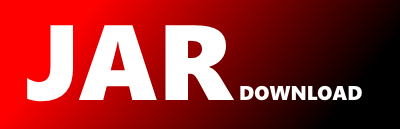
org.apache.jackrabbit.jcr2spi.hierarchy.ChildNodeEntries Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.jackrabbit.jcr2spi.hierarchy;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
import javax.jcr.ItemNotFoundException;
import javax.jcr.RepositoryException;
import org.apache.jackrabbit.spi.Name;
import org.apache.jackrabbit.spi.Path;
/**
* ChildNodeEntries
represents a collection of NodeEntry
s that
* also maintains the index values of same-name siblings on insertion and removal.
*/
public interface ChildNodeEntries {
/**
* @return true
if this ChildNodeEntries
have
* been updated or completely loaded without being invalidated in the
* mean time.
*/
boolean isComplete();
/**
* Reloads this ChildNodeEntries
object.
*
* @throws ItemNotFoundException
* @throws RepositoryException
*/
void reload() throws ItemNotFoundException, RepositoryException;
/**
* Returns an unmodifiable iterator over all NodeEntry objects present in
* this ChildNodeEntries collection irrespective of their status.
*
* @return Iterator over all NodeEntry object
*/
Iterator iterator();
/**
* Returns a List
of NodeEntry
s for the
* given nodeName
. This method does not filter out
* removed NodeEntry
s.
*
* @param nodeName the child node name.
* @return same name sibling nodes with the given nodeName
.
*/
List get(Name nodeName);
/**
* Returns the NodeEntry
with the given
* nodeName
and index
. Note, that this method
* does not filter out removed NodeEntry
s.
*
* @param nodeName name of the child node entry.
* @param index the index of the child node entry.
* @return the NodeEntry
or null
if there
* is no such NodeEntry
.
*/
NodeEntry get(Name nodeName, int index);
/**
* Return the NodeEntry
that matches the given nodeName and
* uniqueID or null
if no matching entry can be found.
*
* @param nodeName
* @param uniqueID
* @return
* @throws IllegalArgumentException if the given uniqueID is null.
*/
NodeEntry get(Name nodeName, String uniqueID);
/**
* Adds a NodeEntry
to the end of the list. Same as
* {@link #add(NodeEntry, int)}, where the index is {@link Path#INDEX_UNDEFINED}.
*
* @param cne the NodeEntry
to add.
*/
void add(NodeEntry cne);
/**
* Adds a NodeEntry
.
* Note the following special cases:
*
* - If an entry with the given index already exists, the the new sibling
* is inserted before.
* - If the given index is bigger that the last entry in the siblings list,
* intermediate entries will be created.
*
*
* @param cne the NodeEntry
to add.
*/
void add(NodeEntry cne, int index);
/**
* Adds a the new NodeEntry
before beforeEntry
.
*
* @param entry
* @param index
* @param beforeEntry
*/
void add(NodeEntry entry, int index, NodeEntry beforeEntry);
/**
* Removes the child node entry referring to the node state.
*
* @param childEntry the entry to be removed.
* @return the removed entry or null
if there is no such entry.
*/
NodeEntry remove(NodeEntry childEntry);
/**
* Reorders an existing NodeEntry
before another
* NodeEntry
. If beforeEntry
is
* null
insertEntry
is moved to the end of the
* child node entries.
*
* @param insertEntry the NodeEntry to move.
* @param beforeEntry the NodeEntry where insertEntry
is
* reordered to.
* @return the NodeEntry that followed the 'insertEntry' before the reordering.
* @throws NoSuchElementException if insertEntry
or
* beforeEntry
does not have a NodeEntry
* in this ChildNodeEntries
.
*/
NodeEntry reorder(NodeEntry insertEntry, NodeEntry beforeEntry);
/**
* Reorders an existing NodeEntry
after another
* NodeEntry
. If afterEntry
is
* null
insertEntry
is moved to the beginning of
* the child node entries.
*
* @param insertEntry the NodeEntry to move.
* @param afterEntry the NodeEntry where insertEntry
is
* reordered behind.
* @throws NoSuchElementException if insertEntry
or
* afterEntry
does not have a NodeEntry
* in this ChildNodeEntries
.
*/
void reorderAfter(NodeEntry insertEntry, NodeEntry afterEntry);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy