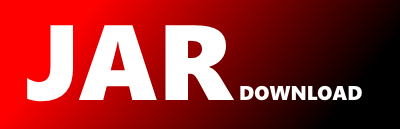
org.apache.jackrabbit.jcr2spi.hierarchy.NodeEntry Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.jackrabbit.jcr2spi.hierarchy;
import org.apache.jackrabbit.jcr2spi.state.NodeState;
import org.apache.jackrabbit.spi.ChildInfo;
import org.apache.jackrabbit.spi.Name;
import org.apache.jackrabbit.spi.Path;
import org.apache.jackrabbit.spi.NodeId;
import org.apache.jackrabbit.spi.QNodeDefinition;
import org.apache.jackrabbit.spi.QPropertyDefinition;
import org.apache.jackrabbit.spi.Event;
import org.apache.jackrabbit.spi.QValue;
import javax.jcr.ItemExistsException;
import javax.jcr.RepositoryException;
import javax.jcr.PathNotFoundException;
import javax.jcr.ItemNotFoundException;
import javax.jcr.InvalidItemStateException;
import java.util.Iterator;
import java.util.List;
import java.util.Collection;
/**
* NodeEntry
...
*/
public interface NodeEntry extends HierarchyEntry {
/**
* @return the NodeId
of this child node entry.
*/
public NodeId getId() throws InvalidItemStateException, RepositoryException;
/**
* Returns the ID that must be used for resolving this entry OR loading its
* children entries from the persistent layer. This is the same as
* getId()
unless this entry or any of its ancestors has been
* transiently moved.
*
* @return
* @see #getId()
*/
public NodeId getWorkspaceId() throws InvalidItemStateException, RepositoryException;
/**
* @return the unique ID of the node state which is referenced by this
* child node entry or null
if the node state cannot be
* identified with a unique ID.
*/
public String getUniqueID();
/**
*
* @param uniqueID
*/
public void setUniqueID(String uniqueID);
/**
* @return the index of this child node entry to support same-name siblings.
* If the index of this entry cannot be determined
* {@link org.apache.jackrabbit.spi.Path#INDEX_UNDEFINED} is returned.
* @throws InvalidItemStateException
* @throws RepositoryException
*/
public int getIndex() throws InvalidItemStateException, RepositoryException;
/**
* @return the referenced NodeState
.
* @throws ItemNotFoundException if the NodeState
does not
* exist.
* @throws RepositoryException If an error occurs while retrieving the
* NodeState
.
*/
public NodeState getNodeState() throws ItemNotFoundException, RepositoryException;
/**
* Traverse the tree below this entry and return the child entry matching
* the given path. If that entry has not been loaded yet, try to do so.
* NOTE: In contrast to getNodeEntry, getNodeEntries this method may return
* invalid entries, i.e. entries connected to a removed or stale ItemState.
*
* @param path
* @return the entry at the given path.
* @throws PathNotFoundException
* @throws RepositoryException
*/
public NodeEntry getDeepNodeEntry(Path path) throws PathNotFoundException, RepositoryException;
/**
* Traverse the tree below this entry and return the child entry matching
* the given path. If that entry has not been loaded yet, try to do so.
* NOTE: In contrast to getPropertyEntry and getPropertyEntries this method
* may return invalid entries, i.e. entries connected to a removed or stale
* ItemState.
*
* @param path
* @return the property entry at the given path.
* @throws PathNotFoundException
* @throws RepositoryException
*/
public PropertyEntry getDeepPropertyEntry(Path path) throws PathNotFoundException, RepositoryException;
/**
* Traverse the tree below this entry and return the child entry matching
* the given 'workspacePath', i.e. transient modifications and new entries
* are ignored.
* If no matching entry can be found, null
is return.
*
* @param workspacePath
* @return matching entry or null
.
*/
public HierarchyEntry lookupDeepEntry(Path workspacePath);
/**
* Determines if there is a valid NodeEntry
with the
* specified nodeName
.
*
* @param nodeName Name
object specifying a node name
* @return true
if there is a NodeEntry
with
* the specified nodeName
.
*/
public boolean hasNodeEntry(Name nodeName);
/**
* Determines if there is a valid NodeEntry
with the
* specified name
and index
.
*
* @param nodeName Name
object specifying a node name.
* @param index 1-based index if there are same-name child node entries.
* @return true
if there is a NodeEntry
with
* the specified name
and index
.
*/
public boolean hasNodeEntry(Name nodeName, int index);
/**
* Returns the valid NodeEntry
with the specified name
* and index or null
if there's no matching entry.
*
* @param nodeName Name
object specifying a node name.
* @param index 1-based index if there are same-name child node entries.
* @return The NodeEntry
with the specified name and index
* or null
if there's no matching entry.
* @throws RepositoryException If an unexpected error occurs.
*/
public NodeEntry getNodeEntry(Name nodeName, int index) throws RepositoryException;
/**
* Returns the valid NodeEntry
with the specified name
* and index or null
if there's no matching entry. If
* loadIfNotFound
is true, the implementation must make
* sure, that it's list of child entries is up to date and eventually
* try to load the node entry.
*
* @param nodeName Name
object specifying a node name.
* @param index 1-based index if there are same-name child node entries.
* @param loadIfNotFound
* @return The NodeEntry
with the specified name and index
* or null
if there's no matching entry.
* @throws RepositoryException If an unexpected error occurs.
*/
public NodeEntry getNodeEntry(Name nodeName, int index, boolean loadIfNotFound) throws RepositoryException;
/**
* Returns a unmodifiable iterator of NodeEntry
objects
* denoting the the valid child NodeEntries present on this NodeEntry
.
*
* @return iterator of NodeEntry
objects
* @throws RepositoryException If an unexpected error occurs.
*/
public Iterator getNodeEntries() throws RepositoryException;
/**
* Returns a unmodifiable List of NodeEntry
s with the
* specified name.
*
* @param nodeName name of the child node entries that should be returned
* @return list of NodeEntry
objects
* @throws RepositoryException If an unexpected error occurs.
*/
public List getNodeEntries(Name nodeName) throws RepositoryException;
/**
* Creates or updates the ChildNodeEntries
of this node.
*
* @param childInfos
* @throws RepositoryException
*/
public void setNodeEntries(Iterator childInfos) throws RepositoryException;
/**
* Adds a child NodeEntry to this entry if it not yet present with this
* node entry.
*
* @param nodeName
* @param index
* @param uniqueID
* @return the NodeEntry
.
* @throws RepositoryException If an unexpected error occurs.
*/
public NodeEntry getOrAddNodeEntry(Name nodeName, int index, String uniqueID) throws RepositoryException;
/**
* Adds a new, transient child NodeEntry
*
* @param nodeName
* @param uniqueID
* @param primaryNodeType
* @param definition
* @return
* @throws RepositoryException If an error occurs.
*/
public NodeEntry addNewNodeEntry(Name nodeName, String uniqueID, Name primaryNodeType, QNodeDefinition definition) throws RepositoryException;
/**
* Determines if there is a property entry with the specified Name
.
*
* @param propName Name
object specifying a property name
* @return true
if there is a property entry with the specified
* Name
.
*/
public boolean hasPropertyEntry(Name propName);
/**
* Returns the valid PropertyEntry
with the specified name
* or null
if no matching entry exists.
*
* @param propName Name
object specifying a property name.
* @return The PropertyEntry
with the specified name or
* null
if no matching entry exists.
* @throws RepositoryException If an unexpected error occurs.
*/
public PropertyEntry getPropertyEntry(Name propName) throws RepositoryException;
/**
* Returns the valid PropertyEntry
with the specified name
* or null
if no matching entry exists. If
* loadIfNotFound
is true, the implementation must make
* sure, that it's list of property entries is up to date and eventually
* try to load the property entry with the given name.
*
* @param propName Name
object specifying a property name.
* @param loadIfNotFound
* @return The PropertyEntry
with the specified name or
* null
if no matching entry exists.
* @throws RepositoryException If an unexpected error occurs.
*/
public PropertyEntry getPropertyEntry(Name propName, boolean loadIfNotFound) throws RepositoryException;
/**
* Returns an unmodifiable Iterator over those children that represent valid
* PropertyEntries.
*
* @return an unmodifiable Iterator over those children that represent valid
* PropertyEntries.
*/
public Iterator getPropertyEntries();
/**
* Add an existing PropertyEntry
with the given name if it is
* not yet contained in this NodeEntry
.
* Please note the difference to {@link #addNewPropertyEntry(Name, QPropertyDefinition, QValue[], int)}
* which adds a new, transient entry.
*
* @param propName
* @return the PropertyEntry
* @throws ItemExistsException if a child item exists with the given name
* @throws RepositoryException if an unexpected error occurs.
*/
public PropertyEntry getOrAddPropertyEntry(Name propName) throws ItemExistsException, RepositoryException;
/**
* Adds property entries for the given Name
s. It depends on
* the status of this NodeEntry
, how conflicts are resolved
* and whether or not existing entries that are missing in the iterator
* get removed.
*
* @param propNames
* @throws ItemExistsException
* @throws RepositoryException if an unexpected error occurs.
*/
public void setPropertyEntries(Collection propNames) throws ItemExistsException, RepositoryException;
/**
* Add a new, transient PropertyEntry
to this NodeEntry
* and return the PropertyState
associated with the new entry.
*
* @param propName
* @param definition
* @param values
* @param propertyType
* @return the new entry.
* @throws ItemExistsException
* @throws RepositoryException
*/
public PropertyEntry addNewPropertyEntry(Name propName, QPropertyDefinition definition, QValue[] values, int propertyType) throws ItemExistsException, RepositoryException;
/**
* Reorders this NodeEntry before the sibling entry specified by the given
* beforeEntry
.
*
* @param beforeEntry the child node where to insert the node before. If
* null
this entry is moved to the end of its parents child node entries.
* @throws RepositoryException If an unexpected error occurs.
*/
public void orderBefore(NodeEntry beforeEntry) throws RepositoryException;
/**
* Moves this NodeEntry
as new child entry of the
* NodeEntry
identified by newParent
and/or renames
* it to newName
. If transientMove
is true, an
* implementation must make sure, that reverting this modification by calling
* {@link HierarchyEntry#revert()} on the common ancestor of both parents
* moves this NodeEntry back and resets the name to its original value.
*
* @param newName
* @param newParent
* @return the moved entry
* @throws RepositoryException If the entry to be moved is not a child of this
* NodeEntry or if an unexpected error occurs.
*/
public NodeEntry move(Name newName, NodeEntry newParent, boolean transientMove) throws RepositoryException;
/**
* @return true if this NodeEntry
is transiently moved.
*/
public boolean isTransientlyMoved();
/**
* The parent entry of a external event gets informed about the modification.
* Note, that {@link Event#getParentId()} of the given childEvent must point
* to this NodeEntry
.
*
* @param childEvent
*/
public void refresh(Event childEvent) ;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy