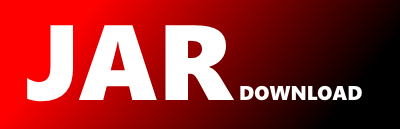
org.apache.iotdb.cluster.rpc.thrift.MultSeriesQueryRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotdb-thrift-cluster
Show all versions of iotdb-thrift-cluster
RPC (Thrift) framework among servers.
/**
* Autogenerated by Thrift Compiler (0.14.1)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package org.apache.iotdb.cluster.rpc.thrift;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked", "unused"})
@javax.annotation.Generated(value = "Autogenerated by Thrift Compiler (0.14.1)", date = "2022-07-31")
public class MultSeriesQueryRequest implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("MultSeriesQueryRequest");
private static final org.apache.thrift.protocol.TField PATH_FIELD_DESC = new org.apache.thrift.protocol.TField("path", org.apache.thrift.protocol.TType.LIST, (short)1);
private static final org.apache.thrift.protocol.TField TIME_FILTER_BYTES_FIELD_DESC = new org.apache.thrift.protocol.TField("timeFilterBytes", org.apache.thrift.protocol.TType.STRING, (short)2);
private static final org.apache.thrift.protocol.TField VALUE_FILTER_BYTES_FIELD_DESC = new org.apache.thrift.protocol.TField("valueFilterBytes", org.apache.thrift.protocol.TType.STRING, (short)3);
private static final org.apache.thrift.protocol.TField QUERY_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("queryId", org.apache.thrift.protocol.TType.I64, (short)4);
private static final org.apache.thrift.protocol.TField REQUESTER_FIELD_DESC = new org.apache.thrift.protocol.TField("requester", org.apache.thrift.protocol.TType.STRUCT, (short)5);
private static final org.apache.thrift.protocol.TField HEADER_FIELD_DESC = new org.apache.thrift.protocol.TField("header", org.apache.thrift.protocol.TType.STRUCT, (short)6);
private static final org.apache.thrift.protocol.TField DATA_TYPE_ORDINAL_FIELD_DESC = new org.apache.thrift.protocol.TField("dataTypeOrdinal", org.apache.thrift.protocol.TType.LIST, (short)7);
private static final org.apache.thrift.protocol.TField DEVICE_MEASUREMENTS_FIELD_DESC = new org.apache.thrift.protocol.TField("deviceMeasurements", org.apache.thrift.protocol.TType.MAP, (short)8);
private static final org.apache.thrift.protocol.TField ASCENDING_FIELD_DESC = new org.apache.thrift.protocol.TField("ascending", org.apache.thrift.protocol.TType.BOOL, (short)9);
private static final org.apache.thrift.protocol.TField FETCH_SIZE_FIELD_DESC = new org.apache.thrift.protocol.TField("fetchSize", org.apache.thrift.protocol.TType.I32, (short)10);
private static final org.apache.thrift.protocol.TField DEDUPLICATED_PATH_NUM_FIELD_DESC = new org.apache.thrift.protocol.TField("deduplicatedPathNum", org.apache.thrift.protocol.TType.I32, (short)11);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new MultSeriesQueryRequestStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new MultSeriesQueryRequestTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable java.util.List path; // required
public @org.apache.thrift.annotation.Nullable java.nio.ByteBuffer timeFilterBytes; // optional
public @org.apache.thrift.annotation.Nullable java.nio.ByteBuffer valueFilterBytes; // optional
public long queryId; // required
public @org.apache.thrift.annotation.Nullable Node requester; // required
public @org.apache.thrift.annotation.Nullable RaftNode header; // required
public @org.apache.thrift.annotation.Nullable java.util.List dataTypeOrdinal; // required
public @org.apache.thrift.annotation.Nullable java.util.Map> deviceMeasurements; // required
public boolean ascending; // required
public int fetchSize; // required
public int deduplicatedPathNum; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
PATH((short)1, "path"),
TIME_FILTER_BYTES((short)2, "timeFilterBytes"),
VALUE_FILTER_BYTES((short)3, "valueFilterBytes"),
QUERY_ID((short)4, "queryId"),
REQUESTER((short)5, "requester"),
HEADER((short)6, "header"),
DATA_TYPE_ORDINAL((short)7, "dataTypeOrdinal"),
DEVICE_MEASUREMENTS((short)8, "deviceMeasurements"),
ASCENDING((short)9, "ascending"),
FETCH_SIZE((short)10, "fetchSize"),
DEDUPLICATED_PATH_NUM((short)11, "deduplicatedPathNum");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // PATH
return PATH;
case 2: // TIME_FILTER_BYTES
return TIME_FILTER_BYTES;
case 3: // VALUE_FILTER_BYTES
return VALUE_FILTER_BYTES;
case 4: // QUERY_ID
return QUERY_ID;
case 5: // REQUESTER
return REQUESTER;
case 6: // HEADER
return HEADER;
case 7: // DATA_TYPE_ORDINAL
return DATA_TYPE_ORDINAL;
case 8: // DEVICE_MEASUREMENTS
return DEVICE_MEASUREMENTS;
case 9: // ASCENDING
return ASCENDING;
case 10: // FETCH_SIZE
return FETCH_SIZE;
case 11: // DEDUPLICATED_PATH_NUM
return DEDUPLICATED_PATH_NUM;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __QUERYID_ISSET_ID = 0;
private static final int __ASCENDING_ISSET_ID = 1;
private static final int __FETCHSIZE_ISSET_ID = 2;
private static final int __DEDUPLICATEDPATHNUM_ISSET_ID = 3;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.TIME_FILTER_BYTES,_Fields.VALUE_FILTER_BYTES};
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.PATH, new org.apache.thrift.meta_data.FieldMetaData("path", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING))));
tmpMap.put(_Fields.TIME_FILTER_BYTES, new org.apache.thrift.meta_data.FieldMetaData("timeFilterBytes", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.VALUE_FILTER_BYTES, new org.apache.thrift.meta_data.FieldMetaData("valueFilterBytes", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.QUERY_ID, new org.apache.thrift.meta_data.FieldMetaData("queryId", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.REQUESTER, new org.apache.thrift.meta_data.FieldMetaData("requester", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Node.class)));
tmpMap.put(_Fields.HEADER, new org.apache.thrift.meta_data.FieldMetaData("header", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, RaftNode.class)));
tmpMap.put(_Fields.DATA_TYPE_ORDINAL, new org.apache.thrift.meta_data.FieldMetaData("dataTypeOrdinal", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32 , "int"))));
tmpMap.put(_Fields.DEVICE_MEASUREMENTS, new org.apache.thrift.meta_data.FieldMetaData("deviceMeasurements", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.MapMetaData(org.apache.thrift.protocol.TType.MAP,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING),
new org.apache.thrift.meta_data.SetMetaData(org.apache.thrift.protocol.TType.SET,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)))));
tmpMap.put(_Fields.ASCENDING, new org.apache.thrift.meta_data.FieldMetaData("ascending", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.FETCH_SIZE, new org.apache.thrift.meta_data.FieldMetaData("fetchSize", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32 , "int")));
tmpMap.put(_Fields.DEDUPLICATED_PATH_NUM, new org.apache.thrift.meta_data.FieldMetaData("deduplicatedPathNum", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32 , "int")));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(MultSeriesQueryRequest.class, metaDataMap);
}
public MultSeriesQueryRequest() {
}
public MultSeriesQueryRequest(
java.util.List path,
long queryId,
Node requester,
RaftNode header,
java.util.List dataTypeOrdinal,
java.util.Map> deviceMeasurements,
boolean ascending,
int fetchSize,
int deduplicatedPathNum)
{
this();
this.path = path;
this.queryId = queryId;
setQueryIdIsSet(true);
this.requester = requester;
this.header = header;
this.dataTypeOrdinal = dataTypeOrdinal;
this.deviceMeasurements = deviceMeasurements;
this.ascending = ascending;
setAscendingIsSet(true);
this.fetchSize = fetchSize;
setFetchSizeIsSet(true);
this.deduplicatedPathNum = deduplicatedPathNum;
setDeduplicatedPathNumIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public MultSeriesQueryRequest(MultSeriesQueryRequest other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetPath()) {
java.util.List __this__path = new java.util.ArrayList(other.path);
this.path = __this__path;
}
if (other.isSetTimeFilterBytes()) {
this.timeFilterBytes = org.apache.thrift.TBaseHelper.copyBinary(other.timeFilterBytes);
}
if (other.isSetValueFilterBytes()) {
this.valueFilterBytes = org.apache.thrift.TBaseHelper.copyBinary(other.valueFilterBytes);
}
this.queryId = other.queryId;
if (other.isSetRequester()) {
this.requester = new Node(other.requester);
}
if (other.isSetHeader()) {
this.header = new RaftNode(other.header);
}
if (other.isSetDataTypeOrdinal()) {
java.util.List __this__dataTypeOrdinal = new java.util.ArrayList(other.dataTypeOrdinal.size());
for (java.lang.Integer other_element : other.dataTypeOrdinal) {
__this__dataTypeOrdinal.add(other_element);
}
this.dataTypeOrdinal = __this__dataTypeOrdinal;
}
if (other.isSetDeviceMeasurements()) {
java.util.Map> __this__deviceMeasurements = new java.util.HashMap>(other.deviceMeasurements.size());
for (java.util.Map.Entry> other_element : other.deviceMeasurements.entrySet()) {
java.lang.String other_element_key = other_element.getKey();
java.util.Set other_element_value = other_element.getValue();
java.lang.String __this__deviceMeasurements_copy_key = other_element_key;
java.util.Set __this__deviceMeasurements_copy_value = new java.util.HashSet(other_element_value);
__this__deviceMeasurements.put(__this__deviceMeasurements_copy_key, __this__deviceMeasurements_copy_value);
}
this.deviceMeasurements = __this__deviceMeasurements;
}
this.ascending = other.ascending;
this.fetchSize = other.fetchSize;
this.deduplicatedPathNum = other.deduplicatedPathNum;
}
public MultSeriesQueryRequest deepCopy() {
return new MultSeriesQueryRequest(this);
}
@Override
public void clear() {
this.path = null;
this.timeFilterBytes = null;
this.valueFilterBytes = null;
setQueryIdIsSet(false);
this.queryId = 0;
this.requester = null;
this.header = null;
this.dataTypeOrdinal = null;
this.deviceMeasurements = null;
setAscendingIsSet(false);
this.ascending = false;
setFetchSizeIsSet(false);
this.fetchSize = 0;
setDeduplicatedPathNumIsSet(false);
this.deduplicatedPathNum = 0;
}
public int getPathSize() {
return (this.path == null) ? 0 : this.path.size();
}
@org.apache.thrift.annotation.Nullable
public java.util.Iterator getPathIterator() {
return (this.path == null) ? null : this.path.iterator();
}
public void addToPath(java.lang.String elem) {
if (this.path == null) {
this.path = new java.util.ArrayList();
}
this.path.add(elem);
}
@org.apache.thrift.annotation.Nullable
public java.util.List getPath() {
return this.path;
}
public MultSeriesQueryRequest setPath(@org.apache.thrift.annotation.Nullable java.util.List path) {
this.path = path;
return this;
}
public void unsetPath() {
this.path = null;
}
/** Returns true if field path is set (has been assigned a value) and false otherwise */
public boolean isSetPath() {
return this.path != null;
}
public void setPathIsSet(boolean value) {
if (!value) {
this.path = null;
}
}
public byte[] getTimeFilterBytes() {
setTimeFilterBytes(org.apache.thrift.TBaseHelper.rightSize(timeFilterBytes));
return timeFilterBytes == null ? null : timeFilterBytes.array();
}
public java.nio.ByteBuffer bufferForTimeFilterBytes() {
return org.apache.thrift.TBaseHelper.copyBinary(timeFilterBytes);
}
public MultSeriesQueryRequest setTimeFilterBytes(byte[] timeFilterBytes) {
this.timeFilterBytes = timeFilterBytes == null ? (java.nio.ByteBuffer)null : java.nio.ByteBuffer.wrap(timeFilterBytes.clone());
return this;
}
public MultSeriesQueryRequest setTimeFilterBytes(@org.apache.thrift.annotation.Nullable java.nio.ByteBuffer timeFilterBytes) {
this.timeFilterBytes = org.apache.thrift.TBaseHelper.copyBinary(timeFilterBytes);
return this;
}
public void unsetTimeFilterBytes() {
this.timeFilterBytes = null;
}
/** Returns true if field timeFilterBytes is set (has been assigned a value) and false otherwise */
public boolean isSetTimeFilterBytes() {
return this.timeFilterBytes != null;
}
public void setTimeFilterBytesIsSet(boolean value) {
if (!value) {
this.timeFilterBytes = null;
}
}
public byte[] getValueFilterBytes() {
setValueFilterBytes(org.apache.thrift.TBaseHelper.rightSize(valueFilterBytes));
return valueFilterBytes == null ? null : valueFilterBytes.array();
}
public java.nio.ByteBuffer bufferForValueFilterBytes() {
return org.apache.thrift.TBaseHelper.copyBinary(valueFilterBytes);
}
public MultSeriesQueryRequest setValueFilterBytes(byte[] valueFilterBytes) {
this.valueFilterBytes = valueFilterBytes == null ? (java.nio.ByteBuffer)null : java.nio.ByteBuffer.wrap(valueFilterBytes.clone());
return this;
}
public MultSeriesQueryRequest setValueFilterBytes(@org.apache.thrift.annotation.Nullable java.nio.ByteBuffer valueFilterBytes) {
this.valueFilterBytes = org.apache.thrift.TBaseHelper.copyBinary(valueFilterBytes);
return this;
}
public void unsetValueFilterBytes() {
this.valueFilterBytes = null;
}
/** Returns true if field valueFilterBytes is set (has been assigned a value) and false otherwise */
public boolean isSetValueFilterBytes() {
return this.valueFilterBytes != null;
}
public void setValueFilterBytesIsSet(boolean value) {
if (!value) {
this.valueFilterBytes = null;
}
}
public long getQueryId() {
return this.queryId;
}
public MultSeriesQueryRequest setQueryId(long queryId) {
this.queryId = queryId;
setQueryIdIsSet(true);
return this;
}
public void unsetQueryId() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __QUERYID_ISSET_ID);
}
/** Returns true if field queryId is set (has been assigned a value) and false otherwise */
public boolean isSetQueryId() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __QUERYID_ISSET_ID);
}
public void setQueryIdIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __QUERYID_ISSET_ID, value);
}
@org.apache.thrift.annotation.Nullable
public Node getRequester() {
return this.requester;
}
public MultSeriesQueryRequest setRequester(@org.apache.thrift.annotation.Nullable Node requester) {
this.requester = requester;
return this;
}
public void unsetRequester() {
this.requester = null;
}
/** Returns true if field requester is set (has been assigned a value) and false otherwise */
public boolean isSetRequester() {
return this.requester != null;
}
public void setRequesterIsSet(boolean value) {
if (!value) {
this.requester = null;
}
}
@org.apache.thrift.annotation.Nullable
public RaftNode getHeader() {
return this.header;
}
public MultSeriesQueryRequest setHeader(@org.apache.thrift.annotation.Nullable RaftNode header) {
this.header = header;
return this;
}
public void unsetHeader() {
this.header = null;
}
/** Returns true if field header is set (has been assigned a value) and false otherwise */
public boolean isSetHeader() {
return this.header != null;
}
public void setHeaderIsSet(boolean value) {
if (!value) {
this.header = null;
}
}
public int getDataTypeOrdinalSize() {
return (this.dataTypeOrdinal == null) ? 0 : this.dataTypeOrdinal.size();
}
@org.apache.thrift.annotation.Nullable
public java.util.Iterator getDataTypeOrdinalIterator() {
return (this.dataTypeOrdinal == null) ? null : this.dataTypeOrdinal.iterator();
}
public void addToDataTypeOrdinal(int elem) {
if (this.dataTypeOrdinal == null) {
this.dataTypeOrdinal = new java.util.ArrayList();
}
this.dataTypeOrdinal.add(elem);
}
@org.apache.thrift.annotation.Nullable
public java.util.List getDataTypeOrdinal() {
return this.dataTypeOrdinal;
}
public MultSeriesQueryRequest setDataTypeOrdinal(@org.apache.thrift.annotation.Nullable java.util.List dataTypeOrdinal) {
this.dataTypeOrdinal = dataTypeOrdinal;
return this;
}
public void unsetDataTypeOrdinal() {
this.dataTypeOrdinal = null;
}
/** Returns true if field dataTypeOrdinal is set (has been assigned a value) and false otherwise */
public boolean isSetDataTypeOrdinal() {
return this.dataTypeOrdinal != null;
}
public void setDataTypeOrdinalIsSet(boolean value) {
if (!value) {
this.dataTypeOrdinal = null;
}
}
public int getDeviceMeasurementsSize() {
return (this.deviceMeasurements == null) ? 0 : this.deviceMeasurements.size();
}
public void putToDeviceMeasurements(java.lang.String key, java.util.Set val) {
if (this.deviceMeasurements == null) {
this.deviceMeasurements = new java.util.HashMap>();
}
this.deviceMeasurements.put(key, val);
}
@org.apache.thrift.annotation.Nullable
public java.util.Map> getDeviceMeasurements() {
return this.deviceMeasurements;
}
public MultSeriesQueryRequest setDeviceMeasurements(@org.apache.thrift.annotation.Nullable java.util.Map> deviceMeasurements) {
this.deviceMeasurements = deviceMeasurements;
return this;
}
public void unsetDeviceMeasurements() {
this.deviceMeasurements = null;
}
/** Returns true if field deviceMeasurements is set (has been assigned a value) and false otherwise */
public boolean isSetDeviceMeasurements() {
return this.deviceMeasurements != null;
}
public void setDeviceMeasurementsIsSet(boolean value) {
if (!value) {
this.deviceMeasurements = null;
}
}
public boolean isAscending() {
return this.ascending;
}
public MultSeriesQueryRequest setAscending(boolean ascending) {
this.ascending = ascending;
setAscendingIsSet(true);
return this;
}
public void unsetAscending() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __ASCENDING_ISSET_ID);
}
/** Returns true if field ascending is set (has been assigned a value) and false otherwise */
public boolean isSetAscending() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __ASCENDING_ISSET_ID);
}
public void setAscendingIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __ASCENDING_ISSET_ID, value);
}
public int getFetchSize() {
return this.fetchSize;
}
public MultSeriesQueryRequest setFetchSize(int fetchSize) {
this.fetchSize = fetchSize;
setFetchSizeIsSet(true);
return this;
}
public void unsetFetchSize() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __FETCHSIZE_ISSET_ID);
}
/** Returns true if field fetchSize is set (has been assigned a value) and false otherwise */
public boolean isSetFetchSize() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __FETCHSIZE_ISSET_ID);
}
public void setFetchSizeIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __FETCHSIZE_ISSET_ID, value);
}
public int getDeduplicatedPathNum() {
return this.deduplicatedPathNum;
}
public MultSeriesQueryRequest setDeduplicatedPathNum(int deduplicatedPathNum) {
this.deduplicatedPathNum = deduplicatedPathNum;
setDeduplicatedPathNumIsSet(true);
return this;
}
public void unsetDeduplicatedPathNum() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __DEDUPLICATEDPATHNUM_ISSET_ID);
}
/** Returns true if field deduplicatedPathNum is set (has been assigned a value) and false otherwise */
public boolean isSetDeduplicatedPathNum() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __DEDUPLICATEDPATHNUM_ISSET_ID);
}
public void setDeduplicatedPathNumIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __DEDUPLICATEDPATHNUM_ISSET_ID, value);
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case PATH:
if (value == null) {
unsetPath();
} else {
setPath((java.util.List)value);
}
break;
case TIME_FILTER_BYTES:
if (value == null) {
unsetTimeFilterBytes();
} else {
if (value instanceof byte[]) {
setTimeFilterBytes((byte[])value);
} else {
setTimeFilterBytes((java.nio.ByteBuffer)value);
}
}
break;
case VALUE_FILTER_BYTES:
if (value == null) {
unsetValueFilterBytes();
} else {
if (value instanceof byte[]) {
setValueFilterBytes((byte[])value);
} else {
setValueFilterBytes((java.nio.ByteBuffer)value);
}
}
break;
case QUERY_ID:
if (value == null) {
unsetQueryId();
} else {
setQueryId((java.lang.Long)value);
}
break;
case REQUESTER:
if (value == null) {
unsetRequester();
} else {
setRequester((Node)value);
}
break;
case HEADER:
if (value == null) {
unsetHeader();
} else {
setHeader((RaftNode)value);
}
break;
case DATA_TYPE_ORDINAL:
if (value == null) {
unsetDataTypeOrdinal();
} else {
setDataTypeOrdinal((java.util.List)value);
}
break;
case DEVICE_MEASUREMENTS:
if (value == null) {
unsetDeviceMeasurements();
} else {
setDeviceMeasurements((java.util.Map>)value);
}
break;
case ASCENDING:
if (value == null) {
unsetAscending();
} else {
setAscending((java.lang.Boolean)value);
}
break;
case FETCH_SIZE:
if (value == null) {
unsetFetchSize();
} else {
setFetchSize((java.lang.Integer)value);
}
break;
case DEDUPLICATED_PATH_NUM:
if (value == null) {
unsetDeduplicatedPathNum();
} else {
setDeduplicatedPathNum((java.lang.Integer)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case PATH:
return getPath();
case TIME_FILTER_BYTES:
return getTimeFilterBytes();
case VALUE_FILTER_BYTES:
return getValueFilterBytes();
case QUERY_ID:
return getQueryId();
case REQUESTER:
return getRequester();
case HEADER:
return getHeader();
case DATA_TYPE_ORDINAL:
return getDataTypeOrdinal();
case DEVICE_MEASUREMENTS:
return getDeviceMeasurements();
case ASCENDING:
return isAscending();
case FETCH_SIZE:
return getFetchSize();
case DEDUPLICATED_PATH_NUM:
return getDeduplicatedPathNum();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case PATH:
return isSetPath();
case TIME_FILTER_BYTES:
return isSetTimeFilterBytes();
case VALUE_FILTER_BYTES:
return isSetValueFilterBytes();
case QUERY_ID:
return isSetQueryId();
case REQUESTER:
return isSetRequester();
case HEADER:
return isSetHeader();
case DATA_TYPE_ORDINAL:
return isSetDataTypeOrdinal();
case DEVICE_MEASUREMENTS:
return isSetDeviceMeasurements();
case ASCENDING:
return isSetAscending();
case FETCH_SIZE:
return isSetFetchSize();
case DEDUPLICATED_PATH_NUM:
return isSetDeduplicatedPathNum();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof MultSeriesQueryRequest)
return this.equals((MultSeriesQueryRequest)that);
return false;
}
public boolean equals(MultSeriesQueryRequest that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_path = true && this.isSetPath();
boolean that_present_path = true && that.isSetPath();
if (this_present_path || that_present_path) {
if (!(this_present_path && that_present_path))
return false;
if (!this.path.equals(that.path))
return false;
}
boolean this_present_timeFilterBytes = true && this.isSetTimeFilterBytes();
boolean that_present_timeFilterBytes = true && that.isSetTimeFilterBytes();
if (this_present_timeFilterBytes || that_present_timeFilterBytes) {
if (!(this_present_timeFilterBytes && that_present_timeFilterBytes))
return false;
if (!this.timeFilterBytes.equals(that.timeFilterBytes))
return false;
}
boolean this_present_valueFilterBytes = true && this.isSetValueFilterBytes();
boolean that_present_valueFilterBytes = true && that.isSetValueFilterBytes();
if (this_present_valueFilterBytes || that_present_valueFilterBytes) {
if (!(this_present_valueFilterBytes && that_present_valueFilterBytes))
return false;
if (!this.valueFilterBytes.equals(that.valueFilterBytes))
return false;
}
boolean this_present_queryId = true;
boolean that_present_queryId = true;
if (this_present_queryId || that_present_queryId) {
if (!(this_present_queryId && that_present_queryId))
return false;
if (this.queryId != that.queryId)
return false;
}
boolean this_present_requester = true && this.isSetRequester();
boolean that_present_requester = true && that.isSetRequester();
if (this_present_requester || that_present_requester) {
if (!(this_present_requester && that_present_requester))
return false;
if (!this.requester.equals(that.requester))
return false;
}
boolean this_present_header = true && this.isSetHeader();
boolean that_present_header = true && that.isSetHeader();
if (this_present_header || that_present_header) {
if (!(this_present_header && that_present_header))
return false;
if (!this.header.equals(that.header))
return false;
}
boolean this_present_dataTypeOrdinal = true && this.isSetDataTypeOrdinal();
boolean that_present_dataTypeOrdinal = true && that.isSetDataTypeOrdinal();
if (this_present_dataTypeOrdinal || that_present_dataTypeOrdinal) {
if (!(this_present_dataTypeOrdinal && that_present_dataTypeOrdinal))
return false;
if (!this.dataTypeOrdinal.equals(that.dataTypeOrdinal))
return false;
}
boolean this_present_deviceMeasurements = true && this.isSetDeviceMeasurements();
boolean that_present_deviceMeasurements = true && that.isSetDeviceMeasurements();
if (this_present_deviceMeasurements || that_present_deviceMeasurements) {
if (!(this_present_deviceMeasurements && that_present_deviceMeasurements))
return false;
if (!this.deviceMeasurements.equals(that.deviceMeasurements))
return false;
}
boolean this_present_ascending = true;
boolean that_present_ascending = true;
if (this_present_ascending || that_present_ascending) {
if (!(this_present_ascending && that_present_ascending))
return false;
if (this.ascending != that.ascending)
return false;
}
boolean this_present_fetchSize = true;
boolean that_present_fetchSize = true;
if (this_present_fetchSize || that_present_fetchSize) {
if (!(this_present_fetchSize && that_present_fetchSize))
return false;
if (this.fetchSize != that.fetchSize)
return false;
}
boolean this_present_deduplicatedPathNum = true;
boolean that_present_deduplicatedPathNum = true;
if (this_present_deduplicatedPathNum || that_present_deduplicatedPathNum) {
if (!(this_present_deduplicatedPathNum && that_present_deduplicatedPathNum))
return false;
if (this.deduplicatedPathNum != that.deduplicatedPathNum)
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetPath()) ? 131071 : 524287);
if (isSetPath())
hashCode = hashCode * 8191 + path.hashCode();
hashCode = hashCode * 8191 + ((isSetTimeFilterBytes()) ? 131071 : 524287);
if (isSetTimeFilterBytes())
hashCode = hashCode * 8191 + timeFilterBytes.hashCode();
hashCode = hashCode * 8191 + ((isSetValueFilterBytes()) ? 131071 : 524287);
if (isSetValueFilterBytes())
hashCode = hashCode * 8191 + valueFilterBytes.hashCode();
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(queryId);
hashCode = hashCode * 8191 + ((isSetRequester()) ? 131071 : 524287);
if (isSetRequester())
hashCode = hashCode * 8191 + requester.hashCode();
hashCode = hashCode * 8191 + ((isSetHeader()) ? 131071 : 524287);
if (isSetHeader())
hashCode = hashCode * 8191 + header.hashCode();
hashCode = hashCode * 8191 + ((isSetDataTypeOrdinal()) ? 131071 : 524287);
if (isSetDataTypeOrdinal())
hashCode = hashCode * 8191 + dataTypeOrdinal.hashCode();
hashCode = hashCode * 8191 + ((isSetDeviceMeasurements()) ? 131071 : 524287);
if (isSetDeviceMeasurements())
hashCode = hashCode * 8191 + deviceMeasurements.hashCode();
hashCode = hashCode * 8191 + ((ascending) ? 131071 : 524287);
hashCode = hashCode * 8191 + fetchSize;
hashCode = hashCode * 8191 + deduplicatedPathNum;
return hashCode;
}
@Override
public int compareTo(MultSeriesQueryRequest other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetPath(), other.isSetPath());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetPath()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.path, other.path);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetTimeFilterBytes(), other.isSetTimeFilterBytes());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetTimeFilterBytes()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.timeFilterBytes, other.timeFilterBytes);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetValueFilterBytes(), other.isSetValueFilterBytes());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetValueFilterBytes()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.valueFilterBytes, other.valueFilterBytes);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetQueryId(), other.isSetQueryId());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetQueryId()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.queryId, other.queryId);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetRequester(), other.isSetRequester());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequester()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.requester, other.requester);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetHeader(), other.isSetHeader());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetHeader()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.header, other.header);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetDataTypeOrdinal(), other.isSetDataTypeOrdinal());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetDataTypeOrdinal()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.dataTypeOrdinal, other.dataTypeOrdinal);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetDeviceMeasurements(), other.isSetDeviceMeasurements());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetDeviceMeasurements()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.deviceMeasurements, other.deviceMeasurements);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetAscending(), other.isSetAscending());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetAscending()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.ascending, other.ascending);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetFetchSize(), other.isSetFetchSize());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetFetchSize()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.fetchSize, other.fetchSize);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetDeduplicatedPathNum(), other.isSetDeduplicatedPathNum());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetDeduplicatedPathNum()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.deduplicatedPathNum, other.deduplicatedPathNum);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("MultSeriesQueryRequest(");
boolean first = true;
sb.append("path:");
if (this.path == null) {
sb.append("null");
} else {
sb.append(this.path);
}
first = false;
if (isSetTimeFilterBytes()) {
if (!first) sb.append(", ");
sb.append("timeFilterBytes:");
if (this.timeFilterBytes == null) {
sb.append("null");
} else {
org.apache.thrift.TBaseHelper.toString(this.timeFilterBytes, sb);
}
first = false;
}
if (isSetValueFilterBytes()) {
if (!first) sb.append(", ");
sb.append("valueFilterBytes:");
if (this.valueFilterBytes == null) {
sb.append("null");
} else {
org.apache.thrift.TBaseHelper.toString(this.valueFilterBytes, sb);
}
first = false;
}
if (!first) sb.append(", ");
sb.append("queryId:");
sb.append(this.queryId);
first = false;
if (!first) sb.append(", ");
sb.append("requester:");
if (this.requester == null) {
sb.append("null");
} else {
sb.append(this.requester);
}
first = false;
if (!first) sb.append(", ");
sb.append("header:");
if (this.header == null) {
sb.append("null");
} else {
sb.append(this.header);
}
first = false;
if (!first) sb.append(", ");
sb.append("dataTypeOrdinal:");
if (this.dataTypeOrdinal == null) {
sb.append("null");
} else {
sb.append(this.dataTypeOrdinal);
}
first = false;
if (!first) sb.append(", ");
sb.append("deviceMeasurements:");
if (this.deviceMeasurements == null) {
sb.append("null");
} else {
sb.append(this.deviceMeasurements);
}
first = false;
if (!first) sb.append(", ");
sb.append("ascending:");
sb.append(this.ascending);
first = false;
if (!first) sb.append(", ");
sb.append("fetchSize:");
sb.append(this.fetchSize);
first = false;
if (!first) sb.append(", ");
sb.append("deduplicatedPathNum:");
sb.append(this.deduplicatedPathNum);
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
if (path == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'path' was not present! Struct: " + toString());
}
// alas, we cannot check 'queryId' because it's a primitive and you chose the non-beans generator.
if (requester == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'requester' was not present! Struct: " + toString());
}
if (header == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'header' was not present! Struct: " + toString());
}
if (dataTypeOrdinal == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'dataTypeOrdinal' was not present! Struct: " + toString());
}
if (deviceMeasurements == null) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'deviceMeasurements' was not present! Struct: " + toString());
}
// alas, we cannot check 'ascending' because it's a primitive and you chose the non-beans generator.
// alas, we cannot check 'fetchSize' because it's a primitive and you chose the non-beans generator.
// alas, we cannot check 'deduplicatedPathNum' because it's a primitive and you chose the non-beans generator.
// check for sub-struct validity
if (requester != null) {
requester.validate();
}
if (header != null) {
header.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
// it doesn't seem like you should have to do this, but java serialization is wacky, and doesn't call the default constructor.
__isset_bitfield = 0;
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class MultSeriesQueryRequestStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public MultSeriesQueryRequestStandardScheme getScheme() {
return new MultSeriesQueryRequestStandardScheme();
}
}
private static class MultSeriesQueryRequestStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, MultSeriesQueryRequest struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // PATH
if (schemeField.type == org.apache.thrift.protocol.TType.LIST) {
{
org.apache.thrift.protocol.TList _list58 = iprot.readListBegin();
struct.path = new java.util.ArrayList(_list58.size);
@org.apache.thrift.annotation.Nullable java.lang.String _elem59;
for (int _i60 = 0; _i60 < _list58.size; ++_i60)
{
_elem59 = iprot.readString();
struct.path.add(_elem59);
}
iprot.readListEnd();
}
struct.setPathIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 2: // TIME_FILTER_BYTES
if (schemeField.type == org.apache.thrift.protocol.TType.STRING) {
struct.timeFilterBytes = iprot.readBinary();
struct.setTimeFilterBytesIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 3: // VALUE_FILTER_BYTES
if (schemeField.type == org.apache.thrift.protocol.TType.STRING) {
struct.valueFilterBytes = iprot.readBinary();
struct.setValueFilterBytesIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 4: // QUERY_ID
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.queryId = iprot.readI64();
struct.setQueryIdIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 5: // REQUESTER
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.requester = new Node();
struct.requester.read(iprot);
struct.setRequesterIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 6: // HEADER
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.header = new RaftNode();
struct.header.read(iprot);
struct.setHeaderIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 7: // DATA_TYPE_ORDINAL
if (schemeField.type == org.apache.thrift.protocol.TType.LIST) {
{
org.apache.thrift.protocol.TList _list61 = iprot.readListBegin();
struct.dataTypeOrdinal = new java.util.ArrayList(_list61.size);
int _elem62;
for (int _i63 = 0; _i63 < _list61.size; ++_i63)
{
_elem62 = iprot.readI32();
struct.dataTypeOrdinal.add(_elem62);
}
iprot.readListEnd();
}
struct.setDataTypeOrdinalIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 8: // DEVICE_MEASUREMENTS
if (schemeField.type == org.apache.thrift.protocol.TType.MAP) {
{
org.apache.thrift.protocol.TMap _map64 = iprot.readMapBegin();
struct.deviceMeasurements = new java.util.HashMap>(2*_map64.size);
@org.apache.thrift.annotation.Nullable java.lang.String _key65;
@org.apache.thrift.annotation.Nullable java.util.Set _val66;
for (int _i67 = 0; _i67 < _map64.size; ++_i67)
{
_key65 = iprot.readString();
{
org.apache.thrift.protocol.TSet _set68 = iprot.readSetBegin();
_val66 = new java.util.HashSet(2*_set68.size);
@org.apache.thrift.annotation.Nullable java.lang.String _elem69;
for (int _i70 = 0; _i70 < _set68.size; ++_i70)
{
_elem69 = iprot.readString();
_val66.add(_elem69);
}
iprot.readSetEnd();
}
struct.deviceMeasurements.put(_key65, _val66);
}
iprot.readMapEnd();
}
struct.setDeviceMeasurementsIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 9: // ASCENDING
if (schemeField.type == org.apache.thrift.protocol.TType.BOOL) {
struct.ascending = iprot.readBool();
struct.setAscendingIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 10: // FETCH_SIZE
if (schemeField.type == org.apache.thrift.protocol.TType.I32) {
struct.fetchSize = iprot.readI32();
struct.setFetchSizeIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 11: // DEDUPLICATED_PATH_NUM
if (schemeField.type == org.apache.thrift.protocol.TType.I32) {
struct.deduplicatedPathNum = iprot.readI32();
struct.setDeduplicatedPathNumIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
if (!struct.isSetQueryId()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'queryId' was not found in serialized data! Struct: " + toString());
}
if (!struct.isSetAscending()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'ascending' was not found in serialized data! Struct: " + toString());
}
if (!struct.isSetFetchSize()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'fetchSize' was not found in serialized data! Struct: " + toString());
}
if (!struct.isSetDeduplicatedPathNum()) {
throw new org.apache.thrift.protocol.TProtocolException("Required field 'deduplicatedPathNum' was not found in serialized data! Struct: " + toString());
}
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, MultSeriesQueryRequest struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.path != null) {
oprot.writeFieldBegin(PATH_FIELD_DESC);
{
oprot.writeListBegin(new org.apache.thrift.protocol.TList(org.apache.thrift.protocol.TType.STRING, struct.path.size()));
for (java.lang.String _iter71 : struct.path)
{
oprot.writeString(_iter71);
}
oprot.writeListEnd();
}
oprot.writeFieldEnd();
}
if (struct.timeFilterBytes != null) {
if (struct.isSetTimeFilterBytes()) {
oprot.writeFieldBegin(TIME_FILTER_BYTES_FIELD_DESC);
oprot.writeBinary(struct.timeFilterBytes);
oprot.writeFieldEnd();
}
}
if (struct.valueFilterBytes != null) {
if (struct.isSetValueFilterBytes()) {
oprot.writeFieldBegin(VALUE_FILTER_BYTES_FIELD_DESC);
oprot.writeBinary(struct.valueFilterBytes);
oprot.writeFieldEnd();
}
}
oprot.writeFieldBegin(QUERY_ID_FIELD_DESC);
oprot.writeI64(struct.queryId);
oprot.writeFieldEnd();
if (struct.requester != null) {
oprot.writeFieldBegin(REQUESTER_FIELD_DESC);
struct.requester.write(oprot);
oprot.writeFieldEnd();
}
if (struct.header != null) {
oprot.writeFieldBegin(HEADER_FIELD_DESC);
struct.header.write(oprot);
oprot.writeFieldEnd();
}
if (struct.dataTypeOrdinal != null) {
oprot.writeFieldBegin(DATA_TYPE_ORDINAL_FIELD_DESC);
{
oprot.writeListBegin(new org.apache.thrift.protocol.TList(org.apache.thrift.protocol.TType.I32, struct.dataTypeOrdinal.size()));
for (int _iter72 : struct.dataTypeOrdinal)
{
oprot.writeI32(_iter72);
}
oprot.writeListEnd();
}
oprot.writeFieldEnd();
}
if (struct.deviceMeasurements != null) {
oprot.writeFieldBegin(DEVICE_MEASUREMENTS_FIELD_DESC);
{
oprot.writeMapBegin(new org.apache.thrift.protocol.TMap(org.apache.thrift.protocol.TType.STRING, org.apache.thrift.protocol.TType.SET, struct.deviceMeasurements.size()));
for (java.util.Map.Entry> _iter73 : struct.deviceMeasurements.entrySet())
{
oprot.writeString(_iter73.getKey());
{
oprot.writeSetBegin(new org.apache.thrift.protocol.TSet(org.apache.thrift.protocol.TType.STRING, _iter73.getValue().size()));
for (java.lang.String _iter74 : _iter73.getValue())
{
oprot.writeString(_iter74);
}
oprot.writeSetEnd();
}
}
oprot.writeMapEnd();
}
oprot.writeFieldEnd();
}
oprot.writeFieldBegin(ASCENDING_FIELD_DESC);
oprot.writeBool(struct.ascending);
oprot.writeFieldEnd();
oprot.writeFieldBegin(FETCH_SIZE_FIELD_DESC);
oprot.writeI32(struct.fetchSize);
oprot.writeFieldEnd();
oprot.writeFieldBegin(DEDUPLICATED_PATH_NUM_FIELD_DESC);
oprot.writeI32(struct.deduplicatedPathNum);
oprot.writeFieldEnd();
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class MultSeriesQueryRequestTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public MultSeriesQueryRequestTupleScheme getScheme() {
return new MultSeriesQueryRequestTupleScheme();
}
}
private static class MultSeriesQueryRequestTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, MultSeriesQueryRequest struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
{
oprot.writeI32(struct.path.size());
for (java.lang.String _iter75 : struct.path)
{
oprot.writeString(_iter75);
}
}
oprot.writeI64(struct.queryId);
struct.requester.write(oprot);
struct.header.write(oprot);
{
oprot.writeI32(struct.dataTypeOrdinal.size());
for (int _iter76 : struct.dataTypeOrdinal)
{
oprot.writeI32(_iter76);
}
}
{
oprot.writeI32(struct.deviceMeasurements.size());
for (java.util.Map.Entry> _iter77 : struct.deviceMeasurements.entrySet())
{
oprot.writeString(_iter77.getKey());
{
oprot.writeI32(_iter77.getValue().size());
for (java.lang.String _iter78 : _iter77.getValue())
{
oprot.writeString(_iter78);
}
}
}
}
oprot.writeBool(struct.ascending);
oprot.writeI32(struct.fetchSize);
oprot.writeI32(struct.deduplicatedPathNum);
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetTimeFilterBytes()) {
optionals.set(0);
}
if (struct.isSetValueFilterBytes()) {
optionals.set(1);
}
oprot.writeBitSet(optionals, 2);
if (struct.isSetTimeFilterBytes()) {
oprot.writeBinary(struct.timeFilterBytes);
}
if (struct.isSetValueFilterBytes()) {
oprot.writeBinary(struct.valueFilterBytes);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, MultSeriesQueryRequest struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
{
org.apache.thrift.protocol.TList _list79 = iprot.readListBegin(org.apache.thrift.protocol.TType.STRING);
struct.path = new java.util.ArrayList(_list79.size);
@org.apache.thrift.annotation.Nullable java.lang.String _elem80;
for (int _i81 = 0; _i81 < _list79.size; ++_i81)
{
_elem80 = iprot.readString();
struct.path.add(_elem80);
}
}
struct.setPathIsSet(true);
struct.queryId = iprot.readI64();
struct.setQueryIdIsSet(true);
struct.requester = new Node();
struct.requester.read(iprot);
struct.setRequesterIsSet(true);
struct.header = new RaftNode();
struct.header.read(iprot);
struct.setHeaderIsSet(true);
{
org.apache.thrift.protocol.TList _list82 = iprot.readListBegin(org.apache.thrift.protocol.TType.I32);
struct.dataTypeOrdinal = new java.util.ArrayList(_list82.size);
int _elem83;
for (int _i84 = 0; _i84 < _list82.size; ++_i84)
{
_elem83 = iprot.readI32();
struct.dataTypeOrdinal.add(_elem83);
}
}
struct.setDataTypeOrdinalIsSet(true);
{
org.apache.thrift.protocol.TMap _map85 = iprot.readMapBegin(org.apache.thrift.protocol.TType.STRING, org.apache.thrift.protocol.TType.SET);
struct.deviceMeasurements = new java.util.HashMap>(2*_map85.size);
@org.apache.thrift.annotation.Nullable java.lang.String _key86;
@org.apache.thrift.annotation.Nullable java.util.Set _val87;
for (int _i88 = 0; _i88 < _map85.size; ++_i88)
{
_key86 = iprot.readString();
{
org.apache.thrift.protocol.TSet _set89 = iprot.readSetBegin(org.apache.thrift.protocol.TType.STRING);
_val87 = new java.util.HashSet(2*_set89.size);
@org.apache.thrift.annotation.Nullable java.lang.String _elem90;
for (int _i91 = 0; _i91 < _set89.size; ++_i91)
{
_elem90 = iprot.readString();
_val87.add(_elem90);
}
}
struct.deviceMeasurements.put(_key86, _val87);
}
}
struct.setDeviceMeasurementsIsSet(true);
struct.ascending = iprot.readBool();
struct.setAscendingIsSet(true);
struct.fetchSize = iprot.readI32();
struct.setFetchSizeIsSet(true);
struct.deduplicatedPathNum = iprot.readI32();
struct.setDeduplicatedPathNumIsSet(true);
java.util.BitSet incoming = iprot.readBitSet(2);
if (incoming.get(0)) {
struct.timeFilterBytes = iprot.readBinary();
struct.setTimeFilterBytesIsSet(true);
}
if (incoming.get(1)) {
struct.valueFilterBytes = iprot.readBinary();
struct.setValueFilterBytesIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy