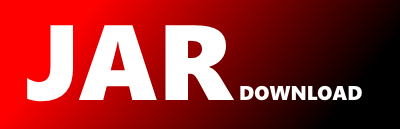
org.apache.jackrabbit.oak.segment.SegmentBufferWriterPool Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.jackrabbit.oak.segment;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.google.common.base.Preconditions.checkState;
import static com.google.common.collect.Lists.newArrayList;
import static com.google.common.collect.Maps.newHashMap;
import static com.google.common.collect.Sets.newHashSet;
import static java.lang.Thread.currentThread;
import java.io.IOException;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.annotation.Nonnull;
import com.google.common.base.Supplier;
import com.google.common.util.concurrent.Monitor;
import com.google.common.util.concurrent.Monitor.Guard;
/**
* This {@link WriteOperationHandler} uses a pool of {@link SegmentBufferWriter}s,
* which it passes to its {@link #execute(WriteOperation) execute} method.
*
* Instances of this class are thread safe. See also the class comment of
* {@link SegmentWriter}.
*/
public class SegmentBufferWriterPool implements WriteOperationHandler {
/**
* Monitor protecting the state of this pool. Neither of {@link #writers},
* {@link #borrowed} and {@link #disposed} must be modified without owning
* this monitor.
*/
private final Monitor poolMonitor = new Monitor(true);
/**
* Pool of current writers that are not in use
*/
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy