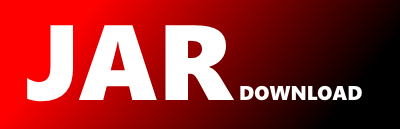
org.apache.iotdb.consensus.multileader.thrift.MultiLeaderConsensusIService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thrift-multi-leader-consensus
Show all versions of thrift-multi-leader-consensus
Rpc modules for multi leader consensus algorithm
The newest version!
/**
* Autogenerated by Thrift Compiler (0.14.1)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package org.apache.iotdb.consensus.multileader.thrift;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked", "unused"})
@javax.annotation.Generated(value = "Autogenerated by Thrift Compiler (0.14.1)", date = "2022-10-14")
public class MultiLeaderConsensusIService {
public interface Iface {
public TSyncLogRes syncLog(TSyncLogReq req) throws org.apache.thrift.TException;
public TInactivatePeerRes inactivatePeer(TInactivatePeerReq req) throws org.apache.thrift.TException;
public TActivatePeerRes activatePeer(TActivatePeerReq req) throws org.apache.thrift.TException;
public TBuildSyncLogChannelRes buildSyncLogChannel(TBuildSyncLogChannelReq req) throws org.apache.thrift.TException;
public TRemoveSyncLogChannelRes removeSyncLogChannel(TRemoveSyncLogChannelReq req) throws org.apache.thrift.TException;
public TSendSnapshotFragmentRes sendSnapshotFragment(TSendSnapshotFragmentReq req) throws org.apache.thrift.TException;
public TTriggerSnapshotLoadRes triggerSnapshotLoad(TTriggerSnapshotLoadReq req) throws org.apache.thrift.TException;
}
public interface AsyncIface {
public void syncLog(TSyncLogReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void inactivatePeer(TInactivatePeerReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void activatePeer(TActivatePeerReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void buildSyncLogChannel(TBuildSyncLogChannelReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void removeSyncLogChannel(TRemoveSyncLogChannelReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void sendSnapshotFragment(TSendSnapshotFragmentReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void triggerSnapshotLoad(TTriggerSnapshotLoadReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
}
public static class Client extends org.apache.thrift.TServiceClient implements Iface {
public static class Factory implements org.apache.thrift.TServiceClientFactory {
public Factory() {}
public Client getClient(org.apache.thrift.protocol.TProtocol prot) {
return new Client(prot);
}
public Client getClient(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TProtocol oprot) {
return new Client(iprot, oprot);
}
}
public Client(org.apache.thrift.protocol.TProtocol prot)
{
super(prot, prot);
}
public Client(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TProtocol oprot) {
super(iprot, oprot);
}
public TSyncLogRes syncLog(TSyncLogReq req) throws org.apache.thrift.TException
{
send_syncLog(req);
return recv_syncLog();
}
public void send_syncLog(TSyncLogReq req) throws org.apache.thrift.TException
{
syncLog_args args = new syncLog_args();
args.setReq(req);
sendBase("syncLog", args);
}
public TSyncLogRes recv_syncLog() throws org.apache.thrift.TException
{
syncLog_result result = new syncLog_result();
receiveBase(result, "syncLog");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "syncLog failed: unknown result");
}
public TInactivatePeerRes inactivatePeer(TInactivatePeerReq req) throws org.apache.thrift.TException
{
send_inactivatePeer(req);
return recv_inactivatePeer();
}
public void send_inactivatePeer(TInactivatePeerReq req) throws org.apache.thrift.TException
{
inactivatePeer_args args = new inactivatePeer_args();
args.setReq(req);
sendBase("inactivatePeer", args);
}
public TInactivatePeerRes recv_inactivatePeer() throws org.apache.thrift.TException
{
inactivatePeer_result result = new inactivatePeer_result();
receiveBase(result, "inactivatePeer");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "inactivatePeer failed: unknown result");
}
public TActivatePeerRes activatePeer(TActivatePeerReq req) throws org.apache.thrift.TException
{
send_activatePeer(req);
return recv_activatePeer();
}
public void send_activatePeer(TActivatePeerReq req) throws org.apache.thrift.TException
{
activatePeer_args args = new activatePeer_args();
args.setReq(req);
sendBase("activatePeer", args);
}
public TActivatePeerRes recv_activatePeer() throws org.apache.thrift.TException
{
activatePeer_result result = new activatePeer_result();
receiveBase(result, "activatePeer");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "activatePeer failed: unknown result");
}
public TBuildSyncLogChannelRes buildSyncLogChannel(TBuildSyncLogChannelReq req) throws org.apache.thrift.TException
{
send_buildSyncLogChannel(req);
return recv_buildSyncLogChannel();
}
public void send_buildSyncLogChannel(TBuildSyncLogChannelReq req) throws org.apache.thrift.TException
{
buildSyncLogChannel_args args = new buildSyncLogChannel_args();
args.setReq(req);
sendBase("buildSyncLogChannel", args);
}
public TBuildSyncLogChannelRes recv_buildSyncLogChannel() throws org.apache.thrift.TException
{
buildSyncLogChannel_result result = new buildSyncLogChannel_result();
receiveBase(result, "buildSyncLogChannel");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "buildSyncLogChannel failed: unknown result");
}
public TRemoveSyncLogChannelRes removeSyncLogChannel(TRemoveSyncLogChannelReq req) throws org.apache.thrift.TException
{
send_removeSyncLogChannel(req);
return recv_removeSyncLogChannel();
}
public void send_removeSyncLogChannel(TRemoveSyncLogChannelReq req) throws org.apache.thrift.TException
{
removeSyncLogChannel_args args = new removeSyncLogChannel_args();
args.setReq(req);
sendBase("removeSyncLogChannel", args);
}
public TRemoveSyncLogChannelRes recv_removeSyncLogChannel() throws org.apache.thrift.TException
{
removeSyncLogChannel_result result = new removeSyncLogChannel_result();
receiveBase(result, "removeSyncLogChannel");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "removeSyncLogChannel failed: unknown result");
}
public TSendSnapshotFragmentRes sendSnapshotFragment(TSendSnapshotFragmentReq req) throws org.apache.thrift.TException
{
send_sendSnapshotFragment(req);
return recv_sendSnapshotFragment();
}
public void send_sendSnapshotFragment(TSendSnapshotFragmentReq req) throws org.apache.thrift.TException
{
sendSnapshotFragment_args args = new sendSnapshotFragment_args();
args.setReq(req);
sendBase("sendSnapshotFragment", args);
}
public TSendSnapshotFragmentRes recv_sendSnapshotFragment() throws org.apache.thrift.TException
{
sendSnapshotFragment_result result = new sendSnapshotFragment_result();
receiveBase(result, "sendSnapshotFragment");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "sendSnapshotFragment failed: unknown result");
}
public TTriggerSnapshotLoadRes triggerSnapshotLoad(TTriggerSnapshotLoadReq req) throws org.apache.thrift.TException
{
send_triggerSnapshotLoad(req);
return recv_triggerSnapshotLoad();
}
public void send_triggerSnapshotLoad(TTriggerSnapshotLoadReq req) throws org.apache.thrift.TException
{
triggerSnapshotLoad_args args = new triggerSnapshotLoad_args();
args.setReq(req);
sendBase("triggerSnapshotLoad", args);
}
public TTriggerSnapshotLoadRes recv_triggerSnapshotLoad() throws org.apache.thrift.TException
{
triggerSnapshotLoad_result result = new triggerSnapshotLoad_result();
receiveBase(result, "triggerSnapshotLoad");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "triggerSnapshotLoad failed: unknown result");
}
}
public static class AsyncClient extends org.apache.thrift.async.TAsyncClient implements AsyncIface {
public static class Factory implements org.apache.thrift.async.TAsyncClientFactory {
private org.apache.thrift.async.TAsyncClientManager clientManager;
private org.apache.thrift.protocol.TProtocolFactory protocolFactory;
public Factory(org.apache.thrift.async.TAsyncClientManager clientManager, org.apache.thrift.protocol.TProtocolFactory protocolFactory) {
this.clientManager = clientManager;
this.protocolFactory = protocolFactory;
}
public AsyncClient getAsyncClient(org.apache.thrift.transport.TNonblockingTransport transport) {
return new AsyncClient(protocolFactory, clientManager, transport);
}
}
public AsyncClient(org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.async.TAsyncClientManager clientManager, org.apache.thrift.transport.TNonblockingTransport transport) {
super(protocolFactory, clientManager, transport);
}
public void syncLog(TSyncLogReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
syncLog_call method_call = new syncLog_call(req, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class syncLog_call extends org.apache.thrift.async.TAsyncMethodCall {
private TSyncLogReq req;
public syncLog_call(TSyncLogReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.req = req;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("syncLog", org.apache.thrift.protocol.TMessageType.CALL, 0));
syncLog_args args = new syncLog_args();
args.setReq(req);
args.write(prot);
prot.writeMessageEnd();
}
public TSyncLogRes getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_syncLog();
}
}
public void inactivatePeer(TInactivatePeerReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
inactivatePeer_call method_call = new inactivatePeer_call(req, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class inactivatePeer_call extends org.apache.thrift.async.TAsyncMethodCall {
private TInactivatePeerReq req;
public inactivatePeer_call(TInactivatePeerReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.req = req;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("inactivatePeer", org.apache.thrift.protocol.TMessageType.CALL, 0));
inactivatePeer_args args = new inactivatePeer_args();
args.setReq(req);
args.write(prot);
prot.writeMessageEnd();
}
public TInactivatePeerRes getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_inactivatePeer();
}
}
public void activatePeer(TActivatePeerReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
activatePeer_call method_call = new activatePeer_call(req, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class activatePeer_call extends org.apache.thrift.async.TAsyncMethodCall {
private TActivatePeerReq req;
public activatePeer_call(TActivatePeerReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.req = req;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("activatePeer", org.apache.thrift.protocol.TMessageType.CALL, 0));
activatePeer_args args = new activatePeer_args();
args.setReq(req);
args.write(prot);
prot.writeMessageEnd();
}
public TActivatePeerRes getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_activatePeer();
}
}
public void buildSyncLogChannel(TBuildSyncLogChannelReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
buildSyncLogChannel_call method_call = new buildSyncLogChannel_call(req, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class buildSyncLogChannel_call extends org.apache.thrift.async.TAsyncMethodCall {
private TBuildSyncLogChannelReq req;
public buildSyncLogChannel_call(TBuildSyncLogChannelReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.req = req;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("buildSyncLogChannel", org.apache.thrift.protocol.TMessageType.CALL, 0));
buildSyncLogChannel_args args = new buildSyncLogChannel_args();
args.setReq(req);
args.write(prot);
prot.writeMessageEnd();
}
public TBuildSyncLogChannelRes getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_buildSyncLogChannel();
}
}
public void removeSyncLogChannel(TRemoveSyncLogChannelReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
removeSyncLogChannel_call method_call = new removeSyncLogChannel_call(req, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class removeSyncLogChannel_call extends org.apache.thrift.async.TAsyncMethodCall {
private TRemoveSyncLogChannelReq req;
public removeSyncLogChannel_call(TRemoveSyncLogChannelReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.req = req;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("removeSyncLogChannel", org.apache.thrift.protocol.TMessageType.CALL, 0));
removeSyncLogChannel_args args = new removeSyncLogChannel_args();
args.setReq(req);
args.write(prot);
prot.writeMessageEnd();
}
public TRemoveSyncLogChannelRes getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_removeSyncLogChannel();
}
}
public void sendSnapshotFragment(TSendSnapshotFragmentReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
sendSnapshotFragment_call method_call = new sendSnapshotFragment_call(req, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class sendSnapshotFragment_call extends org.apache.thrift.async.TAsyncMethodCall {
private TSendSnapshotFragmentReq req;
public sendSnapshotFragment_call(TSendSnapshotFragmentReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.req = req;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("sendSnapshotFragment", org.apache.thrift.protocol.TMessageType.CALL, 0));
sendSnapshotFragment_args args = new sendSnapshotFragment_args();
args.setReq(req);
args.write(prot);
prot.writeMessageEnd();
}
public TSendSnapshotFragmentRes getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_sendSnapshotFragment();
}
}
public void triggerSnapshotLoad(TTriggerSnapshotLoadReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
triggerSnapshotLoad_call method_call = new triggerSnapshotLoad_call(req, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class triggerSnapshotLoad_call extends org.apache.thrift.async.TAsyncMethodCall {
private TTriggerSnapshotLoadReq req;
public triggerSnapshotLoad_call(TTriggerSnapshotLoadReq req, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.req = req;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("triggerSnapshotLoad", org.apache.thrift.protocol.TMessageType.CALL, 0));
triggerSnapshotLoad_args args = new triggerSnapshotLoad_args();
args.setReq(req);
args.write(prot);
prot.writeMessageEnd();
}
public TTriggerSnapshotLoadRes getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_triggerSnapshotLoad();
}
}
}
public static class Processor extends org.apache.thrift.TBaseProcessor implements org.apache.thrift.TProcessor {
private static final org.slf4j.Logger _LOGGER = org.slf4j.LoggerFactory.getLogger(Processor.class.getName());
public Processor(I iface) {
super(iface, getProcessMap(new java.util.HashMap>()));
}
protected Processor(I iface, java.util.Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static java.util.Map> getProcessMap(java.util.Map> processMap) {
processMap.put("syncLog", new syncLog());
processMap.put("inactivatePeer", new inactivatePeer());
processMap.put("activatePeer", new activatePeer());
processMap.put("buildSyncLogChannel", new buildSyncLogChannel());
processMap.put("removeSyncLogChannel", new removeSyncLogChannel());
processMap.put("sendSnapshotFragment", new sendSnapshotFragment());
processMap.put("triggerSnapshotLoad", new triggerSnapshotLoad());
return processMap;
}
public static class syncLog extends org.apache.thrift.ProcessFunction {
public syncLog() {
super("syncLog");
}
public syncLog_args getEmptyArgsInstance() {
return new syncLog_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public syncLog_result getResult(I iface, syncLog_args args) throws org.apache.thrift.TException {
syncLog_result result = new syncLog_result();
result.success = iface.syncLog(args.req);
return result;
}
}
public static class inactivatePeer extends org.apache.thrift.ProcessFunction {
public inactivatePeer() {
super("inactivatePeer");
}
public inactivatePeer_args getEmptyArgsInstance() {
return new inactivatePeer_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public inactivatePeer_result getResult(I iface, inactivatePeer_args args) throws org.apache.thrift.TException {
inactivatePeer_result result = new inactivatePeer_result();
result.success = iface.inactivatePeer(args.req);
return result;
}
}
public static class activatePeer extends org.apache.thrift.ProcessFunction {
public activatePeer() {
super("activatePeer");
}
public activatePeer_args getEmptyArgsInstance() {
return new activatePeer_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public activatePeer_result getResult(I iface, activatePeer_args args) throws org.apache.thrift.TException {
activatePeer_result result = new activatePeer_result();
result.success = iface.activatePeer(args.req);
return result;
}
}
public static class buildSyncLogChannel extends org.apache.thrift.ProcessFunction {
public buildSyncLogChannel() {
super("buildSyncLogChannel");
}
public buildSyncLogChannel_args getEmptyArgsInstance() {
return new buildSyncLogChannel_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public buildSyncLogChannel_result getResult(I iface, buildSyncLogChannel_args args) throws org.apache.thrift.TException {
buildSyncLogChannel_result result = new buildSyncLogChannel_result();
result.success = iface.buildSyncLogChannel(args.req);
return result;
}
}
public static class removeSyncLogChannel extends org.apache.thrift.ProcessFunction {
public removeSyncLogChannel() {
super("removeSyncLogChannel");
}
public removeSyncLogChannel_args getEmptyArgsInstance() {
return new removeSyncLogChannel_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public removeSyncLogChannel_result getResult(I iface, removeSyncLogChannel_args args) throws org.apache.thrift.TException {
removeSyncLogChannel_result result = new removeSyncLogChannel_result();
result.success = iface.removeSyncLogChannel(args.req);
return result;
}
}
public static class sendSnapshotFragment extends org.apache.thrift.ProcessFunction {
public sendSnapshotFragment() {
super("sendSnapshotFragment");
}
public sendSnapshotFragment_args getEmptyArgsInstance() {
return new sendSnapshotFragment_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public sendSnapshotFragment_result getResult(I iface, sendSnapshotFragment_args args) throws org.apache.thrift.TException {
sendSnapshotFragment_result result = new sendSnapshotFragment_result();
result.success = iface.sendSnapshotFragment(args.req);
return result;
}
}
public static class triggerSnapshotLoad extends org.apache.thrift.ProcessFunction {
public triggerSnapshotLoad() {
super("triggerSnapshotLoad");
}
public triggerSnapshotLoad_args getEmptyArgsInstance() {
return new triggerSnapshotLoad_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public triggerSnapshotLoad_result getResult(I iface, triggerSnapshotLoad_args args) throws org.apache.thrift.TException {
triggerSnapshotLoad_result result = new triggerSnapshotLoad_result();
result.success = iface.triggerSnapshotLoad(args.req);
return result;
}
}
}
public static class AsyncProcessor extends org.apache.thrift.TBaseAsyncProcessor {
private static final org.slf4j.Logger _LOGGER = org.slf4j.LoggerFactory.getLogger(AsyncProcessor.class.getName());
public AsyncProcessor(I iface) {
super(iface, getProcessMap(new java.util.HashMap>()));
}
protected AsyncProcessor(I iface, java.util.Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static java.util.Map> getProcessMap(java.util.Map> processMap) {
processMap.put("syncLog", new syncLog());
processMap.put("inactivatePeer", new inactivatePeer());
processMap.put("activatePeer", new activatePeer());
processMap.put("buildSyncLogChannel", new buildSyncLogChannel());
processMap.put("removeSyncLogChannel", new removeSyncLogChannel());
processMap.put("sendSnapshotFragment", new sendSnapshotFragment());
processMap.put("triggerSnapshotLoad", new triggerSnapshotLoad());
return processMap;
}
public static class syncLog extends org.apache.thrift.AsyncProcessFunction {
public syncLog() {
super("syncLog");
}
public syncLog_args getEmptyArgsInstance() {
return new syncLog_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(TSyncLogRes o) {
syncLog_result result = new syncLog_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
syncLog_result result = new syncLog_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, syncLog_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.syncLog(args.req,resultHandler);
}
}
public static class inactivatePeer extends org.apache.thrift.AsyncProcessFunction {
public inactivatePeer() {
super("inactivatePeer");
}
public inactivatePeer_args getEmptyArgsInstance() {
return new inactivatePeer_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(TInactivatePeerRes o) {
inactivatePeer_result result = new inactivatePeer_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
inactivatePeer_result result = new inactivatePeer_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, inactivatePeer_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.inactivatePeer(args.req,resultHandler);
}
}
public static class activatePeer extends org.apache.thrift.AsyncProcessFunction {
public activatePeer() {
super("activatePeer");
}
public activatePeer_args getEmptyArgsInstance() {
return new activatePeer_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(TActivatePeerRes o) {
activatePeer_result result = new activatePeer_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
activatePeer_result result = new activatePeer_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, activatePeer_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.activatePeer(args.req,resultHandler);
}
}
public static class buildSyncLogChannel extends org.apache.thrift.AsyncProcessFunction {
public buildSyncLogChannel() {
super("buildSyncLogChannel");
}
public buildSyncLogChannel_args getEmptyArgsInstance() {
return new buildSyncLogChannel_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(TBuildSyncLogChannelRes o) {
buildSyncLogChannel_result result = new buildSyncLogChannel_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
buildSyncLogChannel_result result = new buildSyncLogChannel_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, buildSyncLogChannel_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.buildSyncLogChannel(args.req,resultHandler);
}
}
public static class removeSyncLogChannel extends org.apache.thrift.AsyncProcessFunction {
public removeSyncLogChannel() {
super("removeSyncLogChannel");
}
public removeSyncLogChannel_args getEmptyArgsInstance() {
return new removeSyncLogChannel_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(TRemoveSyncLogChannelRes o) {
removeSyncLogChannel_result result = new removeSyncLogChannel_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
removeSyncLogChannel_result result = new removeSyncLogChannel_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, removeSyncLogChannel_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.removeSyncLogChannel(args.req,resultHandler);
}
}
public static class sendSnapshotFragment extends org.apache.thrift.AsyncProcessFunction {
public sendSnapshotFragment() {
super("sendSnapshotFragment");
}
public sendSnapshotFragment_args getEmptyArgsInstance() {
return new sendSnapshotFragment_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(TSendSnapshotFragmentRes o) {
sendSnapshotFragment_result result = new sendSnapshotFragment_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
sendSnapshotFragment_result result = new sendSnapshotFragment_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, sendSnapshotFragment_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.sendSnapshotFragment(args.req,resultHandler);
}
}
public static class triggerSnapshotLoad extends org.apache.thrift.AsyncProcessFunction {
public triggerSnapshotLoad() {
super("triggerSnapshotLoad");
}
public triggerSnapshotLoad_args getEmptyArgsInstance() {
return new triggerSnapshotLoad_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(TTriggerSnapshotLoadRes o) {
triggerSnapshotLoad_result result = new triggerSnapshotLoad_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
triggerSnapshotLoad_result result = new triggerSnapshotLoad_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, triggerSnapshotLoad_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.triggerSnapshotLoad(args.req,resultHandler);
}
}
}
public static class syncLog_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("syncLog_args");
private static final org.apache.thrift.protocol.TField REQ_FIELD_DESC = new org.apache.thrift.protocol.TField("req", org.apache.thrift.protocol.TType.STRUCT, (short)-1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new syncLog_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new syncLog_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TSyncLogReq req; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQ((short)-1, "req");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case -1: // REQ
return REQ;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQ, new org.apache.thrift.meta_data.FieldMetaData("req", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TSyncLogReq.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(syncLog_args.class, metaDataMap);
}
public syncLog_args() {
}
public syncLog_args(
TSyncLogReq req)
{
this();
this.req = req;
}
/**
* Performs a deep copy on other.
*/
public syncLog_args(syncLog_args other) {
if (other.isSetReq()) {
this.req = new TSyncLogReq(other.req);
}
}
public syncLog_args deepCopy() {
return new syncLog_args(this);
}
@Override
public void clear() {
this.req = null;
}
@org.apache.thrift.annotation.Nullable
public TSyncLogReq getReq() {
return this.req;
}
public syncLog_args setReq(@org.apache.thrift.annotation.Nullable TSyncLogReq req) {
this.req = req;
return this;
}
public void unsetReq() {
this.req = null;
}
/** Returns true if field req is set (has been assigned a value) and false otherwise */
public boolean isSetReq() {
return this.req != null;
}
public void setReqIsSet(boolean value) {
if (!value) {
this.req = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQ:
if (value == null) {
unsetReq();
} else {
setReq((TSyncLogReq)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQ:
return getReq();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQ:
return isSetReq();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof syncLog_args)
return this.equals((syncLog_args)that);
return false;
}
public boolean equals(syncLog_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_req = true && this.isSetReq();
boolean that_present_req = true && that.isSetReq();
if (this_present_req || that_present_req) {
if (!(this_present_req && that_present_req))
return false;
if (!this.req.equals(that.req))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetReq()) ? 131071 : 524287);
if (isSetReq())
hashCode = hashCode * 8191 + req.hashCode();
return hashCode;
}
@Override
public int compareTo(syncLog_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetReq(), other.isSetReq());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetReq()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.req, other.req);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("syncLog_args(");
boolean first = true;
sb.append("req:");
if (this.req == null) {
sb.append("null");
} else {
sb.append(this.req);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (req != null) {
req.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class syncLog_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public syncLog_argsStandardScheme getScheme() {
return new syncLog_argsStandardScheme();
}
}
private static class syncLog_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, syncLog_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case -1: // REQ
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.req = new TSyncLogReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, syncLog_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.req != null) {
oprot.writeFieldBegin(REQ_FIELD_DESC);
struct.req.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class syncLog_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public syncLog_argsTupleScheme getScheme() {
return new syncLog_argsTupleScheme();
}
}
private static class syncLog_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, syncLog_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetReq()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetReq()) {
struct.req.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, syncLog_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.req = new TSyncLogReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class syncLog_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("syncLog_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new syncLog_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new syncLog_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TSyncLogRes success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TSyncLogRes.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(syncLog_result.class, metaDataMap);
}
public syncLog_result() {
}
public syncLog_result(
TSyncLogRes success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public syncLog_result(syncLog_result other) {
if (other.isSetSuccess()) {
this.success = new TSyncLogRes(other.success);
}
}
public syncLog_result deepCopy() {
return new syncLog_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public TSyncLogRes getSuccess() {
return this.success;
}
public syncLog_result setSuccess(@org.apache.thrift.annotation.Nullable TSyncLogRes success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((TSyncLogRes)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof syncLog_result)
return this.equals((syncLog_result)that);
return false;
}
public boolean equals(syncLog_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(syncLog_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("syncLog_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class syncLog_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public syncLog_resultStandardScheme getScheme() {
return new syncLog_resultStandardScheme();
}
}
private static class syncLog_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, syncLog_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new TSyncLogRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, syncLog_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class syncLog_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public syncLog_resultTupleScheme getScheme() {
return new syncLog_resultTupleScheme();
}
}
private static class syncLog_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, syncLog_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, syncLog_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new TSyncLogRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class inactivatePeer_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("inactivatePeer_args");
private static final org.apache.thrift.protocol.TField REQ_FIELD_DESC = new org.apache.thrift.protocol.TField("req", org.apache.thrift.protocol.TType.STRUCT, (short)-1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new inactivatePeer_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new inactivatePeer_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TInactivatePeerReq req; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQ((short)-1, "req");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case -1: // REQ
return REQ;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQ, new org.apache.thrift.meta_data.FieldMetaData("req", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TInactivatePeerReq.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(inactivatePeer_args.class, metaDataMap);
}
public inactivatePeer_args() {
}
public inactivatePeer_args(
TInactivatePeerReq req)
{
this();
this.req = req;
}
/**
* Performs a deep copy on other.
*/
public inactivatePeer_args(inactivatePeer_args other) {
if (other.isSetReq()) {
this.req = new TInactivatePeerReq(other.req);
}
}
public inactivatePeer_args deepCopy() {
return new inactivatePeer_args(this);
}
@Override
public void clear() {
this.req = null;
}
@org.apache.thrift.annotation.Nullable
public TInactivatePeerReq getReq() {
return this.req;
}
public inactivatePeer_args setReq(@org.apache.thrift.annotation.Nullable TInactivatePeerReq req) {
this.req = req;
return this;
}
public void unsetReq() {
this.req = null;
}
/** Returns true if field req is set (has been assigned a value) and false otherwise */
public boolean isSetReq() {
return this.req != null;
}
public void setReqIsSet(boolean value) {
if (!value) {
this.req = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQ:
if (value == null) {
unsetReq();
} else {
setReq((TInactivatePeerReq)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQ:
return getReq();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQ:
return isSetReq();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof inactivatePeer_args)
return this.equals((inactivatePeer_args)that);
return false;
}
public boolean equals(inactivatePeer_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_req = true && this.isSetReq();
boolean that_present_req = true && that.isSetReq();
if (this_present_req || that_present_req) {
if (!(this_present_req && that_present_req))
return false;
if (!this.req.equals(that.req))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetReq()) ? 131071 : 524287);
if (isSetReq())
hashCode = hashCode * 8191 + req.hashCode();
return hashCode;
}
@Override
public int compareTo(inactivatePeer_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetReq(), other.isSetReq());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetReq()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.req, other.req);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("inactivatePeer_args(");
boolean first = true;
sb.append("req:");
if (this.req == null) {
sb.append("null");
} else {
sb.append(this.req);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (req != null) {
req.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class inactivatePeer_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public inactivatePeer_argsStandardScheme getScheme() {
return new inactivatePeer_argsStandardScheme();
}
}
private static class inactivatePeer_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, inactivatePeer_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case -1: // REQ
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.req = new TInactivatePeerReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, inactivatePeer_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.req != null) {
oprot.writeFieldBegin(REQ_FIELD_DESC);
struct.req.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class inactivatePeer_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public inactivatePeer_argsTupleScheme getScheme() {
return new inactivatePeer_argsTupleScheme();
}
}
private static class inactivatePeer_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, inactivatePeer_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetReq()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetReq()) {
struct.req.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, inactivatePeer_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.req = new TInactivatePeerReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class inactivatePeer_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("inactivatePeer_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new inactivatePeer_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new inactivatePeer_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TInactivatePeerRes success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TInactivatePeerRes.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(inactivatePeer_result.class, metaDataMap);
}
public inactivatePeer_result() {
}
public inactivatePeer_result(
TInactivatePeerRes success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public inactivatePeer_result(inactivatePeer_result other) {
if (other.isSetSuccess()) {
this.success = new TInactivatePeerRes(other.success);
}
}
public inactivatePeer_result deepCopy() {
return new inactivatePeer_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public TInactivatePeerRes getSuccess() {
return this.success;
}
public inactivatePeer_result setSuccess(@org.apache.thrift.annotation.Nullable TInactivatePeerRes success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((TInactivatePeerRes)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof inactivatePeer_result)
return this.equals((inactivatePeer_result)that);
return false;
}
public boolean equals(inactivatePeer_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(inactivatePeer_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("inactivatePeer_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class inactivatePeer_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public inactivatePeer_resultStandardScheme getScheme() {
return new inactivatePeer_resultStandardScheme();
}
}
private static class inactivatePeer_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, inactivatePeer_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new TInactivatePeerRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, inactivatePeer_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class inactivatePeer_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public inactivatePeer_resultTupleScheme getScheme() {
return new inactivatePeer_resultTupleScheme();
}
}
private static class inactivatePeer_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, inactivatePeer_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, inactivatePeer_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new TInactivatePeerRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class activatePeer_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("activatePeer_args");
private static final org.apache.thrift.protocol.TField REQ_FIELD_DESC = new org.apache.thrift.protocol.TField("req", org.apache.thrift.protocol.TType.STRUCT, (short)-1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new activatePeer_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new activatePeer_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TActivatePeerReq req; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQ((short)-1, "req");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case -1: // REQ
return REQ;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQ, new org.apache.thrift.meta_data.FieldMetaData("req", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TActivatePeerReq.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(activatePeer_args.class, metaDataMap);
}
public activatePeer_args() {
}
public activatePeer_args(
TActivatePeerReq req)
{
this();
this.req = req;
}
/**
* Performs a deep copy on other.
*/
public activatePeer_args(activatePeer_args other) {
if (other.isSetReq()) {
this.req = new TActivatePeerReq(other.req);
}
}
public activatePeer_args deepCopy() {
return new activatePeer_args(this);
}
@Override
public void clear() {
this.req = null;
}
@org.apache.thrift.annotation.Nullable
public TActivatePeerReq getReq() {
return this.req;
}
public activatePeer_args setReq(@org.apache.thrift.annotation.Nullable TActivatePeerReq req) {
this.req = req;
return this;
}
public void unsetReq() {
this.req = null;
}
/** Returns true if field req is set (has been assigned a value) and false otherwise */
public boolean isSetReq() {
return this.req != null;
}
public void setReqIsSet(boolean value) {
if (!value) {
this.req = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQ:
if (value == null) {
unsetReq();
} else {
setReq((TActivatePeerReq)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQ:
return getReq();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQ:
return isSetReq();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof activatePeer_args)
return this.equals((activatePeer_args)that);
return false;
}
public boolean equals(activatePeer_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_req = true && this.isSetReq();
boolean that_present_req = true && that.isSetReq();
if (this_present_req || that_present_req) {
if (!(this_present_req && that_present_req))
return false;
if (!this.req.equals(that.req))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetReq()) ? 131071 : 524287);
if (isSetReq())
hashCode = hashCode * 8191 + req.hashCode();
return hashCode;
}
@Override
public int compareTo(activatePeer_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetReq(), other.isSetReq());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetReq()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.req, other.req);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("activatePeer_args(");
boolean first = true;
sb.append("req:");
if (this.req == null) {
sb.append("null");
} else {
sb.append(this.req);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (req != null) {
req.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class activatePeer_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public activatePeer_argsStandardScheme getScheme() {
return new activatePeer_argsStandardScheme();
}
}
private static class activatePeer_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, activatePeer_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case -1: // REQ
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.req = new TActivatePeerReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, activatePeer_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.req != null) {
oprot.writeFieldBegin(REQ_FIELD_DESC);
struct.req.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class activatePeer_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public activatePeer_argsTupleScheme getScheme() {
return new activatePeer_argsTupleScheme();
}
}
private static class activatePeer_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, activatePeer_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetReq()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetReq()) {
struct.req.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, activatePeer_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.req = new TActivatePeerReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class activatePeer_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("activatePeer_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new activatePeer_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new activatePeer_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TActivatePeerRes success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TActivatePeerRes.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(activatePeer_result.class, metaDataMap);
}
public activatePeer_result() {
}
public activatePeer_result(
TActivatePeerRes success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public activatePeer_result(activatePeer_result other) {
if (other.isSetSuccess()) {
this.success = new TActivatePeerRes(other.success);
}
}
public activatePeer_result deepCopy() {
return new activatePeer_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public TActivatePeerRes getSuccess() {
return this.success;
}
public activatePeer_result setSuccess(@org.apache.thrift.annotation.Nullable TActivatePeerRes success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((TActivatePeerRes)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof activatePeer_result)
return this.equals((activatePeer_result)that);
return false;
}
public boolean equals(activatePeer_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(activatePeer_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("activatePeer_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class activatePeer_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public activatePeer_resultStandardScheme getScheme() {
return new activatePeer_resultStandardScheme();
}
}
private static class activatePeer_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, activatePeer_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new TActivatePeerRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, activatePeer_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class activatePeer_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public activatePeer_resultTupleScheme getScheme() {
return new activatePeer_resultTupleScheme();
}
}
private static class activatePeer_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, activatePeer_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, activatePeer_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new TActivatePeerRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class buildSyncLogChannel_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("buildSyncLogChannel_args");
private static final org.apache.thrift.protocol.TField REQ_FIELD_DESC = new org.apache.thrift.protocol.TField("req", org.apache.thrift.protocol.TType.STRUCT, (short)-1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new buildSyncLogChannel_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new buildSyncLogChannel_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TBuildSyncLogChannelReq req; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQ((short)-1, "req");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case -1: // REQ
return REQ;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQ, new org.apache.thrift.meta_data.FieldMetaData("req", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TBuildSyncLogChannelReq.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(buildSyncLogChannel_args.class, metaDataMap);
}
public buildSyncLogChannel_args() {
}
public buildSyncLogChannel_args(
TBuildSyncLogChannelReq req)
{
this();
this.req = req;
}
/**
* Performs a deep copy on other.
*/
public buildSyncLogChannel_args(buildSyncLogChannel_args other) {
if (other.isSetReq()) {
this.req = new TBuildSyncLogChannelReq(other.req);
}
}
public buildSyncLogChannel_args deepCopy() {
return new buildSyncLogChannel_args(this);
}
@Override
public void clear() {
this.req = null;
}
@org.apache.thrift.annotation.Nullable
public TBuildSyncLogChannelReq getReq() {
return this.req;
}
public buildSyncLogChannel_args setReq(@org.apache.thrift.annotation.Nullable TBuildSyncLogChannelReq req) {
this.req = req;
return this;
}
public void unsetReq() {
this.req = null;
}
/** Returns true if field req is set (has been assigned a value) and false otherwise */
public boolean isSetReq() {
return this.req != null;
}
public void setReqIsSet(boolean value) {
if (!value) {
this.req = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQ:
if (value == null) {
unsetReq();
} else {
setReq((TBuildSyncLogChannelReq)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQ:
return getReq();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQ:
return isSetReq();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof buildSyncLogChannel_args)
return this.equals((buildSyncLogChannel_args)that);
return false;
}
public boolean equals(buildSyncLogChannel_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_req = true && this.isSetReq();
boolean that_present_req = true && that.isSetReq();
if (this_present_req || that_present_req) {
if (!(this_present_req && that_present_req))
return false;
if (!this.req.equals(that.req))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetReq()) ? 131071 : 524287);
if (isSetReq())
hashCode = hashCode * 8191 + req.hashCode();
return hashCode;
}
@Override
public int compareTo(buildSyncLogChannel_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetReq(), other.isSetReq());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetReq()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.req, other.req);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("buildSyncLogChannel_args(");
boolean first = true;
sb.append("req:");
if (this.req == null) {
sb.append("null");
} else {
sb.append(this.req);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (req != null) {
req.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class buildSyncLogChannel_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public buildSyncLogChannel_argsStandardScheme getScheme() {
return new buildSyncLogChannel_argsStandardScheme();
}
}
private static class buildSyncLogChannel_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, buildSyncLogChannel_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case -1: // REQ
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.req = new TBuildSyncLogChannelReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, buildSyncLogChannel_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.req != null) {
oprot.writeFieldBegin(REQ_FIELD_DESC);
struct.req.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class buildSyncLogChannel_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public buildSyncLogChannel_argsTupleScheme getScheme() {
return new buildSyncLogChannel_argsTupleScheme();
}
}
private static class buildSyncLogChannel_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, buildSyncLogChannel_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetReq()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetReq()) {
struct.req.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, buildSyncLogChannel_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.req = new TBuildSyncLogChannelReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class buildSyncLogChannel_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("buildSyncLogChannel_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new buildSyncLogChannel_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new buildSyncLogChannel_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TBuildSyncLogChannelRes success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TBuildSyncLogChannelRes.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(buildSyncLogChannel_result.class, metaDataMap);
}
public buildSyncLogChannel_result() {
}
public buildSyncLogChannel_result(
TBuildSyncLogChannelRes success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public buildSyncLogChannel_result(buildSyncLogChannel_result other) {
if (other.isSetSuccess()) {
this.success = new TBuildSyncLogChannelRes(other.success);
}
}
public buildSyncLogChannel_result deepCopy() {
return new buildSyncLogChannel_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public TBuildSyncLogChannelRes getSuccess() {
return this.success;
}
public buildSyncLogChannel_result setSuccess(@org.apache.thrift.annotation.Nullable TBuildSyncLogChannelRes success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((TBuildSyncLogChannelRes)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof buildSyncLogChannel_result)
return this.equals((buildSyncLogChannel_result)that);
return false;
}
public boolean equals(buildSyncLogChannel_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(buildSyncLogChannel_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("buildSyncLogChannel_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class buildSyncLogChannel_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public buildSyncLogChannel_resultStandardScheme getScheme() {
return new buildSyncLogChannel_resultStandardScheme();
}
}
private static class buildSyncLogChannel_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, buildSyncLogChannel_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new TBuildSyncLogChannelRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, buildSyncLogChannel_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class buildSyncLogChannel_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public buildSyncLogChannel_resultTupleScheme getScheme() {
return new buildSyncLogChannel_resultTupleScheme();
}
}
private static class buildSyncLogChannel_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, buildSyncLogChannel_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, buildSyncLogChannel_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new TBuildSyncLogChannelRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class removeSyncLogChannel_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("removeSyncLogChannel_args");
private static final org.apache.thrift.protocol.TField REQ_FIELD_DESC = new org.apache.thrift.protocol.TField("req", org.apache.thrift.protocol.TType.STRUCT, (short)-1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new removeSyncLogChannel_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new removeSyncLogChannel_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TRemoveSyncLogChannelReq req; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQ((short)-1, "req");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case -1: // REQ
return REQ;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQ, new org.apache.thrift.meta_data.FieldMetaData("req", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TRemoveSyncLogChannelReq.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(removeSyncLogChannel_args.class, metaDataMap);
}
public removeSyncLogChannel_args() {
}
public removeSyncLogChannel_args(
TRemoveSyncLogChannelReq req)
{
this();
this.req = req;
}
/**
* Performs a deep copy on other.
*/
public removeSyncLogChannel_args(removeSyncLogChannel_args other) {
if (other.isSetReq()) {
this.req = new TRemoveSyncLogChannelReq(other.req);
}
}
public removeSyncLogChannel_args deepCopy() {
return new removeSyncLogChannel_args(this);
}
@Override
public void clear() {
this.req = null;
}
@org.apache.thrift.annotation.Nullable
public TRemoveSyncLogChannelReq getReq() {
return this.req;
}
public removeSyncLogChannel_args setReq(@org.apache.thrift.annotation.Nullable TRemoveSyncLogChannelReq req) {
this.req = req;
return this;
}
public void unsetReq() {
this.req = null;
}
/** Returns true if field req is set (has been assigned a value) and false otherwise */
public boolean isSetReq() {
return this.req != null;
}
public void setReqIsSet(boolean value) {
if (!value) {
this.req = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQ:
if (value == null) {
unsetReq();
} else {
setReq((TRemoveSyncLogChannelReq)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQ:
return getReq();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQ:
return isSetReq();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof removeSyncLogChannel_args)
return this.equals((removeSyncLogChannel_args)that);
return false;
}
public boolean equals(removeSyncLogChannel_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_req = true && this.isSetReq();
boolean that_present_req = true && that.isSetReq();
if (this_present_req || that_present_req) {
if (!(this_present_req && that_present_req))
return false;
if (!this.req.equals(that.req))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetReq()) ? 131071 : 524287);
if (isSetReq())
hashCode = hashCode * 8191 + req.hashCode();
return hashCode;
}
@Override
public int compareTo(removeSyncLogChannel_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetReq(), other.isSetReq());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetReq()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.req, other.req);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("removeSyncLogChannel_args(");
boolean first = true;
sb.append("req:");
if (this.req == null) {
sb.append("null");
} else {
sb.append(this.req);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (req != null) {
req.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class removeSyncLogChannel_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public removeSyncLogChannel_argsStandardScheme getScheme() {
return new removeSyncLogChannel_argsStandardScheme();
}
}
private static class removeSyncLogChannel_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, removeSyncLogChannel_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case -1: // REQ
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.req = new TRemoveSyncLogChannelReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, removeSyncLogChannel_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.req != null) {
oprot.writeFieldBegin(REQ_FIELD_DESC);
struct.req.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class removeSyncLogChannel_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public removeSyncLogChannel_argsTupleScheme getScheme() {
return new removeSyncLogChannel_argsTupleScheme();
}
}
private static class removeSyncLogChannel_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, removeSyncLogChannel_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetReq()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetReq()) {
struct.req.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, removeSyncLogChannel_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.req = new TRemoveSyncLogChannelReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class removeSyncLogChannel_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("removeSyncLogChannel_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new removeSyncLogChannel_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new removeSyncLogChannel_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TRemoveSyncLogChannelRes success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TRemoveSyncLogChannelRes.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(removeSyncLogChannel_result.class, metaDataMap);
}
public removeSyncLogChannel_result() {
}
public removeSyncLogChannel_result(
TRemoveSyncLogChannelRes success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public removeSyncLogChannel_result(removeSyncLogChannel_result other) {
if (other.isSetSuccess()) {
this.success = new TRemoveSyncLogChannelRes(other.success);
}
}
public removeSyncLogChannel_result deepCopy() {
return new removeSyncLogChannel_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public TRemoveSyncLogChannelRes getSuccess() {
return this.success;
}
public removeSyncLogChannel_result setSuccess(@org.apache.thrift.annotation.Nullable TRemoveSyncLogChannelRes success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((TRemoveSyncLogChannelRes)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof removeSyncLogChannel_result)
return this.equals((removeSyncLogChannel_result)that);
return false;
}
public boolean equals(removeSyncLogChannel_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(removeSyncLogChannel_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("removeSyncLogChannel_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class removeSyncLogChannel_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public removeSyncLogChannel_resultStandardScheme getScheme() {
return new removeSyncLogChannel_resultStandardScheme();
}
}
private static class removeSyncLogChannel_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, removeSyncLogChannel_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new TRemoveSyncLogChannelRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, removeSyncLogChannel_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class removeSyncLogChannel_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public removeSyncLogChannel_resultTupleScheme getScheme() {
return new removeSyncLogChannel_resultTupleScheme();
}
}
private static class removeSyncLogChannel_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, removeSyncLogChannel_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, removeSyncLogChannel_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new TRemoveSyncLogChannelRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class sendSnapshotFragment_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("sendSnapshotFragment_args");
private static final org.apache.thrift.protocol.TField REQ_FIELD_DESC = new org.apache.thrift.protocol.TField("req", org.apache.thrift.protocol.TType.STRUCT, (short)-1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new sendSnapshotFragment_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new sendSnapshotFragment_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TSendSnapshotFragmentReq req; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQ((short)-1, "req");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case -1: // REQ
return REQ;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQ, new org.apache.thrift.meta_data.FieldMetaData("req", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TSendSnapshotFragmentReq.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(sendSnapshotFragment_args.class, metaDataMap);
}
public sendSnapshotFragment_args() {
}
public sendSnapshotFragment_args(
TSendSnapshotFragmentReq req)
{
this();
this.req = req;
}
/**
* Performs a deep copy on other.
*/
public sendSnapshotFragment_args(sendSnapshotFragment_args other) {
if (other.isSetReq()) {
this.req = new TSendSnapshotFragmentReq(other.req);
}
}
public sendSnapshotFragment_args deepCopy() {
return new sendSnapshotFragment_args(this);
}
@Override
public void clear() {
this.req = null;
}
@org.apache.thrift.annotation.Nullable
public TSendSnapshotFragmentReq getReq() {
return this.req;
}
public sendSnapshotFragment_args setReq(@org.apache.thrift.annotation.Nullable TSendSnapshotFragmentReq req) {
this.req = req;
return this;
}
public void unsetReq() {
this.req = null;
}
/** Returns true if field req is set (has been assigned a value) and false otherwise */
public boolean isSetReq() {
return this.req != null;
}
public void setReqIsSet(boolean value) {
if (!value) {
this.req = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQ:
if (value == null) {
unsetReq();
} else {
setReq((TSendSnapshotFragmentReq)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQ:
return getReq();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQ:
return isSetReq();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof sendSnapshotFragment_args)
return this.equals((sendSnapshotFragment_args)that);
return false;
}
public boolean equals(sendSnapshotFragment_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_req = true && this.isSetReq();
boolean that_present_req = true && that.isSetReq();
if (this_present_req || that_present_req) {
if (!(this_present_req && that_present_req))
return false;
if (!this.req.equals(that.req))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetReq()) ? 131071 : 524287);
if (isSetReq())
hashCode = hashCode * 8191 + req.hashCode();
return hashCode;
}
@Override
public int compareTo(sendSnapshotFragment_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetReq(), other.isSetReq());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetReq()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.req, other.req);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("sendSnapshotFragment_args(");
boolean first = true;
sb.append("req:");
if (this.req == null) {
sb.append("null");
} else {
sb.append(this.req);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (req != null) {
req.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class sendSnapshotFragment_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public sendSnapshotFragment_argsStandardScheme getScheme() {
return new sendSnapshotFragment_argsStandardScheme();
}
}
private static class sendSnapshotFragment_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, sendSnapshotFragment_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case -1: // REQ
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.req = new TSendSnapshotFragmentReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, sendSnapshotFragment_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.req != null) {
oprot.writeFieldBegin(REQ_FIELD_DESC);
struct.req.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class sendSnapshotFragment_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public sendSnapshotFragment_argsTupleScheme getScheme() {
return new sendSnapshotFragment_argsTupleScheme();
}
}
private static class sendSnapshotFragment_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, sendSnapshotFragment_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetReq()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetReq()) {
struct.req.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, sendSnapshotFragment_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.req = new TSendSnapshotFragmentReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class sendSnapshotFragment_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("sendSnapshotFragment_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new sendSnapshotFragment_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new sendSnapshotFragment_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TSendSnapshotFragmentRes success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TSendSnapshotFragmentRes.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(sendSnapshotFragment_result.class, metaDataMap);
}
public sendSnapshotFragment_result() {
}
public sendSnapshotFragment_result(
TSendSnapshotFragmentRes success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public sendSnapshotFragment_result(sendSnapshotFragment_result other) {
if (other.isSetSuccess()) {
this.success = new TSendSnapshotFragmentRes(other.success);
}
}
public sendSnapshotFragment_result deepCopy() {
return new sendSnapshotFragment_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public TSendSnapshotFragmentRes getSuccess() {
return this.success;
}
public sendSnapshotFragment_result setSuccess(@org.apache.thrift.annotation.Nullable TSendSnapshotFragmentRes success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((TSendSnapshotFragmentRes)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof sendSnapshotFragment_result)
return this.equals((sendSnapshotFragment_result)that);
return false;
}
public boolean equals(sendSnapshotFragment_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(sendSnapshotFragment_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("sendSnapshotFragment_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class sendSnapshotFragment_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public sendSnapshotFragment_resultStandardScheme getScheme() {
return new sendSnapshotFragment_resultStandardScheme();
}
}
private static class sendSnapshotFragment_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, sendSnapshotFragment_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new TSendSnapshotFragmentRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, sendSnapshotFragment_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class sendSnapshotFragment_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public sendSnapshotFragment_resultTupleScheme getScheme() {
return new sendSnapshotFragment_resultTupleScheme();
}
}
private static class sendSnapshotFragment_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, sendSnapshotFragment_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, sendSnapshotFragment_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new TSendSnapshotFragmentRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class triggerSnapshotLoad_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("triggerSnapshotLoad_args");
private static final org.apache.thrift.protocol.TField REQ_FIELD_DESC = new org.apache.thrift.protocol.TField("req", org.apache.thrift.protocol.TType.STRUCT, (short)-1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new triggerSnapshotLoad_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new triggerSnapshotLoad_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TTriggerSnapshotLoadReq req; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQ((short)-1, "req");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case -1: // REQ
return REQ;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQ, new org.apache.thrift.meta_data.FieldMetaData("req", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TTriggerSnapshotLoadReq.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(triggerSnapshotLoad_args.class, metaDataMap);
}
public triggerSnapshotLoad_args() {
}
public triggerSnapshotLoad_args(
TTriggerSnapshotLoadReq req)
{
this();
this.req = req;
}
/**
* Performs a deep copy on other.
*/
public triggerSnapshotLoad_args(triggerSnapshotLoad_args other) {
if (other.isSetReq()) {
this.req = new TTriggerSnapshotLoadReq(other.req);
}
}
public triggerSnapshotLoad_args deepCopy() {
return new triggerSnapshotLoad_args(this);
}
@Override
public void clear() {
this.req = null;
}
@org.apache.thrift.annotation.Nullable
public TTriggerSnapshotLoadReq getReq() {
return this.req;
}
public triggerSnapshotLoad_args setReq(@org.apache.thrift.annotation.Nullable TTriggerSnapshotLoadReq req) {
this.req = req;
return this;
}
public void unsetReq() {
this.req = null;
}
/** Returns true if field req is set (has been assigned a value) and false otherwise */
public boolean isSetReq() {
return this.req != null;
}
public void setReqIsSet(boolean value) {
if (!value) {
this.req = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQ:
if (value == null) {
unsetReq();
} else {
setReq((TTriggerSnapshotLoadReq)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQ:
return getReq();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQ:
return isSetReq();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof triggerSnapshotLoad_args)
return this.equals((triggerSnapshotLoad_args)that);
return false;
}
public boolean equals(triggerSnapshotLoad_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_req = true && this.isSetReq();
boolean that_present_req = true && that.isSetReq();
if (this_present_req || that_present_req) {
if (!(this_present_req && that_present_req))
return false;
if (!this.req.equals(that.req))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetReq()) ? 131071 : 524287);
if (isSetReq())
hashCode = hashCode * 8191 + req.hashCode();
return hashCode;
}
@Override
public int compareTo(triggerSnapshotLoad_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetReq(), other.isSetReq());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetReq()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.req, other.req);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("triggerSnapshotLoad_args(");
boolean first = true;
sb.append("req:");
if (this.req == null) {
sb.append("null");
} else {
sb.append(this.req);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (req != null) {
req.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class triggerSnapshotLoad_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public triggerSnapshotLoad_argsStandardScheme getScheme() {
return new triggerSnapshotLoad_argsStandardScheme();
}
}
private static class triggerSnapshotLoad_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, triggerSnapshotLoad_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case -1: // REQ
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.req = new TTriggerSnapshotLoadReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, triggerSnapshotLoad_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.req != null) {
oprot.writeFieldBegin(REQ_FIELD_DESC);
struct.req.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class triggerSnapshotLoad_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public triggerSnapshotLoad_argsTupleScheme getScheme() {
return new triggerSnapshotLoad_argsTupleScheme();
}
}
private static class triggerSnapshotLoad_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, triggerSnapshotLoad_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetReq()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetReq()) {
struct.req.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, triggerSnapshotLoad_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.req = new TTriggerSnapshotLoadReq();
struct.req.read(iprot);
struct.setReqIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class triggerSnapshotLoad_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("triggerSnapshotLoad_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new triggerSnapshotLoad_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new triggerSnapshotLoad_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable TTriggerSnapshotLoadRes success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TTriggerSnapshotLoadRes.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(triggerSnapshotLoad_result.class, metaDataMap);
}
public triggerSnapshotLoad_result() {
}
public triggerSnapshotLoad_result(
TTriggerSnapshotLoadRes success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public triggerSnapshotLoad_result(triggerSnapshotLoad_result other) {
if (other.isSetSuccess()) {
this.success = new TTriggerSnapshotLoadRes(other.success);
}
}
public triggerSnapshotLoad_result deepCopy() {
return new triggerSnapshotLoad_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public TTriggerSnapshotLoadRes getSuccess() {
return this.success;
}
public triggerSnapshotLoad_result setSuccess(@org.apache.thrift.annotation.Nullable TTriggerSnapshotLoadRes success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((TTriggerSnapshotLoadRes)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof triggerSnapshotLoad_result)
return this.equals((triggerSnapshotLoad_result)that);
return false;
}
public boolean equals(triggerSnapshotLoad_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(triggerSnapshotLoad_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("triggerSnapshotLoad_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class triggerSnapshotLoad_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public triggerSnapshotLoad_resultStandardScheme getScheme() {
return new triggerSnapshotLoad_resultStandardScheme();
}
}
private static class triggerSnapshotLoad_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, triggerSnapshotLoad_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new TTriggerSnapshotLoadRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, triggerSnapshotLoad_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class triggerSnapshotLoad_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public triggerSnapshotLoad_resultTupleScheme getScheme() {
return new triggerSnapshotLoad_resultTupleScheme();
}
}
private static class triggerSnapshotLoad_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, triggerSnapshotLoad_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, triggerSnapshotLoad_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new TTriggerSnapshotLoadRes();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy