
}
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, AppendEntryRequest struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
oprot.writeFieldBegin(TERM_FIELD_DESC);
oprot.writeI64(struct.term);
oprot.writeFieldEnd();
if (struct.leader != null) {
oprot.writeFieldBegin(LEADER_FIELD_DESC);
struct.leader.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldBegin(PREV_LOG_INDEX_FIELD_DESC);
oprot.writeI64(struct.prevLogIndex);
oprot.writeFieldEnd();
oprot.writeFieldBegin(PREV_LOG_TERM_FIELD_DESC);
oprot.writeI64(struct.prevLogTerm);
oprot.writeFieldEnd();
oprot.writeFieldBegin(LEADER_COMMIT_FIELD_DESC);
oprot.writeI64(struct.leaderCommit);
oprot.writeFieldEnd();
if (struct.entry != null) {
oprot.writeFieldBegin(ENTRY_FIELD_DESC);
oprot.writeBinary(struct.entry);
oprot.writeFieldEnd();
}
if (struct.header != null) {
if (struct.isSetHeader()) {
oprot.writeFieldBegin(HEADER_FIELD_DESC);
struct.header.write(oprot);
oprot.writeFieldEnd();
}
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class AppendEntryRequestTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public AppendEntryRequestTupleScheme getScheme() {
return new AppendEntryRequestTupleScheme();
}
}
private static class AppendEntryRequestTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, AppendEntryRequest struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
oprot.writeI64(struct.term);
struct.leader.write(oprot);
oprot.writeI64(struct.prevLogIndex);
oprot.writeI64(struct.prevLogTerm);
oprot.writeI64(struct.leaderCommit);
oprot.writeBinary(struct.entry);
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetHeader()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetHeader()) {
struct.header.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, AppendEntryRequest struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
struct.term = iprot.readI64();
struct.setTermIsSet(true);
struct.leader = new Node();
struct.leader.read(iprot);
struct.setLeaderIsSet(true);
struct.prevLogIndex = iprot.readI64();
struct.setPrevLogIndexIsSet(true);
struct.prevLogTerm = iprot.readI64();
struct.setPrevLogTermIsSet(true);
struct.leaderCommit = iprot.readI64();
struct.setLeaderCommitIsSet(true);
struct.entry = iprot.readBinary();
struct.setEntryIsSet(true);
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.header = new RaftNode();
struct.header.read(iprot);
struct.setHeaderIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy