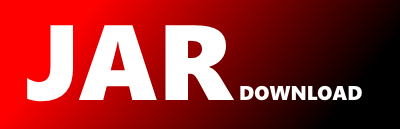
org.apache.iotdb.cluster.rpc.thrift.RaftService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotdb-thrift-cluster
Show all versions of iotdb-thrift-cluster
RPC (Thrift) framework among servers.
/**
* Autogenerated by Thrift Compiler (0.14.1)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package org.apache.iotdb.cluster.rpc.thrift;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked", "unused"})
@javax.annotation.Generated(value = "Autogenerated by Thrift Compiler (0.14.1)", date = "2022-07-31")
public class RaftService {
public interface Iface {
/**
* Leader will call this method to all followers to ensure its authority.
*
For the receiver,
* The method will check the authority of the leader.
*
* @param request information of the leader
* @return if the leader is valid, HeartBeatResponse.term will set -1, and the follower will tell
* leader its lastLogIndex; otherwise, the follower will tell the fake leader its term.
*
*
* @param request
*/
public HeartBeatResponse sendHeartbeat(HeartBeatRequest request) throws org.apache.thrift.TException;
/**
* If a node wants to be a leader, it'll call the method to other nodes to get a vote.
*
For the receiver,
* The method will check whether the node can be a leader.
*
* @param voteRequest a candidate that wants to be a leader.
* @return -1 means agree, otherwise return the voter's term
*
*
* @param request
*/
public long startElection(ElectionRequest request) throws org.apache.thrift.TException;
/**
* Leader will call this method to send a batch of entries to all followers.
*
For the receiver,
* The method will check the authority of the leader and if the local log is complete.
* If the leader is valid and local log is complete, the follower will append these entries to local log.
*
* @param request entries that need to be appended and the information of the leader.
* @return -1: agree, -2: log index mismatch , otherwise return the follower's term
*
*
* @param request
*/
public long appendEntries(AppendEntriesRequest request) throws org.apache.thrift.TException;
/**
* Leader will call this method to send a entry to all followers.
*
For the receiver,
* The method will check the authority of the leader and if the local log is complete.
* If the leader is valid and local log is complete, the follower will append the entry to local log.
*
* @param request entry that needs to be appended and the information of the leader.
* @return -1: agree, -2: log index mismatch , otherwise return the follower's term
*
*
* @param request
*/
public long appendEntry(AppendEntryRequest request) throws org.apache.thrift.TException;
public void sendSnapshot(SendSnapshotRequest request) throws org.apache.thrift.TException;
/**
* Execute a binarized non-query PhysicalPlan
*
*
* @param request
*/
public org.apache.iotdb.service.rpc.thrift.TSStatus executeNonQueryPlan(ExecutNonQueryReq request) throws org.apache.thrift.TException;
/**
* Ask the leader for its commit index, used to check whether the node has caught up with the
* leader.
*
*
* @param header
*/
public RequestCommitIndexResponse requestCommitIndex(RaftNode header) throws org.apache.thrift.TException;
/**
* Read a chunk of a file from the client. If the remaining of the file does not have enough
* bytes, only the remaining will be returned.
* Notice that when the last chunk of the file is read, the file will be deleted immediately.
*
*
* @param filePath
* @param offset
* @param length
*/
public java.nio.ByteBuffer readFile(java.lang.String filePath, long offset, int length) throws org.apache.thrift.TException;
/**
* Test if a log of "index" and "term" exists.
*
*
* @param index
* @param term
* @param header
*/
public boolean matchTerm(long index, long term, RaftNode header) throws org.apache.thrift.TException;
/**
* When a follower finds that it already has a file in a snapshot locally, it calls this
* interface to notify the leader to remove the associated hardlink.
*
*
* @param hardLinkPath
*/
public void removeHardLink(java.lang.String hardLinkPath) throws org.apache.thrift.TException;
}
public interface AsyncIface {
public void sendHeartbeat(HeartBeatRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void startElection(ElectionRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void appendEntries(AppendEntriesRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void appendEntry(AppendEntryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void sendSnapshot(SendSnapshotRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void executeNonQueryPlan(ExecutNonQueryReq request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void requestCommitIndex(RaftNode header, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void readFile(java.lang.String filePath, long offset, int length, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void matchTerm(long index, long term, RaftNode header, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void removeHardLink(java.lang.String hardLinkPath, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
}
public static class Client extends org.apache.thrift.TServiceClient implements Iface {
public static class Factory implements org.apache.thrift.TServiceClientFactory {
public Factory() {}
public Client getClient(org.apache.thrift.protocol.TProtocol prot) {
return new Client(prot);
}
public Client getClient(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TProtocol oprot) {
return new Client(iprot, oprot);
}
}
public Client(org.apache.thrift.protocol.TProtocol prot)
{
super(prot, prot);
}
public Client(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TProtocol oprot) {
super(iprot, oprot);
}
public HeartBeatResponse sendHeartbeat(HeartBeatRequest request) throws org.apache.thrift.TException
{
send_sendHeartbeat(request);
return recv_sendHeartbeat();
}
public void send_sendHeartbeat(HeartBeatRequest request) throws org.apache.thrift.TException
{
sendHeartbeat_args args = new sendHeartbeat_args();
args.setRequest(request);
sendBase("sendHeartbeat", args);
}
public HeartBeatResponse recv_sendHeartbeat() throws org.apache.thrift.TException
{
sendHeartbeat_result result = new sendHeartbeat_result();
receiveBase(result, "sendHeartbeat");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "sendHeartbeat failed: unknown result");
}
public long startElection(ElectionRequest request) throws org.apache.thrift.TException
{
send_startElection(request);
return recv_startElection();
}
public void send_startElection(ElectionRequest request) throws org.apache.thrift.TException
{
startElection_args args = new startElection_args();
args.setRequest(request);
sendBase("startElection", args);
}
public long recv_startElection() throws org.apache.thrift.TException
{
startElection_result result = new startElection_result();
receiveBase(result, "startElection");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "startElection failed: unknown result");
}
public long appendEntries(AppendEntriesRequest request) throws org.apache.thrift.TException
{
send_appendEntries(request);
return recv_appendEntries();
}
public void send_appendEntries(AppendEntriesRequest request) throws org.apache.thrift.TException
{
appendEntries_args args = new appendEntries_args();
args.setRequest(request);
sendBase("appendEntries", args);
}
public long recv_appendEntries() throws org.apache.thrift.TException
{
appendEntries_result result = new appendEntries_result();
receiveBase(result, "appendEntries");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "appendEntries failed: unknown result");
}
public long appendEntry(AppendEntryRequest request) throws org.apache.thrift.TException
{
send_appendEntry(request);
return recv_appendEntry();
}
public void send_appendEntry(AppendEntryRequest request) throws org.apache.thrift.TException
{
appendEntry_args args = new appendEntry_args();
args.setRequest(request);
sendBase("appendEntry", args);
}
public long recv_appendEntry() throws org.apache.thrift.TException
{
appendEntry_result result = new appendEntry_result();
receiveBase(result, "appendEntry");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "appendEntry failed: unknown result");
}
public void sendSnapshot(SendSnapshotRequest request) throws org.apache.thrift.TException
{
send_sendSnapshot(request);
recv_sendSnapshot();
}
public void send_sendSnapshot(SendSnapshotRequest request) throws org.apache.thrift.TException
{
sendSnapshot_args args = new sendSnapshot_args();
args.setRequest(request);
sendBase("sendSnapshot", args);
}
public void recv_sendSnapshot() throws org.apache.thrift.TException
{
sendSnapshot_result result = new sendSnapshot_result();
receiveBase(result, "sendSnapshot");
return;
}
public org.apache.iotdb.service.rpc.thrift.TSStatus executeNonQueryPlan(ExecutNonQueryReq request) throws org.apache.thrift.TException
{
send_executeNonQueryPlan(request);
return recv_executeNonQueryPlan();
}
public void send_executeNonQueryPlan(ExecutNonQueryReq request) throws org.apache.thrift.TException
{
executeNonQueryPlan_args args = new executeNonQueryPlan_args();
args.setRequest(request);
sendBase("executeNonQueryPlan", args);
}
public org.apache.iotdb.service.rpc.thrift.TSStatus recv_executeNonQueryPlan() throws org.apache.thrift.TException
{
executeNonQueryPlan_result result = new executeNonQueryPlan_result();
receiveBase(result, "executeNonQueryPlan");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "executeNonQueryPlan failed: unknown result");
}
public RequestCommitIndexResponse requestCommitIndex(RaftNode header) throws org.apache.thrift.TException
{
send_requestCommitIndex(header);
return recv_requestCommitIndex();
}
public void send_requestCommitIndex(RaftNode header) throws org.apache.thrift.TException
{
requestCommitIndex_args args = new requestCommitIndex_args();
args.setHeader(header);
sendBase("requestCommitIndex", args);
}
public RequestCommitIndexResponse recv_requestCommitIndex() throws org.apache.thrift.TException
{
requestCommitIndex_result result = new requestCommitIndex_result();
receiveBase(result, "requestCommitIndex");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "requestCommitIndex failed: unknown result");
}
public java.nio.ByteBuffer readFile(java.lang.String filePath, long offset, int length) throws org.apache.thrift.TException
{
send_readFile(filePath, offset, length);
return recv_readFile();
}
public void send_readFile(java.lang.String filePath, long offset, int length) throws org.apache.thrift.TException
{
readFile_args args = new readFile_args();
args.setFilePath(filePath);
args.setOffset(offset);
args.setLength(length);
sendBase("readFile", args);
}
public java.nio.ByteBuffer recv_readFile() throws org.apache.thrift.TException
{
readFile_result result = new readFile_result();
receiveBase(result, "readFile");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "readFile failed: unknown result");
}
public boolean matchTerm(long index, long term, RaftNode header) throws org.apache.thrift.TException
{
send_matchTerm(index, term, header);
return recv_matchTerm();
}
public void send_matchTerm(long index, long term, RaftNode header) throws org.apache.thrift.TException
{
matchTerm_args args = new matchTerm_args();
args.setIndex(index);
args.setTerm(term);
args.setHeader(header);
sendBase("matchTerm", args);
}
public boolean recv_matchTerm() throws org.apache.thrift.TException
{
matchTerm_result result = new matchTerm_result();
receiveBase(result, "matchTerm");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "matchTerm failed: unknown result");
}
public void removeHardLink(java.lang.String hardLinkPath) throws org.apache.thrift.TException
{
send_removeHardLink(hardLinkPath);
recv_removeHardLink();
}
public void send_removeHardLink(java.lang.String hardLinkPath) throws org.apache.thrift.TException
{
removeHardLink_args args = new removeHardLink_args();
args.setHardLinkPath(hardLinkPath);
sendBase("removeHardLink", args);
}
public void recv_removeHardLink() throws org.apache.thrift.TException
{
removeHardLink_result result = new removeHardLink_result();
receiveBase(result, "removeHardLink");
return;
}
}
public static class AsyncClient extends org.apache.thrift.async.TAsyncClient implements AsyncIface {
public static class Factory implements org.apache.thrift.async.TAsyncClientFactory {
private org.apache.thrift.async.TAsyncClientManager clientManager;
private org.apache.thrift.protocol.TProtocolFactory protocolFactory;
public Factory(org.apache.thrift.async.TAsyncClientManager clientManager, org.apache.thrift.protocol.TProtocolFactory protocolFactory) {
this.clientManager = clientManager;
this.protocolFactory = protocolFactory;
}
public AsyncClient getAsyncClient(org.apache.thrift.transport.TNonblockingTransport transport) {
return new AsyncClient(protocolFactory, clientManager, transport);
}
}
public AsyncClient(org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.async.TAsyncClientManager clientManager, org.apache.thrift.transport.TNonblockingTransport transport) {
super(protocolFactory, clientManager, transport);
}
public void sendHeartbeat(HeartBeatRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
sendHeartbeat_call method_call = new sendHeartbeat_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class sendHeartbeat_call extends org.apache.thrift.async.TAsyncMethodCall {
private HeartBeatRequest request;
public sendHeartbeat_call(HeartBeatRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("sendHeartbeat", org.apache.thrift.protocol.TMessageType.CALL, 0));
sendHeartbeat_args args = new sendHeartbeat_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public HeartBeatResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_sendHeartbeat();
}
}
public void startElection(ElectionRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
startElection_call method_call = new startElection_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class startElection_call extends org.apache.thrift.async.TAsyncMethodCall {
private ElectionRequest request;
public startElection_call(ElectionRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("startElection", org.apache.thrift.protocol.TMessageType.CALL, 0));
startElection_args args = new startElection_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public java.lang.Long getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_startElection();
}
}
public void appendEntries(AppendEntriesRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
appendEntries_call method_call = new appendEntries_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class appendEntries_call extends org.apache.thrift.async.TAsyncMethodCall {
private AppendEntriesRequest request;
public appendEntries_call(AppendEntriesRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("appendEntries", org.apache.thrift.protocol.TMessageType.CALL, 0));
appendEntries_args args = new appendEntries_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public java.lang.Long getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_appendEntries();
}
}
public void appendEntry(AppendEntryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
appendEntry_call method_call = new appendEntry_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class appendEntry_call extends org.apache.thrift.async.TAsyncMethodCall {
private AppendEntryRequest request;
public appendEntry_call(AppendEntryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("appendEntry", org.apache.thrift.protocol.TMessageType.CALL, 0));
appendEntry_args args = new appendEntry_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public java.lang.Long getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_appendEntry();
}
}
public void sendSnapshot(SendSnapshotRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
sendSnapshot_call method_call = new sendSnapshot_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class sendSnapshot_call extends org.apache.thrift.async.TAsyncMethodCall {
private SendSnapshotRequest request;
public sendSnapshot_call(SendSnapshotRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("sendSnapshot", org.apache.thrift.protocol.TMessageType.CALL, 0));
sendSnapshot_args args = new sendSnapshot_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public Void getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return null;
}
}
public void executeNonQueryPlan(ExecutNonQueryReq request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
executeNonQueryPlan_call method_call = new executeNonQueryPlan_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class executeNonQueryPlan_call extends org.apache.thrift.async.TAsyncMethodCall {
private ExecutNonQueryReq request;
public executeNonQueryPlan_call(ExecutNonQueryReq request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("executeNonQueryPlan", org.apache.thrift.protocol.TMessageType.CALL, 0));
executeNonQueryPlan_args args = new executeNonQueryPlan_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public org.apache.iotdb.service.rpc.thrift.TSStatus getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_executeNonQueryPlan();
}
}
public void requestCommitIndex(RaftNode header, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
requestCommitIndex_call method_call = new requestCommitIndex_call(header, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class requestCommitIndex_call extends org.apache.thrift.async.TAsyncMethodCall {
private RaftNode header;
public requestCommitIndex_call(RaftNode header, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("requestCommitIndex", org.apache.thrift.protocol.TMessageType.CALL, 0));
requestCommitIndex_args args = new requestCommitIndex_args();
args.setHeader(header);
args.write(prot);
prot.writeMessageEnd();
}
public RequestCommitIndexResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_requestCommitIndex();
}
}
public void readFile(java.lang.String filePath, long offset, int length, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
readFile_call method_call = new readFile_call(filePath, offset, length, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class readFile_call extends org.apache.thrift.async.TAsyncMethodCall {
private java.lang.String filePath;
private long offset;
private int length;
public readFile_call(java.lang.String filePath, long offset, int length, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.filePath = filePath;
this.offset = offset;
this.length = length;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("readFile", org.apache.thrift.protocol.TMessageType.CALL, 0));
readFile_args args = new readFile_args();
args.setFilePath(filePath);
args.setOffset(offset);
args.setLength(length);
args.write(prot);
prot.writeMessageEnd();
}
public java.nio.ByteBuffer getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_readFile();
}
}
public void matchTerm(long index, long term, RaftNode header, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
matchTerm_call method_call = new matchTerm_call(index, term, header, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class matchTerm_call extends org.apache.thrift.async.TAsyncMethodCall {
private long index;
private long term;
private RaftNode header;
public matchTerm_call(long index, long term, RaftNode header, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.index = index;
this.term = term;
this.header = header;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("matchTerm", org.apache.thrift.protocol.TMessageType.CALL, 0));
matchTerm_args args = new matchTerm_args();
args.setIndex(index);
args.setTerm(term);
args.setHeader(header);
args.write(prot);
prot.writeMessageEnd();
}
public java.lang.Boolean getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_matchTerm();
}
}
public void removeHardLink(java.lang.String hardLinkPath, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
removeHardLink_call method_call = new removeHardLink_call(hardLinkPath, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class removeHardLink_call extends org.apache.thrift.async.TAsyncMethodCall {
private java.lang.String hardLinkPath;
public removeHardLink_call(java.lang.String hardLinkPath, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.hardLinkPath = hardLinkPath;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("removeHardLink", org.apache.thrift.protocol.TMessageType.CALL, 0));
removeHardLink_args args = new removeHardLink_args();
args.setHardLinkPath(hardLinkPath);
args.write(prot);
prot.writeMessageEnd();
}
public Void getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return null;
}
}
}
public static class Processor extends org.apache.thrift.TBaseProcessor implements org.apache.thrift.TProcessor {
private static final org.slf4j.Logger _LOGGER = org.slf4j.LoggerFactory.getLogger(Processor.class.getName());
public Processor(I iface) {
super(iface, getProcessMap(new java.util.HashMap>()));
}
protected Processor(I iface, java.util.Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static java.util.Map> getProcessMap(java.util.Map> processMap) {
processMap.put("sendHeartbeat", new sendHeartbeat());
processMap.put("startElection", new startElection());
processMap.put("appendEntries", new appendEntries());
processMap.put("appendEntry", new appendEntry());
processMap.put("sendSnapshot", new sendSnapshot());
processMap.put("executeNonQueryPlan", new executeNonQueryPlan());
processMap.put("requestCommitIndex", new requestCommitIndex());
processMap.put("readFile", new readFile());
processMap.put("matchTerm", new matchTerm());
processMap.put("removeHardLink", new removeHardLink());
return processMap;
}
public static class sendHeartbeat extends org.apache.thrift.ProcessFunction {
public sendHeartbeat() {
super("sendHeartbeat");
}
public sendHeartbeat_args getEmptyArgsInstance() {
return new sendHeartbeat_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public sendHeartbeat_result getResult(I iface, sendHeartbeat_args args) throws org.apache.thrift.TException {
sendHeartbeat_result result = new sendHeartbeat_result();
result.success = iface.sendHeartbeat(args.request);
return result;
}
}
public static class startElection extends org.apache.thrift.ProcessFunction {
public startElection() {
super("startElection");
}
public startElection_args getEmptyArgsInstance() {
return new startElection_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public startElection_result getResult(I iface, startElection_args args) throws org.apache.thrift.TException {
startElection_result result = new startElection_result();
result.success = iface.startElection(args.request);
result.setSuccessIsSet(true);
return result;
}
}
public static class appendEntries extends org.apache.thrift.ProcessFunction {
public appendEntries() {
super("appendEntries");
}
public appendEntries_args getEmptyArgsInstance() {
return new appendEntries_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public appendEntries_result getResult(I iface, appendEntries_args args) throws org.apache.thrift.TException {
appendEntries_result result = new appendEntries_result();
result.success = iface.appendEntries(args.request);
result.setSuccessIsSet(true);
return result;
}
}
public static class appendEntry extends org.apache.thrift.ProcessFunction {
public appendEntry() {
super("appendEntry");
}
public appendEntry_args getEmptyArgsInstance() {
return new appendEntry_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public appendEntry_result getResult(I iface, appendEntry_args args) throws org.apache.thrift.TException {
appendEntry_result result = new appendEntry_result();
result.success = iface.appendEntry(args.request);
result.setSuccessIsSet(true);
return result;
}
}
public static class sendSnapshot extends org.apache.thrift.ProcessFunction {
public sendSnapshot() {
super("sendSnapshot");
}
public sendSnapshot_args getEmptyArgsInstance() {
return new sendSnapshot_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public sendSnapshot_result getResult(I iface, sendSnapshot_args args) throws org.apache.thrift.TException {
sendSnapshot_result result = new sendSnapshot_result();
iface.sendSnapshot(args.request);
return result;
}
}
public static class executeNonQueryPlan extends org.apache.thrift.ProcessFunction {
public executeNonQueryPlan() {
super("executeNonQueryPlan");
}
public executeNonQueryPlan_args getEmptyArgsInstance() {
return new executeNonQueryPlan_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public executeNonQueryPlan_result getResult(I iface, executeNonQueryPlan_args args) throws org.apache.thrift.TException {
executeNonQueryPlan_result result = new executeNonQueryPlan_result();
result.success = iface.executeNonQueryPlan(args.request);
return result;
}
}
public static class requestCommitIndex extends org.apache.thrift.ProcessFunction {
public requestCommitIndex() {
super("requestCommitIndex");
}
public requestCommitIndex_args getEmptyArgsInstance() {
return new requestCommitIndex_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public requestCommitIndex_result getResult(I iface, requestCommitIndex_args args) throws org.apache.thrift.TException {
requestCommitIndex_result result = new requestCommitIndex_result();
result.success = iface.requestCommitIndex(args.header);
return result;
}
}
public static class readFile extends org.apache.thrift.ProcessFunction {
public readFile() {
super("readFile");
}
public readFile_args getEmptyArgsInstance() {
return new readFile_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public readFile_result getResult(I iface, readFile_args args) throws org.apache.thrift.TException {
readFile_result result = new readFile_result();
result.success = iface.readFile(args.filePath, args.offset, args.length);
return result;
}
}
public static class matchTerm extends org.apache.thrift.ProcessFunction {
public matchTerm() {
super("matchTerm");
}
public matchTerm_args getEmptyArgsInstance() {
return new matchTerm_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public matchTerm_result getResult(I iface, matchTerm_args args) throws org.apache.thrift.TException {
matchTerm_result result = new matchTerm_result();
result.success = iface.matchTerm(args.index, args.term, args.header);
result.setSuccessIsSet(true);
return result;
}
}
public static class removeHardLink extends org.apache.thrift.ProcessFunction {
public removeHardLink() {
super("removeHardLink");
}
public removeHardLink_args getEmptyArgsInstance() {
return new removeHardLink_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public removeHardLink_result getResult(I iface, removeHardLink_args args) throws org.apache.thrift.TException {
removeHardLink_result result = new removeHardLink_result();
iface.removeHardLink(args.hardLinkPath);
return result;
}
}
}
public static class AsyncProcessor extends org.apache.thrift.TBaseAsyncProcessor {
private static final org.slf4j.Logger _LOGGER = org.slf4j.LoggerFactory.getLogger(AsyncProcessor.class.getName());
public AsyncProcessor(I iface) {
super(iface, getProcessMap(new java.util.HashMap>()));
}
protected AsyncProcessor(I iface, java.util.Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static java.util.Map> getProcessMap(java.util.Map> processMap) {
processMap.put("sendHeartbeat", new sendHeartbeat());
processMap.put("startElection", new startElection());
processMap.put("appendEntries", new appendEntries());
processMap.put("appendEntry", new appendEntry());
processMap.put("sendSnapshot", new sendSnapshot());
processMap.put("executeNonQueryPlan", new executeNonQueryPlan());
processMap.put("requestCommitIndex", new requestCommitIndex());
processMap.put("readFile", new readFile());
processMap.put("matchTerm", new matchTerm());
processMap.put("removeHardLink", new removeHardLink());
return processMap;
}
public static class sendHeartbeat extends org.apache.thrift.AsyncProcessFunction {
public sendHeartbeat() {
super("sendHeartbeat");
}
public sendHeartbeat_args getEmptyArgsInstance() {
return new sendHeartbeat_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(HeartBeatResponse o) {
sendHeartbeat_result result = new sendHeartbeat_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
sendHeartbeat_result result = new sendHeartbeat_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, sendHeartbeat_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.sendHeartbeat(args.request,resultHandler);
}
}
public static class startElection extends org.apache.thrift.AsyncProcessFunction {
public startElection() {
super("startElection");
}
public startElection_args getEmptyArgsInstance() {
return new startElection_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(java.lang.Long o) {
startElection_result result = new startElection_result();
result.success = o;
result.setSuccessIsSet(true);
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
startElection_result result = new startElection_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, startElection_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.startElection(args.request,resultHandler);
}
}
public static class appendEntries extends org.apache.thrift.AsyncProcessFunction {
public appendEntries() {
super("appendEntries");
}
public appendEntries_args getEmptyArgsInstance() {
return new appendEntries_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(java.lang.Long o) {
appendEntries_result result = new appendEntries_result();
result.success = o;
result.setSuccessIsSet(true);
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
appendEntries_result result = new appendEntries_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, appendEntries_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.appendEntries(args.request,resultHandler);
}
}
public static class appendEntry extends org.apache.thrift.AsyncProcessFunction {
public appendEntry() {
super("appendEntry");
}
public appendEntry_args getEmptyArgsInstance() {
return new appendEntry_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(java.lang.Long o) {
appendEntry_result result = new appendEntry_result();
result.success = o;
result.setSuccessIsSet(true);
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
appendEntry_result result = new appendEntry_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, appendEntry_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.appendEntry(args.request,resultHandler);
}
}
public static class sendSnapshot extends org.apache.thrift.AsyncProcessFunction {
public sendSnapshot() {
super("sendSnapshot");
}
public sendSnapshot_args getEmptyArgsInstance() {
return new sendSnapshot_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(Void o) {
sendSnapshot_result result = new sendSnapshot_result();
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
sendSnapshot_result result = new sendSnapshot_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, sendSnapshot_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.sendSnapshot(args.request,resultHandler);
}
}
public static class executeNonQueryPlan extends org.apache.thrift.AsyncProcessFunction {
public executeNonQueryPlan() {
super("executeNonQueryPlan");
}
public executeNonQueryPlan_args getEmptyArgsInstance() {
return new executeNonQueryPlan_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(org.apache.iotdb.service.rpc.thrift.TSStatus o) {
executeNonQueryPlan_result result = new executeNonQueryPlan_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
executeNonQueryPlan_result result = new executeNonQueryPlan_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, executeNonQueryPlan_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.executeNonQueryPlan(args.request,resultHandler);
}
}
public static class requestCommitIndex extends org.apache.thrift.AsyncProcessFunction {
public requestCommitIndex() {
super("requestCommitIndex");
}
public requestCommitIndex_args getEmptyArgsInstance() {
return new requestCommitIndex_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(RequestCommitIndexResponse o) {
requestCommitIndex_result result = new requestCommitIndex_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
requestCommitIndex_result result = new requestCommitIndex_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, requestCommitIndex_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.requestCommitIndex(args.header,resultHandler);
}
}
public static class readFile extends org.apache.thrift.AsyncProcessFunction {
public readFile() {
super("readFile");
}
public readFile_args getEmptyArgsInstance() {
return new readFile_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(java.nio.ByteBuffer o) {
readFile_result result = new readFile_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
readFile_result result = new readFile_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, readFile_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.readFile(args.filePath, args.offset, args.length,resultHandler);
}
}
public static class matchTerm extends org.apache.thrift.AsyncProcessFunction {
public matchTerm() {
super("matchTerm");
}
public matchTerm_args getEmptyArgsInstance() {
return new matchTerm_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(java.lang.Boolean o) {
matchTerm_result result = new matchTerm_result();
result.success = o;
result.setSuccessIsSet(true);
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
matchTerm_result result = new matchTerm_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, matchTerm_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.matchTerm(args.index, args.term, args.header,resultHandler);
}
}
public static class removeHardLink extends org.apache.thrift.AsyncProcessFunction {
public removeHardLink() {
super("removeHardLink");
}
public removeHardLink_args getEmptyArgsInstance() {
return new removeHardLink_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(Void o) {
removeHardLink_result result = new removeHardLink_result();
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
removeHardLink_result result = new removeHardLink_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, removeHardLink_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.removeHardLink(args.hardLinkPath,resultHandler);
}
}
}
public static class sendHeartbeat_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("sendHeartbeat_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new sendHeartbeat_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new sendHeartbeat_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable HeartBeatRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, HeartBeatRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(sendHeartbeat_args.class, metaDataMap);
}
public sendHeartbeat_args() {
}
public sendHeartbeat_args(
HeartBeatRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public sendHeartbeat_args(sendHeartbeat_args other) {
if (other.isSetRequest()) {
this.request = new HeartBeatRequest(other.request);
}
}
public sendHeartbeat_args deepCopy() {
return new sendHeartbeat_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public HeartBeatRequest getRequest() {
return this.request;
}
public sendHeartbeat_args setRequest(@org.apache.thrift.annotation.Nullable HeartBeatRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((HeartBeatRequest)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof sendHeartbeat_args)
return this.equals((sendHeartbeat_args)that);
return false;
}
public boolean equals(sendHeartbeat_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(sendHeartbeat_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("sendHeartbeat_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class sendHeartbeat_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public sendHeartbeat_argsStandardScheme getScheme() {
return new sendHeartbeat_argsStandardScheme();
}
}
private static class sendHeartbeat_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, sendHeartbeat_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new HeartBeatRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, sendHeartbeat_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class sendHeartbeat_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public sendHeartbeat_argsTupleScheme getScheme() {
return new sendHeartbeat_argsTupleScheme();
}
}
private static class sendHeartbeat_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, sendHeartbeat_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, sendHeartbeat_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new HeartBeatRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class sendHeartbeat_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("sendHeartbeat_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new sendHeartbeat_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new sendHeartbeat_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable HeartBeatResponse success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, HeartBeatResponse.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(sendHeartbeat_result.class, metaDataMap);
}
public sendHeartbeat_result() {
}
public sendHeartbeat_result(
HeartBeatResponse success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public sendHeartbeat_result(sendHeartbeat_result other) {
if (other.isSetSuccess()) {
this.success = new HeartBeatResponse(other.success);
}
}
public sendHeartbeat_result deepCopy() {
return new sendHeartbeat_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public HeartBeatResponse getSuccess() {
return this.success;
}
public sendHeartbeat_result setSuccess(@org.apache.thrift.annotation.Nullable HeartBeatResponse success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((HeartBeatResponse)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof sendHeartbeat_result)
return this.equals((sendHeartbeat_result)that);
return false;
}
public boolean equals(sendHeartbeat_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(sendHeartbeat_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("sendHeartbeat_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class sendHeartbeat_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public sendHeartbeat_resultStandardScheme getScheme() {
return new sendHeartbeat_resultStandardScheme();
}
}
private static class sendHeartbeat_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, sendHeartbeat_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new HeartBeatResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, sendHeartbeat_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class sendHeartbeat_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public sendHeartbeat_resultTupleScheme getScheme() {
return new sendHeartbeat_resultTupleScheme();
}
}
private static class sendHeartbeat_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, sendHeartbeat_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, sendHeartbeat_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new HeartBeatResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class startElection_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("startElection_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new startElection_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new startElection_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable ElectionRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, ElectionRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(startElection_args.class, metaDataMap);
}
public startElection_args() {
}
public startElection_args(
ElectionRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public startElection_args(startElection_args other) {
if (other.isSetRequest()) {
this.request = new ElectionRequest(other.request);
}
}
public startElection_args deepCopy() {
return new startElection_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public ElectionRequest getRequest() {
return this.request;
}
public startElection_args setRequest(@org.apache.thrift.annotation.Nullable ElectionRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((ElectionRequest)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof startElection_args)
return this.equals((startElection_args)that);
return false;
}
public boolean equals(startElection_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(startElection_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("startElection_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class startElection_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public startElection_argsStandardScheme getScheme() {
return new startElection_argsStandardScheme();
}
}
private static class startElection_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, startElection_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new ElectionRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, startElection_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class startElection_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public startElection_argsTupleScheme getScheme() {
return new startElection_argsTupleScheme();
}
}
private static class startElection_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, startElection_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, startElection_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new ElectionRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class startElection_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("startElection_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.I64, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new startElection_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new startElection_resultTupleSchemeFactory();
public long success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __SUCCESS_ISSET_ID = 0;
private byte __isset_bitfield = 0;
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(startElection_result.class, metaDataMap);
}
public startElection_result() {
}
public startElection_result(
long success)
{
this();
this.success = success;
setSuccessIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public startElection_result(startElection_result other) {
__isset_bitfield = other.__isset_bitfield;
this.success = other.success;
}
public startElection_result deepCopy() {
return new startElection_result(this);
}
@Override
public void clear() {
setSuccessIsSet(false);
this.success = 0;
}
public long getSuccess() {
return this.success;
}
public startElection_result setSuccess(long success) {
this.success = success;
setSuccessIsSet(true);
return this;
}
public void unsetSuccess() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __SUCCESS_ISSET_ID);
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __SUCCESS_ISSET_ID);
}
public void setSuccessIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __SUCCESS_ISSET_ID, value);
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((java.lang.Long)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof startElection_result)
return this.equals((startElection_result)that);
return false;
}
public boolean equals(startElection_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true;
boolean that_present_success = true;
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (this.success != that.success)
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(success);
return hashCode;
}
@Override
public int compareTo(startElection_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("startElection_result(");
boolean first = true;
sb.append("success:");
sb.append(this.success);
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
// it doesn't seem like you should have to do this, but java serialization is wacky, and doesn't call the default constructor.
__isset_bitfield = 0;
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class startElection_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public startElection_resultStandardScheme getScheme() {
return new startElection_resultStandardScheme();
}
}
private static class startElection_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, startElection_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.success = iprot.readI64();
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, startElection_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.isSetSuccess()) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
oprot.writeI64(struct.success);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class startElection_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public startElection_resultTupleScheme getScheme() {
return new startElection_resultTupleScheme();
}
}
private static class startElection_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, startElection_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
oprot.writeI64(struct.success);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, startElection_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = iprot.readI64();
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class appendEntries_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("appendEntries_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new appendEntries_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new appendEntries_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable AppendEntriesRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, AppendEntriesRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(appendEntries_args.class, metaDataMap);
}
public appendEntries_args() {
}
public appendEntries_args(
AppendEntriesRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public appendEntries_args(appendEntries_args other) {
if (other.isSetRequest()) {
this.request = new AppendEntriesRequest(other.request);
}
}
public appendEntries_args deepCopy() {
return new appendEntries_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public AppendEntriesRequest getRequest() {
return this.request;
}
public appendEntries_args setRequest(@org.apache.thrift.annotation.Nullable AppendEntriesRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((AppendEntriesRequest)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof appendEntries_args)
return this.equals((appendEntries_args)that);
return false;
}
public boolean equals(appendEntries_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(appendEntries_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("appendEntries_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class appendEntries_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public appendEntries_argsStandardScheme getScheme() {
return new appendEntries_argsStandardScheme();
}
}
private static class appendEntries_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, appendEntries_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new AppendEntriesRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, appendEntries_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class appendEntries_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public appendEntries_argsTupleScheme getScheme() {
return new appendEntries_argsTupleScheme();
}
}
private static class appendEntries_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, appendEntries_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, appendEntries_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new AppendEntriesRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class appendEntries_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("appendEntries_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.I64, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new appendEntries_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new appendEntries_resultTupleSchemeFactory();
public long success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __SUCCESS_ISSET_ID = 0;
private byte __isset_bitfield = 0;
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(appendEntries_result.class, metaDataMap);
}
public appendEntries_result() {
}
public appendEntries_result(
long success)
{
this();
this.success = success;
setSuccessIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public appendEntries_result(appendEntries_result other) {
__isset_bitfield = other.__isset_bitfield;
this.success = other.success;
}
public appendEntries_result deepCopy() {
return new appendEntries_result(this);
}
@Override
public void clear() {
setSuccessIsSet(false);
this.success = 0;
}
public long getSuccess() {
return this.success;
}
public appendEntries_result setSuccess(long success) {
this.success = success;
setSuccessIsSet(true);
return this;
}
public void unsetSuccess() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __SUCCESS_ISSET_ID);
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __SUCCESS_ISSET_ID);
}
public void setSuccessIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __SUCCESS_ISSET_ID, value);
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((java.lang.Long)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof appendEntries_result)
return this.equals((appendEntries_result)that);
return false;
}
public boolean equals(appendEntries_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true;
boolean that_present_success = true;
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (this.success != that.success)
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(success);
return hashCode;
}
@Override
public int compareTo(appendEntries_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("appendEntries_result(");
boolean first = true;
sb.append("success:");
sb.append(this.success);
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
// it doesn't seem like you should have to do this, but java serialization is wacky, and doesn't call the default constructor.
__isset_bitfield = 0;
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class appendEntries_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public appendEntries_resultStandardScheme getScheme() {
return new appendEntries_resultStandardScheme();
}
}
private static class appendEntries_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, appendEntries_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.success = iprot.readI64();
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, appendEntries_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.isSetSuccess()) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
oprot.writeI64(struct.success);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class appendEntries_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public appendEntries_resultTupleScheme getScheme() {
return new appendEntries_resultTupleScheme();
}
}
private static class appendEntries_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, appendEntries_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
oprot.writeI64(struct.success);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, appendEntries_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = iprot.readI64();
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class appendEntry_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("appendEntry_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new appendEntry_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new appendEntry_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable AppendEntryRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, AppendEntryRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(appendEntry_args.class, metaDataMap);
}
public appendEntry_args() {
}
public appendEntry_args(
AppendEntryRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public appendEntry_args(appendEntry_args other) {
if (other.isSetRequest()) {
this.request = new AppendEntryRequest(other.request);
}
}
public appendEntry_args deepCopy() {
return new appendEntry_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public AppendEntryRequest getRequest() {
return this.request;
}
public appendEntry_args setRequest(@org.apache.thrift.annotation.Nullable AppendEntryRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((AppendEntryRequest)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof appendEntry_args)
return this.equals((appendEntry_args)that);
return false;
}
public boolean equals(appendEntry_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(appendEntry_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("appendEntry_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class appendEntry_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public appendEntry_argsStandardScheme getScheme() {
return new appendEntry_argsStandardScheme();
}
}
private static class appendEntry_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, appendEntry_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new AppendEntryRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, appendEntry_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class appendEntry_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public appendEntry_argsTupleScheme getScheme() {
return new appendEntry_argsTupleScheme();
}
}
private static class appendEntry_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, appendEntry_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, appendEntry_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new AppendEntryRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class appendEntry_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("appendEntry_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.I64, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new appendEntry_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new appendEntry_resultTupleSchemeFactory();
public long success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __SUCCESS_ISSET_ID = 0;
private byte __isset_bitfield = 0;
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(appendEntry_result.class, metaDataMap);
}
public appendEntry_result() {
}
public appendEntry_result(
long success)
{
this();
this.success = success;
setSuccessIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public appendEntry_result(appendEntry_result other) {
__isset_bitfield = other.__isset_bitfield;
this.success = other.success;
}
public appendEntry_result deepCopy() {
return new appendEntry_result(this);
}
@Override
public void clear() {
setSuccessIsSet(false);
this.success = 0;
}
public long getSuccess() {
return this.success;
}
public appendEntry_result setSuccess(long success) {
this.success = success;
setSuccessIsSet(true);
return this;
}
public void unsetSuccess() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __SUCCESS_ISSET_ID);
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __SUCCESS_ISSET_ID);
}
public void setSuccessIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __SUCCESS_ISSET_ID, value);
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((java.lang.Long)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof appendEntry_result)
return this.equals((appendEntry_result)that);
return false;
}
public boolean equals(appendEntry_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true;
boolean that_present_success = true;
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (this.success != that.success)
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(success);
return hashCode;
}
@Override
public int compareTo(appendEntry_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("appendEntry_result(");
boolean first = true;
sb.append("success:");
sb.append(this.success);
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
// it doesn't seem like you should have to do this, but java serialization is wacky, and doesn't call the default constructor.
__isset_bitfield = 0;
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class appendEntry_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public appendEntry_resultStandardScheme getScheme() {
return new appendEntry_resultStandardScheme();
}
}
private static class appendEntry_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, appendEntry_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.success = iprot.readI64();
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, appendEntry_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.isSetSuccess()) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
oprot.writeI64(struct.success);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class appendEntry_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public appendEntry_resultTupleScheme getScheme() {
return new appendEntry_resultTupleScheme();
}
}
private static class appendEntry_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, appendEntry_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
oprot.writeI64(struct.success);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, appendEntry_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = iprot.readI64();
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class sendSnapshot_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("sendSnapshot_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new sendSnapshot_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new sendSnapshot_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable SendSnapshotRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, SendSnapshotRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(sendSnapshot_args.class, metaDataMap);
}
public sendSnapshot_args() {
}
public sendSnapshot_args(
SendSnapshotRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public sendSnapshot_args(sendSnapshot_args other) {
if (other.isSetRequest()) {
this.request = new SendSnapshotRequest(other.request);
}
}
public sendSnapshot_args deepCopy() {
return new sendSnapshot_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public SendSnapshotRequest getRequest() {
return this.request;
}
public sendSnapshot_args setRequest(@org.apache.thrift.annotation.Nullable SendSnapshotRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((SendSnapshotRequest)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof sendSnapshot_args)
return this.equals((sendSnapshot_args)that);
return false;
}
public boolean equals(sendSnapshot_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(sendSnapshot_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("sendSnapshot_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class sendSnapshot_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public sendSnapshot_argsStandardScheme getScheme() {
return new sendSnapshot_argsStandardScheme();
}
}
private static class sendSnapshot_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, sendSnapshot_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new SendSnapshotRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, sendSnapshot_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class sendSnapshot_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public sendSnapshot_argsTupleScheme getScheme() {
return new sendSnapshot_argsTupleScheme();
}
}
private static class sendSnapshot_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, sendSnapshot_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, sendSnapshot_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new SendSnapshotRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class sendSnapshot_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("sendSnapshot_result");
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new sendSnapshot_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new sendSnapshot_resultTupleSchemeFactory();
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
;
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(sendSnapshot_result.class, metaDataMap);
}
public sendSnapshot_result() {
}
/**
* Performs a deep copy on other.
*/
public sendSnapshot_result(sendSnapshot_result other) {
}
public sendSnapshot_result deepCopy() {
return new sendSnapshot_result(this);
}
@Override
public void clear() {
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof sendSnapshot_result)
return this.equals((sendSnapshot_result)that);
return false;
}
public boolean equals(sendSnapshot_result that) {
if (that == null)
return false;
if (this == that)
return true;
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
return hashCode;
}
@Override
public int compareTo(sendSnapshot_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("sendSnapshot_result(");
boolean first = true;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class sendSnapshot_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public sendSnapshot_resultStandardScheme getScheme() {
return new sendSnapshot_resultStandardScheme();
}
}
private static class sendSnapshot_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, sendSnapshot_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, sendSnapshot_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class sendSnapshot_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public sendSnapshot_resultTupleScheme getScheme() {
return new sendSnapshot_resultTupleScheme();
}
}
private static class sendSnapshot_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, sendSnapshot_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, sendSnapshot_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class executeNonQueryPlan_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("executeNonQueryPlan_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new executeNonQueryPlan_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new executeNonQueryPlan_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable ExecutNonQueryReq request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, ExecutNonQueryReq.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(executeNonQueryPlan_args.class, metaDataMap);
}
public executeNonQueryPlan_args() {
}
public executeNonQueryPlan_args(
ExecutNonQueryReq request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public executeNonQueryPlan_args(executeNonQueryPlan_args other) {
if (other.isSetRequest()) {
this.request = new ExecutNonQueryReq(other.request);
}
}
public executeNonQueryPlan_args deepCopy() {
return new executeNonQueryPlan_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public ExecutNonQueryReq getRequest() {
return this.request;
}
public executeNonQueryPlan_args setRequest(@org.apache.thrift.annotation.Nullable ExecutNonQueryReq request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((ExecutNonQueryReq)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof executeNonQueryPlan_args)
return this.equals((executeNonQueryPlan_args)that);
return false;
}
public boolean equals(executeNonQueryPlan_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(executeNonQueryPlan_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("executeNonQueryPlan_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class executeNonQueryPlan_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public executeNonQueryPlan_argsStandardScheme getScheme() {
return new executeNonQueryPlan_argsStandardScheme();
}
}
private static class executeNonQueryPlan_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, executeNonQueryPlan_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new ExecutNonQueryReq();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, executeNonQueryPlan_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class executeNonQueryPlan_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public executeNonQueryPlan_argsTupleScheme getScheme() {
return new executeNonQueryPlan_argsTupleScheme();
}
}
private static class executeNonQueryPlan_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, executeNonQueryPlan_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, executeNonQueryPlan_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new ExecutNonQueryReq();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class executeNonQueryPlan_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("executeNonQueryPlan_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new executeNonQueryPlan_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new executeNonQueryPlan_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable org.apache.iotdb.service.rpc.thrift.TSStatus success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, org.apache.iotdb.service.rpc.thrift.TSStatus.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(executeNonQueryPlan_result.class, metaDataMap);
}
public executeNonQueryPlan_result() {
}
public executeNonQueryPlan_result(
org.apache.iotdb.service.rpc.thrift.TSStatus success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public executeNonQueryPlan_result(executeNonQueryPlan_result other) {
if (other.isSetSuccess()) {
this.success = new org.apache.iotdb.service.rpc.thrift.TSStatus(other.success);
}
}
public executeNonQueryPlan_result deepCopy() {
return new executeNonQueryPlan_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public org.apache.iotdb.service.rpc.thrift.TSStatus getSuccess() {
return this.success;
}
public executeNonQueryPlan_result setSuccess(@org.apache.thrift.annotation.Nullable org.apache.iotdb.service.rpc.thrift.TSStatus success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((org.apache.iotdb.service.rpc.thrift.TSStatus)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof executeNonQueryPlan_result)
return this.equals((executeNonQueryPlan_result)that);
return false;
}
public boolean equals(executeNonQueryPlan_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(executeNonQueryPlan_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("executeNonQueryPlan_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class executeNonQueryPlan_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public executeNonQueryPlan_resultStandardScheme getScheme() {
return new executeNonQueryPlan_resultStandardScheme();
}
}
private static class executeNonQueryPlan_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, executeNonQueryPlan_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new org.apache.iotdb.service.rpc.thrift.TSStatus();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, executeNonQueryPlan_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class executeNonQueryPlan_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public executeNonQueryPlan_resultTupleScheme getScheme() {
return new executeNonQueryPlan_resultTupleScheme();
}
}
private static class executeNonQueryPlan_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, executeNonQueryPlan_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, executeNonQueryPlan_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new org.apache.iotdb.service.rpc.thrift.TSStatus();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class requestCommitIndex_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("requestCommitIndex_args");
private static final org.apache.thrift.protocol.TField HEADER_FIELD_DESC = new org.apache.thrift.protocol.TField("header", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new requestCommitIndex_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new requestCommitIndex_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable RaftNode header; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
HEADER((short)1, "header");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // HEADER
return HEADER;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.HEADER, new org.apache.thrift.meta_data.FieldMetaData("header", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, RaftNode.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(requestCommitIndex_args.class, metaDataMap);
}
public requestCommitIndex_args() {
}
public requestCommitIndex_args(
RaftNode header)
{
this();
this.header = header;
}
/**
* Performs a deep copy on other.
*/
public requestCommitIndex_args(requestCommitIndex_args other) {
if (other.isSetHeader()) {
this.header = new RaftNode(other.header);
}
}
public requestCommitIndex_args deepCopy() {
return new requestCommitIndex_args(this);
}
@Override
public void clear() {
this.header = null;
}
@org.apache.thrift.annotation.Nullable
public RaftNode getHeader() {
return this.header;
}
public requestCommitIndex_args setHeader(@org.apache.thrift.annotation.Nullable RaftNode header) {
this.header = header;
return this;
}
public void unsetHeader() {
this.header = null;
}
/** Returns true if field header is set (has been assigned a value) and false otherwise */
public boolean isSetHeader() {
return this.header != null;
}
public void setHeaderIsSet(boolean value) {
if (!value) {
this.header = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case HEADER:
if (value == null) {
unsetHeader();
} else {
setHeader((RaftNode)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case HEADER:
return getHeader();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case HEADER:
return isSetHeader();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof requestCommitIndex_args)
return this.equals((requestCommitIndex_args)that);
return false;
}
public boolean equals(requestCommitIndex_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_header = true && this.isSetHeader();
boolean that_present_header = true && that.isSetHeader();
if (this_present_header || that_present_header) {
if (!(this_present_header && that_present_header))
return false;
if (!this.header.equals(that.header))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetHeader()) ? 131071 : 524287);
if (isSetHeader())
hashCode = hashCode * 8191 + header.hashCode();
return hashCode;
}
@Override
public int compareTo(requestCommitIndex_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetHeader(), other.isSetHeader());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetHeader()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.header, other.header);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("requestCommitIndex_args(");
boolean first = true;
sb.append("header:");
if (this.header == null) {
sb.append("null");
} else {
sb.append(this.header);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (header != null) {
header.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class requestCommitIndex_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public requestCommitIndex_argsStandardScheme getScheme() {
return new requestCommitIndex_argsStandardScheme();
}
}
private static class requestCommitIndex_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, requestCommitIndex_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // HEADER
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.header = new RaftNode();
struct.header.read(iprot);
struct.setHeaderIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, requestCommitIndex_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.header != null) {
oprot.writeFieldBegin(HEADER_FIELD_DESC);
struct.header.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class requestCommitIndex_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public requestCommitIndex_argsTupleScheme getScheme() {
return new requestCommitIndex_argsTupleScheme();
}
}
private static class requestCommitIndex_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, requestCommitIndex_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetHeader()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetHeader()) {
struct.header.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, requestCommitIndex_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.header = new RaftNode();
struct.header.read(iprot);
struct.setHeaderIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class requestCommitIndex_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("requestCommitIndex_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new requestCommitIndex_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new requestCommitIndex_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable RequestCommitIndexResponse success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, RequestCommitIndexResponse.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(requestCommitIndex_result.class, metaDataMap);
}
public requestCommitIndex_result() {
}
public requestCommitIndex_result(
RequestCommitIndexResponse success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public requestCommitIndex_result(requestCommitIndex_result other) {
if (other.isSetSuccess()) {
this.success = new RequestCommitIndexResponse(other.success);
}
}
public requestCommitIndex_result deepCopy() {
return new requestCommitIndex_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public RequestCommitIndexResponse getSuccess() {
return this.success;
}
public requestCommitIndex_result setSuccess(@org.apache.thrift.annotation.Nullable RequestCommitIndexResponse success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((RequestCommitIndexResponse)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof requestCommitIndex_result)
return this.equals((requestCommitIndex_result)that);
return false;
}
public boolean equals(requestCommitIndex_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(requestCommitIndex_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("requestCommitIndex_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class requestCommitIndex_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public requestCommitIndex_resultStandardScheme getScheme() {
return new requestCommitIndex_resultStandardScheme();
}
}
private static class requestCommitIndex_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, requestCommitIndex_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new RequestCommitIndexResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, requestCommitIndex_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class requestCommitIndex_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public requestCommitIndex_resultTupleScheme getScheme() {
return new requestCommitIndex_resultTupleScheme();
}
}
private static class requestCommitIndex_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, requestCommitIndex_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, requestCommitIndex_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new RequestCommitIndexResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class readFile_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("readFile_args");
private static final org.apache.thrift.protocol.TField FILE_PATH_FIELD_DESC = new org.apache.thrift.protocol.TField("filePath", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField OFFSET_FIELD_DESC = new org.apache.thrift.protocol.TField("offset", org.apache.thrift.protocol.TType.I64, (short)2);
private static final org.apache.thrift.protocol.TField LENGTH_FIELD_DESC = new org.apache.thrift.protocol.TField("length", org.apache.thrift.protocol.TType.I32, (short)3);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new readFile_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new readFile_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable java.lang.String filePath; // required
public long offset; // required
public int length; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
FILE_PATH((short)1, "filePath"),
OFFSET((short)2, "offset"),
LENGTH((short)3, "length");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // FILE_PATH
return FILE_PATH;
case 2: // OFFSET
return OFFSET;
case 3: // LENGTH
return LENGTH;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __OFFSET_ISSET_ID = 0;
private static final int __LENGTH_ISSET_ID = 1;
private byte __isset_bitfield = 0;
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.FILE_PATH, new org.apache.thrift.meta_data.FieldMetaData("filePath", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.OFFSET, new org.apache.thrift.meta_data.FieldMetaData("offset", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.LENGTH, new org.apache.thrift.meta_data.FieldMetaData("length", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32 , "int")));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(readFile_args.class, metaDataMap);
}
public readFile_args() {
}
public readFile_args(
java.lang.String filePath,
long offset,
int length)
{
this();
this.filePath = filePath;
this.offset = offset;
setOffsetIsSet(true);
this.length = length;
setLengthIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public readFile_args(readFile_args other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetFilePath()) {
this.filePath = other.filePath;
}
this.offset = other.offset;
this.length = other.length;
}
public readFile_args deepCopy() {
return new readFile_args(this);
}
@Override
public void clear() {
this.filePath = null;
setOffsetIsSet(false);
this.offset = 0;
setLengthIsSet(false);
this.length = 0;
}
@org.apache.thrift.annotation.Nullable
public java.lang.String getFilePath() {
return this.filePath;
}
public readFile_args setFilePath(@org.apache.thrift.annotation.Nullable java.lang.String filePath) {
this.filePath = filePath;
return this;
}
public void unsetFilePath() {
this.filePath = null;
}
/** Returns true if field filePath is set (has been assigned a value) and false otherwise */
public boolean isSetFilePath() {
return this.filePath != null;
}
public void setFilePathIsSet(boolean value) {
if (!value) {
this.filePath = null;
}
}
public long getOffset() {
return this.offset;
}
public readFile_args setOffset(long offset) {
this.offset = offset;
setOffsetIsSet(true);
return this;
}
public void unsetOffset() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __OFFSET_ISSET_ID);
}
/** Returns true if field offset is set (has been assigned a value) and false otherwise */
public boolean isSetOffset() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __OFFSET_ISSET_ID);
}
public void setOffsetIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __OFFSET_ISSET_ID, value);
}
public int getLength() {
return this.length;
}
public readFile_args setLength(int length) {
this.length = length;
setLengthIsSet(true);
return this;
}
public void unsetLength() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __LENGTH_ISSET_ID);
}
/** Returns true if field length is set (has been assigned a value) and false otherwise */
public boolean isSetLength() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __LENGTH_ISSET_ID);
}
public void setLengthIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __LENGTH_ISSET_ID, value);
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case FILE_PATH:
if (value == null) {
unsetFilePath();
} else {
setFilePath((java.lang.String)value);
}
break;
case OFFSET:
if (value == null) {
unsetOffset();
} else {
setOffset((java.lang.Long)value);
}
break;
case LENGTH:
if (value == null) {
unsetLength();
} else {
setLength((java.lang.Integer)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case FILE_PATH:
return getFilePath();
case OFFSET:
return getOffset();
case LENGTH:
return getLength();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case FILE_PATH:
return isSetFilePath();
case OFFSET:
return isSetOffset();
case LENGTH:
return isSetLength();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof readFile_args)
return this.equals((readFile_args)that);
return false;
}
public boolean equals(readFile_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_filePath = true && this.isSetFilePath();
boolean that_present_filePath = true && that.isSetFilePath();
if (this_present_filePath || that_present_filePath) {
if (!(this_present_filePath && that_present_filePath))
return false;
if (!this.filePath.equals(that.filePath))
return false;
}
boolean this_present_offset = true;
boolean that_present_offset = true;
if (this_present_offset || that_present_offset) {
if (!(this_present_offset && that_present_offset))
return false;
if (this.offset != that.offset)
return false;
}
boolean this_present_length = true;
boolean that_present_length = true;
if (this_present_length || that_present_length) {
if (!(this_present_length && that_present_length))
return false;
if (this.length != that.length)
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetFilePath()) ? 131071 : 524287);
if (isSetFilePath())
hashCode = hashCode * 8191 + filePath.hashCode();
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(offset);
hashCode = hashCode * 8191 + length;
return hashCode;
}
@Override
public int compareTo(readFile_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetFilePath(), other.isSetFilePath());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetFilePath()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.filePath, other.filePath);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetOffset(), other.isSetOffset());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetOffset()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.offset, other.offset);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetLength(), other.isSetLength());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetLength()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.length, other.length);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("readFile_args(");
boolean first = true;
sb.append("filePath:");
if (this.filePath == null) {
sb.append("null");
} else {
sb.append(this.filePath);
}
first = false;
if (!first) sb.append(", ");
sb.append("offset:");
sb.append(this.offset);
first = false;
if (!first) sb.append(", ");
sb.append("length:");
sb.append(this.length);
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
// it doesn't seem like you should have to do this, but java serialization is wacky, and doesn't call the default constructor.
__isset_bitfield = 0;
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class readFile_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public readFile_argsStandardScheme getScheme() {
return new readFile_argsStandardScheme();
}
}
private static class readFile_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, readFile_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // FILE_PATH
if (schemeField.type == org.apache.thrift.protocol.TType.STRING) {
struct.filePath = iprot.readString();
struct.setFilePathIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 2: // OFFSET
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.offset = iprot.readI64();
struct.setOffsetIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 3: // LENGTH
if (schemeField.type == org.apache.thrift.protocol.TType.I32) {
struct.length = iprot.readI32();
struct.setLengthIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, readFile_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.filePath != null) {
oprot.writeFieldBegin(FILE_PATH_FIELD_DESC);
oprot.writeString(struct.filePath);
oprot.writeFieldEnd();
}
oprot.writeFieldBegin(OFFSET_FIELD_DESC);
oprot.writeI64(struct.offset);
oprot.writeFieldEnd();
oprot.writeFieldBegin(LENGTH_FIELD_DESC);
oprot.writeI32(struct.length);
oprot.writeFieldEnd();
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class readFile_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public readFile_argsTupleScheme getScheme() {
return new readFile_argsTupleScheme();
}
}
private static class readFile_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, readFile_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetFilePath()) {
optionals.set(0);
}
if (struct.isSetOffset()) {
optionals.set(1);
}
if (struct.isSetLength()) {
optionals.set(2);
}
oprot.writeBitSet(optionals, 3);
if (struct.isSetFilePath()) {
oprot.writeString(struct.filePath);
}
if (struct.isSetOffset()) {
oprot.writeI64(struct.offset);
}
if (struct.isSetLength()) {
oprot.writeI32(struct.length);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, readFile_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(3);
if (incoming.get(0)) {
struct.filePath = iprot.readString();
struct.setFilePathIsSet(true);
}
if (incoming.get(1)) {
struct.offset = iprot.readI64();
struct.setOffsetIsSet(true);
}
if (incoming.get(2)) {
struct.length = iprot.readI32();
struct.setLengthIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class readFile_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("readFile_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRING, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new readFile_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new readFile_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable java.nio.ByteBuffer success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(readFile_result.class, metaDataMap);
}
public readFile_result() {
}
public readFile_result(
java.nio.ByteBuffer success)
{
this();
this.success = org.apache.thrift.TBaseHelper.copyBinary(success);
}
/**
* Performs a deep copy on other.
*/
public readFile_result(readFile_result other) {
if (other.isSetSuccess()) {
this.success = org.apache.thrift.TBaseHelper.copyBinary(other.success);
}
}
public readFile_result deepCopy() {
return new readFile_result(this);
}
@Override
public void clear() {
this.success = null;
}
public byte[] getSuccess() {
setSuccess(org.apache.thrift.TBaseHelper.rightSize(success));
return success == null ? null : success.array();
}
public java.nio.ByteBuffer bufferForSuccess() {
return org.apache.thrift.TBaseHelper.copyBinary(success);
}
public readFile_result setSuccess(byte[] success) {
this.success = success == null ? (java.nio.ByteBuffer)null : java.nio.ByteBuffer.wrap(success.clone());
return this;
}
public readFile_result setSuccess(@org.apache.thrift.annotation.Nullable java.nio.ByteBuffer success) {
this.success = org.apache.thrift.TBaseHelper.copyBinary(success);
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
if (value instanceof byte[]) {
setSuccess((byte[])value);
} else {
setSuccess((java.nio.ByteBuffer)value);
}
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof readFile_result)
return this.equals((readFile_result)that);
return false;
}
public boolean equals(readFile_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(readFile_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("readFile_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
org.apache.thrift.TBaseHelper.toString(this.success, sb);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class readFile_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public readFile_resultStandardScheme getScheme() {
return new readFile_resultStandardScheme();
}
}
private static class readFile_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, readFile_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRING) {
struct.success = iprot.readBinary();
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, readFile_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
oprot.writeBinary(struct.success);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class readFile_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public readFile_resultTupleScheme getScheme() {
return new readFile_resultTupleScheme();
}
}
private static class readFile_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, readFile_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
oprot.writeBinary(struct.success);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, readFile_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = iprot.readBinary();
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class matchTerm_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("matchTerm_args");
private static final org.apache.thrift.protocol.TField INDEX_FIELD_DESC = new org.apache.thrift.protocol.TField("index", org.apache.thrift.protocol.TType.I64, (short)1);
private static final org.apache.thrift.protocol.TField TERM_FIELD_DESC = new org.apache.thrift.protocol.TField("term", org.apache.thrift.protocol.TType.I64, (short)2);
private static final org.apache.thrift.protocol.TField HEADER_FIELD_DESC = new org.apache.thrift.protocol.TField("header", org.apache.thrift.protocol.TType.STRUCT, (short)3);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new matchTerm_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new matchTerm_argsTupleSchemeFactory();
public long index; // required
public long term; // required
public @org.apache.thrift.annotation.Nullable RaftNode header; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
INDEX((short)1, "index"),
TERM((short)2, "term"),
HEADER((short)3, "header");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // INDEX
return INDEX;
case 2: // TERM
return TERM;
case 3: // HEADER
return HEADER;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __INDEX_ISSET_ID = 0;
private static final int __TERM_ISSET_ID = 1;
private byte __isset_bitfield = 0;
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.INDEX, new org.apache.thrift.meta_data.FieldMetaData("index", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.TERM, new org.apache.thrift.meta_data.FieldMetaData("term", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
tmpMap.put(_Fields.HEADER, new org.apache.thrift.meta_data.FieldMetaData("header", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, RaftNode.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(matchTerm_args.class, metaDataMap);
}
public matchTerm_args() {
}
public matchTerm_args(
long index,
long term,
RaftNode header)
{
this();
this.index = index;
setIndexIsSet(true);
this.term = term;
setTermIsSet(true);
this.header = header;
}
/**
* Performs a deep copy on other.
*/
public matchTerm_args(matchTerm_args other) {
__isset_bitfield = other.__isset_bitfield;
this.index = other.index;
this.term = other.term;
if (other.isSetHeader()) {
this.header = new RaftNode(other.header);
}
}
public matchTerm_args deepCopy() {
return new matchTerm_args(this);
}
@Override
public void clear() {
setIndexIsSet(false);
this.index = 0;
setTermIsSet(false);
this.term = 0;
this.header = null;
}
public long getIndex() {
return this.index;
}
public matchTerm_args setIndex(long index) {
this.index = index;
setIndexIsSet(true);
return this;
}
public void unsetIndex() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __INDEX_ISSET_ID);
}
/** Returns true if field index is set (has been assigned a value) and false otherwise */
public boolean isSetIndex() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __INDEX_ISSET_ID);
}
public void setIndexIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __INDEX_ISSET_ID, value);
}
public long getTerm() {
return this.term;
}
public matchTerm_args setTerm(long term) {
this.term = term;
setTermIsSet(true);
return this;
}
public void unsetTerm() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __TERM_ISSET_ID);
}
/** Returns true if field term is set (has been assigned a value) and false otherwise */
public boolean isSetTerm() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __TERM_ISSET_ID);
}
public void setTermIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __TERM_ISSET_ID, value);
}
@org.apache.thrift.annotation.Nullable
public RaftNode getHeader() {
return this.header;
}
public matchTerm_args setHeader(@org.apache.thrift.annotation.Nullable RaftNode header) {
this.header = header;
return this;
}
public void unsetHeader() {
this.header = null;
}
/** Returns true if field header is set (has been assigned a value) and false otherwise */
public boolean isSetHeader() {
return this.header != null;
}
public void setHeaderIsSet(boolean value) {
if (!value) {
this.header = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case INDEX:
if (value == null) {
unsetIndex();
} else {
setIndex((java.lang.Long)value);
}
break;
case TERM:
if (value == null) {
unsetTerm();
} else {
setTerm((java.lang.Long)value);
}
break;
case HEADER:
if (value == null) {
unsetHeader();
} else {
setHeader((RaftNode)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case INDEX:
return getIndex();
case TERM:
return getTerm();
case HEADER:
return getHeader();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case INDEX:
return isSetIndex();
case TERM:
return isSetTerm();
case HEADER:
return isSetHeader();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof matchTerm_args)
return this.equals((matchTerm_args)that);
return false;
}
public boolean equals(matchTerm_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_index = true;
boolean that_present_index = true;
if (this_present_index || that_present_index) {
if (!(this_present_index && that_present_index))
return false;
if (this.index != that.index)
return false;
}
boolean this_present_term = true;
boolean that_present_term = true;
if (this_present_term || that_present_term) {
if (!(this_present_term && that_present_term))
return false;
if (this.term != that.term)
return false;
}
boolean this_present_header = true && this.isSetHeader();
boolean that_present_header = true && that.isSetHeader();
if (this_present_header || that_present_header) {
if (!(this_present_header && that_present_header))
return false;
if (!this.header.equals(that.header))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(index);
hashCode = hashCode * 8191 + org.apache.thrift.TBaseHelper.hashCode(term);
hashCode = hashCode * 8191 + ((isSetHeader()) ? 131071 : 524287);
if (isSetHeader())
hashCode = hashCode * 8191 + header.hashCode();
return hashCode;
}
@Override
public int compareTo(matchTerm_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetIndex(), other.isSetIndex());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetIndex()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.index, other.index);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetTerm(), other.isSetTerm());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetTerm()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.term, other.term);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.compare(isSetHeader(), other.isSetHeader());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetHeader()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.header, other.header);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("matchTerm_args(");
boolean first = true;
sb.append("index:");
sb.append(this.index);
first = false;
if (!first) sb.append(", ");
sb.append("term:");
sb.append(this.term);
first = false;
if (!first) sb.append(", ");
sb.append("header:");
if (this.header == null) {
sb.append("null");
} else {
sb.append(this.header);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (header != null) {
header.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
// it doesn't seem like you should have to do this, but java serialization is wacky, and doesn't call the default constructor.
__isset_bitfield = 0;
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class matchTerm_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public matchTerm_argsStandardScheme getScheme() {
return new matchTerm_argsStandardScheme();
}
}
private static class matchTerm_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, matchTerm_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // INDEX
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.index = iprot.readI64();
struct.setIndexIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 2: // TERM
if (schemeField.type == org.apache.thrift.protocol.TType.I64) {
struct.term = iprot.readI64();
struct.setTermIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 3: // HEADER
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.header = new RaftNode();
struct.header.read(iprot);
struct.setHeaderIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, matchTerm_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
oprot.writeFieldBegin(INDEX_FIELD_DESC);
oprot.writeI64(struct.index);
oprot.writeFieldEnd();
oprot.writeFieldBegin(TERM_FIELD_DESC);
oprot.writeI64(struct.term);
oprot.writeFieldEnd();
if (struct.header != null) {
oprot.writeFieldBegin(HEADER_FIELD_DESC);
struct.header.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class matchTerm_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public matchTerm_argsTupleScheme getScheme() {
return new matchTerm_argsTupleScheme();
}
}
private static class matchTerm_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, matchTerm_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetIndex()) {
optionals.set(0);
}
if (struct.isSetTerm()) {
optionals.set(1);
}
if (struct.isSetHeader()) {
optionals.set(2);
}
oprot.writeBitSet(optionals, 3);
if (struct.isSetIndex()) {
oprot.writeI64(struct.index);
}
if (struct.isSetTerm()) {
oprot.writeI64(struct.term);
}
if (struct.isSetHeader()) {
struct.header.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, matchTerm_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(3);
if (incoming.get(0)) {
struct.index = iprot.readI64();
struct.setIndexIsSet(true);
}
if (incoming.get(1)) {
struct.term = iprot.readI64();
struct.setTermIsSet(true);
}
if (incoming.get(2)) {
struct.header = new RaftNode();
struct.header.read(iprot);
struct.setHeaderIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class matchTerm_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("matchTerm_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.BOOL, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new matchTerm_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new matchTerm_resultTupleSchemeFactory();
public boolean success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __SUCCESS_ISSET_ID = 0;
private byte __isset_bitfield = 0;
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(matchTerm_result.class, metaDataMap);
}
public matchTerm_result() {
}
public matchTerm_result(
boolean success)
{
this();
this.success = success;
setSuccessIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public matchTerm_result(matchTerm_result other) {
__isset_bitfield = other.__isset_bitfield;
this.success = other.success;
}
public matchTerm_result deepCopy() {
return new matchTerm_result(this);
}
@Override
public void clear() {
setSuccessIsSet(false);
this.success = false;
}
public boolean isSuccess() {
return this.success;
}
public matchTerm_result setSuccess(boolean success) {
this.success = success;
setSuccessIsSet(true);
return this;
}
public void unsetSuccess() {
__isset_bitfield = org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __SUCCESS_ISSET_ID);
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __SUCCESS_ISSET_ID);
}
public void setSuccessIsSet(boolean value) {
__isset_bitfield = org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __SUCCESS_ISSET_ID, value);
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((java.lang.Boolean)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return isSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof matchTerm_result)
return this.equals((matchTerm_result)that);
return false;
}
public boolean equals(matchTerm_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true;
boolean that_present_success = true;
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (this.success != that.success)
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((success) ? 131071 : 524287);
return hashCode;
}
@Override
public int compareTo(matchTerm_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("matchTerm_result(");
boolean first = true;
sb.append("success:");
sb.append(this.success);
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
// it doesn't seem like you should have to do this, but java serialization is wacky, and doesn't call the default constructor.
__isset_bitfield = 0;
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class matchTerm_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public matchTerm_resultStandardScheme getScheme() {
return new matchTerm_resultStandardScheme();
}
}
private static class matchTerm_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, matchTerm_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.BOOL) {
struct.success = iprot.readBool();
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, matchTerm_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.isSetSuccess()) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
oprot.writeBool(struct.success);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class matchTerm_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public matchTerm_resultTupleScheme getScheme() {
return new matchTerm_resultTupleScheme();
}
}
private static class matchTerm_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme