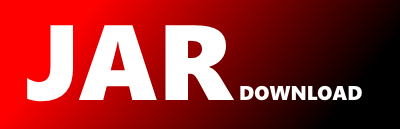
org.apache.jackrabbit.jcr2spi.state.ItemStateFactory Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.jackrabbit.jcr2spi.state;
import org.apache.jackrabbit.spi.ChildInfo;
import org.apache.jackrabbit.spi.NodeId;
import org.apache.jackrabbit.spi.PropertyId;
import org.apache.jackrabbit.spi.Name;
import org.apache.jackrabbit.jcr2spi.hierarchy.NodeEntry;
import org.apache.jackrabbit.jcr2spi.hierarchy.PropertyEntry;
import javax.jcr.RepositoryException;
import javax.jcr.ItemNotFoundException;
import java.util.Iterator;
/**
* ItemStateFactory
provides methods to create child
* NodeState
s and PropertyState
s for a given
* NodeState
.
*/
public interface ItemStateFactory {
/**
* @param entry
* @return
* @throws ItemNotFoundException
* @throws RepositoryException
*/
public NodeState createRootState(NodeEntry entry) throws ItemNotFoundException, RepositoryException;
/**
* Creates the child NodeState
with the given
* nodeId
.
*
* @param nodeId the id of the NodeState
to create.
* @param entry the HierarchyEntry
the new state should
* be attached to.
* @return the created NodeState
.
* @throws ItemNotFoundException if there is no such NodeState
.
* @throws RepositoryException if an error occurs while retrieving the NodeState
.
*/
public NodeState createNodeState(NodeId nodeId, NodeEntry entry)
throws ItemNotFoundException, RepositoryException;
/**
* Tries to retrieve the NodeState
with the given NodeId
* and if the state exists, fills in the NodeEntries missing between the
* last known NodeEntry marked by anyParent
.
*
* @param nodeId
* @param anyParent
* @return the created NodeState
.
* @throws ItemNotFoundException if there is no such NodeState
.
* @throws RepositoryException if an error occurs while retrieving the NodeState
.
*/
public NodeState createDeepNodeState(NodeId nodeId, NodeEntry anyParent)
throws ItemNotFoundException, RepositoryException;
/**
* Creates the PropertyState
with the given
* propertyId
.
*
* @param propertyId the id of the PropertyState
to create.
* @param entry the HierarchyEntry
the new state should
* be attached to.
* @return the created PropertyState
.
* @throws ItemNotFoundException if there is no such PropertyState
.
* @throws RepositoryException if an error occurs while retrieving the
* PropertyState
.
*/
public PropertyState createPropertyState(PropertyId propertyId, PropertyEntry entry)
throws ItemNotFoundException, RepositoryException;
/**
* Tries to retrieve the PropertyState
with the given PropertyId
* and if the state exists, fills in the HierarchyEntries missing between the
* last known NodeEntry marked by anyParent
.
*
* @param propertyId
* @param anyParent
* @return
* @throws ItemNotFoundException if there is no such NodeState
.
* @throws RepositoryException if an error occurs while retrieving the NodeState
.
*/
public PropertyState createDeepPropertyState(PropertyId propertyId, NodeEntry anyParent) throws ItemNotFoundException, RepositoryException;
/**
* Returns an Iterator over ChildInfo
s for the given NodeState
.
*
* @param nodeId
* @throws ItemNotFoundException
* @throws RepositoryException
*/
public Iterator getChildNodeInfos(NodeId nodeId) throws ItemNotFoundException, RepositoryException;
/**
* Returns the identifiers of all reference properties that point to
* the given node.
*
* @param nodeState reference target
* @param propertyName
* @param weak Boolean flag indicating whether weak references should be
* returned or not.
* @return reference property identifiers
*/
public Iterator getNodeReferences(NodeState nodeState, Name propertyName, boolean weak);
/**
* Adds the given ItemStateCreationListener
.
*
* @param listener
*/
public void addCreationListener(ItemStateCreationListener listener);
/**
* Removes the given ItemStateCreationListener
.
*
* @param listener
*/
public void removeCreationListener(ItemStateCreationListener listener);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy