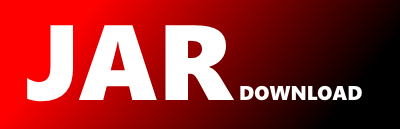
org.apache.iotdb.cluster.rpc.thrift.LastQueryRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotdb-thrift-cluster
Show all versions of iotdb-thrift-cluster
RPC (Thrift) framework among servers.
/**
* Autogenerated by Thrift Compiler (0.14.1)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package org.apache.iotdb.cluster.rpc.thrift;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked", "unused"})
@javax.annotation.Generated(value = "Autogenerated by Thrift Compiler (0.14.1)", date = "2022-07-31")
public class LastQueryRequest implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("LastQueryRequest");
private static final org.apache.thrift.protocol.TField PATHS_FIELD_DESC = new org.apache.thrift.protocol.TField("paths", org.apache.thrift.protocol.TType.LIST, (short)1);
private static final org.apache.thrift.protocol.TField DATA_TYPE_ORDINALS_FIELD_DESC = new org.apache.thrift.protocol.TField("dataTypeOrdinals", org.apache.thrift.protocol.TType.LIST, (short)2);
private static final org.apache.thrift.protocol.TField QUERY_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("queryId", org.apache.thrift.protocol.TType.I64, (short)3);
private static final org.apache.thrift.protocol.TField DEVICE_MEASUREMENTS_FIELD_DESC = new org.apache.thrift.protocol.TField("deviceMeasurements", org.apache.thrift.protocol.TType.MAP, (short)4);
private static final org.apache.thrift.protocol.TField FILTER_BYTES_FIELD_DESC = new org.apache.thrift.protocol.TField("filterBytes", org.apache.thrift.protocol.TType.STRING, (short)5);
private static final org.apache.thrift.protocol.TField HEADER_FIELD_DESC = new org.apache.thrift.protocol.TField("header", org.apache.thrift.protocol.TType.STRUCT, (short)6);
private static final org.apache.thrift.protocol.TField REQUESTOR_FIELD_DESC = new org.apache.thrift.protocol.TField("requestor", org.apache.thrift.protocol.TType.STRUCT, (short)7);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new LastQueryRequestStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new LastQueryRequestTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable java.util.List paths; // required
public @org.apache.thrift.annotation.Nullable java.util.List dataTypeOrdinals; // required
public long queryId; // required
public @org.apache.thrift.annotation.Nullable java.util.Map> deviceMeasurements; // required
public @org.apache.thrift.annotation.Nullable java.nio.ByteBuffer filterBytes; // optional
public @org.apache.thrift.annotation.Nullable RaftNode header; // required
public @org.apache.thrift.annotation.Nullable Node requestor; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
PATHS((short)1, "paths"),
DATA_TYPE_ORDINALS((short)2, "dataTypeOrdinals"),
QUERY_ID((short)3, "queryId"),
DEVICE_MEASUREMENTS((short)4, "deviceMeasurements"),
FILTER_BYTES((short)5, "filterBytes"),
HEADER((short)6, "header"),
REQUESTOR((short)7, "requestor");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // PATHS
return PATHS;
case 2: // DATA_TYPE_ORDINALS
return DATA_TYPE_ORDINALS;
case 3: // QUERY_ID
return QUERY_ID;
case 4: // DEVICE_MEASUREMENTS
return DEVICE_MEASUREMENTS;
case 5: // FILTER_BYTES
return FILTER_BYTES;
case 6: // HEADER
return HEADER;
case 7: // REQUESTOR
return REQUESTOR;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __QUERYID_ISSET_ID = 0;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.FILTER_BYTES};
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.PATHS, new org.apache.thrift.meta_data.FieldMetaData("paths", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING))));
tmpMap.put(_Fields.DATA_TYPE_ORDINALS, new org.apache.thrift.meta_data.FieldMetaData("dataTypeOrdinals", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32 , "int"))));
tmpMap.put(_Fields.QUERY_ID, new org.apache.thrift.meta_data.FieldMetaData("queryId", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.DEVICE_MEASUREMENTS, new org.apache.thrift.meta_data.FieldMetaData("deviceMeasurements", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.MapMetaData(org.apache.thrift.protocol.TType.MAP,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING),
new org.apache.thrift.meta_data.SetMetaData(org.apache.thrift.protocol.TType.SET,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)))));
tmpMap.put(_Fields.FILTER_BYTES, new org.apache.thrift.meta_data.FieldMetaData("filterBytes", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.HEADER, new org.apache.thrift.meta_data.FieldMetaData("header", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, RaftNode.class)));
tmpMap.put(_Fields.REQUESTOR, new org.apache.thrift.meta_data.FieldMetaData("requestor", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Node.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(LastQueryRequest.class, metaDataMap);
}
public LastQueryRequest() {
}
public LastQueryRequest(
java.util.List paths,
java.util.List dataTypeOrdinals,
long queryId,
java.util.Map> deviceMeasurements,
RaftNode header,
Node requestor)
{
this();
this.paths = paths;
this.dataTypeOrdinals = dataTypeOrdinals;
this.queryId = queryId;
setQueryIdIsSet(true);
this.deviceMeasurements = deviceMeasurements;
this.header = header;
this.requestor = requestor;
}
/**
* Performs a deep copy on other.
*/
public LastQueryRequest(LastQueryRequest other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetPaths()) {
java.util.List __this__paths = new java.util.ArrayList(other.paths);
this.paths = __this__paths;
}
if (other.isSetDataTypeOrdinals()) {
java.util.List __this__dataTypeOrdinals = new java.util.ArrayList(other.dataTypeOrdinals.size());
for (java.lang.Integer other_element : other.dataTypeOrdinals) {
__this__dataTypeOrdinals.add(other_element);
}
this.dataTypeOrdinals = __this__dataTypeOrdinals;
}
this.queryId = other.queryId;
if (other.isSetDeviceMeasurements()) {
java.util.Map> __this__deviceMeasurements = new java.util.HashMap>(other.deviceMeasurements.size());
for (java.util.Map.Entry> other_element : other.deviceMeasurements.entrySet()) {
java.lang.String other_element_key = other_element.getKey();
java.util.Set other_element_value = other_element.getValue();
java.lang.String __this__deviceMeasurements_copy_key = other_element_key;
java.util.Set __this__deviceMeasurements_copy_value = new java.util.HashSet(other_element_value);
__this__deviceMeasurements.put(__this__deviceMeasurements_copy_key, __this__deviceMeasurements_copy_value);
}
this.deviceMeasurements = __this__deviceMeasurements;
}
if (other.isSetFilterBytes()) {
this.filterBytes = org.apache.thrift.TBaseHelper.copyBinary(other.filterBytes);
}
if (other.isSetHeader()) {
this.header = new RaftNode(other.header);
}
if (other.isSetRequestor()) {
this.requestor = new Node(other.requestor);
}
}
public LastQueryRequest deepCopy() {
return new LastQueryRequest(this);
}
@Override
public void clear() {
this.paths = null;
this.dataTypeOrdinals = null;
setQueryIdIsSet(false);
this.queryId = 0;
this.deviceMeasurements = null;
this.filterBytes = null;
this.header = null;
this.requestor = null;
}
public int getPathsSize() {
return (this.paths == null) ? 0 : this.paths.size();
}
@org.apache.thrift.annotation.Nullable
public java.util.Iterator getPathsIterator() {
return (this.paths == null) ? null : this.paths.iterator();
}
public void addToPaths(java.lang.String elem) {
if (this.paths == null) {
this.paths = new java.util.ArrayList();
}
this.paths.add(elem);
}
@org.apache.thrift.annotation.Nullable
public java.util.List getPaths() {
return this.paths;
}
public LastQueryRequest setPaths(@org.apache.thrift.annotation.Nullable java.util.List paths) {
this.paths = paths;
return this;
}
public void unsetPaths() {
this.paths = null;
}
/** Returns true if field paths is set (has been assigned a value) and false otherwise */
public boolean isSetPaths() {
return this.paths != null;
}
public void setPathsIsSet(boolean value) {
if (!value) {
this.paths = null;
}
}
public int getDataTypeOrdinalsSize() {
return (this.dataTypeOrdinals == null) ? 0 : this.dataTypeOrdinals.size();
}
@org.apache.thrift.annotation.Nullable
public java.util.Iterator getDataTypeOrdinalsIterator() {
return (this.dataTypeOrdinals == null) ? null : this.dataTypeOrdinals.iterator();
}
public void addToDataTypeOrdinals(int elem) {
if (this.dataTypeOrdinals == null) {
this.dataTypeOrdinals = new java.util.ArrayList();
}
this.dataTypeOrdinals.add(elem);
}
@org.apache.thrift.annotation.Nullable
public java.util.List getDataTypeOrdinals() {
return this.dataTypeOrdinals;
}
public LastQueryRequest setDataTypeOrdinals(@org.apache.thrift.annotation.Nullable java.util.List dataTypeOrdinals) {
this.dataTypeOrdinals = dataTypeOrdinals;
return this;
}
public void unsetDataTypeOrdinals() {
this.dataTypeOrdinals = null;
}
/** Returns true if field dataTypeOrdinals is set (has been assigned a value) and false otherwise */
public boolean isSetDataTypeOrdinals() {
return this.dataTypeOrdinals != null;
}
public void setDataTypeOrdinalsIsSet(boolean value) {
if (!value) {
this.dataTypeOrdinals = null;
}
}
public long getQueryId() {
return this.queryId;
}
public LastQueryRequest setQueryId(long queryId) {
this.queryId = queryId;
setQueryIdIsSet(true);
return this;
}
public void unsetQueryId() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __QUERYID_ISSET_ID);
}
/** Returns true if field queryId is set (has been assigned a value) and false otherwise */
public boolean isSetQueryId() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __QUERYID_ISSET_ID);
}
public void setQueryIdIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __QUERYID_ISSET_ID, value);
}
public int getDeviceMeasurementsSize() {
return (this.deviceMeasurements == null) ? 0 : this.deviceMeasurements.size();
}
public void putToDeviceMeasurements(java.lang.String key, java.util.Set val) {
if (this.deviceMeasurements == null) {
this.deviceMeasurements = new java.util.HashMap>();
}
this.deviceMeasurements.put(key, val);
}
@org.apache.thrift.annotation.Nullable
public java.util.Map> getDeviceMeasurements() {
return this.deviceMeasurements;
}
public LastQueryRequest setDeviceMeasurements(@org.apache.thrift.annotation.Nullable java.util.Map> deviceMeasurements) {
this.deviceMeasurements = deviceMeasurements;
return this;
}
public void unsetDeviceMeasurements() {
this.deviceMeasurements = null;
}
/** Returns true if field deviceMeasurements is set (has been assigned a value) and false otherwise */
public boolean isSetDeviceMeasurements() {
return this.deviceMeasurements != null;
}
public void setDeviceMeasurementsIsSet(boolean value) {
if (!value) {
this.deviceMeasurements = null;
}
}
public byte[] getFilterBytes() {
setFilterBytes(org.apache.thrift.TBaseHelper.rightSize(filterBytes));
return filterBytes == null ? null : filterBytes.array();
}
public java.nio.ByteBuffer bufferForFilterBytes() {
return org.apache.thrift.TBaseHelper.copyBinary(filterBytes);
}
public LastQueryRequest setFilterBytes(byte[] filterBytes) {
this.filterBytes = filterBytes == null ? (java.nio.ByteBuffer)null : java.nio.ByteBuffer.wrap(filterBytes.clone());
return this;
}
public LastQueryRequest setFilterBytes(@org.apache.thrift.annotation.Nullable java.nio.ByteBuffer filterBytes) {
this.filterBytes = org.apache.thrift.TBaseHelper.copyBinary(filterBytes);
return this;
}
public void unsetFilterBytes() {
this.filterBytes = null;
}
/** Returns true if field filterBytes is set (has been assigned a value) and false otherwise */
public boolean isSetFilterBytes() {
return this.filterBytes != null;
}
public void setFilterBytesIsSet(boolean value) {
if (!value) {
this.filterBytes = null;
}
}
@org.apache.thrift.annotation.Nullable
public RaftNode getHeader() {
return this.header;
}
public LastQueryRequest setHeader(@org.apache.thrift.annotation.Nullable RaftNode header) {
this.header = header;
return this;
}
public void unsetHeader() {
this.header = null;
}
/** Returns true if field header is set (has been assigned a value) and false otherwise */
public boolean isSetHeader() {
return this.header != null;
}
public void setHeaderIsSet(boolean value) {
if (!value) {
this.header = null;
}
}
@org.apache.thrift.annotation.Nullable
public Node getRequestor() {
return this.requestor;
}
public LastQueryRequest setRequestor(@org.apache.thrift.annotation.Nullable Node requestor) {
this.requestor = requestor;
return this;
}
public void unsetRequestor() {
this.requestor = null;
}
/** Returns true if field requestor is set (has been assigned a value) and false otherwise */
public boolean isSetRequestor() {
return this.requestor != null;
}
public void setRequestorIsSet(boolean value) {
if (!value) {
this.requestor = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case PATHS:
if (value == null) {
unsetPaths();
} else {
setPaths((java.util.List)value);
}
break;
case DATA_TYPE_ORDINALS:
if (value == null) {
unsetDataTypeOrdinals();
} else {
setDataTypeOrdinals((java.util.List)value);
}
break;
case QUERY_ID:
if (value == null) {
unsetQueryId();
} else {
setQueryId((java.lang.Long)value);
}
break;
case DEVICE_MEASUREMENTS:
if (value == null) {
unsetDeviceMeasurements();
} else {
setDeviceMeasurements((java.util.Map>)value);
}
break;
case FILTER_BYTES:
if (value == null) {
unsetFilterBytes();
} else {
if (value instanceof byte[]) {
setFilterBytes((byte[])value);
} else {
setFilterBytes((java.nio.ByteBuffer)value);
}
}
break;
case HEADER:
if (value == null) {
unsetHeader();
} else {
setHeader((RaftNode)value);
}
break;
case REQUESTOR:
if (value == null) {
unsetRequestor();
} else {
setRequestor((Node)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case PATHS:
return getPaths();
case DATA_TYPE_ORDINALS:
return getDataTypeOrdinals();
case QUERY_ID:
return getQueryId();
case DEVICE_MEASUREMENTS:
return getDeviceMeasurements();
case FILTER_BYTES:
return getFilterBytes();
case HEADER:
return getHeader();
case REQUESTOR:
return getRequestor();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case PATHS:
return isSetPaths();
case DATA_TYPE_ORDINALS:
return isSetDataTypeOrdinals();
case QUERY_ID:
return isSetQueryId();
case DEVICE_MEASUREMENTS:
return isSetDeviceMeasurements();
case FILTER_BYTES:
return isSetFilterBytes();
case HEADER:
return isSetHeader();
case REQUESTOR:
return isSetRequestor();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof LastQueryRequest)
return this.equals((LastQueryRequest)that);
return false;
}
public boolean equals(LastQueryRequest that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_paths = true && this.isSetPaths();
boolean that_present_paths = true && that.isSetPaths();
if (this_present_paths || that_present_paths) {
if (!(this_present_paths && that_present_paths))
return false;
if (!this.paths.equals(that.paths))
return false;
}
boolean this_present_dataTypeOrdinals = true && this.isSetDataTypeOrdinals();
boolean that_present_dataTypeOrdinals = true && that.isSetDataTypeOrdinals();
if (this_present_dataTypeOrdinals || that_present_dataTypeOrdinals) {
if (!(this_present_dataTypeOrdinals && that_present_dataTypeOrdinals))
return false;
if (!this.dataTypeOrdinals.equals(that.dataTypeOrdinals))
return false;
}
boolean this_present_queryId = true;
boolean that_present_queryId = true;
if (this_present_queryId || that_present_queryId) {
if (!(this_present_queryId && that_present_queryId))
return false;
if (this.queryId != that.queryId)
return false;
}
boolean this_present_deviceMeasurements = true && this.isSetDeviceMeasurements();
boolean that_present_deviceMeasurements = true && that.isSetDeviceMeasurements();
if (this_present_deviceMeasurements || that_present_deviceMeasurements) {
if (!(this_present_deviceMeasurements && that_present_deviceMeasurements))
return false;
if (!this.deviceMeasurements.equals(that.deviceMeasurements))
return false;
}
boolean this_present_filterBytes = true && this.isSetFilterBytes();
boolean that_present_filterBytes = true && that.isSetFilterBytes();
if (this_present_filterBytes || that_present_filterBytes) {
if (!(this_present_filterBytes && that_present_filterBytes))
return false;
if (!this.filterBytes.equals(that.filterBytes))
return false;
}
boolean this_present_header = true && this.isSetHeader();
boolean that_present_header = true && that.isSetHeader();
if (this_present_header || that_present_header) {
if (!(this_present_header && that_present_header))
return false;
if (!this.header.equals(that.header))
return false;
}
boolean this_present_requestor = true && this.isSetRequestor();
boolean that_present_requestor = true && that.isSetRequestor();
if (this_present_requestor || that_present_requestor) {
if (!(this_present_requestor && that_present_requestor))
return false;
if (!this.requestor.equals(that.requestor))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetPaths()) ? 131071 : 524287);
if (isSetPaths())
hashCode = hashCode * 8191 + paths.hashCode();
hashCode = hashCode * 8191 + ((isSetDataTypeOrdinals()) ? 131071 : 524287);
if (isSetDataTypeOrdinals())
hashCode = hashCode * 8191 + dataTypeOrdinals.hashCode();
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(queryId);
hashCode = hashCode * 8191 + ((isSetDeviceMeasurements()) ? 131071 : 524287);
if (isSetDeviceMeasurements())
hashCode = hashCode * 8191 + deviceMeasurements.hashCode();
hashCode = hashCode * 8191 + ((isSetFilterBytes()) ? 131071 : 524287);
if (isSetFilterBytes())
hashCode = hashCode * 8191 + filterBytes.hashCode();
hashCode = hashCode * 8191 + ((isSetHeader()) ? 131071 : 524287);
if (isSetHeader())
hashCode = hashCode * 8191 + header.hashCode();
hashCode = hashCode * 8191 + ((isSetRequestor()) ? 131071 : 524287);
if (isSetRequestor())
hashCode = hashCode * 8191 + requestor.hashCode();
return hashCode;
}
@Override
public int compareTo(LastQueryRequest other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetPaths(), other.isSetPaths());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetPaths()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.paths, other.paths);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetDataTypeOrdinals(), other.isSetDataTypeOrdinals());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetDataTypeOrdinals()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.dataTypeOrdinals, other.dataTypeOrdinals);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetQueryId(), other.isSetQueryId());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetQueryId()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.queryId, other.queryId);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetDeviceMeasurements(), other.isSetDeviceMeasurements());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetDeviceMeasurements()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.deviceMeasurements, other.deviceMeasurements);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetFilterBytes(), other.isSetFilterBytes());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetFilterBytes()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.filterBytes, other.filterBytes);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetHeader(), other.isSetHeader());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetHeader()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.header, other.header);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetRequestor(), other.isSetRequestor());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequestor()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.requestor, other.requestor);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("LastQueryRequest(");
boolean first = true;
sb.append("paths:");
if (this.paths == null) {
sb.append("null");
} else {
sb.append(this.paths);
}
first = false;
if (!first) sb.append(", ");
sb.append("dataTypeOrdinals:");
if (this.dataTypeOrdinals == null) {
sb.append("null");
} else {
sb.append(this.dataTypeOrdinals);
}
first = false;
if (!first) sb.append(", ");
sb.append("queryId:");
sb.append(this.queryId);
first = false;
if (!first) sb.append(", ");
sb.append("deviceMeasurements:");
if (this.deviceMeasurements == null) {
sb.append("null");
} else {
sb.append(this.deviceMeasurements);
}
first = false;
if (isSetFilterBytes()) {
if (!first) sb.append(", ");
sb.append("filterBytes:");
if (this.filterBytes == null) {
sb.append("null");
} else {
org.apache.thrift.TBaseHelper.toString(this.filterBytes, sb);
}
first = false;
}
if (!first) sb.append(", ");
sb.append("header:");
if (this.header == null) {
sb.append("null");
} else {
sb.append(this.header);
}
first = false;
if (!first) sb.append(", ");
sb.append("requestor:");
if (this.requestor == null) {
sb.append("null");
} else {
sb.append(this.requestor);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
if (paths == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'paths' was not present! Struct: " + toString());
}
if (dataTypeOrdinals == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'dataTypeOrdinals' was not present! Struct: " + toString());
}
// alas, we cannot check 'queryId' because it's a primitive and you chose the non-beans generator.
if (deviceMeasurements == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'deviceMeasurements' was not present! Struct: " + toString());
}
if (header == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'header' was not present! Struct: " + toString());
}
if (requestor == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'requestor' was not present! Struct: " + toString());
}
// check for sub-struct validity
if (header != null) {
header.validate();
}
if (requestor != null) {
requestor.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
// it doesn't seem like you should have to do this, but java serialization is wacky, and doesn't call the default constructor.
__isset_bitfield = 0;
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class LastQueryRequestStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public LastQueryRequestStandardScheme getScheme() {
return new LastQueryRequestStandardScheme();
}
}
private static class LastQueryRequestStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, LastQueryRequest struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // PATHS
if (schemeField.type == org.apache.thrift.protocol.TType.LIST) {
{
org.apache.thrift.protocol.TList _list132 = iprot.readListBegin();
struct.paths = new java.util.ArrayList(_list132.size);
@org.apache.thrift.annotation.Nullable java.lang.String _elem133;
for (int _i134 = 0; _i134 < _list132.size; ++_i134)
{
_elem133 = iprot.readString();
struct.paths.add(_elem133);
}
iprot.readListEnd();
}
struct.setPathsIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 2: // DATA_TYPE_ORDINALS
if (schemeField.type == org.apache.thrift.protocol.TType.LIST) {
{
org.apache.thrift.protocol.TList _list135 = iprot.readListBegin();
struct.dataTypeOrdinals = new java.util.ArrayList(_list135.size);
int _elem136;
for (int _i137 = 0; _i137 < _list135.size; ++_i137)
{
_elem136 = iprot.readI32();
struct.dataTypeOrdinals.add(_elem136);
}
iprot.readListEnd();
}
struct.setDataTypeOrdinalsIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 3: // QUERY_ID
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.queryId = iprot.readI64();
struct.setQueryIdIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 4: // DEVICE_MEASUREMENTS
if (schemeField.type == org.apache.thrift.protocol.TType.MAP) {
{
org.apache.thrift.protocol.TMap _map138 = iprot.readMapBegin();
struct.deviceMeasurements = new java.util.HashMap>(2*_map138.size);
@org.apache.thrift.annotation.Nullable java.lang.String _key139;
@org.apache.thrift.annotation.Nullable java.util.Set _val140;
for (int _i141 = 0; _i141 < _map138.size; ++_i141)
{
_key139 = iprot.readString();
{
org.apache.thrift.protocol.TSet _set142 = iprot.readSetBegin();
_val140 = new java.util.HashSet(2*_set142.size);
@org.apache.thrift.annotation.Nullable java.lang.String _elem143;
for (int _i144 = 0; _i144 < _set142.size; ++_i144)
{
_elem143 = iprot.readString();
_val140.add(_elem143);
}
iprot.readSetEnd();
}
struct.deviceMeasurements.put(_key139, _val140);
}
iprot.readMapEnd();
}
struct.setDeviceMeasurementsIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 5: // FILTER_BYTES
if (schemeField.type == org.apache.thrift.protocol.TType.STRING) {
struct.filterBytes = iprot.readBinary();
struct.setFilterBytesIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 6: // HEADER
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.header = new RaftNode();
struct.header.read(iprot);
struct.setHeaderIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 7: // REQUESTOR
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.requestor = new Node();
struct.requestor.read(iprot);
struct.setRequestorIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
if (!struct.isSetQueryId()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'queryId' was not found in serialized data! Struct: " + toString());
}
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, LastQueryRequest struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.paths != null) {
oprot.writeFieldBegin(PATHS_FIELD_DESC);
{
oprot.writeListBegin(new org.apache.thrift.protocol.TList(org.apache.thrift.protocol.TType.STRING, struct.paths.size()));
for (java.lang.String _iter145 : struct.paths)
{
oprot.writeString(_iter145);
}
oprot.writeListEnd();
}
oprot.writeFieldEnd();
}
if (struct.dataTypeOrdinals != null) {
oprot.writeFieldBegin(DATA_TYPE_ORDINALS_FIELD_DESC);
{
oprot.writeListBegin(new org.apache.thrift.protocol.TList(org.apache.thrift.protocol.TType.I32, struct.dataTypeOrdinals.size()));
for (int _iter146 : struct.dataTypeOrdinals)
{
oprot.writeI32(_iter146);
}
oprot.writeListEnd();
}
oprot.writeFieldEnd();
}
oprot.writeFieldBegin(QUERY_ID_FIELD_DESC);
oprot.writeI64(struct.queryId);
oprot.writeFieldEnd();
if (struct.deviceMeasurements != null) {
oprot.writeFieldBegin(DEVICE_MEASUREMENTS_FIELD_DESC);
{
oprot.writeMapBegin(new org.apache.thrift.protocol.TMap(org.apache.thrift.protocol.TType.STRING, org.apache.thrift.protocol.TType.SET, struct.deviceMeasurements.size()));
for (java.util.Map.Entry> _iter147 : struct.deviceMeasurements.entrySet())
{
oprot.writeString(_iter147.getKey());
{
oprot.writeSetBegin(new org.apache.thrift.protocol.TSet(org.apache.thrift.protocol.TType.STRING, _iter147.getValue().size()));
for (java.lang.String _iter148 : _iter147.getValue())
{
oprot.writeString(_iter148);
}
oprot.writeSetEnd();
}
}
oprot.writeMapEnd();
}
oprot.writeFieldEnd();
}
if (struct.filterBytes != null) {
if (struct.isSetFilterBytes()) {
oprot.writeFieldBegin(FILTER_BYTES_FIELD_DESC);
oprot.writeBinary(struct.filterBytes);
oprot.writeFieldEnd();
}
}
if (struct.header != null) {
oprot.writeFieldBegin(HEADER_FIELD_DESC);
struct.header.write(oprot);
oprot.writeFieldEnd();
}
if (struct.requestor != null) {
oprot.writeFieldBegin(REQUESTOR_FIELD_DESC);
struct.requestor.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class LastQueryRequestTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public LastQueryRequestTupleScheme getScheme() {
return new LastQueryRequestTupleScheme();
}
}
private static class LastQueryRequestTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, LastQueryRequest struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
{
oprot.writeI32(struct.paths.size());
for (java.lang.String _iter149 : struct.paths)
{
oprot.writeString(_iter149);
}
}
{
oprot.writeI32(struct.dataTypeOrdinals.size());
for (int _iter150 : struct.dataTypeOrdinals)
{
oprot.writeI32(_iter150);
}
}
oprot.writeI64(struct.queryId);
{
oprot.writeI32(struct.deviceMeasurements.size());
for (java.util.Map.Entry> _iter151 : struct.deviceMeasurements.entrySet())
{
oprot.writeString(_iter151.getKey());
{
oprot.writeI32(_iter151.getValue().size());
for (java.lang.String _iter152 : _iter151.getValue())
{
oprot.writeString(_iter152);
}
}
}
}
struct.header.write(oprot);
struct.requestor.write(oprot);
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetFilterBytes()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetFilterBytes()) {
oprot.writeBinary(struct.filterBytes);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, LastQueryRequest struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
{
org.apache.thrift.protocol.TList _list153 = iprot.readListBegin(org.apache.thrift.protocol.TType.STRING);
struct.paths = new java.util.ArrayList(_list153.size);
@org.apache.thrift.annotation.Nullable java.lang.String _elem154;
for (int _i155 = 0; _i155 < _list153.size; ++_i155)
{
_elem154 = iprot.readString();
struct.paths.add(_elem154);
}
}
struct.setPathsIsSet(true);
{
org.apache.thrift.protocol.TList _list156 = iprot.readListBegin(org.apache.thrift.protocol.TType.I32);
struct.dataTypeOrdinals = new java.util.ArrayList(_list156.size);
int _elem157;
for (int _i158 = 0; _i158 < _list156.size; ++_i158)
{
_elem157 = iprot.readI32();
struct.dataTypeOrdinals.add(_elem157);
}
}
struct.setDataTypeOrdinalsIsSet(true);
struct.queryId = iprot.readI64();
struct.setQueryIdIsSet(true);
{
org.apache.thrift.protocol.TMap _map159 = iprot.readMapBegin(org.apache.thrift.protocol.TType.STRING, org.apache.thrift.protocol.TType.SET);
struct.deviceMeasurements = new java.util.HashMap>(2*_map159.size);
@org.apache.thrift.annotation.Nullable java.lang.String _key160;
@org.apache.thrift.annotation.Nullable java.util.Set _val161;
for (int _i162 = 0; _i162 < _map159.size; ++_i162)
{
_key160 = iprot.readString();
{
org.apache.thrift.protocol.TSet _set163 = iprot.readSetBegin(org.apache.thrift.protocol.TType.STRING);
_val161 = new java.util.HashSet(2*_set163.size);
@org.apache.thrift.annotation.Nullable java.lang.String _elem164;
for (int _i165 = 0; _i165 < _set163.size; ++_i165)
{
_elem164 = iprot.readString();
_val161.add(_elem164);
}
}
struct.deviceMeasurements.put(_key160, _val161);
}
}
struct.setDeviceMeasurementsIsSet(true);
struct.header = new RaftNode();
struct.header.read(iprot);
struct.setHeaderIsSet(true);
struct.requestor = new Node();
struct.requestor.read(iprot);
struct.setRequestorIsSet(true);
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.filterBytes = iprot.readBinary();
struct.setFilterBytesIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy