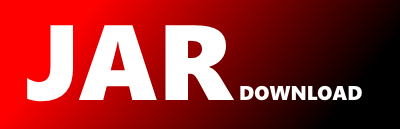
org.apache.iotdb.cluster.rpc.thrift.TSDataService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotdb-thrift-cluster
Show all versions of iotdb-thrift-cluster
RPC (Thrift) framework among servers.
/**
* Autogenerated by Thrift Compiler (0.14.1)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package org.apache.iotdb.cluster.rpc.thrift;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked", "unused"})
@javax.annotation.Generated(value = "Autogenerated by Thrift Compiler (0.14.1)", date = "2022-07-31")
public class TSDataService {
public interface Iface extends RaftService.Iface {
/**
* Query a time series without value filter.
* @return a readerId >= 0 if the query succeeds, otherwise the query fails
* TODO-Cluster: support query multiple series in a request
*
*
* @param request
*/
public long querySingleSeries(SingleSeriesQueryRequest request) throws org.apache.thrift.TException;
/**
* Query mult time series without value filter.
* @return a readerId >= 0 if the query succeeds, otherwise the query fails
*
*
* @param request
*/
public long queryMultSeries(MultSeriesQueryRequest request) throws org.apache.thrift.TException;
/**
* Fetch at max fetchSize time-value pairs using the resultSetId generated by querySingleSeries.
* @return a ByteBuffer containing the serialized time-value pairs or an empty buffer if there
* are not more results.
*
*
* @param header
* @param readerId
*/
public java.nio.ByteBuffer fetchSingleSeries(RaftNode header, long readerId) throws org.apache.thrift.TException;
/**
* Fetch mult series at max fetchSize time-value pairs using the resultSetId generated by querySingleSeries.
* @return a map containing key-value,the serialized time-value pairs or an empty buffer if there
* are not more results.
*
*
* @param header
* @param readerId
* @param paths
*/
public java.util.Map fetchMultSeries(RaftNode header, long readerId, java.util.List paths) throws org.apache.thrift.TException;
/**
* Query a time series and generate an IReaderByTimestamp.
* @return a readerId >= 0 if the query succeeds, otherwise the query fails
*
*
* @param request
*/
public long querySingleSeriesByTimestamp(SingleSeriesQueryRequest request) throws org.apache.thrift.TException;
/**
* Fetch values at given timestamps using the resultSetId generated by
* querySingleSeriesByTimestamp.
* @return a ByteBuffer containing the serialized value or an empty buffer if there
* are not more results.
*
*
* @param header
* @param readerId
* @param timestamps
*/
public java.nio.ByteBuffer fetchSingleSeriesByTimestamps(RaftNode header, long readerId, java.util.List timestamps) throws org.apache.thrift.TException;
/**
* Find the local query established for the remote query and release all its resource.
*
*
* @param header
* @param thisNode
* @param queryId
*/
public void endQuery(RaftNode header, Node thisNode, long queryId) throws org.apache.thrift.TException;
/**
* Given path patterns (paths with wildcard), return all paths they match.
*
*
* @param header
* @param paths
* @param withAlias
*/
public GetAllPathsResult getAllPaths(RaftNode header, java.util.List paths, boolean withAlias) throws org.apache.thrift.TException;
/**
* Given path patterns (paths with wildcard), return all devices they match.
*
*
* @param header
* @param path
* @param isPrefixMatch
*/
public java.util.Set getAllDevices(RaftNode header, java.util.List path, boolean isPrefixMatch) throws org.apache.thrift.TException;
/**
* Get the devices from the header according to the showDevicesPlan
*
*
* @param header
* @param planBinary
*/
public java.nio.ByteBuffer getDevices(RaftNode header, java.nio.ByteBuffer planBinary) throws org.apache.thrift.TException;
public java.util.List getNodeList(RaftNode header, java.lang.String path, int nodeLevel) throws org.apache.thrift.TException;
/**
* Given path patterns(paths with wildcard), return all children nodes they match
*
*
* @param header
* @param path
*/
public java.util.Set getChildNodeInNextLevel(RaftNode header, java.lang.String path) throws org.apache.thrift.TException;
public java.util.Set getChildNodePathInNextLevel(RaftNode header, java.lang.String path) throws org.apache.thrift.TException;
public java.nio.ByteBuffer getAllMeasurementSchema(MeasurementSchemaRequest request) throws org.apache.thrift.TException;
public java.util.List getAggrResult(GetAggrResultRequest request) throws org.apache.thrift.TException;
public java.util.List getUnregisteredTimeseries(RaftNode header, java.util.List timeseriesList) throws org.apache.thrift.TException;
public PullSnapshotResp pullSnapshot(PullSnapshotRequest request) throws org.apache.thrift.TException;
/**
* Create a GroupByExecutor for a path, executing the given aggregations.
* @return the executorId
*
*
* @param request
*/
public long getGroupByExecutor(GroupByRequest request) throws org.apache.thrift.TException;
/**
* Fetch the group by result in the interval [startTime, endTime) from the given executor.
* @return the serialized AggregationResults, each is the result of one of the previously
* required aggregations, and their orders are the same.
*
*
* @param header
* @param executorId
* @param startTime
* @param endTime
*/
public java.util.List getGroupByResult(RaftNode header, long executorId, long startTime, long endTime) throws org.apache.thrift.TException;
/**
* Pull all timeseries schemas prefixed by a given path.
*
*
* @param request
*/
public PullSchemaResp pullTimeSeriesSchema(PullSchemaRequest request) throws org.apache.thrift.TException;
/**
* Pull all measurement schemas prefixed by a given path.
*
*
* @param request
*/
public PullSchemaResp pullMeasurementSchema(PullSchemaRequest request) throws org.apache.thrift.TException;
/**
* Perform a previous fill and return the timevalue pair in binary.
* @return a binary TimeValuePair
*
*
* @param request
*/
public java.nio.ByteBuffer previousFill(PreviousFillRequest request) throws org.apache.thrift.TException;
/**
* Query the last point of a series.
* @return a binary TimeValuePair
*
*
* @param request
*/
public java.nio.ByteBuffer last(LastQueryRequest request) throws org.apache.thrift.TException;
public int getPathCount(RaftNode header, java.util.List pathsToQuery, int level) throws org.apache.thrift.TException;
public int getDeviceCount(RaftNode header, java.util.List pathsToQuery) throws org.apache.thrift.TException;
/**
* During slot transfer, when a member has pulled snapshot from a group, the member will use this
* method to inform the group that one replica of such slots has been pulled.
*
*
* @param header
* @param slots
*/
public boolean onSnapshotApplied(RaftNode header, java.util.List slots) throws org.apache.thrift.TException;
public java.nio.ByteBuffer peekNextNotNullValue(RaftNode header, long executorId, long startTime, long endTime) throws org.apache.thrift.TException;
}
public interface AsyncIface extends RaftService .AsyncIface {
public void querySingleSeries(SingleSeriesQueryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void queryMultSeries(MultSeriesQueryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void fetchSingleSeries(RaftNode header, long readerId, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void fetchMultSeries(RaftNode header, long readerId, java.util.List paths, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException;
public void querySingleSeriesByTimestamp(SingleSeriesQueryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void fetchSingleSeriesByTimestamps(RaftNode header, long readerId, java.util.List timestamps, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void endQuery(RaftNode header, Node thisNode, long queryId, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getAllPaths(RaftNode header, java.util.List paths, boolean withAlias, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getAllDevices(RaftNode header, java.util.List path, boolean isPrefixMatch, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException;
public void getDevices(RaftNode header, java.nio.ByteBuffer planBinary, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getNodeList(RaftNode header, java.lang.String path, int nodeLevel, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException;
public void getChildNodeInNextLevel(RaftNode header, java.lang.String path, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException;
public void getChildNodePathInNextLevel(RaftNode header, java.lang.String path, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException;
public void getAllMeasurementSchema(MeasurementSchemaRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getAggrResult(GetAggrResultRequest request, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException;
public void getUnregisteredTimeseries(RaftNode header, java.util.List timeseriesList, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException;
public void pullSnapshot(PullSnapshotRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getGroupByExecutor(GroupByRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getGroupByResult(RaftNode header, long executorId, long startTime, long endTime, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException;
public void pullTimeSeriesSchema(PullSchemaRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void pullMeasurementSchema(PullSchemaRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void previousFill(PreviousFillRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void last(LastQueryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getPathCount(RaftNode header, java.util.List pathsToQuery, int level, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getDeviceCount(RaftNode header, java.util.List pathsToQuery, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void onSnapshotApplied(RaftNode header, java.util.List slots, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void peekNextNotNullValue(RaftNode header, long executorId, long startTime, long endTime, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
}
public static class Client extends RaftService.Client implements Iface {
public static class Factory implements org.apache.thrift.TServiceClientFactory {
public Factory() {}
public Client getClient(org.apache.thrift.protocol.TProtocol prot) {
return new Client(prot);
}
public Client getClient(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TProtocol oprot) {
return new Client(iprot, oprot);
}
}
public Client(org.apache.thrift.protocol.TProtocol prot)
{
super(prot, prot);
}
public Client(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TProtocol oprot) {
super(iprot, oprot);
}
public long querySingleSeries(SingleSeriesQueryRequest request) throws org.apache.thrift.TException
{
send_querySingleSeries(request);
return recv_querySingleSeries();
}
public void send_querySingleSeries(SingleSeriesQueryRequest request) throws org.apache.thrift.TException
{
querySingleSeries_args args = new querySingleSeries_args();
args.setRequest(request);
sendBase("querySingleSeries", args);
}
public long recv_querySingleSeries() throws org.apache.thrift.TException
{
querySingleSeries_result result = new querySingleSeries_result();
receiveBase(result, "querySingleSeries");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "querySingleSeries failed: unknown result");
}
public long queryMultSeries(MultSeriesQueryRequest request) throws org.apache.thrift.TException
{
send_queryMultSeries(request);
return recv_queryMultSeries();
}
public void send_queryMultSeries(MultSeriesQueryRequest request) throws org.apache.thrift.TException
{
queryMultSeries_args args = new queryMultSeries_args();
args.setRequest(request);
sendBase("queryMultSeries", args);
}
public long recv_queryMultSeries() throws org.apache.thrift.TException
{
queryMultSeries_result result = new queryMultSeries_result();
receiveBase(result, "queryMultSeries");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "queryMultSeries failed: unknown result");
}
public java.nio.ByteBuffer fetchSingleSeries(RaftNode header, long readerId) throws org.apache.thrift.TException
{
send_fetchSingleSeries(header, readerId);
return recv_fetchSingleSeries();
}
public void send_fetchSingleSeries(RaftNode header, long readerId) throws org.apache.thrift.TException
{
fetchSingleSeries_args args = new fetchSingleSeries_args();
args.setHeader(header);
args.setReaderId(readerId);
sendBase("fetchSingleSeries", args);
}
public java.nio.ByteBuffer recv_fetchSingleSeries() throws org.apache.thrift.TException
{
fetchSingleSeries_result result = new fetchSingleSeries_result();
receiveBase(result, "fetchSingleSeries");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "fetchSingleSeries failed: unknown result");
}
public java.util.Map fetchMultSeries(RaftNode header, long readerId, java.util.List paths) throws org.apache.thrift.TException
{
send_fetchMultSeries(header, readerId, paths);
return recv_fetchMultSeries();
}
public void send_fetchMultSeries(RaftNode header, long readerId, java.util.List paths) throws org.apache.thrift.TException
{
fetchMultSeries_args args = new fetchMultSeries_args();
args.setHeader(header);
args.setReaderId(readerId);
args.setPaths(paths);
sendBase("fetchMultSeries", args);
}
public java.util.Map recv_fetchMultSeries() throws org.apache.thrift.TException
{
fetchMultSeries_result result = new fetchMultSeries_result();
receiveBase(result, "fetchMultSeries");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "fetchMultSeries failed: unknown result");
}
public long querySingleSeriesByTimestamp(SingleSeriesQueryRequest request) throws org.apache.thrift.TException
{
send_querySingleSeriesByTimestamp(request);
return recv_querySingleSeriesByTimestamp();
}
public void send_querySingleSeriesByTimestamp(SingleSeriesQueryRequest request) throws org.apache.thrift.TException
{
querySingleSeriesByTimestamp_args args = new querySingleSeriesByTimestamp_args();
args.setRequest(request);
sendBase("querySingleSeriesByTimestamp", args);
}
public long recv_querySingleSeriesByTimestamp() throws org.apache.thrift.TException
{
querySingleSeriesByTimestamp_result result = new querySingleSeriesByTimestamp_result();
receiveBase(result, "querySingleSeriesByTimestamp");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "querySingleSeriesByTimestamp failed: unknown result");
}
public java.nio.ByteBuffer fetchSingleSeriesByTimestamps(RaftNode header, long readerId, java.util.List timestamps) throws org.apache.thrift.TException
{
send_fetchSingleSeriesByTimestamps(header, readerId, timestamps);
return recv_fetchSingleSeriesByTimestamps();
}
public void send_fetchSingleSeriesByTimestamps(RaftNode header, long readerId, java.util.List timestamps) throws org.apache.thrift.TException
{
fetchSingleSeriesByTimestamps_args args = new fetchSingleSeriesByTimestamps_args();
args.setHeader(header);
args.setReaderId(readerId);
args.setTimestamps(timestamps);
sendBase("fetchSingleSeriesByTimestamps", args);
}
public java.nio.ByteBuffer recv_fetchSingleSeriesByTimestamps() throws org.apache.thrift.TException
{
fetchSingleSeriesByTimestamps_result result = new fetchSingleSeriesByTimestamps_result();
receiveBase(result, "fetchSingleSeriesByTimestamps");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "fetchSingleSeriesByTimestamps failed: unknown result");
}
public void endQuery(RaftNode header, Node thisNode, long queryId) throws org.apache.thrift.TException
{
send_endQuery(header, thisNode, queryId);
recv_endQuery();
}
public void send_endQuery(RaftNode header, Node thisNode, long queryId) throws org.apache.thrift.TException
{
endQuery_args args = new endQuery_args();
args.setHeader(header);
args.setThisNode(thisNode);
args.setQueryId(queryId);
sendBase("endQuery", args);
}
public void recv_endQuery() throws org.apache.thrift.TException
{
endQuery_result result = new endQuery_result();
receiveBase(result, "endQuery");
return;
}
public GetAllPathsResult getAllPaths(RaftNode header, java.util.List paths, boolean withAlias) throws org.apache.thrift.TException
{
send_getAllPaths(header, paths, withAlias);
return recv_getAllPaths();
}
public void send_getAllPaths(RaftNode header, java.util.List paths, boolean withAlias) throws org.apache.thrift.TException
{
getAllPaths_args args = new getAllPaths_args();
args.setHeader(header);
args.setPaths(paths);
args.setWithAlias(withAlias);
sendBase("getAllPaths", args);
}
public GetAllPathsResult recv_getAllPaths() throws org.apache.thrift.TException
{
getAllPaths_result result = new getAllPaths_result();
receiveBase(result, "getAllPaths");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getAllPaths failed: unknown result");
}
public java.util.Set getAllDevices(RaftNode header, java.util.List path, boolean isPrefixMatch) throws org.apache.thrift.TException
{
send_getAllDevices(header, path, isPrefixMatch);
return recv_getAllDevices();
}
public void send_getAllDevices(RaftNode header, java.util.List path, boolean isPrefixMatch) throws org.apache.thrift.TException
{
getAllDevices_args args = new getAllDevices_args();
args.setHeader(header);
args.setPath(path);
args.setIsPrefixMatch(isPrefixMatch);
sendBase("getAllDevices", args);
}
public java.util.Set recv_getAllDevices() throws org.apache.thrift.TException
{
getAllDevices_result result = new getAllDevices_result();
receiveBase(result, "getAllDevices");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getAllDevices failed: unknown result");
}
public java.nio.ByteBuffer getDevices(RaftNode header, java.nio.ByteBuffer planBinary) throws org.apache.thrift.TException
{
send_getDevices(header, planBinary);
return recv_getDevices();
}
public void send_getDevices(RaftNode header, java.nio.ByteBuffer planBinary) throws org.apache.thrift.TException
{
getDevices_args args = new getDevices_args();
args.setHeader(header);
args.setPlanBinary(planBinary);
sendBase("getDevices", args);
}
public java.nio.ByteBuffer recv_getDevices() throws org.apache.thrift.TException
{
getDevices_result result = new getDevices_result();
receiveBase(result, "getDevices");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getDevices failed: unknown result");
}
public java.util.List getNodeList(RaftNode header, java.lang.String path, int nodeLevel) throws org.apache.thrift.TException
{
send_getNodeList(header, path, nodeLevel);
return recv_getNodeList();
}
public void send_getNodeList(RaftNode header, java.lang.String path, int nodeLevel) throws org.apache.thrift.TException
{
getNodeList_args args = new getNodeList_args();
args.setHeader(header);
args.setPath(path);
args.setNodeLevel(nodeLevel);
sendBase("getNodeList", args);
}
public java.util.List recv_getNodeList() throws org.apache.thrift.TException
{
getNodeList_result result = new getNodeList_result();
receiveBase(result, "getNodeList");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getNodeList failed: unknown result");
}
public java.util.Set getChildNodeInNextLevel(RaftNode header, java.lang.String path) throws org.apache.thrift.TException
{
send_getChildNodeInNextLevel(header, path);
return recv_getChildNodeInNextLevel();
}
public void send_getChildNodeInNextLevel(RaftNode header, java.lang.String path) throws org.apache.thrift.TException
{
getChildNodeInNextLevel_args args = new getChildNodeInNextLevel_args();
args.setHeader(header);
args.setPath(path);
sendBase("getChildNodeInNextLevel", args);
}
public java.util.Set recv_getChildNodeInNextLevel() throws org.apache.thrift.TException
{
getChildNodeInNextLevel_result result = new getChildNodeInNextLevel_result();
receiveBase(result, "getChildNodeInNextLevel");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getChildNodeInNextLevel failed: unknown result");
}
public java.util.Set getChildNodePathInNextLevel(RaftNode header, java.lang.String path) throws org.apache.thrift.TException
{
send_getChildNodePathInNextLevel(header, path);
return recv_getChildNodePathInNextLevel();
}
public void send_getChildNodePathInNextLevel(RaftNode header, java.lang.String path) throws org.apache.thrift.TException
{
getChildNodePathInNextLevel_args args = new getChildNodePathInNextLevel_args();
args.setHeader(header);
args.setPath(path);
sendBase("getChildNodePathInNextLevel", args);
}
public java.util.Set recv_getChildNodePathInNextLevel() throws org.apache.thrift.TException
{
getChildNodePathInNextLevel_result result = new getChildNodePathInNextLevel_result();
receiveBase(result, "getChildNodePathInNextLevel");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getChildNodePathInNextLevel failed: unknown result");
}
public java.nio.ByteBuffer getAllMeasurementSchema(MeasurementSchemaRequest request) throws org.apache.thrift.TException
{
send_getAllMeasurementSchema(request);
return recv_getAllMeasurementSchema();
}
public void send_getAllMeasurementSchema(MeasurementSchemaRequest request) throws org.apache.thrift.TException
{
getAllMeasurementSchema_args args = new getAllMeasurementSchema_args();
args.setRequest(request);
sendBase("getAllMeasurementSchema", args);
}
public java.nio.ByteBuffer recv_getAllMeasurementSchema() throws org.apache.thrift.TException
{
getAllMeasurementSchema_result result = new getAllMeasurementSchema_result();
receiveBase(result, "getAllMeasurementSchema");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getAllMeasurementSchema failed: unknown result");
}
public java.util.List getAggrResult(GetAggrResultRequest request) throws org.apache.thrift.TException
{
send_getAggrResult(request);
return recv_getAggrResult();
}
public void send_getAggrResult(GetAggrResultRequest request) throws org.apache.thrift.TException
{
getAggrResult_args args = new getAggrResult_args();
args.setRequest(request);
sendBase("getAggrResult", args);
}
public java.util.List recv_getAggrResult() throws org.apache.thrift.TException
{
getAggrResult_result result = new getAggrResult_result();
receiveBase(result, "getAggrResult");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getAggrResult failed: unknown result");
}
public java.util.List getUnregisteredTimeseries(RaftNode header, java.util.List timeseriesList) throws org.apache.thrift.TException
{
send_getUnregisteredTimeseries(header, timeseriesList);
return recv_getUnregisteredTimeseries();
}
public void send_getUnregisteredTimeseries(RaftNode header, java.util.List timeseriesList) throws org.apache.thrift.TException
{
getUnregisteredTimeseries_args args = new getUnregisteredTimeseries_args();
args.setHeader(header);
args.setTimeseriesList(timeseriesList);
sendBase("getUnregisteredTimeseries", args);
}
public java.util.List recv_getUnregisteredTimeseries() throws org.apache.thrift.TException
{
getUnregisteredTimeseries_result result = new getUnregisteredTimeseries_result();
receiveBase(result, "getUnregisteredTimeseries");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getUnregisteredTimeseries failed: unknown result");
}
public PullSnapshotResp pullSnapshot(PullSnapshotRequest request) throws org.apache.thrift.TException
{
send_pullSnapshot(request);
return recv_pullSnapshot();
}
public void send_pullSnapshot(PullSnapshotRequest request) throws org.apache.thrift.TException
{
pullSnapshot_args args = new pullSnapshot_args();
args.setRequest(request);
sendBase("pullSnapshot", args);
}
public PullSnapshotResp recv_pullSnapshot() throws org.apache.thrift.TException
{
pullSnapshot_result result = new pullSnapshot_result();
receiveBase(result, "pullSnapshot");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "pullSnapshot failed: unknown result");
}
public long getGroupByExecutor(GroupByRequest request) throws org.apache.thrift.TException
{
send_getGroupByExecutor(request);
return recv_getGroupByExecutor();
}
public void send_getGroupByExecutor(GroupByRequest request) throws org.apache.thrift.TException
{
getGroupByExecutor_args args = new getGroupByExecutor_args();
args.setRequest(request);
sendBase("getGroupByExecutor", args);
}
public long recv_getGroupByExecutor() throws org.apache.thrift.TException
{
getGroupByExecutor_result result = new getGroupByExecutor_result();
receiveBase(result, "getGroupByExecutor");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getGroupByExecutor failed: unknown result");
}
public java.util.List getGroupByResult(RaftNode header, long executorId, long startTime, long endTime) throws org.apache.thrift.TException
{
send_getGroupByResult(header, executorId, startTime, endTime);
return recv_getGroupByResult();
}
public void send_getGroupByResult(RaftNode header, long executorId, long startTime, long endTime) throws org.apache.thrift.TException
{
getGroupByResult_args args = new getGroupByResult_args();
args.setHeader(header);
args.setExecutorId(executorId);
args.setStartTime(startTime);
args.setEndTime(endTime);
sendBase("getGroupByResult", args);
}
public java.util.List recv_getGroupByResult() throws org.apache.thrift.TException
{
getGroupByResult_result result = new getGroupByResult_result();
receiveBase(result, "getGroupByResult");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getGroupByResult failed: unknown result");
}
public PullSchemaResp pullTimeSeriesSchema(PullSchemaRequest request) throws org.apache.thrift.TException
{
send_pullTimeSeriesSchema(request);
return recv_pullTimeSeriesSchema();
}
public void send_pullTimeSeriesSchema(PullSchemaRequest request) throws org.apache.thrift.TException
{
pullTimeSeriesSchema_args args = new pullTimeSeriesSchema_args();
args.setRequest(request);
sendBase("pullTimeSeriesSchema", args);
}
public PullSchemaResp recv_pullTimeSeriesSchema() throws org.apache.thrift.TException
{
pullTimeSeriesSchema_result result = new pullTimeSeriesSchema_result();
receiveBase(result, "pullTimeSeriesSchema");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "pullTimeSeriesSchema failed: unknown result");
}
public PullSchemaResp pullMeasurementSchema(PullSchemaRequest request) throws org.apache.thrift.TException
{
send_pullMeasurementSchema(request);
return recv_pullMeasurementSchema();
}
public void send_pullMeasurementSchema(PullSchemaRequest request) throws org.apache.thrift.TException
{
pullMeasurementSchema_args args = new pullMeasurementSchema_args();
args.setRequest(request);
sendBase("pullMeasurementSchema", args);
}
public PullSchemaResp recv_pullMeasurementSchema() throws org.apache.thrift.TException
{
pullMeasurementSchema_result result = new pullMeasurementSchema_result();
receiveBase(result, "pullMeasurementSchema");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "pullMeasurementSchema failed: unknown result");
}
public java.nio.ByteBuffer previousFill(PreviousFillRequest request) throws org.apache.thrift.TException
{
send_previousFill(request);
return recv_previousFill();
}
public void send_previousFill(PreviousFillRequest request) throws org.apache.thrift.TException
{
previousFill_args args = new previousFill_args();
args.setRequest(request);
sendBase("previousFill", args);
}
public java.nio.ByteBuffer recv_previousFill() throws org.apache.thrift.TException
{
previousFill_result result = new previousFill_result();
receiveBase(result, "previousFill");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "previousFill failed: unknown result");
}
public java.nio.ByteBuffer last(LastQueryRequest request) throws org.apache.thrift.TException
{
send_last(request);
return recv_last();
}
public void send_last(LastQueryRequest request) throws org.apache.thrift.TException
{
last_args args = new last_args();
args.setRequest(request);
sendBase("last", args);
}
public java.nio.ByteBuffer recv_last() throws org.apache.thrift.TException
{
last_result result = new last_result();
receiveBase(result, "last");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "last failed: unknown result");
}
public int getPathCount(RaftNode header, java.util.List pathsToQuery, int level) throws org.apache.thrift.TException
{
send_getPathCount(header, pathsToQuery, level);
return recv_getPathCount();
}
public void send_getPathCount(RaftNode header, java.util.List pathsToQuery, int level) throws org.apache.thrift.TException
{
getPathCount_args args = new getPathCount_args();
args.setHeader(header);
args.setPathsToQuery(pathsToQuery);
args.setLevel(level);
sendBase("getPathCount", args);
}
public int recv_getPathCount() throws org.apache.thrift.TException
{
getPathCount_result result = new getPathCount_result();
receiveBase(result, "getPathCount");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getPathCount failed: unknown result");
}
public int getDeviceCount(RaftNode header, java.util.List pathsToQuery) throws org.apache.thrift.TException
{
send_getDeviceCount(header, pathsToQuery);
return recv_getDeviceCount();
}
public void send_getDeviceCount(RaftNode header, java.util.List pathsToQuery) throws org.apache.thrift.TException
{
getDeviceCount_args args = new getDeviceCount_args();
args.setHeader(header);
args.setPathsToQuery(pathsToQuery);
sendBase("getDeviceCount", args);
}
public int recv_getDeviceCount() throws org.apache.thrift.TException
{
getDeviceCount_result result = new getDeviceCount_result();
receiveBase(result, "getDeviceCount");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getDeviceCount failed: unknown result");
}
public boolean onSnapshotApplied(RaftNode header, java.util.List slots) throws org.apache.thrift.TException
{
send_onSnapshotApplied(header, slots);
return recv_onSnapshotApplied();
}
public void send_onSnapshotApplied(RaftNode header, java.util.List slots) throws org.apache.thrift.TException
{
onSnapshotApplied_args args = new onSnapshotApplied_args();
args.setHeader(header);
args.setSlots(slots);
sendBase("onSnapshotApplied", args);
}
public boolean recv_onSnapshotApplied() throws org.apache.thrift.TException
{
onSnapshotApplied_result result = new onSnapshotApplied_result();
receiveBase(result, "onSnapshotApplied");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "onSnapshotApplied failed: unknown result");
}
public java.nio.ByteBuffer peekNextNotNullValue(RaftNode header, long executorId, long startTime, long endTime) throws org.apache.thrift.TException
{
send_peekNextNotNullValue(header, executorId, startTime, endTime);
return recv_peekNextNotNullValue();
}
public void send_peekNextNotNullValue(RaftNode header, long executorId, long startTime, long endTime) throws org.apache.thrift.TException
{
peekNextNotNullValue_args args = new peekNextNotNullValue_args();
args.setHeader(header);
args.setExecutorId(executorId);
args.setStartTime(startTime);
args.setEndTime(endTime);
sendBase("peekNextNotNullValue", args);
}
public java.nio.ByteBuffer recv_peekNextNotNullValue() throws org.apache.thrift.TException
{
peekNextNotNullValue_result result = new peekNextNotNullValue_result();
receiveBase(result, "peekNextNotNullValue");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "peekNextNotNullValue failed: unknown result");
}
}
public static class AsyncClient extends RaftService.AsyncClient implements AsyncIface {
public static class Factory implements org.apache.thrift.async.TAsyncClientFactory {
private org.apache.thrift.async.TAsyncClientManager clientManager;
private org.apache.thrift.protocol.TProtocolFactory protocolFactory;
public Factory(org.apache.thrift.async.TAsyncClientManager clientManager, org.apache.thrift.protocol.TProtocolFactory protocolFactory) {
this.clientManager = clientManager;
this.protocolFactory = protocolFactory;
}
public AsyncClient getAsyncClient(org.apache.thrift.transport.TNonblockingTransport transport) {
return new AsyncClient(protocolFactory, clientManager, transport);
}
}
public AsyncClient(org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.async.TAsyncClientManager clientManager, org.apache.thrift.transport.TNonblockingTransport transport) {
super(protocolFactory, clientManager, transport);
}
public void querySingleSeries(SingleSeriesQueryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
querySingleSeries_call method_call = new querySingleSeries_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class querySingleSeries_call extends org.apache.thrift.async.TAsyncMethodCall {
private SingleSeriesQueryRequest request;
public querySingleSeries_call(SingleSeriesQueryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("querySingleSeries", org.apache.thrift.protocol.TMessageType.CALL, 0));
querySingleSeries_args args = new querySingleSeries_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public java.lang.Long getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_querySingleSeries();
}
}
public void queryMultSeries(MultSeriesQueryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
queryMultSeries_call method_call = new queryMultSeries_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class queryMultSeries_call extends org.apache.thrift.async.TAsyncMethodCall {
private MultSeriesQueryRequest request;
public queryMultSeries_call(MultSeriesQueryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("queryMultSeries", org.apache.thrift.protocol.TMessageType.CALL, 0));
queryMultSeries_args args = new queryMultSeries_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public java.lang.Long getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_queryMultSeries();
}
}
public void fetchSingleSeries(RaftNode header, long readerId, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
fetchSingleSeries_call method_call = new fetchSingleSeries_call(header, readerId, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class fetchSingleSeries_call extends org.apache.thrift.async.TAsyncMethodCall {
private RaftNode header;
private long readerId;
public fetchSingleSeries_call(RaftNode header, long readerId, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.readerId = readerId;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("fetchSingleSeries", org.apache.thrift.protocol.TMessageType.CALL, 0));
fetchSingleSeries_args args = new fetchSingleSeries_args();
args.setHeader(header);
args.setReaderId(readerId);
args.write(prot);
prot.writeMessageEnd();
}
public java.nio.ByteBuffer getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_fetchSingleSeries();
}
}
public void fetchMultSeries(RaftNode header, long readerId, java.util.List paths, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException {
checkReady();
fetchMultSeries_call method_call = new fetchMultSeries_call(header, readerId, paths, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class fetchMultSeries_call extends org.apache.thrift.async.TAsyncMethodCall> {
private RaftNode header;
private long readerId;
private java.util.List paths;
public fetchMultSeries_call(RaftNode header, long readerId, java.util.List paths, org.apache.thrift.async.AsyncMethodCallback> resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.readerId = readerId;
this.paths = paths;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("fetchMultSeries", org.apache.thrift.protocol.TMessageType.CALL, 0));
fetchMultSeries_args args = new fetchMultSeries_args();
args.setHeader(header);
args.setReaderId(readerId);
args.setPaths(paths);
args.write(prot);
prot.writeMessageEnd();
}
public java.util.Map getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_fetchMultSeries();
}
}
public void querySingleSeriesByTimestamp(SingleSeriesQueryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
querySingleSeriesByTimestamp_call method_call = new querySingleSeriesByTimestamp_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class querySingleSeriesByTimestamp_call extends org.apache.thrift.async.TAsyncMethodCall {
private SingleSeriesQueryRequest request;
public querySingleSeriesByTimestamp_call(SingleSeriesQueryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("querySingleSeriesByTimestamp", org.apache.thrift.protocol.TMessageType.CALL, 0));
querySingleSeriesByTimestamp_args args = new querySingleSeriesByTimestamp_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public java.lang.Long getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_querySingleSeriesByTimestamp();
}
}
public void fetchSingleSeriesByTimestamps(RaftNode header, long readerId, java.util.List timestamps, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
fetchSingleSeriesByTimestamps_call method_call = new fetchSingleSeriesByTimestamps_call(header, readerId, timestamps, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class fetchSingleSeriesByTimestamps_call extends org.apache.thrift.async.TAsyncMethodCall {
private RaftNode header;
private long readerId;
private java.util.List timestamps;
public fetchSingleSeriesByTimestamps_call(RaftNode header, long readerId, java.util.List timestamps, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.readerId = readerId;
this.timestamps = timestamps;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("fetchSingleSeriesByTimestamps", org.apache.thrift.protocol.TMessageType.CALL, 0));
fetchSingleSeriesByTimestamps_args args = new fetchSingleSeriesByTimestamps_args();
args.setHeader(header);
args.setReaderId(readerId);
args.setTimestamps(timestamps);
args.write(prot);
prot.writeMessageEnd();
}
public java.nio.ByteBuffer getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_fetchSingleSeriesByTimestamps();
}
}
public void endQuery(RaftNode header, Node thisNode, long queryId, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
endQuery_call method_call = new endQuery_call(header, thisNode, queryId, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class endQuery_call extends org.apache.thrift.async.TAsyncMethodCall {
private RaftNode header;
private Node thisNode;
private long queryId;
public endQuery_call(RaftNode header, Node thisNode, long queryId, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.thisNode = thisNode;
this.queryId = queryId;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("endQuery", org.apache.thrift.protocol.TMessageType.CALL, 0));
endQuery_args args = new endQuery_args();
args.setHeader(header);
args.setThisNode(thisNode);
args.setQueryId(queryId);
args.write(prot);
prot.writeMessageEnd();
}
public Void getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return null;
}
}
public void getAllPaths(RaftNode header, java.util.List paths, boolean withAlias, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getAllPaths_call method_call = new getAllPaths_call(header, paths, withAlias, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getAllPaths_call extends org.apache.thrift.async.TAsyncMethodCall {
private RaftNode header;
private java.util.List paths;
private boolean withAlias;
public getAllPaths_call(RaftNode header, java.util.List paths, boolean withAlias, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.paths = paths;
this.withAlias = withAlias;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getAllPaths", org.apache.thrift.protocol.TMessageType.CALL, 0));
getAllPaths_args args = new getAllPaths_args();
args.setHeader(header);
args.setPaths(paths);
args.setWithAlias(withAlias);
args.write(prot);
prot.writeMessageEnd();
}
public GetAllPathsResult getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getAllPaths();
}
}
public void getAllDevices(RaftNode header, java.util.List path, boolean isPrefixMatch, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException {
checkReady();
getAllDevices_call method_call = new getAllDevices_call(header, path, isPrefixMatch, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getAllDevices_call extends org.apache.thrift.async.TAsyncMethodCall> {
private RaftNode header;
private java.util.List path;
private boolean isPrefixMatch;
public getAllDevices_call(RaftNode header, java.util.List path, boolean isPrefixMatch, org.apache.thrift.async.AsyncMethodCallback> resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.path = path;
this.isPrefixMatch = isPrefixMatch;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getAllDevices", org.apache.thrift.protocol.TMessageType.CALL, 0));
getAllDevices_args args = new getAllDevices_args();
args.setHeader(header);
args.setPath(path);
args.setIsPrefixMatch(isPrefixMatch);
args.write(prot);
prot.writeMessageEnd();
}
public java.util.Set getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getAllDevices();
}
}
public void getDevices(RaftNode header, java.nio.ByteBuffer planBinary, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getDevices_call method_call = new getDevices_call(header, planBinary, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getDevices_call extends org.apache.thrift.async.TAsyncMethodCall {
private RaftNode header;
private java.nio.ByteBuffer planBinary;
public getDevices_call(RaftNode header, java.nio.ByteBuffer planBinary, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.planBinary = planBinary;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getDevices", org.apache.thrift.protocol.TMessageType.CALL, 0));
getDevices_args args = new getDevices_args();
args.setHeader(header);
args.setPlanBinary(planBinary);
args.write(prot);
prot.writeMessageEnd();
}
public java.nio.ByteBuffer getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getDevices();
}
}
public void getNodeList(RaftNode header, java.lang.String path, int nodeLevel, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException {
checkReady();
getNodeList_call method_call = new getNodeList_call(header, path, nodeLevel, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getNodeList_call extends org.apache.thrift.async.TAsyncMethodCall> {
private RaftNode header;
private java.lang.String path;
private int nodeLevel;
public getNodeList_call(RaftNode header, java.lang.String path, int nodeLevel, org.apache.thrift.async.AsyncMethodCallback> resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.path = path;
this.nodeLevel = nodeLevel;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getNodeList", org.apache.thrift.protocol.TMessageType.CALL, 0));
getNodeList_args args = new getNodeList_args();
args.setHeader(header);
args.setPath(path);
args.setNodeLevel(nodeLevel);
args.write(prot);
prot.writeMessageEnd();
}
public java.util.List getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getNodeList();
}
}
public void getChildNodeInNextLevel(RaftNode header, java.lang.String path, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException {
checkReady();
getChildNodeInNextLevel_call method_call = new getChildNodeInNextLevel_call(header, path, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getChildNodeInNextLevel_call extends org.apache.thrift.async.TAsyncMethodCall> {
private RaftNode header;
private java.lang.String path;
public getChildNodeInNextLevel_call(RaftNode header, java.lang.String path, org.apache.thrift.async.AsyncMethodCallback> resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.path = path;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getChildNodeInNextLevel", org.apache.thrift.protocol.TMessageType.CALL, 0));
getChildNodeInNextLevel_args args = new getChildNodeInNextLevel_args();
args.setHeader(header);
args.setPath(path);
args.write(prot);
prot.writeMessageEnd();
}
public java.util.Set getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getChildNodeInNextLevel();
}
}
public void getChildNodePathInNextLevel(RaftNode header, java.lang.String path, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException {
checkReady();
getChildNodePathInNextLevel_call method_call = new getChildNodePathInNextLevel_call(header, path, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getChildNodePathInNextLevel_call extends org.apache.thrift.async.TAsyncMethodCall> {
private RaftNode header;
private java.lang.String path;
public getChildNodePathInNextLevel_call(RaftNode header, java.lang.String path, org.apache.thrift.async.AsyncMethodCallback> resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.path = path;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getChildNodePathInNextLevel", org.apache.thrift.protocol.TMessageType.CALL, 0));
getChildNodePathInNextLevel_args args = new getChildNodePathInNextLevel_args();
args.setHeader(header);
args.setPath(path);
args.write(prot);
prot.writeMessageEnd();
}
public java.util.Set getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getChildNodePathInNextLevel();
}
}
public void getAllMeasurementSchema(MeasurementSchemaRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getAllMeasurementSchema_call method_call = new getAllMeasurementSchema_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getAllMeasurementSchema_call extends org.apache.thrift.async.TAsyncMethodCall {
private MeasurementSchemaRequest request;
public getAllMeasurementSchema_call(MeasurementSchemaRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getAllMeasurementSchema", org.apache.thrift.protocol.TMessageType.CALL, 0));
getAllMeasurementSchema_args args = new getAllMeasurementSchema_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public java.nio.ByteBuffer getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getAllMeasurementSchema();
}
}
public void getAggrResult(GetAggrResultRequest request, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException {
checkReady();
getAggrResult_call method_call = new getAggrResult_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getAggrResult_call extends org.apache.thrift.async.TAsyncMethodCall> {
private GetAggrResultRequest request;
public getAggrResult_call(GetAggrResultRequest request, org.apache.thrift.async.AsyncMethodCallback> resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getAggrResult", org.apache.thrift.protocol.TMessageType.CALL, 0));
getAggrResult_args args = new getAggrResult_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public java.util.List getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getAggrResult();
}
}
public void getUnregisteredTimeseries(RaftNode header, java.util.List timeseriesList, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException {
checkReady();
getUnregisteredTimeseries_call method_call = new getUnregisteredTimeseries_call(header, timeseriesList, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getUnregisteredTimeseries_call extends org.apache.thrift.async.TAsyncMethodCall> {
private RaftNode header;
private java.util.List timeseriesList;
public getUnregisteredTimeseries_call(RaftNode header, java.util.List timeseriesList, org.apache.thrift.async.AsyncMethodCallback> resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.timeseriesList = timeseriesList;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getUnregisteredTimeseries", org.apache.thrift.protocol.TMessageType.CALL, 0));
getUnregisteredTimeseries_args args = new getUnregisteredTimeseries_args();
args.setHeader(header);
args.setTimeseriesList(timeseriesList);
args.write(prot);
prot.writeMessageEnd();
}
public java.util.List getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getUnregisteredTimeseries();
}
}
public void pullSnapshot(PullSnapshotRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
pullSnapshot_call method_call = new pullSnapshot_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class pullSnapshot_call extends org.apache.thrift.async.TAsyncMethodCall {
private PullSnapshotRequest request;
public pullSnapshot_call(PullSnapshotRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("pullSnapshot", org.apache.thrift.protocol.TMessageType.CALL, 0));
pullSnapshot_args args = new pullSnapshot_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public PullSnapshotResp getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_pullSnapshot();
}
}
public void getGroupByExecutor(GroupByRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getGroupByExecutor_call method_call = new getGroupByExecutor_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getGroupByExecutor_call extends org.apache.thrift.async.TAsyncMethodCall {
private GroupByRequest request;
public getGroupByExecutor_call(GroupByRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getGroupByExecutor", org.apache.thrift.protocol.TMessageType.CALL, 0));
getGroupByExecutor_args args = new getGroupByExecutor_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public java.lang.Long getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getGroupByExecutor();
}
}
public void getGroupByResult(RaftNode header, long executorId, long startTime, long endTime, org.apache.thrift.async.AsyncMethodCallback> resultHandler) throws org.apache.thrift.TException {
checkReady();
getGroupByResult_call method_call = new getGroupByResult_call(header, executorId, startTime, endTime, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getGroupByResult_call extends org.apache.thrift.async.TAsyncMethodCall> {
private RaftNode header;
private long executorId;
private long startTime;
private long endTime;
public getGroupByResult_call(RaftNode header, long executorId, long startTime, long endTime, org.apache.thrift.async.AsyncMethodCallback> resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.executorId = executorId;
this.startTime = startTime;
this.endTime = endTime;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getGroupByResult", org.apache.thrift.protocol.TMessageType.CALL, 0));
getGroupByResult_args args = new getGroupByResult_args();
args.setHeader(header);
args.setExecutorId(executorId);
args.setStartTime(startTime);
args.setEndTime(endTime);
args.write(prot);
prot.writeMessageEnd();
}
public java.util.List getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getGroupByResult();
}
}
public void pullTimeSeriesSchema(PullSchemaRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
pullTimeSeriesSchema_call method_call = new pullTimeSeriesSchema_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class pullTimeSeriesSchema_call extends org.apache.thrift.async.TAsyncMethodCall {
private PullSchemaRequest request;
public pullTimeSeriesSchema_call(PullSchemaRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("pullTimeSeriesSchema", org.apache.thrift.protocol.TMessageType.CALL, 0));
pullTimeSeriesSchema_args args = new pullTimeSeriesSchema_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public PullSchemaResp getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_pullTimeSeriesSchema();
}
}
public void pullMeasurementSchema(PullSchemaRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
pullMeasurementSchema_call method_call = new pullMeasurementSchema_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class pullMeasurementSchema_call extends org.apache.thrift.async.TAsyncMethodCall {
private PullSchemaRequest request;
public pullMeasurementSchema_call(PullSchemaRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("pullMeasurementSchema", org.apache.thrift.protocol.TMessageType.CALL, 0));
pullMeasurementSchema_args args = new pullMeasurementSchema_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public PullSchemaResp getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_pullMeasurementSchema();
}
}
public void previousFill(PreviousFillRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
previousFill_call method_call = new previousFill_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class previousFill_call extends org.apache.thrift.async.TAsyncMethodCall {
private PreviousFillRequest request;
public previousFill_call(PreviousFillRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("previousFill", org.apache.thrift.protocol.TMessageType.CALL, 0));
previousFill_args args = new previousFill_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public java.nio.ByteBuffer getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_previousFill();
}
}
public void last(LastQueryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
last_call method_call = new last_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class last_call extends org.apache.thrift.async.TAsyncMethodCall {
private LastQueryRequest request;
public last_call(LastQueryRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("last", org.apache.thrift.protocol.TMessageType.CALL, 0));
last_args args = new last_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public java.nio.ByteBuffer getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_last();
}
}
public void getPathCount(RaftNode header, java.util.List pathsToQuery, int level, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getPathCount_call method_call = new getPathCount_call(header, pathsToQuery, level, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getPathCount_call extends org.apache.thrift.async.TAsyncMethodCall {
private RaftNode header;
private java.util.List pathsToQuery;
private int level;
public getPathCount_call(RaftNode header, java.util.List pathsToQuery, int level, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.pathsToQuery = pathsToQuery;
this.level = level;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getPathCount", org.apache.thrift.protocol.TMessageType.CALL, 0));
getPathCount_args args = new getPathCount_args();
args.setHeader(header);
args.setPathsToQuery(pathsToQuery);
args.setLevel(level);
args.write(prot);
prot.writeMessageEnd();
}
public java.lang.Integer getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getPathCount();
}
}
public void getDeviceCount(RaftNode header, java.util.List pathsToQuery, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getDeviceCount_call method_call = new getDeviceCount_call(header, pathsToQuery, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getDeviceCount_call extends org.apache.thrift.async.TAsyncMethodCall {
private RaftNode header;
private java.util.List pathsToQuery;
public getDeviceCount_call(RaftNode header, java.util.List pathsToQuery, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.pathsToQuery = pathsToQuery;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getDeviceCount", org.apache.thrift.protocol.TMessageType.CALL, 0));
getDeviceCount_args args = new getDeviceCount_args();
args.setHeader(header);
args.setPathsToQuery(pathsToQuery);
args.write(prot);
prot.writeMessageEnd();
}
public java.lang.Integer getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getDeviceCount();
}
}
public void onSnapshotApplied(RaftNode header, java.util.List slots, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
onSnapshotApplied_call method_call = new onSnapshotApplied_call(header, slots, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class onSnapshotApplied_call extends org.apache.thrift.async.TAsyncMethodCall {
private RaftNode header;
private java.util.List slots;
public onSnapshotApplied_call(RaftNode header, java.util.List slots, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.slots = slots;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("onSnapshotApplied", org.apache.thrift.protocol.TMessageType.CALL, 0));
onSnapshotApplied_args args = new onSnapshotApplied_args();
args.setHeader(header);
args.setSlots(slots);
args.write(prot);
prot.writeMessageEnd();
}
public java.lang.Boolean getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_onSnapshotApplied();
}
}
public void peekNextNotNullValue(RaftNode header, long executorId, long startTime, long endTime, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
peekNextNotNullValue_call method_call = new peekNextNotNullValue_call(header, executorId, startTime, endTime, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class peekNextNotNullValue_call extends org.apache.thrift.async.TAsyncMethodCall {
private RaftNode header;
private long executorId;
private long startTime;
private long endTime;
public peekNextNotNullValue_call(RaftNode header, long executorId, long startTime, long endTime, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.header = header;
this.executorId = executorId;
this.startTime = startTime;
this.endTime = endTime;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("peekNextNotNullValue", org.apache.thrift.protocol.TMessageType.CALL, 0));
peekNextNotNullValue_args args = new peekNextNotNullValue_args();
args.setHeader(header);
args.setExecutorId(executorId);
args.setStartTime(startTime);
args.setEndTime(endTime);
args.write(prot);
prot.writeMessageEnd();
}
public java.nio.ByteBuffer getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_peekNextNotNullValue();
}
}
}
public static class Processor extends RaftService.Processor implements org.apache.thrift.TProcessor {
private static final org.slf4j.Logger _LOGGER = org.slf4j.LoggerFactory.getLogger(Processor.class.getName());
public Processor(I iface) {
super(iface, getProcessMap(new java.util.HashMap>()));
}
protected Processor(I iface, java.util.Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static java.util.Map> getProcessMap(java.util.Map> processMap) {
processMap.put("querySingleSeries", new querySingleSeries());
processMap.put("queryMultSeries", new queryMultSeries());
processMap.put("fetchSingleSeries", new fetchSingleSeries());
processMap.put("fetchMultSeries", new fetchMultSeries());
processMap.put("querySingleSeriesByTimestamp", new querySingleSeriesByTimestamp());
processMap.put("fetchSingleSeriesByTimestamps", new fetchSingleSeriesByTimestamps());
processMap.put("endQuery", new endQuery());
processMap.put("getAllPaths", new getAllPaths());
processMap.put("getAllDevices", new getAllDevices());
processMap.put("getDevices", new getDevices());
processMap.put("getNodeList", new getNodeList());
processMap.put("getChildNodeInNextLevel", new getChildNodeInNextLevel());
processMap.put("getChildNodePathInNextLevel", new getChildNodePathInNextLevel());
processMap.put("getAllMeasurementSchema", new getAllMeasurementSchema());
processMap.put("getAggrResult", new getAggrResult());
processMap.put("getUnregisteredTimeseries", new getUnregisteredTimeseries());
processMap.put("pullSnapshot", new pullSnapshot());
processMap.put("getGroupByExecutor", new getGroupByExecutor());
processMap.put("getGroupByResult", new getGroupByResult());
processMap.put("pullTimeSeriesSchema", new pullTimeSeriesSchema());
processMap.put("pullMeasurementSchema", new pullMeasurementSchema());
processMap.put("previousFill", new previousFill());
processMap.put("last", new last());
processMap.put("getPathCount", new getPathCount());
processMap.put("getDeviceCount", new getDeviceCount());
processMap.put("onSnapshotApplied", new onSnapshotApplied());
processMap.put("peekNextNotNullValue", new peekNextNotNullValue());
return processMap;
}
public static class querySingleSeries extends org.apache.thrift.ProcessFunction {
public querySingleSeries() {
super("querySingleSeries");
}
public querySingleSeries_args getEmptyArgsInstance() {
return new querySingleSeries_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public querySingleSeries_result getResult(I iface, querySingleSeries_args args) throws org.apache.thrift.TException {
querySingleSeries_result result = new querySingleSeries_result();
result.success = iface.querySingleSeries(args.request);
result.setSuccessIsSet(true);
return result;
}
}
public static class queryMultSeries extends org.apache.thrift.ProcessFunction {
public queryMultSeries() {
super("queryMultSeries");
}
public queryMultSeries_args getEmptyArgsInstance() {
return new queryMultSeries_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public queryMultSeries_result getResult(I iface, queryMultSeries_args args) throws org.apache.thrift.TException {
queryMultSeries_result result = new queryMultSeries_result();
result.success = iface.queryMultSeries(args.request);
result.setSuccessIsSet(true);
return result;
}
}
public static class fetchSingleSeries extends org.apache.thrift.ProcessFunction {
public fetchSingleSeries() {
super("fetchSingleSeries");
}
public fetchSingleSeries_args getEmptyArgsInstance() {
return new fetchSingleSeries_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public fetchSingleSeries_result getResult(I iface, fetchSingleSeries_args args) throws org.apache.thrift.TException {
fetchSingleSeries_result result = new fetchSingleSeries_result();
result.success = iface.fetchSingleSeries(args.header, args.readerId);
return result;
}
}
public static class fetchMultSeries extends org.apache.thrift.ProcessFunction {
public fetchMultSeries() {
super("fetchMultSeries");
}
public fetchMultSeries_args getEmptyArgsInstance() {
return new fetchMultSeries_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public fetchMultSeries_result getResult(I iface, fetchMultSeries_args args) throws org.apache.thrift.TException {
fetchMultSeries_result result = new fetchMultSeries_result();
result.success = iface.fetchMultSeries(args.header, args.readerId, args.paths);
return result;
}
}
public static class querySingleSeriesByTimestamp extends org.apache.thrift.ProcessFunction {
public querySingleSeriesByTimestamp() {
super("querySingleSeriesByTimestamp");
}
public querySingleSeriesByTimestamp_args getEmptyArgsInstance() {
return new querySingleSeriesByTimestamp_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public querySingleSeriesByTimestamp_result getResult(I iface, querySingleSeriesByTimestamp_args args) throws org.apache.thrift.TException {
querySingleSeriesByTimestamp_result result = new querySingleSeriesByTimestamp_result();
result.success = iface.querySingleSeriesByTimestamp(args.request);
result.setSuccessIsSet(true);
return result;
}
}
public static class fetchSingleSeriesByTimestamps extends org.apache.thrift.ProcessFunction {
public fetchSingleSeriesByTimestamps() {
super("fetchSingleSeriesByTimestamps");
}
public fetchSingleSeriesByTimestamps_args getEmptyArgsInstance() {
return new fetchSingleSeriesByTimestamps_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public fetchSingleSeriesByTimestamps_result getResult(I iface, fetchSingleSeriesByTimestamps_args args) throws org.apache.thrift.TException {
fetchSingleSeriesByTimestamps_result result = new fetchSingleSeriesByTimestamps_result();
result.success = iface.fetchSingleSeriesByTimestamps(args.header, args.readerId, args.timestamps);
return result;
}
}
public static class endQuery extends org.apache.thrift.ProcessFunction {
public endQuery() {
super("endQuery");
}
public endQuery_args getEmptyArgsInstance() {
return new endQuery_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public endQuery_result getResult(I iface, endQuery_args args) throws org.apache.thrift.TException {
endQuery_result result = new endQuery_result();
iface.endQuery(args.header, args.thisNode, args.queryId);
return result;
}
}
public static class getAllPaths extends org.apache.thrift.ProcessFunction {
public getAllPaths() {
super("getAllPaths");
}
public getAllPaths_args getEmptyArgsInstance() {
return new getAllPaths_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public getAllPaths_result getResult(I iface, getAllPaths_args args) throws org.apache.thrift.TException {
getAllPaths_result result = new getAllPaths_result();
result.success = iface.getAllPaths(args.header, args.paths, args.withAlias);
return result;
}
}
public static class getAllDevices extends org.apache.thrift.ProcessFunction {
public getAllDevices() {
super("getAllDevices");
}
public getAllDevices_args getEmptyArgsInstance() {
return new getAllDevices_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public getAllDevices_result getResult(I iface, getAllDevices_args args) throws org.apache.thrift.TException {
getAllDevices_result result = new getAllDevices_result();
result.success = iface.getAllDevices(args.header, args.path, args.isPrefixMatch);
return result;
}
}
public static class getDevices extends org.apache.thrift.ProcessFunction {
public getDevices() {
super("getDevices");
}
public getDevices_args getEmptyArgsInstance() {
return new getDevices_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public getDevices_result getResult(I iface, getDevices_args args) throws org.apache.thrift.TException {
getDevices_result result = new getDevices_result();
result.success = iface.getDevices(args.header, args.planBinary);
return result;
}
}
public static class getNodeList extends org.apache.thrift.ProcessFunction {
public getNodeList() {
super("getNodeList");
}
public getNodeList_args getEmptyArgsInstance() {
return new getNodeList_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public getNodeList_result getResult(I iface, getNodeList_args args) throws org.apache.thrift.TException {
getNodeList_result result = new getNodeList_result();
result.success = iface.getNodeList(args.header, args.path, args.nodeLevel);
return result;
}
}
public static class getChildNodeInNextLevel extends org.apache.thrift.ProcessFunction {
public getChildNodeInNextLevel() {
super("getChildNodeInNextLevel");
}
public getChildNodeInNextLevel_args getEmptyArgsInstance() {
return new getChildNodeInNextLevel_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public getChildNodeInNextLevel_result getResult(I iface, getChildNodeInNextLevel_args args) throws org.apache.thrift.TException {
getChildNodeInNextLevel_result result = new getChildNodeInNextLevel_result();
result.success = iface.getChildNodeInNextLevel(args.header, args.path);
return result;
}
}
public static class getChildNodePathInNextLevel extends org.apache.thrift.ProcessFunction {
public getChildNodePathInNextLevel() {
super("getChildNodePathInNextLevel");
}
public getChildNodePathInNextLevel_args getEmptyArgsInstance() {
return new getChildNodePathInNextLevel_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public getChildNodePathInNextLevel_result getResult(I iface, getChildNodePathInNextLevel_args args) throws org.apache.thrift.TException {
getChildNodePathInNextLevel_result result = new getChildNodePathInNextLevel_result();
result.success = iface.getChildNodePathInNextLevel(args.header, args.path);
return result;
}
}
public static class getAllMeasurementSchema extends org.apache.thrift.ProcessFunction {
public getAllMeasurementSchema() {
super("getAllMeasurementSchema");
}
public getAllMeasurementSchema_args getEmptyArgsInstance() {
return new getAllMeasurementSchema_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public getAllMeasurementSchema_result getResult(I iface, getAllMeasurementSchema_args args) throws org.apache.thrift.TException {
getAllMeasurementSchema_result result = new getAllMeasurementSchema_result();
result.success = iface.getAllMeasurementSchema(args.request);
return result;
}
}
public static class getAggrResult extends org.apache.thrift.ProcessFunction {
public getAggrResult() {
super("getAggrResult");
}
public getAggrResult_args getEmptyArgsInstance() {
return new getAggrResult_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public getAggrResult_result getResult(I iface, getAggrResult_args args) throws org.apache.thrift.TException {
getAggrResult_result result = new getAggrResult_result();
result.success = iface.getAggrResult(args.request);
return result;
}
}
public static class getUnregisteredTimeseries extends org.apache.thrift.ProcessFunction {
public getUnregisteredTimeseries() {
super("getUnregisteredTimeseries");
}
public getUnregisteredTimeseries_args getEmptyArgsInstance() {
return new getUnregisteredTimeseries_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public getUnregisteredTimeseries_result getResult(I iface, getUnregisteredTimeseries_args args) throws org.apache.thrift.TException {
getUnregisteredTimeseries_result result = new getUnregisteredTimeseries_result();
result.success = iface.getUnregisteredTimeseries(args.header, args.timeseriesList);
return result;
}
}
public static class pullSnapshot extends org.apache.thrift.ProcessFunction {
public pullSnapshot() {
super("pullSnapshot");
}
public pullSnapshot_args getEmptyArgsInstance() {
return new pullSnapshot_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public pullSnapshot_result getResult(I iface, pullSnapshot_args args) throws org.apache.thrift.TException {
pullSnapshot_result result = new pullSnapshot_result();
result.success = iface.pullSnapshot(args.request);
return result;
}
}
public static class getGroupByExecutor extends org.apache.thrift.ProcessFunction {
public getGroupByExecutor() {
super("getGroupByExecutor");
}
public getGroupByExecutor_args getEmptyArgsInstance() {
return new getGroupByExecutor_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public getGroupByExecutor_result getResult(I iface, getGroupByExecutor_args args) throws org.apache.thrift.TException {
getGroupByExecutor_result result = new getGroupByExecutor_result();
result.success = iface.getGroupByExecutor(args.request);
result.setSuccessIsSet(true);
return result;
}
}
public static class getGroupByResult extends org.apache.thrift.ProcessFunction {
public getGroupByResult() {
super("getGroupByResult");
}
public getGroupByResult_args getEmptyArgsInstance() {
return new getGroupByResult_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public getGroupByResult_result getResult(I iface, getGroupByResult_args args) throws org.apache.thrift.TException {
getGroupByResult_result result = new getGroupByResult_result();
result.success = iface.getGroupByResult(args.header, args.executorId, args.startTime, args.endTime);
return result;
}
}
public static class pullTimeSeriesSchema extends org.apache.thrift.ProcessFunction {
public pullTimeSeriesSchema() {
super("pullTimeSeriesSchema");
}
public pullTimeSeriesSchema_args getEmptyArgsInstance() {
return new pullTimeSeriesSchema_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public pullTimeSeriesSchema_result getResult(I iface, pullTimeSeriesSchema_args args) throws org.apache.thrift.TException {
pullTimeSeriesSchema_result result = new pullTimeSeriesSchema_result();
result.success = iface.pullTimeSeriesSchema(args.request);
return result;
}
}
public static class pullMeasurementSchema extends org.apache.thrift.ProcessFunction {
public pullMeasurementSchema() {
super("pullMeasurementSchema");
}
public pullMeasurementSchema_args getEmptyArgsInstance() {
return new pullMeasurementSchema_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public pullMeasurementSchema_result getResult(I iface, pullMeasurementSchema_args args) throws org.apache.thrift.TException {
pullMeasurementSchema_result result = new pullMeasurementSchema_result();
result.success = iface.pullMeasurementSchema(args.request);
return result;
}
}
public static class previousFill extends org.apache.thrift.ProcessFunction {
public previousFill() {
super("previousFill");
}
public previousFill_args getEmptyArgsInstance() {
return new previousFill_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public previousFill_result getResult(I iface, previousFill_args args) throws org.apache.thrift.TException {
previousFill_result result = new previousFill_result();
result.success = iface.previousFill(args.request);
return result;
}
}
public static class last extends org.apache.thrift.ProcessFunction