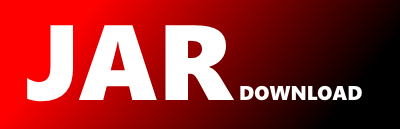
org.apache.isis.extensions.excel.testing.ExcelFixture Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.isis.extensions.excel.testing;
import java.net.URL;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.inject.Inject;
import javax.inject.Named;
import org.apache.isis.applib.annotation.DomainObject;
import org.apache.isis.applib.annotation.Programmatic;
import org.apache.isis.applib.annotation.PropertyLayout;
import org.apache.isis.applib.services.inject.ServiceInjector;
import org.apache.isis.applib.services.metamodel.BeanSort;
import org.apache.isis.applib.services.repository.RepositoryService;
import org.apache.isis.applib.value.Blob;
import org.apache.isis.commons.internal.base._Bytes;
import org.apache.isis.commons.internal.base._NullSafe;
import org.apache.isis.commons.internal.collections._Lists;
import org.apache.isis.commons.internal.collections._Maps;
import org.apache.isis.core.metamodel.spec.ObjectSpecification;
import org.apache.isis.core.metamodel.specloader.SpecificationLoader;
import org.apache.isis.extensions.excel.applib.ExcelService;
import org.apache.isis.extensions.excel.applib.IsisModuleExtExcelApplib;
import org.apache.isis.testing.fixtures.applib.fixturescripts.FixtureResultList;
import org.apache.isis.testing.fixtures.applib.fixturescripts.FixtureScript;
import lombok.Getter;
import lombok.Setter;
import lombok.val;
/**
* This class should be executed using
* {@link org.apache.isis.testing.fixtures.applib.fixturescripts.FixtureScripts.MultipleExecutionStrategy#EXECUTE_ONCE_BY_VALUE}
* (it has value semantics).
*
* @since 2.0 {@index}
*/
@Named(ExcelFixture.LOGICAL_TYPE_NAME)
@DomainObject
public class ExcelFixture extends FixtureScript {
public final static String LOGICAL_TYPE_NAME = IsisModuleExtExcelApplib.NAMESPACE + ".ExcelFixture";
@Inject SpecificationLoader specLoader;
@Inject ExcelService excelService;
private final List> classes;
public ExcelFixture(final URL excelResource, final Class>... classes) {
this(excelResource, Arrays.asList(classes));
}
public ExcelFixture(final URL excelResource, final List> classes) {
this(classes);
setExcelResource(excelResource);
}
public ExcelFixture(final Blob upload, final Class>... classes) {
this(upload, Arrays.asList(classes));
}
public ExcelFixture(final Blob blob, final List> classes) {
this(classes);
setBlob(blob);
}
private ExcelFixture(final List> classes) {
for (Class> cls : classes) {
val beanSort = Optional.ofNullable(specLoader)
.flatMap(sl->sl.specForType(cls))
.filter(_NullSafe::isPresent)
.map(ObjectSpecification::getBeanSort)
.orElse(BeanSort.UNKNOWN);
if (!beanSort.isViewModel() && !beanSort.isEntity()) {
throw new IllegalArgumentException(String.format(
"Class '%s' does not implement '%s', nor is it persistable",
cls.getSimpleName(), ExcelFixtureRowHandler.class.getSimpleName()));
}
}
this.classes = classes;
}
/**
* Input, optional: defines the name of the resource, used as a suffix to override {@link #getQualifiedName()}
* (disambiguate items when added to {@link FixtureResultList} if multiple instances of {@link ExcelFixture} are
* used with different excel spreadsheets).
*/
@Getter @Setter
@PropertyLayout(sequence = "1.1")
private String excelResourceName;
/**
* Input, mandatory ... the Excel spreadsheet to read.
*/
@Getter @Setter
@PropertyLayout(sequence = "1.2")
private URL excelResource;
/**
* Input, mandatory ... the Excel spreadsheet to read.
*/
@Getter @Setter
private Blob blob;
/**
* Output: the objects created by this fixture, for a specific persistable/row handler class.
*/
@Getter
private final Map, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy